Common Mistakes to Avoid When Using JdbcTemplate in Spring
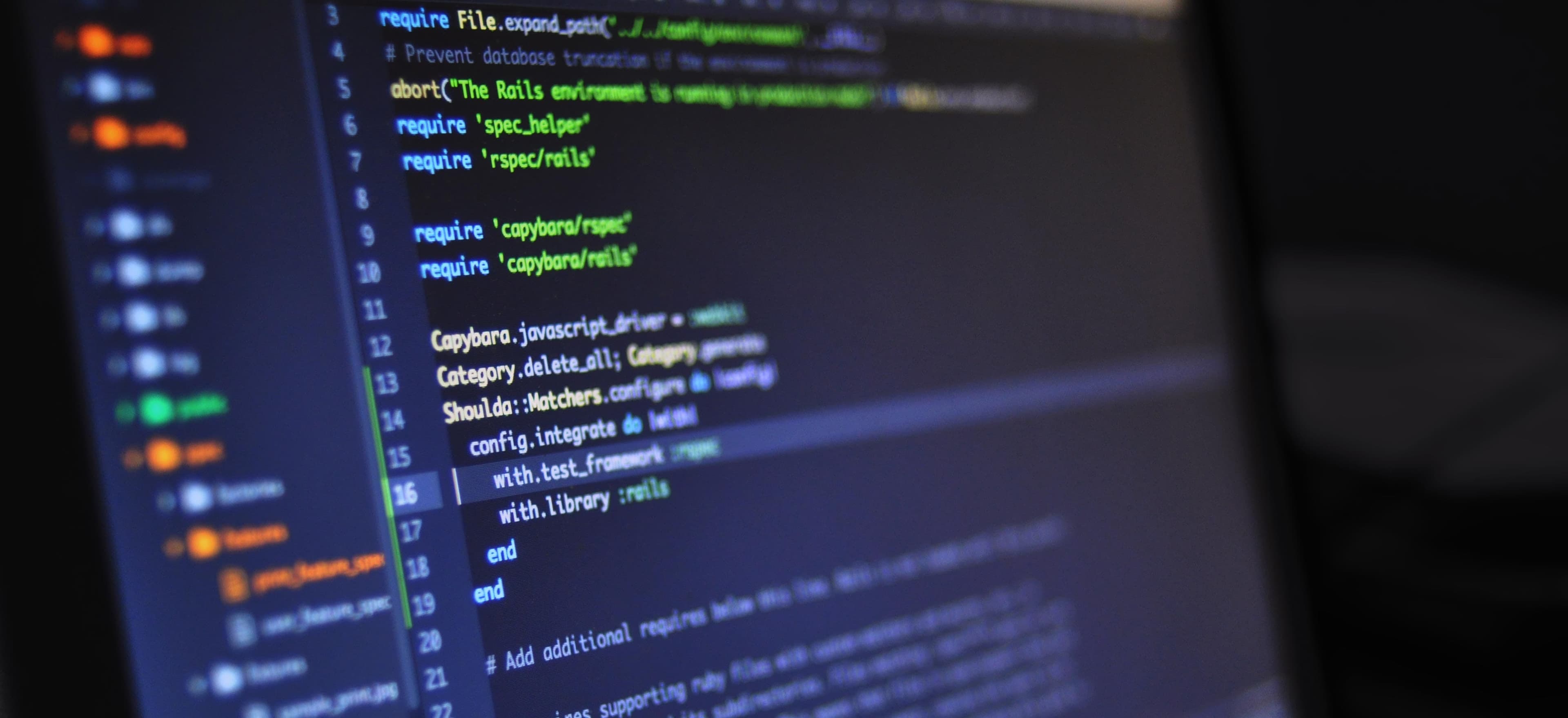
- Published on
Common Mistakes to Avoid When Using JdbcTemplate in Spring
Spring Framework has revolutionized the way developers handle database interactions. One of the shining stars in this framework is JdbcTemplate
. It simplifies data access and offers a clean and efficient way to manage the complexities of JDBC. However, as with any powerful tool, it’s easy to make mistakes. In this post, we’ll explore some common pitfalls when using JdbcTemplate
and how to avoid them to ensure your application remains robust and maintainable.
Understanding JdbcTemplate
Before we dive into the common mistakes, it's essential to have a basic understanding of what JdbcTemplate
is. JdbcTemplate
is a central class in the Spring JDBC framework that simplifies the use of JDBC by handling the creation and release of resources, as well as exception handling.
Here's a simple example of using JdbcTemplate
to query data:
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
@Repository
public class UserRepository {
@Autowired
private JdbcTemplate jdbcTemplate;
public User findUserById(int userId) {
String sql = "SELECT * FROM users WHERE id = ?";
return jdbcTemplate.queryForObject(sql, new Object[]{userId}, new UserRowMapper());
}
}
In the example above:
JdbcTemplate
is injected into the repository class.- The
queryForObject
method retrieves a user by ID.
