Boosting Efficiency in Fast Remote Service Testing
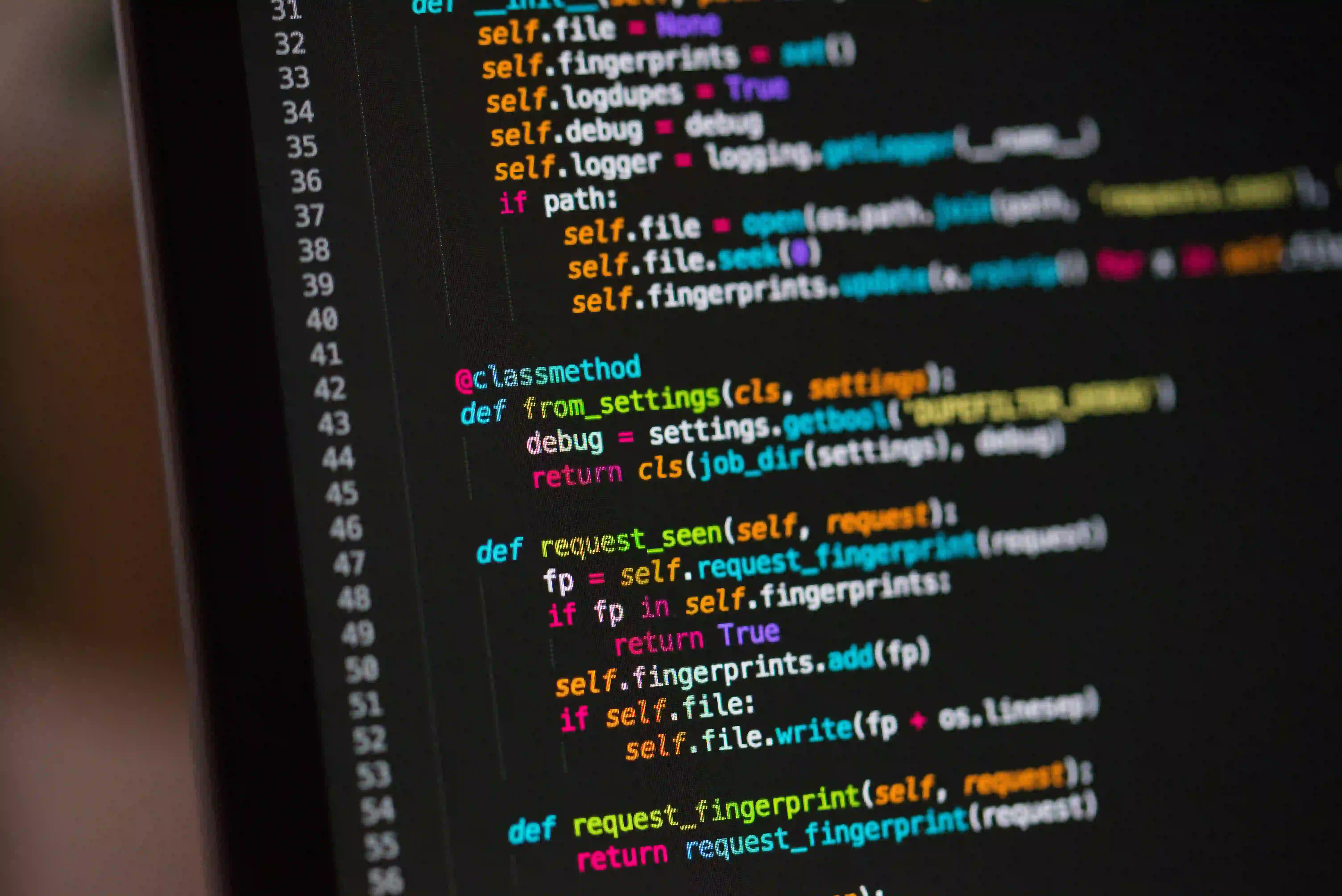
Boosting Efficiency in Fast Remote Service Testing
In today's interconnected world, the demand for rapid software delivery has never been higher. Whether you're working on a microservice architecture or a monolithic application, testing remains a crucial part of your development cycle. This blog post will guide you through effective strategies to enhance the efficiency of remote service testing, enabling your team to deliver high-quality software faster and with more confidence.
Understanding Remote Service Testing
Remote service testing is the practice of assessing the performance, reliability, and functionality of web services that reside on remote servers. This type of testing offers several benefits:
- Scalability: Remote services can be tested in isolation, making it easier to scale up tests as the application grows.
