How Excessive Parameters Complicate Java Method Maintenance
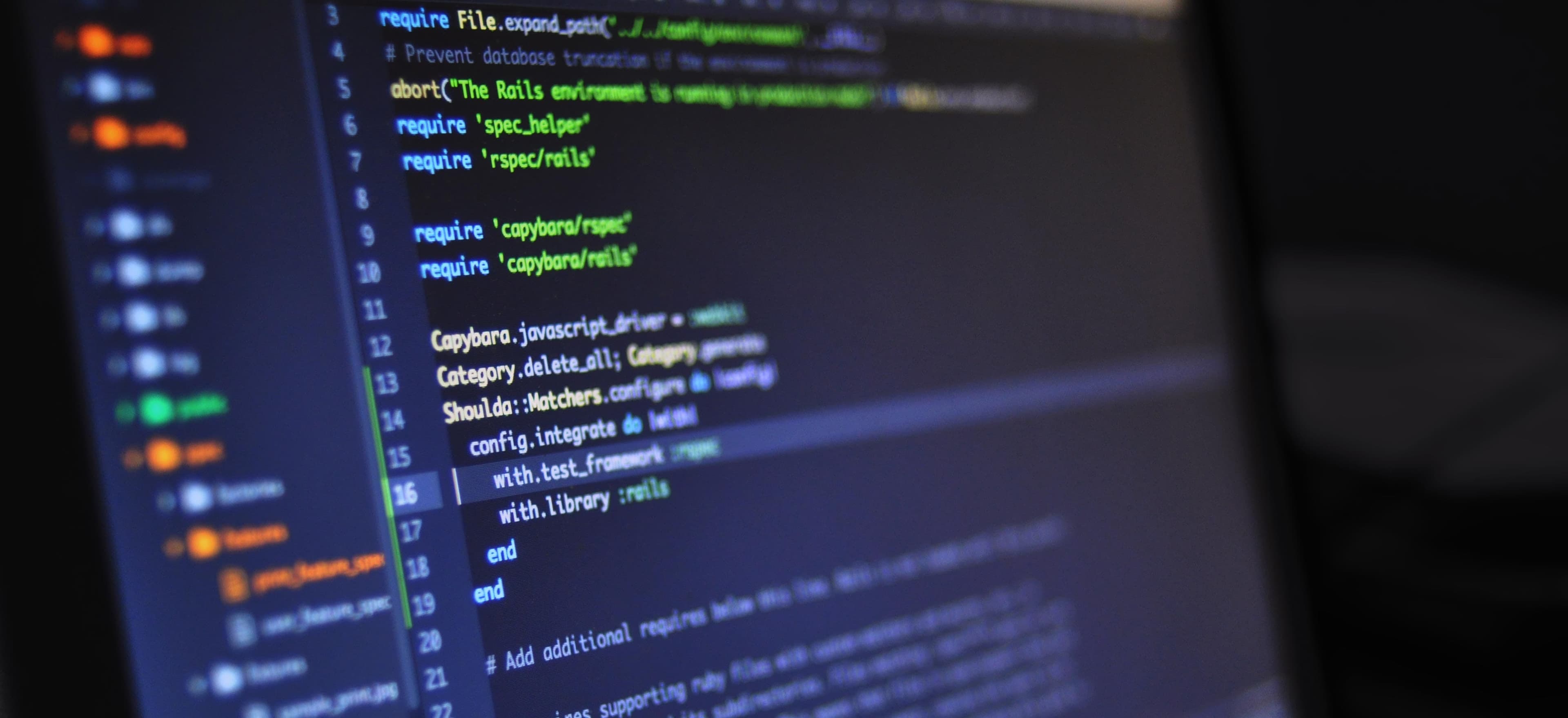
- Published on
How Excessive Parameters Complicate Java Method Maintenance
In Java programming, methods serve as the backbone of modular design. They allow for reusability and division of tasks. However, when methods start to accumulate excessive parameters, maintenance can quickly become a headache. This blog post delves into the complications and consequences of excessive parameters in Java methods, while providing practical tips and solutions to enhance code maintainability.
Understanding Method Parameters
What Are Parameters?
Parameters in Java are variables that are passed to methods as input. They allow methods to perform their functions based on dynamic data provided at runtime. Here is a simple example of a method with parameters:
public int calculateArea(int length, int width) {
return length * width;
}
In this example, length
and width
are parameters that define the inputs for the method calculateArea
.
