Streamlining Spring Boot Deployment on Elastic Beanstalk
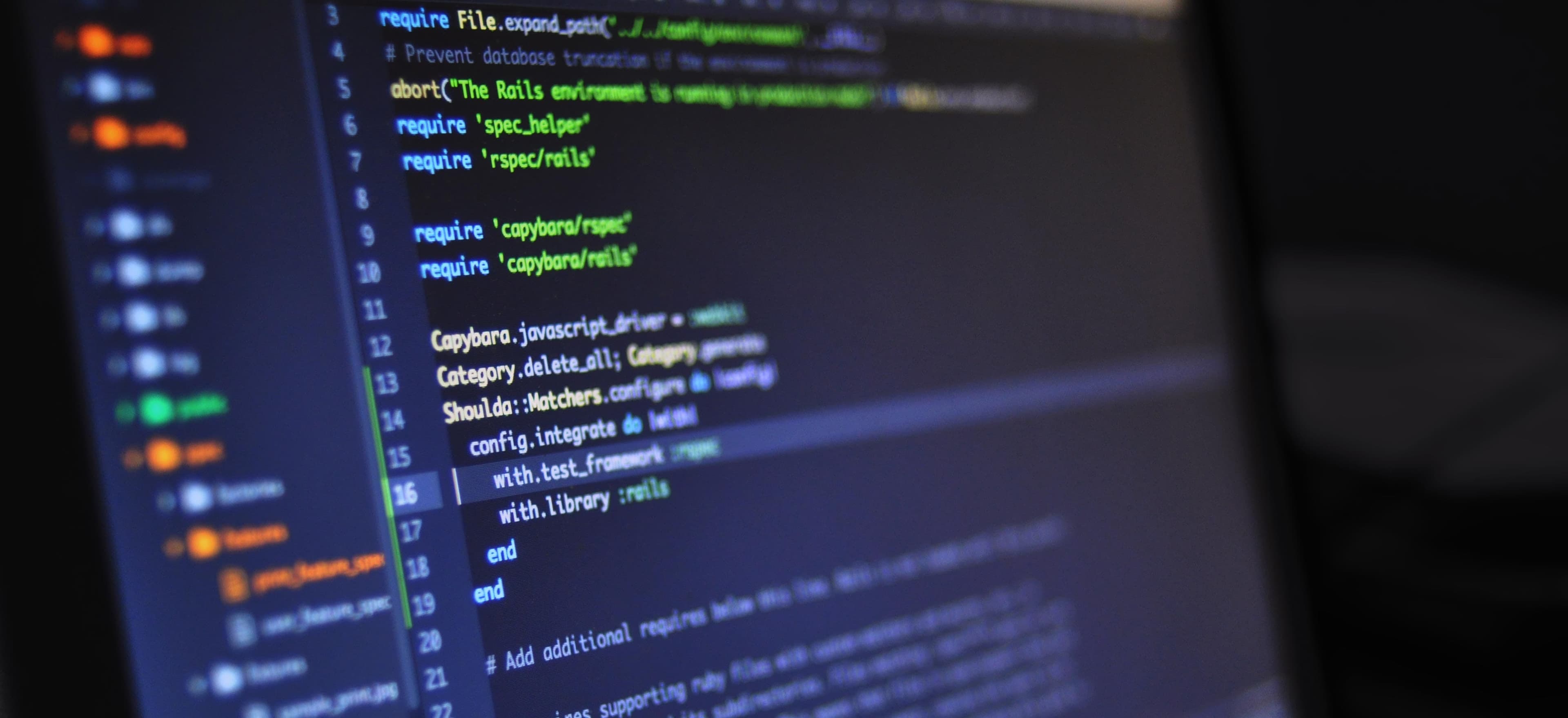
- Published on
Streamlining Spring Boot Deployment on Elastic Beanstalk
Deploying Spring Boot applications can be daunting, especially when considering the various environments and platforms available. Amazon Web Services (AWS) Elastic Beanstalk is a powerful platform that simplifies the deployment, management, and scaling of applications. This blog post will explore how to streamline your Spring Boot deployment on Elastic Beanstalk.
