Resolving XA Transaction Issues in Play 20 Framework
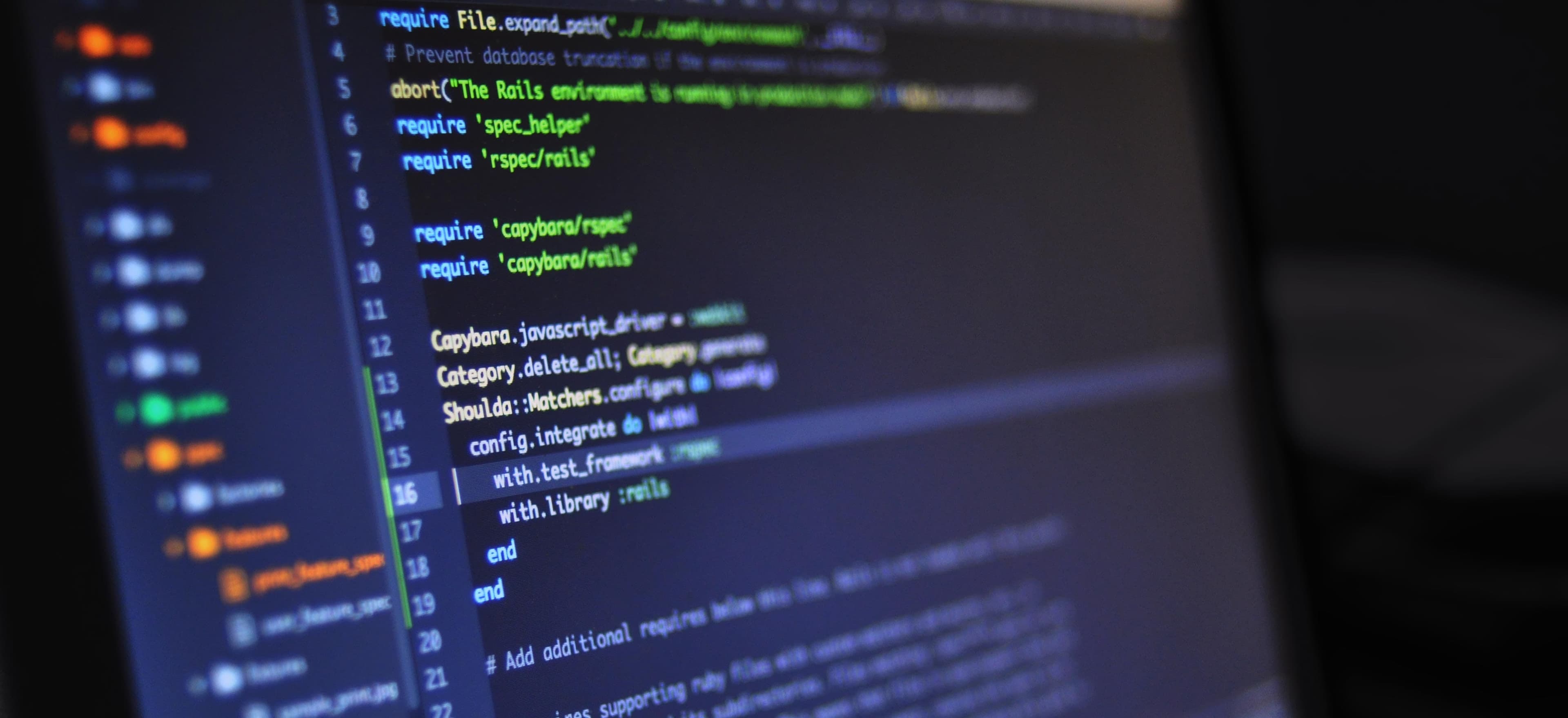
- Published on
Resolving XA Transaction Issues in Play 20 Framework
In distributed systems, ensuring the integrity and consistency of transactions is paramount. One common protocol that helps achieve this is the X/Open XA standard, which allows different resources (like databases, message queues, etc.) to participate in a single transaction. However, integrating XA transactions can present challenges, especially when using frameworks like Play 20. This blog post will guide you through understanding XA transactions and resolving common issues encountered when using them within the Play Framework.
Understanding XA Transactions
XA Transactions allow for two-phase commits, which help manage transactions across multiple resource managers. In the first phase, all the enlisted resources prepare to commit. In the second phase, the coordinator instructs the resources to either commit or roll back the transaction based on the outcome of the prepare phase.
Key Components of XA Transactions
- Transaction Manager: A component that manages the distribution of transactions across multiple resource managers.