Why Parameter Count Matters
While parameters are essential for method functionality, there is an optimal number of parameters a method should have. Best practices usually recommend limiting the number of parameters to three or four. Excessive parameters lead to the following complications:
- Decreased Readability: A method with too many parameters is often challenging to understand at a glance.
- Increased Error Potential: More parameters often mean a higher chance of mixing up values during method calls.
- Difficult Maintenance: Adding or removing parameters over time can introduce bugs, especially in legacy systems.
The Dangers of Excessive Parameters
1. Decreased Readability
Methods with many parameters can quickly turn into a wall of text with little explanation. For instance, consider a method with six parameters:
public void processOrder(String customerName, int orderId, double orderAmount, String shippingAddress, boolean expressShipping, String paymentMethod) {
// processing code
}
At first glance, it's hard to determine what this method actually does. In contrast, a method with clearly defined parameters or a new structure is easier to read and comprehend.
2. Increased Error Potential
Passing multiple parameters increases the likelihood of mistakes. A common pitfall is calling the method with values in the wrong order:
processOrder("John Doe", 12345, 50.99, "123 Elm St", true, "credit card");
Imagine if the shippingAddress
and paymentMethod
were swapped. Unintended consequences could arise, such as delays in processing.
3. Difficult Maintenance
When methods need to evolve — maybe to accommodate new requirements — adjusting the parameters can have ripple effects throughout your codebase. Consider this scenario:
public void updateOrder(String customerName, int orderId, double orderAmount, String shippingAddress, boolean expressShipping, String paymentMethod, String discountCode) {
// updating code
}
Adding a discountCode
may seem harmless, but any existing calls to updateOrder
must now be refactored. This increases workload and potential for errors.
Strategies for Managing Parameter Excess
1. Use Parameter Objects
Instead of passing numerous parameters individually, consider creating a bespoke object that encapsulates these parameters. This is known as a parameter object. For example:
class OrderDetails {
String customerName;
int orderId;
double orderAmount;
String shippingAddress;
boolean expressShipping;
String paymentMethod;
// Constructor and Getters/Setters
}
You can rewrite the method as follows:
public void processOrder(OrderDetails orderDetails) {
// processing code using orderDetails
}
2. Builder Pattern
For configurations that may require multiple optional parameters, the Builder pattern is beneficial. This pattern allows flexibility without overwhelming the method signatures. Here's an example:
public class Order {
private final String customerName;
private final double orderAmount;
private final String shippingAddress;
private final boolean expressShipping;
private Order(Builder builder) {
this.customerName = builder.customerName;
this.orderAmount = builder.orderAmount;
this.shippingAddress = builder.shippingAddress;
this.expressShipping = builder.expressShipping;
}
public static class Builder {
private String customerName;
private double orderAmount;
private String shippingAddress;
private boolean expressShipping;
public Builder(String customerName, double orderAmount) {
this.customerName = customerName;
this.orderAmount = orderAmount;
}
public Builder shippingAddress(String shippingAddress) {
this.shippingAddress = shippingAddress;
return this;
}
public Builder expressShipping(boolean expressShipping) {
this.expressShipping = expressShipping;
return this;
}
public Order build() {
return new Order(this);
}
}
}
You can create orders seamlessly:
Order order = new Order.Builder("John Doe", 50.99)
.shippingAddress("123 Elm St")
.expressShipping(true)
.build();
3. Method Overloading
Sometimes, a method can be overloaded based on different parameter needs. Here's an example of overloaded methods for different shipping methods:
public void processOrder(String customerName, int orderId) {
// Basic order processing
}
public void processOrder(String customerName, int orderId, boolean expressShipping) {
// Express order processing
}
Overloading allows you to tailor how methods are called, reducing the number of parameters in each individual method.
The Last Word
Excessive parameters can lead to drastically challenging code maintenance scenarios in Java. By ensuring that methods are concise, clear, and manageable, you not only improve readability but also make your codebase less error-prone and far easier to maintain.
Strategies such as utilizing parameter objects, employing the Builder pattern, and leveraging method overloading are essential takeaways for any Java developer looking to enhance their code quality. With these effective coding strategies, you can keep your Java projects clean, maintainable, and enjoyable to work with.
For further reading on Java best practices, you may explore Effective Java by Joshua Bloch or research design patterns on Refactoring Guru.
Remember to focus on simplicity and clarity in your code as you continue exploring the expansive world of Java programming. Happy coding!
Checkout our other articles