Overcoming Challenges in Low-Code Development Implementations
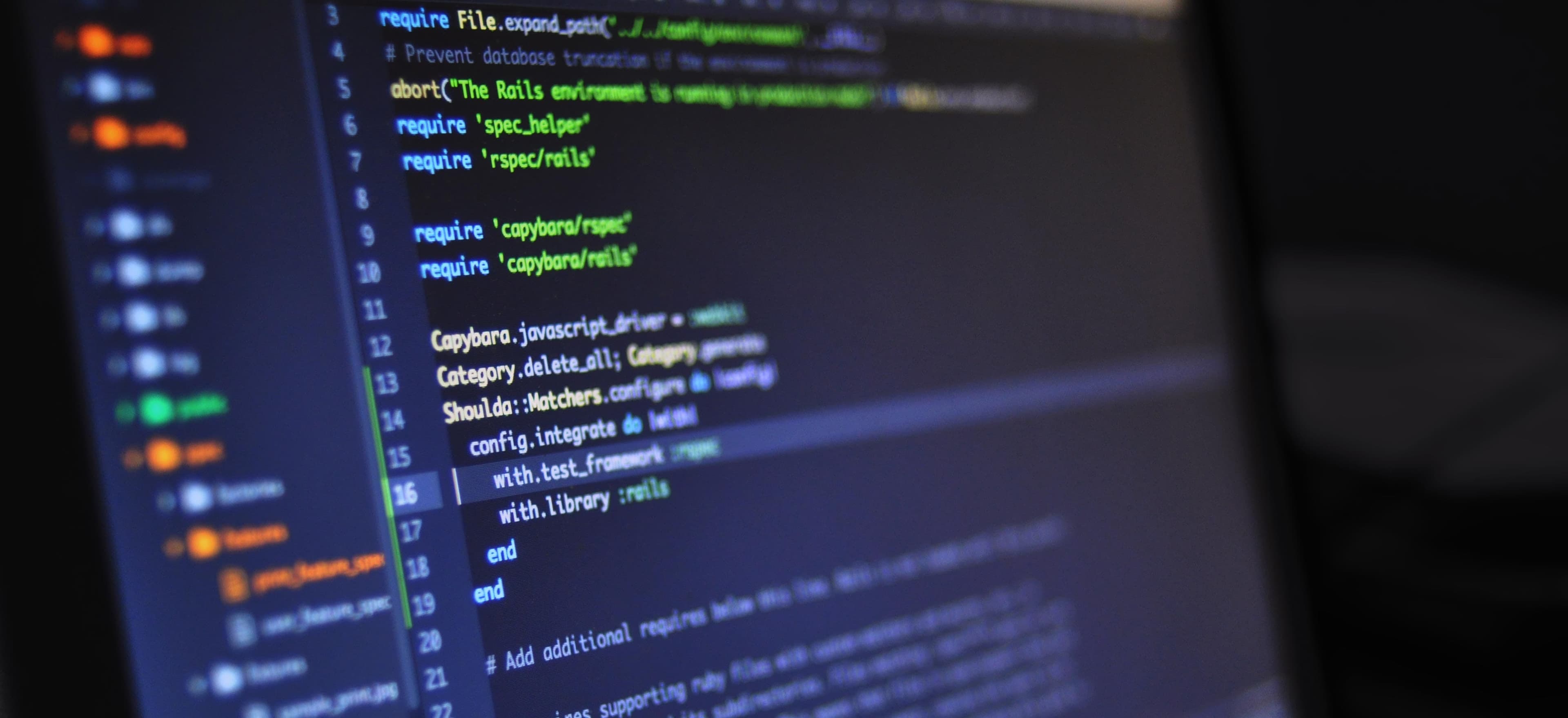
- Published on
Overcoming Challenges in Low-Code Development Implementations
Low-code development is transforming the IT landscape, empowering organizations to create applications with minimal hand-coding. While many aspects of low-code platforms present enticing advantages, they come with unique challenges that need addressing. This blog will explore the hurdles faced during low-code implementations and provide solutions you can use to navigate these challenges successfully.
Beginning Insights to Low-Code Development
Low-code development platforms (LCDPs) allow users to build applications through visual interfaces, largely eliminating the need for traditional coding. According to a report by Gartner, low-code development will account for over 65% of application development by 2024. This surge in popularity is driven by the need for faster time-to-market and the capability to adapt to changing business requirements.
Despite its advantages, low-code development is not devoid of challenges. Understanding these hurdles is key to successfully implementing low-code solutions.
Common Challenges in Low-Code Development Implementations
1. Integration with Existing Systems
Most businesses rely on existing systems and applications, and ensuring that new low-code applications can seamlessly integrate with these can be complex.