- Resource Managers: Systems (like databases) that host transactional systems.
- XA Resources: Resources that implement the XA interface and participate in the distributed transaction.
For an in-depth understanding, refer to the XA Transactions documentation.
Why Use XA Transactions in Play Framework
The Play Framework simplifies web application development with its reactive model and built-in support for databases. However, when you are dealing with microservices or multiple databases, using XA transactions becomes necessary to maintain data integrity. Here are some reasons to use XA Transactions:
- Atomicity: All operations are treated as a single unit, ensuring that changes either all complete or none do.
- Consistency: Resources remain consistent before and after the transaction's completion.
- Isolation: Concurrent transactions do not affect each other, preventing conflicts.
Setting Up XA Transactions in Play Framework
To set up XA transactions in Play Framework, you need to have an understanding of the TransactionManager
and how to configure your HikariCP
and JDBC
data sources. Here is an example configuration:
# application.conf
db.default.driver="com.mysql.cj.jdbc.Driver"
db.default.url="jdbc:mysql://localhost:3306/mydb"
db.default.username="user"
db.default.password="password"
db.default.hikaricp.maximumPoolSize=10
db.default.hikaricp.transactionIsolation="TRANSACTION_SERIALIZABLE"
db.secondary.driver="com.mysql.cj.jdbc.Driver"
db.secondary.url="jdbc:mysql://localhost:3307/anotherdb"
db.secondary.username="user"
db.secondary.password="password"
db.secondary.hikaricp.maximumPoolSize=10
db.secondary.hikaricp.transactionIsolation="TRANSACTION_SERIALIZABLE"
Commentary on Configuration
- The above configuration defines two databases (mydb and anotherdb) with their respective connection properties.
- The
transactionIsolation
property is set toTRANSACTION_SERIALIZABLE
to ensure the highest level of isolation, which is crucial for XA transactions. - By configuring two data sources, we lay the groundwork for a distributed transaction system.
Implementing a Simple XA Transaction
To illustrate the implementation of an XA transaction, consider the following example using Play Framework with Java.
import javax.inject.Inject;
import javax.sql.DataSource;
import javax.transaction.UserTransaction;
import play.db.Database;
import play.db.annotation.Transactional;
public class TransactionService {
private final UserTransaction userTransaction;
private final Database database;
@Inject
public TransactionService(UserTransaction userTransaction, Database database) {
this.userTransaction = userTransaction;
this.database = database;
}
@Transactional
public void executeXaTransaction() {
try {
userTransaction.begin();
// Perform operations on the primary database
executePrimaryDatabaseOperation();
// Perform operations on the secondary database
executeSecondaryDatabaseOperation();
userTransaction.commit();
} catch (Exception e) {
userTransaction.rollback();
throw new RuntimeException("XA Transaction failed, rolled back.", e);
}
}
private void executePrimaryDatabaseOperation() {
// Execute statements for the primary database
}
private void executeSecondaryDatabaseOperation() {
// Execute statements for the secondary database
}
}
Commentary on the Code
- We injected a
UserTransaction
and aDatabase
object, allowing us to manage transactions and interact with databases. - The
@Transactional
annotation indicates that theexecuteXaTransaction
method should be managed by the transaction manager. - If any operation fails within the try block, our catch block ensures that we roll back the transaction to keep data consistent.
Common XA Transaction Issues
Even with the right configuration, you might encounter issues while working with XA transactions in Play. Here are some common problems and their resolutions:
1. Transaction Timeout
A transaction timeout occurs when a transaction takes longer than the configured time limit. You can manage this in your configuration file:
db.default.hikaricp.connectionTimeout=30000 # 30 seconds
2. Resource Locking
Sometimes, if a resource is being accessed concurrently, it can block the transaction. To address this, ensure you are using appropriate locking mechanisms such as pessimistic or optimistic locking based on your use case.
3. Participation Failure
If one of the databases in the XA transaction fails to participate, you need to handle that in your code gracefully. Catch exceptions and ensure a rollback occurs.
Bringing It All Together
Integrating XA transactions within the Play 20 Framework can help maintain data consistency and integrity across multiple resources in distributed systems. Properly configuring your databases and handling transactions is crucial to avoid common pitfalls.
For additional resources on managing XA transactions, check out Play Framework documentation and review the Java Transaction API for broader contexts.
By approaching XA transactions systematically, you can design robust applications that scale efficiently and remain resilient. If you have further questions or need assistance, consider reaching out to the Play Framework community or consult the official documentation.
Happy coding!
Checkout our other articles