- Environment Consistency: Testing services in a production-like environment ensures better predictability for real-world interactions.
- Access to Global Resources: Remote testing allows for the evaluation of services from various geographical locations.
With these advantages in mind, how can we maximize the efficiency of remote service testing?
Key Strategies for Efficient Remote Service Testing
1. Automated Testing Frameworks
Why Automation?
Automation in testing services not only saves time but also reduces human error. Automated tests can be triggered on every code change, allowing for immediate feedback.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
class CalculatorServiceTest {
@Test
void addNumbers_ReturnsCorrectSum() {
CalculatorService calculatorService = new CalculatorService();
int result = calculatorService.add(2, 3);
assertEquals(5, result, "The sum should be 5");
}
}
In this example, we have a simple calculator service test. The use of JUnit allows for streamlined assertions and easier understanding of test results, enhancing team collaboration.
Tips for Automation
- Use Test-Driven Development (TDD): Write tests before code to build more robust services.
- Select Robust Frameworks: Consider using frameworks like JUnit for Java applications or RestAssured for API testing.
- Leverage CI/CD Tools: Incorporate tools like Jenkins or GitHub Actions to automate the entire testing pipeline.
2. Parallel Testing
Why Parallelism?
Running tests in parallel can drastically reduce execution time, especially when dealing with large sets of data or multiple use cases.
import org.junit.jupiter.api.parallel.Execution;
import org.junit.jupiter.api.parallel.ExecutionMode;
@Execution(ExecutionMode.CONCURRENT)
class ParallelServiceTest {
@Test
void testServiceA() {
// Simulate a service call and assertion...
}
@Test
void testServiceB() {
// Simulate another service call and assertion...
}
}
Here, we leverage JUnit's parallel execution capabilities. This helps in significantly cutting down the total test time as multiple tests run concurrently.
Best Practices for Parallel Testing
- Isolate Tests: Ensure tests do not depend on shared states.
- Monitor Resource Usage: Make sure your testing environment can handle multiple concurrent executions.
- Review Results Carefully: Parallel execution can sometimes lead to flaky tests. Analyze results to understand any discrepancies.
3. Use of Mock Servers
Why Mocking?
Testing remote services often involves unpredictable circumstances, such as network latency or service downtime. Mock servers can simulate these remote services and provide consistent, controlled responses for your tests.
public class MockServer {
public String getMockResponse() {
return "{\"message\":\"Success\"}";
}
}
The mock server here provides a predictable response, allowing developers to test their logic without relying on the actual service.
Implementing Mock Services
- Frameworks to Use: Consider using tools like WireMock or Mockito.
- Conditional Logic: Allow tests to switch between mock and real services based on the environment configuration.
- Simulate Errors: Use mocks to simulate error scenarios, ensuring your service handles them gracefully.
4. Continuous Feedback Loop
The Importance of Feedback
To enhance efficiency in remote service testing, setting up an environment that promotes constant feedback is necessary.
- Integrate with Messaging Tools: Use platforms like Slack or Teams to communicate test results.
- Dashboards for Visual Tracking: Rules and monitoring dashboards can provide insights into testing status and performance trends.
5. Load Testing
Why Load Testing Matters?
As remote services handle varying amounts of traffic, it’s essential to recognize how they perform under stress.
Utilizing tools like Apache JMeter or Gatling can allow you to simulate multiple users accessing the service simultaneously.
@Test
public void loadTestService() {
// Simulate multiple users accessing the service
// Code to execute load tests
}
Load testing helps in identifying performance bottlenecks early in the service lifecycle.
Best Practices for Load Testing
- Define Clear Metrics: Focus on response time, throughput, and resource usage.
- Gradual Scaling: Start with a smaller number of users and gradually increase to observe behavior.
- Analyze and Adjust: Use the results to fine-tune your remote service.
Key Takeaways
In conclusion, boosting the efficiency of remote service testing is not just about speed; it's about enhancing the quality of your testing practices. Emphasizing automation, parallel testing, mock servers, continuous feedback, and load testing will provide a strong foundation for achieving optimal performance.
For further reading on better testing practices, you can explore this resource on Continuous Testing and this article for a comprehensive overview of automation testing tools.
By employing these strategies, your team will not only meet the pressing demands of fast delivery cycles but will also ensure that your remote services remain reliable and performant. Happy testing!