Avoiding Common Pitfalls with the Liskov Substitution Principle
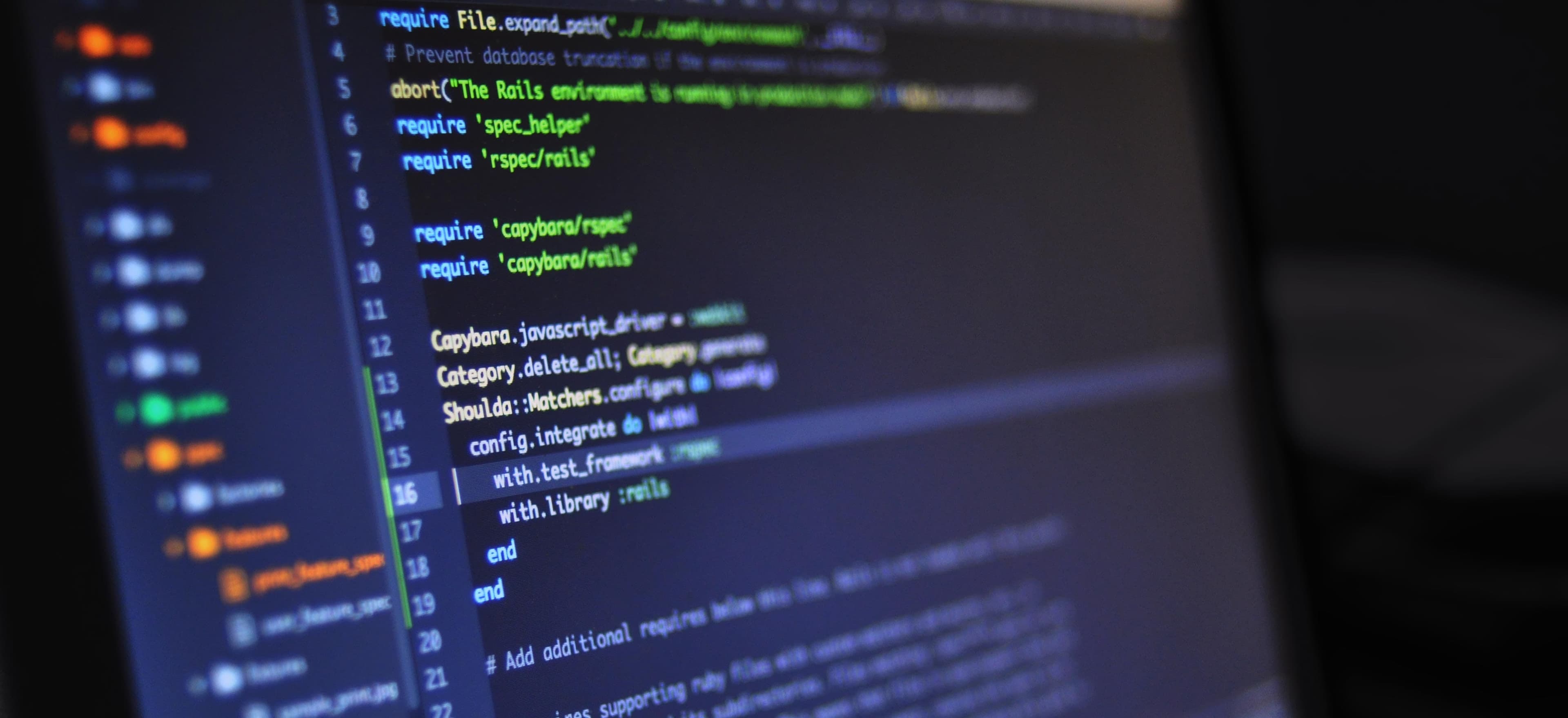
- Published on
Avoiding Common Pitfalls with the Liskov Substitution Principle
In the world of object-oriented programming (OOP), principles guide developers in creating maintainable, scalable, and robust applications. One of these fundamental principles is the Liskov Substitution Principle (LSP). This principle, introduced by Barbara Liskov in 1987, focuses on ensuring that subclasses can stand in for their parent classes without altering the desirable properties of the program. Failing to adhere to LSP can lead to a host of issues in your code, including increased complexity and unexpected behaviors.
In this blog post, we will break down the Liskov Substitution Principle, discuss its importance, and explore common pitfalls developers encounter when trying to implement it. Moreover, we will provide actionable tips alongside relevant Java code snippets to navigate these challenges effectively.
Understanding the Liskov Substitution Principle
The Liskov Substitution Principle states that if S is a subtype of T, then objects of type T should be replaceable with objects of type S without altering any of the desirable properties of that program. In simpler terms, subclasses should extend the behavior of their parents without changing their expected behavior.
