Are You Stuck in Concrete Code? Here's How to Break Free!
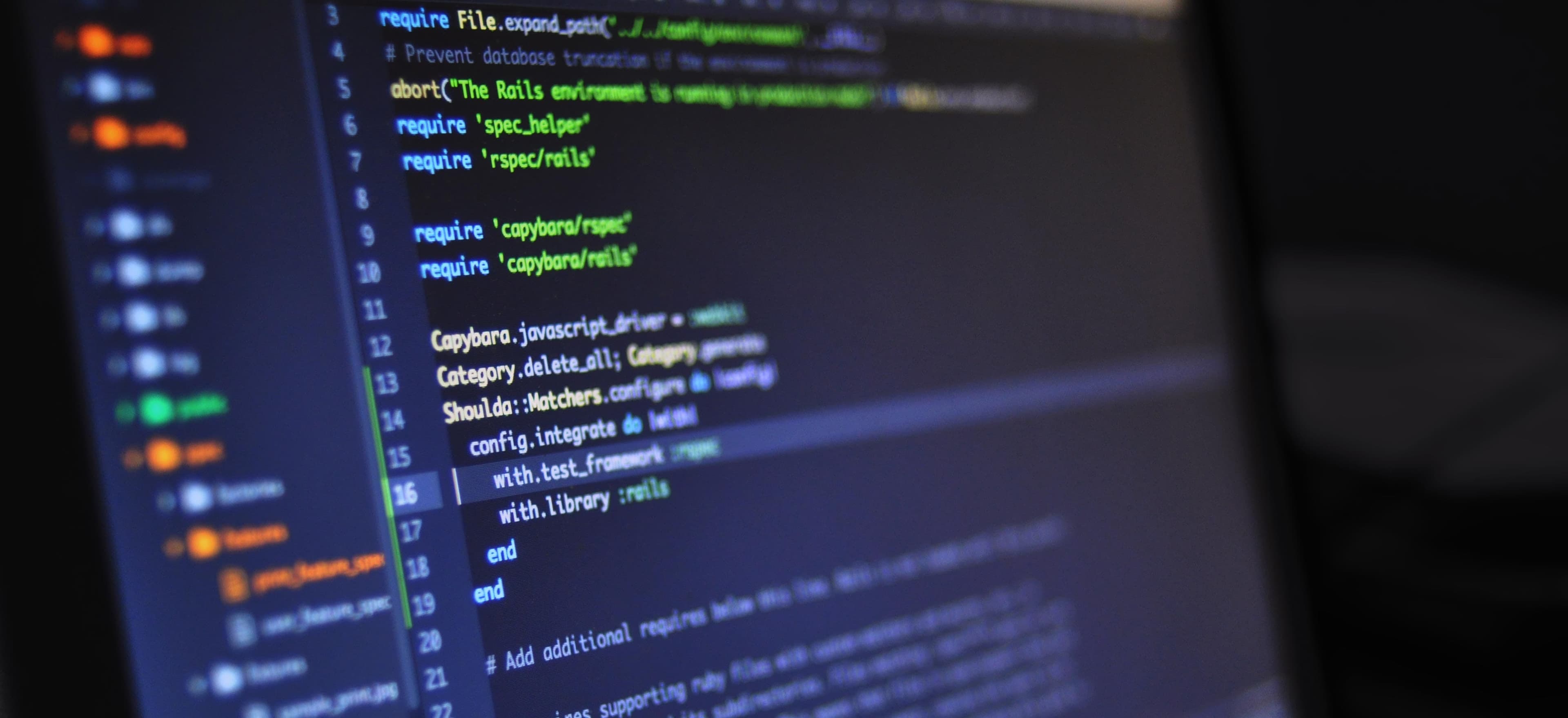
- Published on
Are You Stuck in Concrete Code? Here's How to Break Free!
Concrete code refers to the practice of writing code that is too specific, rigid, and often hard to adapt to future changes. Many developers find themselves trapped in a labyrinth of concrete code that's challenging to maintain and extend. But fear not! In this blog post, we’ll explore effective strategies for breaking free from concrete code and embracing better coding practices that promote flexibility and maintainability.
Understanding Concrete Code
Concrete code is characterized by its inflexible structure, often leading to various issues:
- Difficult Maintenance: Concrete code often requires extensive modifications when changes are needed.
- Code Duplication: A lack of abstraction can lead to repeated code blocks (DRY principle violations).
- Hard to Test: Rigid structures are usually hard to unit test, making quality assurance a daunting task.
The Costs of Concrete Code
According to a study by the Project Management Institute, inefficient coding practices can increase project costs by 50%. This statistic underscores the importance of writing flexible, modular code that allows for easier adaptations in the long run.
Techniques to Break Free from Concrete Code
Here are some tried-and-true practices to help you transition from rigid concrete code to a more adaptable coding style.
1. Embrace Object-Oriented Principles
Object-oriented programming (OOP) is foundational in creating flexible code. By encapsulating data and behavior, OOP promotes reuse and abstraction.
