Common JDBC Insert Errors in Oracle and How to Fix Them
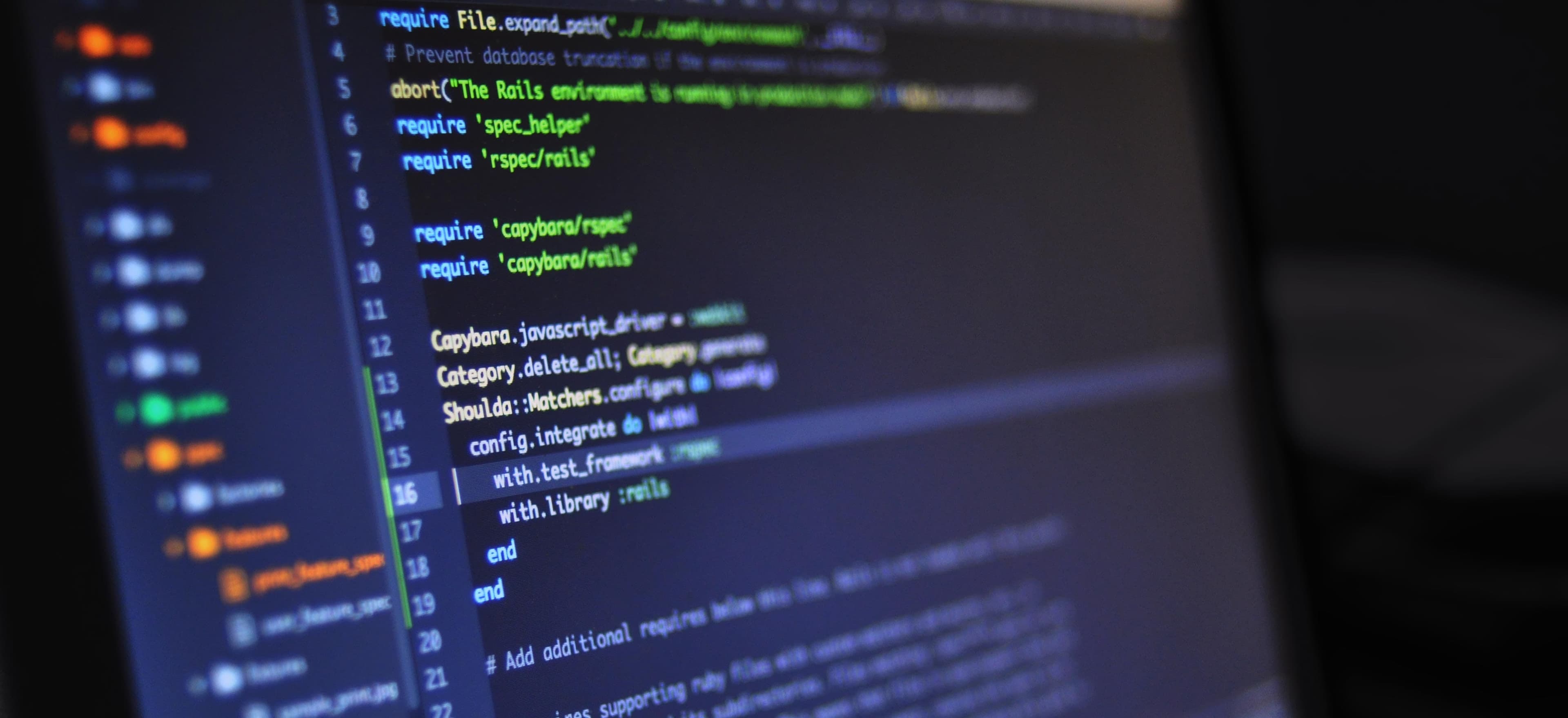
- Published on
Common JDBC Insert Errors in Oracle and How to Fix Them
When working with databases in Java, JDBC (Java Database Connectivity) is the standard API that allows Java applications to interact with various database systems, including Oracle. While JDBC provides a powerful way to carry out database operations, developers often encounter a slew of common errors during insert operations. In this blog post, we will address those errors and provide insights into how to fix them, while keeping things practical and straightforward.
Understanding JDBC and Oracle
JDBC is an API that enables Java applications to execute SQL statements, retrieve results, and manage transactions. While Oracle's database engine supports numerous features, it also introduces unique constraints and considerations that can lead to errors, especially in insert operations.
