Simplifying Complex Switch Cases in Java 12
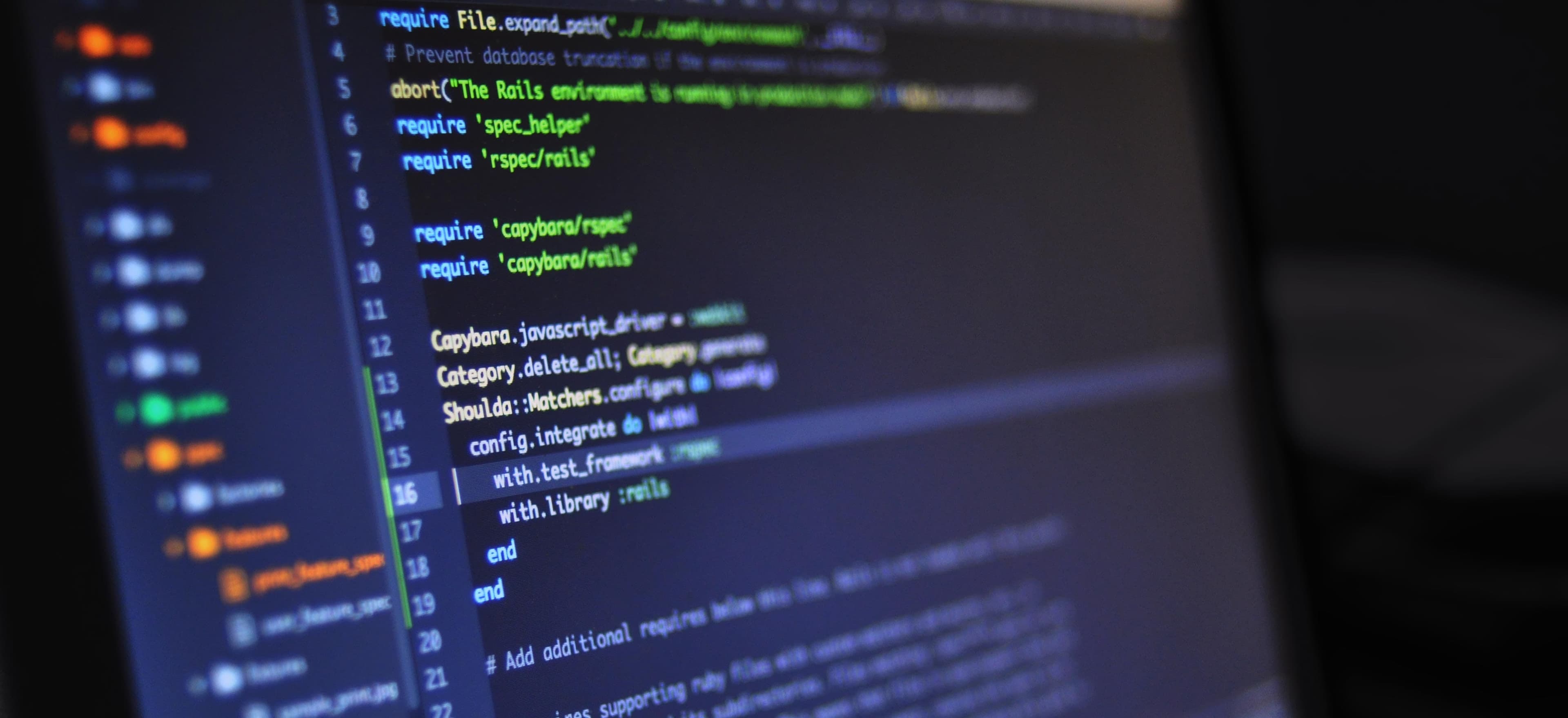
- Published on
Simplifying Complex Switch Cases in Java 12
Java is renowned for its rich feature set and strong community support. One significant enhancement introduced in Java 12 is the new switch expression. This feature not only helps streamline switch statements but also promotes cleaner and more maintainable code. In this article, we will explore the complexities of traditional switch cases, then discover how Java 12 simplifies this with its intuitive switch expressions.