Overview of Elastic Beanstalk
Elastic Beanstalk is an orchestration service by AWS for deploying applications that handles the deployment, from capacity provisioning, load balancing, auto-scaling, to application health monitoring. When combined with Spring Boot, it enables rapid deployment of Java-based applications.
Why Choose Elastic Beanstalk?
- Simplified Deployment: Elastic Beanstalk abstracts the underlying infrastructure, allowing you to focus on your application code.
- Automatic Scaling: You can easily scale your application based on demand without manual intervention.
- Health Monitoring: Built-in health monitoring and logging features let you keep an eye on your application’s performance.
Pre-requisites
Before we get started, ensure you have the following:
- An AWS account
- Java Development Kit (JDK) installed
- Apache Maven installed for building your Spring Boot application
- AWS CLI installed and configured with your credentials
Step 1: Create Your Spring Boot Application
Let's begin with a simple Spring Boot application. We will create a RESTful web service that returns a greeting message.
@SpringBootApplication
@RestController
public class GreetingApplication {
public static void main(String[] args) {
SpringApplication.run(GreetingApplication.class, args);
}
@GetMapping("/greet")
public String greet() {
return "Hello, Welcome to Elastic Beanstalk!";
}
}
Why this code?: This application defines a simple REST endpoint /greet
that returns a greeting message. The @SpringBootApplication
annotation indicates this is a Spring Boot application.
Step 2: Package the Application
Next, we are going to package the application into a deployable artifact. Navigate to your project directory in the terminal and use Maven to create a JAR file.
mvn clean package
Why package?: This command cleans the project and compiles the application, generating a single JAR file located in the target
directory. This JAR file is what we'll deploy to Elastic Beanstalk.
Step 3: Set Up Elastic Beanstalk
-
Create an Application:
- Log in to the AWS Management Console.
- Navigate to Elastic Beanstalk and click on "Create a new application."
- Name your application and provide a description.
-
Create an Environment:
- Click on "Create Environment."
- Choose "Web server environment."
- Select the platform as "Java" and specify the platform version.
- Upload the JAR file you generated in the previous step.
-
Configure Environment:
- Configure instance type, scaling, and load balancing as necessary.
- Options include setting up environment variables that your application might depend on.
-
Launch the Environment:
- Review your settings and click "Create Environment."
Step 4: Environment Configuration
Elastic Beanstalk allows you to set up environment properties. You can do this via the console or using a configuration file.
Using the Console:
- Navigate to your environment.
- Go to “Configuration” > “Software”.
- Under “Environment properties,” add properties as key-value pairs.
Using a Configuration File (e.g., ebextensions
):
You can also create a file named 01_environment.config
inside an ebextensions
directory in your project.
option_settings:
aws:elasticbeanstalk:application:environment:
SPRING_PROFILES_ACTIVE: production
DATABASE_URL: jdbc:mysql://your-db-url
Why use configuration files?: This allows better version control of your configurations that can be deployed alongside your application.
Step 5: Update and Redeploy
Whenever you make changes to your application, you'll need to rebuild it and then redeploy it to Elastic Beanstalk.
-
Package your application again:
mvn clean package
-
Deploy the new artifact:
- In the Elastic Beanstalk console, select your environment.
- Click "Upload and Deploy."
- Choose the newly created JAR and deploy.
Step 6: Monitoring Your Application
Once your application is up and running, use the AWS console to monitor its performance.
- Check the "Health" section for any issues related to your running application.
- Log files can be accessed via the "Logs" section, which is crucial for diagnosing problems.
AWS also offers tools like CloudWatch for monitoring metrics and alerting on various performance indicators. Integrating monitoring in your CI/CD pipeline is an advanced topic; consider checking AWS documentation for further insights.
Step 7: Conclusion
Deploying Spring Boot applications to Elastic Beanstalk significantly simplifies the infrastructure management burden, allowing developers to focus on building features. The benefits of automatic scaling and ease of monitoring make it an attractive option for scalable applications.
For further reading and tools on Spring Boot best practices, you might want to check out the Spring Boot Documentation and the AWS Elastic Beanstalk Documentation.
In the world of cloud computing, mastering deployment platforms like AWS Elastic Beanstalk can greatly enhance your development workflow, ensure seamless deployment, and help you reach your application's performance goals efficiently.
Checkout our other articles