Mastering Apache Drill: Creating Custom Functions Simplified
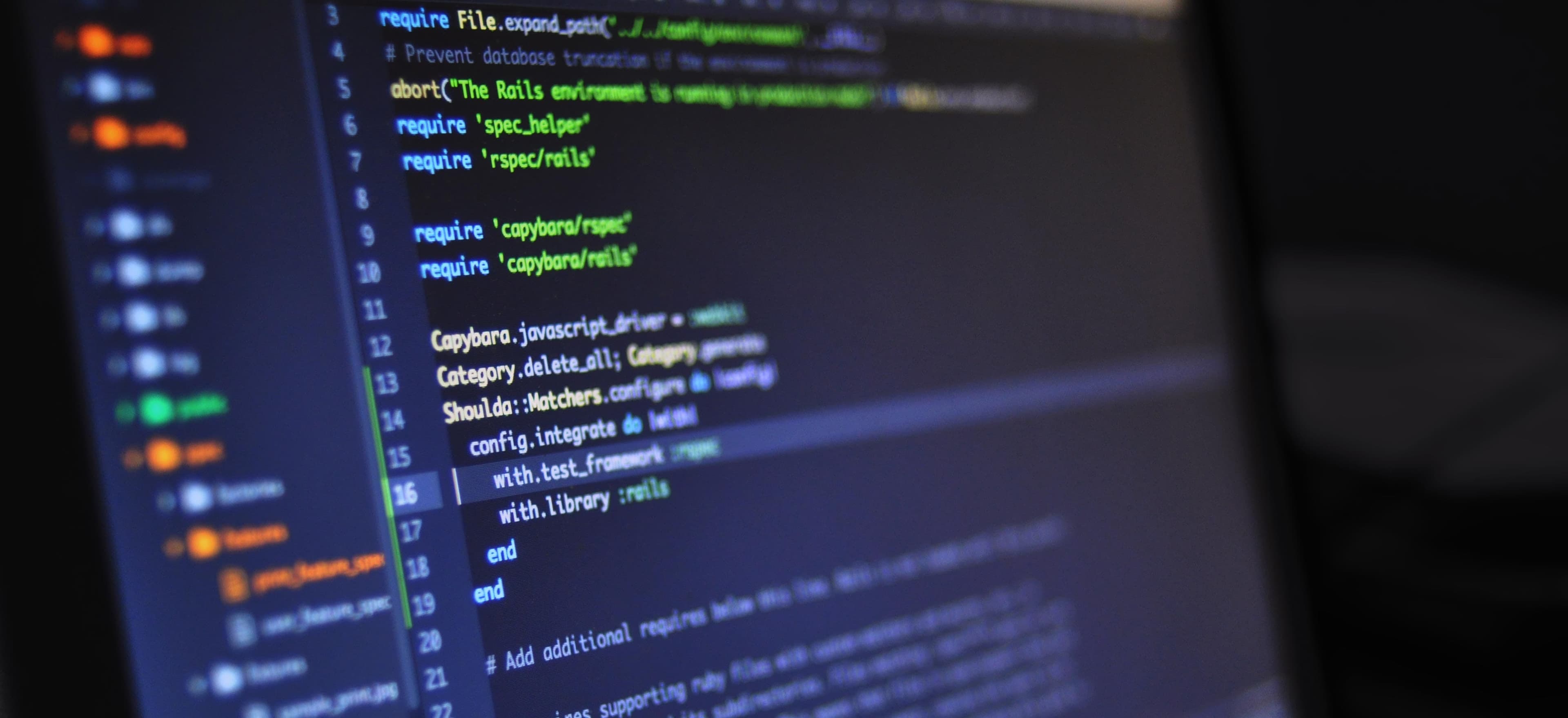
- Published on
Mastering Apache Drill: Creating Custom Functions Simplified
Apache Drill is a powerful framework that enables users to perform interactive analysis across various data sources, including NoSQL databases, Hadoop, and cloud storage. One of the standout features of Drill is its extensibility, allowing developers to create custom functions tailored to specific requirements. In this blog post, we will explore how to create custom functions in Apache Drill, highlighting the essential steps, potential use cases, and providing illustrative code snippets to support your learning.
What is Apache Drill?