Solution:
Utilize built-in APIs or connectors provided by your low-code platform. Most LCDPs come with pre-configured integrations for common enterprise applications like Salesforce, SAP, or Microsoft Dynamics.
Example code for integrating an external REST API might look like this:
import java.net.HttpURLConnection;
import java.net.URL;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class ApiIntegrationExample {
public static void main(String[] args) {
try {
URL url = new URL("https://api.example.com/data");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.connect();
if (conn.getResponseCode() != 200) {
throw new RuntimeException("Failed : HTTP error code : " + conn.getResponseCode());
}
BufferedReader br = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String output;
while ((output = br.readLine()) != null) {
System.out.println(output);
}
conn.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why this code matters: This snippet demonstrates how to connect to an external API, which is crucial for data integration tasks in low-code development. By handling API requests efficiently, developers can ensure real-time data fetching and synchronization between systems, enhancing the overall application performance.
2. Limited Customization
While low-code platforms streamline development, they can impose limitations on customization, making it hard to tailor applications to specific business needs.
Solution:
Look for flexible low-code platforms that allow you to add custom code. Familiarity with Java can be particularly advantageous here, as many low-code platforms enable Java snippets within their interface.
Example of a simple Java component in a low-code platform:
public class CustomBusinessLogic {
public int calculateDiscount(int price, int discount) {
if(discount < 0 || discount > 100) {
throw new IllegalArgumentException("Discount must be between 0 and 100");
}
return price - (price * discount / 100);
}
}
Why this code matters: Providing custom business logic can significantly enhance your application’s functionality. This snippet validates and calculates discounts based on business rules, allowing you to integrate personalized workflows into your applications seamlessly.
3. Governance and Security
As more applications are created, the risk of governance and security lapses increases. Ensuring compliance with regulations is paramount.
Solution:
Establish clear guidelines on governance as part of your development process. Regularly audit applications for compliance with standards such as GDPR or HIPAA. Address security concerns by adopting a robust authentication method.
Example of a basic authentication mechanism:
import java.util.Base64;
public class BasicAuthentication {
public static boolean authenticate(String authHeader) {
String base64Credentials = authHeader.substring("Basic".length()).trim();
String credentials = new String(Base64.getDecoder().decode(base64Credentials));
final String[] values = credentials.split(":", 2);
String username = values[0];
String password = values[1];
// Replace with your own logic to validate username and password
return ("admin".equals(username) && "password123".equals(password));
}
}
Why this code matters: This sample code illustrates a simple authentication mechanism that can be employed in low-code applications. By ensuring that only authorized users can access your applications, you are taking a critical step toward maintaining security and compliance.
4. User Adoption and Training
User adoption is often one of the biggest challenges during any new implementation. If users don’t engage with the platform, it renders your investment moot.
Solution:
Implement a robust training program. Empower your team through workshops and documentation. Create a support system, such as forums or FAQs, where users can engage and share their experiences.
5. Performance Issues
Low-code applications can sometimes suffer from performance drawbacks due to heavy reliance on visual components.
Solution:
Optimize database queries and delegate heavy processing tasks to a back-end service whenever possible. Lean on skilled developers to tune performance and identify bottlenecks early in the development cycle.
Example of a basic SQL query optimization in Java:
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class DatabaseOptimization {
public void getUserData(int userId) {
String query = "SELECT * FROM users WHERE id = ?";
try (Connection conn = Database.getConnection();
PreparedStatement stmt = conn.prepareStatement(query)) {
stmt.setInt(1, userId);
ResultSet rs = stmt.executeQuery();
if (rs.next()) {
System.out.println("User Name: " + rs.getString("name"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why this code matters: Prepared statements enhance performance by pre-compiling SQL queries and avoiding SQL injection vulnerabilities. This approach is crucial for high-performance applications, particularly in low-code development environments where efficiency matters.
6. Change Management
Implementing a new platform may disrupt existing workflows. Managing this change effectively is crucial for a smooth transition.
Solution:
Create a change management plan that engages stakeholders and outlines the steps for rollout and support. Regular feedback loops and surveys can help gauge acceptance and uncover areas for improvement.
Wrapping Up
While low-code development offers significant advantages, from speeding up the application development cycle to empowering non-developers, it is not without its challenges. By proactively addressing issues such as integration, customization limits, governance, user adoption, performance, and change management, organizations can harness the full potential of low-code platforms.
Ultimately, overcoming these challenges leads to streamlined processes, enhanced collaboration, and better alignment with business goals. As low-code continues to evolve, staying informed and adaptable is key.
For additional insights into low-code platforms, check out these resources:
- Gartner on Low-Code Development
- Forrester Research
Feel free to share your experiences with low-code development implementations. What challenges have you faced, and how did you overcome them? Let's engage in a discussion to help each other improve our approaches!
Checkout our other articles