Why is LSP Important?
Maintaining compatibility between classes provides several notable benefits:
- Code Reusability: Forcing subclasses to adhere to the parent class interface promotes reusability.
- Code Maintainability: A well-structured class hierarchy is easier to maintain and expand upon.
- System Reliability: Substitutable classes reduce the likelihood of bugs.
Common Pitfalls of Liskov Substitution Principle
Now that we understand LSP's significance, let's delve into common pitfalls and how to avoid them.
Pitfall 1: Violating Expected Behavior
When a subclass overrides the behavior of a method in a way that diverges from the parent class's contract, it can lead to unexpected results. This often happens when the subclass implements additional checks or throws exceptions that the parent does not.
Example Code
class Bird {
public void fly() {
System.out.println("Flying");
}
}
class Ostrich extends Bird {
@Override
public void fly() {
throw new UnsupportedOperationException("Ostriches cannot fly");
}
}
In the example above, the Ostrich
class violates LSP as it cannot substitute the Bird
class without throwing an exception.
Solution
One solution to avoid such issues is to create a new base class for non-flying birds.
class Bird {
public void makeSound() {
System.out.println("Chirping");
}
}
class FlyingBird extends Bird {
public void fly() {
System.out.println("Flying");
}
}
class Sparrow extends FlyingBird {
// Can fly
}
class Ostrich extends Bird {
// Cannot fly
}
Pitfall 2: Fragile Base Class Problem
Another common pitfall arises when changes to the base class inadvertently break the subclasses due to implicit assumptions. This is commonly known as the fragile base class problem.
Example Code
class Shape {
public double area() {
return 0;
}
}
If a subclass overrides the area
method improperly, it can render the subclass invalid or break existing functionalities.
Solution
It is advisable to define abstract methods and enforce a contract in the base class.
abstract class Shape {
public abstract double area();
}
class Rectangle extends Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
public double area() {
return width * height;
}
}
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
}
Pitfall 3: Incompatible Method Signatures
A subclass may introduce incompatible method signatures, causing issues when substituting it for the parent class.
Example Code
class Vehicle {
public void start() {
System.out.println("Vehicle started");
}
}
class ElectricCar extends Vehicle {
public void start(int batteryLevel) { // incompatible method
System.out.println("Electric car started with battery level: " + batteryLevel);
}
}
In this scenario, the ElectricCar
cannot be substituted for the Vehicle
without introducing confusion.
Solution
Ensure that subclasses maintain the same method signatures.
class ElectricCar extends Vehicle {
@Override
public void start() { // Maintain method signature
System.out.println("Electric car started silently");
}
}
Pitfall 4: State Invariant Violations
Another common issue is maintaining state invariants when overriding methods. If a subclass alters the state of the object incorrectly during substitution, this can lead to significant defects in the overall application behavior.
Example Code
class Account {
protected double balance;
public void deposit(double amount) {
balance += amount;
}
public void withdraw(double amount) {
balance -= amount;
}
}
class SavingsAccount extends Account {
// Introduce a minimum balance check
@Override
public void withdraw(double amount) {
if (balance - amount < 1000) {
throw new IllegalArgumentException("Minimum balance of 1000 required");
}
super.withdraw(amount);
}
}
In this case, SavingsAccount
imposes an additional condition that may violate the contract established by Account
.
Solution
Consider utilizing interfaces or abstract classes to enforce state conditions instead of overriding the method directly.
interface Withdrawable {
void withdraw(double amount);
}
class GeneralAccount implements Withdrawable {
protected double balance;
public void deposit(double amount) {
balance += amount;
}
@Override
public void withdraw(double amount) {
balance -= amount;
}
}
class SavingsAccount extends GeneralAccount {
@Override
public void withdraw(double amount) {
if (balance - amount < 1000) {
throw new IllegalArgumentException("Minimum balance of 1000 required");
}
super.withdraw(amount);
}
}
Summary
Implementing the Liskov Substitution Principle is vital for creating scalable, maintainable, and robust software systems. By diligently avoiding common pitfalls, such as violating expected behavior, succumbing to the fragile base class problem, using incompatible method signatures, and allowing state invariant violations, we can ensure more reliable and predictable code.
By adhering to good design principles, including effective application of the LSP, developers can build systems that are not only easier to extend but also less prone to bugs. Remember, a well-understood and properly applied inheritance hierarchy can be the difference between a successful project and an unmanageable codebase.
Finding more about OOP principles can deepen your knowledge, and you might find resources like Clean Code and Refactoring extremely helpful.
If you have any questions or comments about applying the LSP in your development work, please feel free to share your experiences or thoughts below!
Checkout our other articles