Apache Drill is an open-source SQL query engine designed for big data. It allows users to perform analytics on structured and semi-structured data with a SQL-like syntax. One of its key strengths is its ability to query data where it resides, eliminating the need for ETL (Extract, Transform, Load) processes.
For more details, you can check out the Apache Drill official documentation.
Why Create Custom Functions?
Creating custom functions in Apache Drill can enhance the functionality and usability of your queries. Here are a few reasons to consider:
- Specific Use Cases: You may have specific calculations or operations that are not provided out of the box.
- Reusability: Custom functions can be reused across different queries and projects, saving time and effort.
- Performance Optimization: Tailored functions can lead to more efficient queries, particularly if they can reduce the complexity of your SQL statements.
Setting Up Your Environment
Before diving into custom functions, ensure that you have Apache Drill set up on your machine. You can follow the installation guide provided in the Apache Drill documentation.
To verify your setup, run the Drill shell by typing the following command in your terminal:
bin/drill-embedded
This command will launch Drill in embedded mode, allowing you to execute queries directly.
Creating a Custom Function in Apache Drill
Step 1: Understand the Function Structure
Apache Drill allows you to create User Defined Functions (UDFs) using Java. The basic structure of a Drill UDF is as follows:
package com.example;
import org.apache.drill.exec.vector.accessor.Accessors;
import org.apache.drill.exec.vector.accessor.VectorAccessor;
public class MyCustomFunction {
public static void myFunction(VectorAccessor input) {
// Implement the logic of your function here
}
}
Step 2: Define the Function Logic
The function logic you implement will depend on your specific use case. Let's say you want to create a function that calculates the square of a given integer. Here's an example:
package com.example;
import org.apache.drill.exec.vector.accessor.Accessors;
import org.apache.drill.exec.vector.IntVector;
import org.apache.drill.exec.vector.accessor.VectorAccessor;
public class SquareFunction {
public static int square(int value) {
return value * value;
}
}
In this function, we define a simple method called square
, which takes an integer value as input and returns its square.
Step 3: Registering Your Custom Function
Once you have defined your function, the next step is to register it with Apache Drill. You can do this in the Drill shell. For example:
CREATE FUNCTION my_square(int) RETURNS int AS 'com.example.SquareFunction.square';
The SQL command above registers the square
function, making it available for use in queries.
Step 4: Using the Custom Function
Let’s see how to utilize the custom function you've created. You can call it in your SQL queries like any built-in function. Here’s an example:
SELECT my_square(column_name) AS squared_value
FROM my_table;
This query would return the square of the values retrieved from column_name
in my_table
.
Example Code: Creating a Custom String Function
Let's create another example—a function that checks if a string is a palindrome. Here is how you might implement it:
package com.example;
public class StringFunctions {
public static boolean isPalindrome(String str) {
if (str == null) return false;
String reversed = new StringBuilder(str).reverse().toString();
return str.equalsIgnoreCase(reversed);
}
}
Registering the String Function
Similar to the previous example, you need to register this function:
CREATE FUNCTION is_palindrome(varchar) RETURNS boolean AS 'com.example.StringFunctions.isPalindrome';
Using the Palindrome Function
Now, you can use this function in your SQL queries.
SELECT column_name, is_palindrome(column_name) AS is_palindrome
FROM my_table;
This query will return a boolean flag indicating whether the string in column_name
is a palindrome.
Best Practices for Creating Custom Functions
- Keep It Simple: Ideally, your custom functions should be simple and focused on a single task.
- Document Your Code: Provide comments and documentation to enhance understanding, especially if you plan on sharing your functions with others.
- Test Extensively: Validate your functions with various input values to ensure they behave as expected.
- Optimize for Performance: Be mindful of how the function will impact the query performance, particularly with large datasets.
The Bottom Line
Creating custom functions in Apache Drill opens up a world of flexibility and capability for data analysis. Whether you’re calculating squares, reversing strings, or implementing complex business logic, these UDFs can enhance how you interact with your data.
Harness the power of custom functions to tailor your SQL queries to your specific needs. With the capability to create reusable and optimized code, Apache Drill stands out as an analytical powerhouse.
If you want to dive deeper into Apache Drill and explore various topics like performance tuning and data source integration, be sure to check out the Apache Drill documentation. Happy querying!
Checkout our other articles