Mastering Java 8: Avoiding Common Language Feature Pitfalls
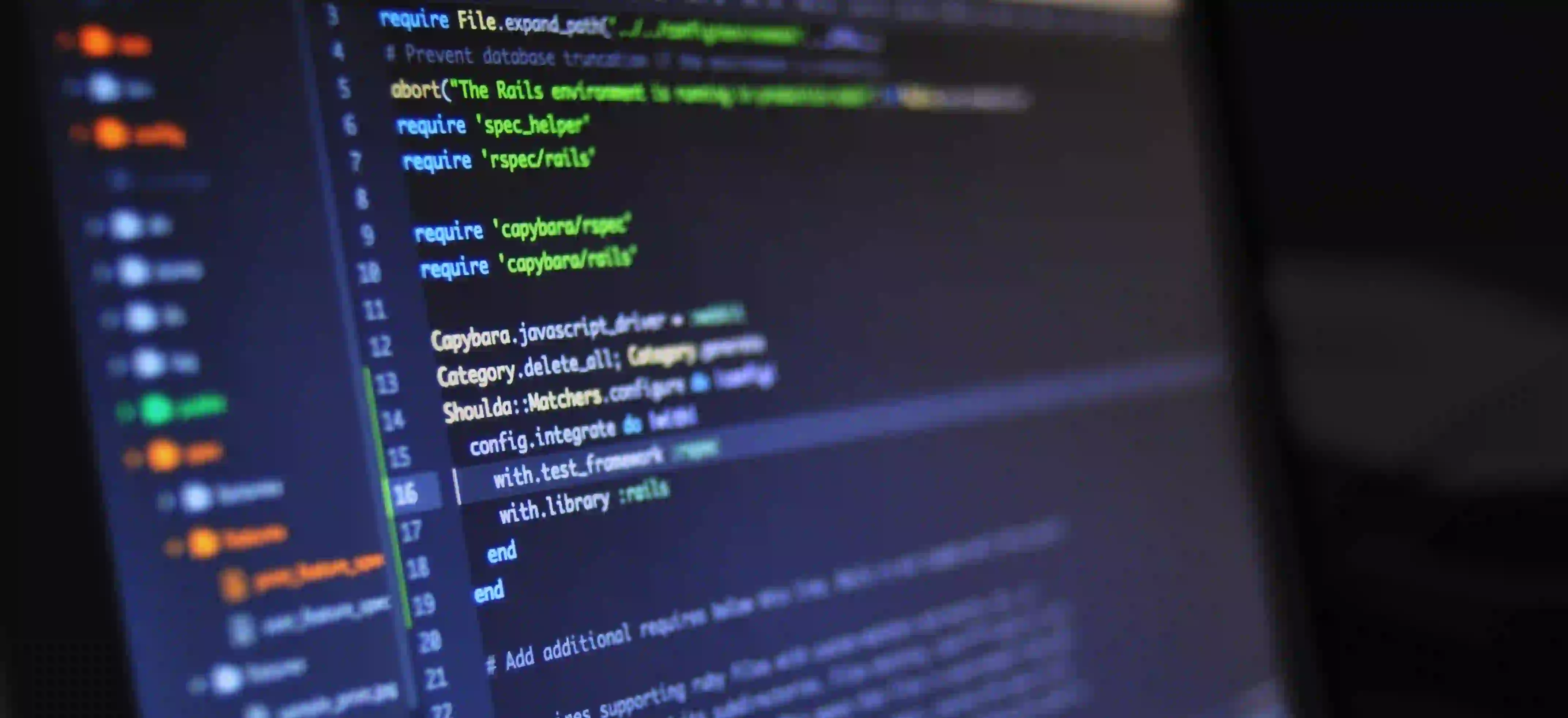
Mastering Java 8: Avoiding Common Language Feature Pitfalls
Java 8 introduced a plethora of enhancements that changed the way developers interact with the language, fostering a more functional style of programming. While many of these features, such as Streams and Lambda expressions, are powerful and convenient, they can also lead to certain pitfalls if not used properly. In this blog post, we'll explore some common mistakes in Java 8 and provide practical solutions to help you code more effectively.
1. Understanding Streams
Java 8 introduced the Stream API, which provides a way to process sequences of elements in a functional style. However, it's easy to misuse streams if you're coming from a more traditional Java background.
Common Pitfall: Not Using Streams Effectively
It’s tempting to use Streams for every collection transformation task. However, streams are not always the best choice. One common mistake is using them for small collections or in situations where performance is critical.