Example: Using Inheritance
abstract class Animal {
public abstract void makeSound();
}
class Dog extends Animal {
public void makeSound() {
System.out.println("Bark");
}
}
class Cat extends Animal {
public void makeSound() {
System.out.println("Meow");
}
}
public class Main {
public static void main(String[] args) {
Animal dog = new Dog();
dog.makeSound(); // Output: Bark
Animal cat = new Cat();
cat.makeSound(); // Output: Meow
}
}
Why? By using abstract classes and inheritance, this approach allows you to extend functionality without modifying existing code. You can easily add new animal types without touching the Animal
class, thus adhering to the Open/Closed principle.
2. Implement Interfaces
Interfaces define a contract that implementing classes must follow but don’t dictate how to implement those methods. This abstraction allows for flexibility.
Example: Interface for Payment Processing
interface Payment {
void processPayment(double amount);
}
class PayPal implements Payment {
public void processPayment(double amount) {
System.out.println("Processing PayPal payment of " + amount);
}
}
class CreditCard implements Payment {
public void processPayment(double amount) {
System.out.println("Processing Credit Card payment of " + amount);
}
}
public class Main {
public static void main(String[] args) {
Payment paymentMethod = new PayPal();
paymentMethod.processPayment(100.0); // Output: Processing PayPal payment of 100.0
paymentMethod = new CreditCard();
paymentMethod.processPayment(150.0); // Output: Processing Credit Card payment of 150.0
}
}
Why? Implementing interfaces allows for easy expansions. You can add new payment methods without altering existing classes, promoting a flexible architecture.
3. Favor Composition Over Inheritance
While inheritance can be effective, overusing it can lead to a fragile base class problem. Favoring composition allows for more flexible code structure and better separation of concerns.
Example: Using Composition
class Engine {
void start() {
System.out.println("Engine started");
}
}
class Car {
private Engine engine;
public Car() {
engine = new Engine(); // Compose a Car with an Engine
}
void start() {
engine.start();
System.out.println("Car started");
}
}
public class Main {
public static void main(String[] args) {
Car car = new Car();
car.start(); // Output: Engine started
// Car started
}
}
Why? Composition gives you the flexibility to change how components are combined without altering the classes themselves, leading to lower coupling. You can swap out the Engine
class without affecting the Car
class directly.
4. Apply the Dependency Injection Pattern
Dependency Injection (DI) allows you to decouple your dependencies, making your code more flexible and testable.
Example: Simple DI in Java
class Service {
public void serve() {
System.out.println("Service called");
}
}
class Consumer {
private Service service;
// Dependency is injected via the constructor
public Consumer(Service service) {
this.service = service;
}
public void doSomething() {
service.serve();
}
}
public class Main {
public static void main(String[] args) {
Service service = new Service();
Consumer consumer = new Consumer(service);
consumer.doSomething(); // Output: Service called
}
}
Why? By injecting the Service
dependency into Consumer
, it becomes easier to test different implementations. DI frameworks like Spring can automate this process, leading to efficient management of application components.
5. Follow SOLID Principles
The acronym SOLID stands for five principles designed to make software designs more understandable, flexible, and maintainable.
- Single Responsibility Principle (SRP): A class should have only one reason to change.
- Open/Closed Principle (OCP): Software entities should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP): Subtypes should be substitutable for their base types.
- Interface Segregation Principle (ISP): No client should be forced to depend on methods it does not use.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules but rather on abstractions.
6. Utilize Design Patterns
Design patterns can simplify and standardize solutions to common problems, thereby reducing the complexity of your code.
- Factory Pattern: For creating objects without specifying the exact class.
- Singleton Pattern: Ensures a class has only one instance and provides a global point of access.
The use of design patterns can elevate your coding practices from mindless repetition to intelligent design. For more on design patterns, check out Refactoring Guru.
7. Refactor Regularly
The best way to combat concrete code is to refactor it regularly. Refactoring not only improves the structure of your existing code but also adapts it to meet changing requirements efficiently.
Example of Refactoring
Transforming a method that directly handles logic into a cleaner structure.
Before Refactoring:
class Report {
void generateReport() {
// Directly handling formatting
System.out.println("Formatting report...");
}
}
After Refactoring:
interface Formatter {
String format(String report);
}
class PDFFormatter implements Formatter {
public String format(String report) {
return "PDF formatted report: " + report;
}
}
// Now the Report class doesn't need to know about the specific format.
class Report {
private Formatter formatter;
public Report(Formatter formatter) {
this.formatter = formatter;
}
void generateReport() {
String report = "Report content here";
System.out.println(formatter.format(report));
}
}
Why? The refactored version allows different formatter implementations while keeping the Report
class streamlined and adaptable.
Key Takeaways
Concrete code can be a bottleneck in software development, leading to complications in maintenance, testing, and scalability. By adopting object-oriented principles, using interfaces, applying dependency injection, and following the SOLID principles, you can effectively escape the trap of concrete code.
Regular refactoring and embracing design patterns will ensure that your codebase remains agile and prepared for change. With these strategies, your development process can become more fluid.
As you continue your journey in Java development, remember: the flexibility of your code defines the flexibility of your project. Don't wait to break free from concrete code; start implementing these strategies today!
For more resources, consider looking into Java Design Patterns to deepen your understanding of creating adaptable code structures. Happy coding!
Checkout our other articles