Understanding Traditional Switch Statements
Before Java 12, switch statements in Java were primarily statements, which could only execute code and not return values. Below is a traditional switch statement:
int dayOfWeek = 3;
String day;
switch (dayOfWeek) {
case 1:
day = "Monday";
break;
case 2:
day = "Tuesday";
break;
case 3:
day = "Wednesday";
break;
case 4:
day = "Thursday";
break;
case 5:
day = "Friday";
break;
case 6:
day = "Saturday";
break;
case 7:
day = "Sunday";
break;
default:
day = "Invalid day";
break;
}
System.out.println(day);
Analysis of the Problem
While the above code works correctly, it has several drawbacks:
- Verbosity: The code is lengthy, requiring a lot of repetitive "case" entries.
- Error-Prone: Missing a break statement can lead to fall-through errors, unexpectedly executing code in subsequent cases.
- Not Returnable: Traditional switch statements can't return values directly, making the code less elegant.
What Is a Switch Expression?
With the introduction of switch expressions, Java 12 remedies these issues. A switch expression can return a value and is syntactically more straightforward.
Here is how the previous code can be rewritten using a switch expression:
int dayOfWeek = 3;
String day = switch (dayOfWeek) {
case 1 -> "Monday";
case 2 -> "Tuesday";
case 3 -> "Wednesday";
case 4 -> "Thursday";
case 5 -> "Friday";
case 6 -> "Saturday";
case 7 -> "Sunday";
default -> "Invalid day";
};
System.out.println(day);
Key Features of Switch Expressions
-
Conciseness: The syntax is much cleaner. The
->
operator (arrow) denotes the return statement, removing the need forbreak
. -
Returning Values: The switch expression can directly assign the resultant value to a variable.
-
Enhanced Control Flow: Using
yield
, we can add more complex operations within the cases without sacrificing conciseness.
Implementing Multi-Line Blocks
Sometimes, a case may require executing multiple lines of code. Here’s how to handle such scenarios with switch expressions using a block:
int dayOfWeek = 5;
String day = switch (dayOfWeek) {
case 1 -> "Monday";
case 2 -> "Tuesday";
case 3 -> "Wednesday";
case 4 -> {
// Multiple statements
String dayName = "Thursday";
yield dayName; // yield is used to return value from a case block
}
case 5 -> {
String dayName = "Friday";
yield dayName;
}
case 6 -> "Saturday";
case 7 -> "Sunday";
default -> "Invalid day";
};
System.out.println(day);
Why Use Switch Expressions?
-
Readability: The clear and concise syntax improves readability, leading to better maintainability.
-
Structural Clarity: The use of an arrow (
->
) distinguishes cases clearly from one another. -
Reduction of Errors: By eliminating the fall-through effect, the risk of bugs is significantly reduced.
-
Versatility: Switch expressions can also return values for various data types, including
String
,int
, or enums.
Example Use Case: Traffic Light System
To illustrate the practical application of switch expressions, let’s create a simple traffic light system:
public enum TrafficLight {
RED, YELLOW, GREEN
}
public class TrafficLightSystem {
public static void main(String[] args) {
TrafficLight light = TrafficLight.YELLOW;
String action = switch (light) {
case RED -> "Stop";
case YELLOW -> "Caution";
case GREEN -> "Go";
};
System.out.println(action);
}
}
Commentary
-
Enum Usage: The use of enums for the
TrafficLight
makes the code more manageable and clearer in intent. -
Direct Mapping: The switch expression provides a direct mapping between traffic lights and their corresponding actions without verbose code blocks.
Lessons Learned
Java 12's switch expressions represent a monumental shift in how Java developers can manage conditional statements. By simplifying complex conditions, improving readability, and reducing code length, they empower developers to write clearer, more maintainable code.
Further Reading
To learn more about switch expressions and other features in Java 12, refer to the Java 12 Documentation and the Oracle Java Tutorials.
By embracing these modern approaches, developers can enhance their productivity and harness the full power of the Java programming language. Happy coding!
Checkout our other articles