Code Example: Inefficient Stream Usage
List<String> names = Arrays.asList("John", "Jane", "Jack");
// Inefficient use of Streams
List<String> upperCaseNames = names.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
Why this might not be the most efficient choice: For small collections, the overhead of creating a stream can outweigh the benefits. In such cases, a simple loop may suffice.
Recommended Approach
When dealing with small data sets, consider using simple loops. Here’s an alternative:
List<String> upperCaseNames = new ArrayList<>();
for (String name : names) {
upperCaseNames.add(name.toUpperCase());
}
This keeps your code simple and performs better with small collections, allowing you to focus on readability and efficiency.
2. The Return Type of Lambdas
Lambdas are powerful, but incautiously labeling your return types can lead to unexpected results.
Common Pitfall: Implicit Return Confusion
In cases where you don't explicitly specify the return type, Java infers it. This can create confusion, especially with more complex expressions.
Code Example: Implicit Return
Function<Integer, String> numberToString = (num) -> {
if (num > 10) {
return "Greater";
} else {
return "Smaller";
}
};
Why this might cause issues: Beginners may overlook the fact that the lambda must return a consistent type for all code paths.
Recommended Approach
Always clarify your return types, especially within block bodies of lambdas.
Function<Integer, String> numberToString = (Integer num) -> {
return (num > 10) ? "Greater" : "Smaller";
};
This clearly communicates intent and prevents future errors related to inferred types.
3. Handling Nulls in Optional
Java 8 introduced Optional
to handle values that may be absent, reducing the risk of NullPointerException
. However, improper uses can negate its benefits.
Common Pitfall: Overusing or Misusing Optional
Using Optional
where a primitive type would suffice is inefficient and can lead to increased complexity.
Code Example: Inappropriate Use of Optional
Optional<Integer> optionalValue = Optional.ofNullable(getValue());
if (optionalValue.isPresent()) {
System.out.println(optionalValue.get());
}
This can be simplified significantly.
Recommended Approach
Utilize Optional
where it makes logical sense. If working with primitives, you might just want to check for null:
Integer value = getValue();
if (value != null) {
System.out.println(value);
}
Why this is better: It avoids unnecessary object creation and keeps your code cleaner.
4. Stream Combinators: Flattening and Reducing
While working with streams, it’s essential to understand flattening and reducing properly.
Common Pitfall: Forgetting to Flatten Nested Structures
When dealing with nested collections, using flatMap
correctly is vital.
Code Example: Flawed Nested Stream Handling
List<List<String>> nestedLists = Arrays.asList(
Arrays.asList("a", "b"),
Arrays.asList("c", "d")
);
// Incorrectly expecting the result to be a flat stream
Stream<String> flatStream = nestedLists.stream()
.flatMap(list -> list.stream()); // Correct usage
Recommended Approach
Flatter your streams only as necessary to avoid complexity. Here’s how it's done correctly:
List<String> flatList = nestedLists.stream()
.flatMap(List::stream)
.collect(Collectors.toList());
Why flattening is critical: It helps you access nested data structures straightforwardly without adding unneeded complexity to your code.
5. Be Aware of Performance Implications
Streams and lambdas can introduce performance overhead, particularly due to their verbose setup.
Common Pitfall: Ignoring Performance Costs
While Streams can improve readability, they may also slow your application if used in tight loops or when dealing with a significant number of elements.
Recommended Approach
Monitor performance and avoid unnecessary operations.
List<String> names = Arrays.asList("John", "Jane", "Jack", "Jill", "Jerry");
// Using Stream (less optimal for small collections)
long count = names.stream()
.filter(name -> name.startsWith("J"))
.count();
Instead, consider a loop for better performance:
long count = 0;
for (String name : names) {
if (name.startsWith("J")) {
count++;
}
}
Why this matters: For performance-sensitive applications, sticking to the basics can significantly enhance speed and responsiveness.
Lessons Learned
Java 8's language features, including Streams, Lambdas, and Optional, provide powerful tools to streamline your Java development. However, being mindful of common pitfalls is equally important.
By understanding when to use these features effectively, you can write more efficient, readable, and maintainable code.
For those looking for a more in-depth understanding of functional programming in Java, I recommend checking out Java 8 in Action and reviewing the official Java documentation.
Mastering Java 8 is not just about learning the new features but also about understanding when and how to use them to your advantage. Happy coding!