Before diving into specific error cases, let’s set the stage with a basic JDBC setup for inserting data into an Oracle database.
Basic JDBC Setup for Inserting Data
Below is an example of how to set up a simple JDBC connection and perform an insert command:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class JdbcInsertExample {
public static void main(String[] args) {
String url = "jdbc:oracle:thin:@localhost:1521:xe";
String user = "username"; // replace with your username
String password = "password"; // replace with your password
Connection conn = null;
PreparedStatement pstmt = null;
try {
// Establishing a connection
conn = DriverManager.getConnection(url, user, password);
String sql = "INSERT INTO employees (id, name, position) VALUES (?, ?, ?)";
// Creating a PreparedStatement
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, 1); // setting ID
pstmt.setString(2, "John Doe"); // setting Name
pstmt.setString(3, "Software Engineer"); // setting Position
// Executing the insert
int rowsAffected = pstmt.executeUpdate();
System.out.println(rowsAffected + " row(s) inserted.");
} catch (SQLException e) {
e.printStackTrace();
} finally {
// Closing resources
try {
if (pstmt != null) pstmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
This snippet establishes a connection to an Oracle database, prepares an SQL insert statement, and executes it. It's essential to handle the SQLException
to catch potential issues.
Common JDBC Insert Errors
Now that we understand the basic setup, let’s explore some common JDBC insert errors in Oracle and how to address them.
1. SQLException: ORA-00001: Unique Constraint Violated
Error Message: ORA-00001: unique constraint (constraint_name) violated
This error occurs when you attempt to insert a duplicate value into a table where a unique constraint exists (such as primary keys).
Solution: Ensure that the value you are trying to insert is unique. If your id
column has a unique constraint, ensure you are not trying to insert an existing id
.
// Check if ID already exists before insertion
int existingId = 1; // Assume we are checking for ID 1
if (!idExists(existingId, conn)) {
pstmt.setInt(1, existingId);
// continue with insertion
} else {
System.out.println("ID already exists. Choose a different ID.");
}
private static boolean idExists(int id, Connection conn) {
// Logic to check if ID exists in the employees table
}
2. SQLException: ORA-00942: Table or View Does Not Exist
Error Message: ORA-00942: table or view does not exist
This error signifies that you are trying to insert data into a table that does not exist in the database schema you're connected to.
Solution: Verify that the table name is correct and that your user has the necessary permissions. You also need to ensure that you are using the correct case for the table name.
String sql = "INSERT INTO EMPLOYEES (id, name, position) VALUES (?, ?, ?)"; // Check case
3. SQLException: ORA-01400: Cannot Insert Null into (column_name)
Error Message: ORA-01400: cannot insert NULL into (column_name)
This error occurs when you try to insert a null value into a column that does not allow null values (as defined by the NOT NULL constraint).
Solution: Ensure that all mandatory fields are set before executing the insert statement.
pstmt.setString(2, "John Doe"); // Name cannot be null
if (name == null) {
throw new IllegalArgumentException("Name cannot be null");
}
4. SQLException: ORA-12899: Value too Large for Column
Error Message: ORA-12899: value too large for column (column_name, size)
This error surfaces when the data being inserted exceeds the size limit defined for the column in the database.
Solution: Check the size limit for the column and ensure that your input data conforms to it. Adjust the column definition in your Oracle database if necessary.
String sql = "CREATE TABLE employees (id NUMBER(5), name VARCHAR2(50), position VARCHAR2(30))";
// Ensure your data fits the size limits.
pstmt.setString(2, "A very long name that exceeds the limit"); // This may cause an error
5. SQLException: ORA-01722: Invalid Number
Error Message: ORA-01722: invalid number
This error occurs when you try to convert a non-numeric string to a number type column.
Solution: Ensure that the data types you are inserting match the column types in your table.
String idString = "abc"; // Invalid number
try {
int id = Integer.parseInt(idString); // Will throw NumberFormatException
pstmt.setInt(1, id);
} catch (NumberFormatException e) {
System.out.println("Invalid ID: " + idString);
}
A Final Look
Working with JDBC and Oracle databases can be complex, but understanding common insert errors can simplify the process significantly. By preparing your code to handle errors gracefully, ensuring valid data types, and adhering to your database's constraints, you can avoid countless headaches down the line.
Additional Resources
For further reading on JDBC and Oracle operations, consider these links:
Maintaining a systematic approach to error handling will help you to efficiently work with database insert operations. Happy coding!
Checkout our other articles