Common Mistakes
1. Ignoring Resource Management
One of the primary benefits of using JdbcTemplate
is automated resource management. However, many developers still create and close their JDBC connections manually.
The Mistake: Opening connections manually can lead to resource leaks.
The Solution: Always leverage JdbcTemplate
methods to manage resources automatically. For instance:
public void saveUser(User user) {
String sql = "INSERT INTO users (name, email) VALUES (?, ?)";
jdbcTemplate.update(sql, user.getName(), user.getEmail());
}
In this example, the connection opens and closes automatically, ensuring no resources are leaked.
2. Inadequate Error Handling
JDBC operations can produce various exceptions, and failing to handle these appropriately can lead to unresponsive applications.
The Mistake: Swallowing exceptions without logging or handling them can make debugging difficult.
The Solution: Use Spring's exception translation feature. Spring converts SQL exceptions into a more manageable hierarchy of exceptions. For example:
public User findUserById(int userId) {
try {
String sql = "SELECT * FROM users WHERE id = ?";
return jdbcTemplate.queryForObject(sql, new Object[]{userId}, new UserRowMapper());
} catch (DataAccessException e) {
// Log the exception and handle accordingly
throw new CustomDataAccessException("User not found", e);
}
}
In this code snippet, we catch the DataAccessException
, allowing us to log and handle the error gracefully.
3. Using Hardcoded SQL Statements
While writing raw SQL directly in your code might seem straightforward, it can lead to issues in maintainability and readability.
The Mistake: Hardcoding SQL strings makes updates and changes cumbersome.
The Solution: Use constants or externalize your SQL into properties files. For example:
public class UserRepository {
private static final String INSERT_USER_SQL = "INSERT INTO users (name, email) VALUES (?, ?)";
public void saveUser(User user) {
jdbcTemplate.update(INSERT_USER_SQL, user.getName(), user.getEmail());
}
}
Alternatively, consider using Spring's support for named parameters or building your SQL queries dynamically with methods like SqlBuilder
for more complex scenarios.
4. Not Using Prepared Statements
Whenever you are writing SQL queries, it's tempting to concatenate strings and create SQL dynamically. This approach introduces security risks such as SQL injection.
The Mistake: Using string concatenation in SQL queries.
The Solution: Always use placeholders with JdbcTemplate
. Here’s an example:
public User findByEmail(String email) {
String sql = "SELECT * FROM users WHERE email = ?";
return jdbcTemplate.queryForObject(sql, new Object[]{email}, new UserRowMapper());
}
Using placeholders makes sure that user input is treated as data rather than executable code, preventing SQL injection attacks.
5. Failure to Minimize Database Calls
Executing multiple database calls can significantly slow down your application if not handled properly.
The Mistake: Making multiple calls to the database instead of batching requests.
The Solution: Use batch operations when applicable. For example:
public void saveMultipleUsers(List<User> users) {
String sql = "INSERT INTO users (name, email) VALUES (?, ?)";
List<Object[]> batchArgs = new ArrayList<>();
for (User user : users) {
batchArgs.add(new Object[]{user.getName(), user.getEmail()});
}
jdbcTemplate.batchUpdate(sql, batchArgs);
}
Batching inserts or updates can drastically reduce the number of database trips, improving performance.
6. Not Using RowMapper Efficiently
RowMapper
is a powerful feature of JdbcTemplate
, but many developers don't utilize it effectively, leading to cumbersome and untested code.
The Mistake: Using anonymous inner classes for RowMapper
repeatedly can clutter the code.
The Solution: Create specific implementations of RowMapper
for clarity.
Example:
public class UserRowMapper implements RowMapper<User> {
@Override
public User mapRow(ResultSet rs, int rowNum) throws SQLException {
User user = new User();
user.setId(rs.getInt("id"));
user.setName(rs.getString("name"));
user.setEmail(rs.getString("email"));
return user;
}
}
This keeps your main logic clean and your mapping logic separate.
7. Not Leveraging the Power of Spring Transactions
Managing transactions is essential for maintaining data integrity. Failing to use Spring's transaction management can lead to inconsistent states.
The Mistake: Not using transactions for multiple database operations.
The Solution: Use @Transactional
annotation to manage transactions easily.
import org.springframework.transaction.annotation.Transactional;
@Transactional
public void transferFunds(int fromAccountId, int toAccountId, double amount) {
withdraw(fromAccountId, amount);
deposit(toAccountId, amount);
}
In this case, if any operation fails, the entire transaction rolls back, preserving the integrity of your data.
Final Considerations
Using JdbcTemplate
in Spring can bring immense benefits to your data access layer when used correctly. Avoiding these common mistakes will help you build a more robust, maintainable, and secure application.
For more information about using Spring JDBC, consider visiting Spring's official documentation.
Remember, the key to successful application development lies in understanding the tools you’re using and applying best practices consistently. Happy coding!
Checkout our other articles