Troubleshooting JWT Decoding Failures: Common Issues Explained
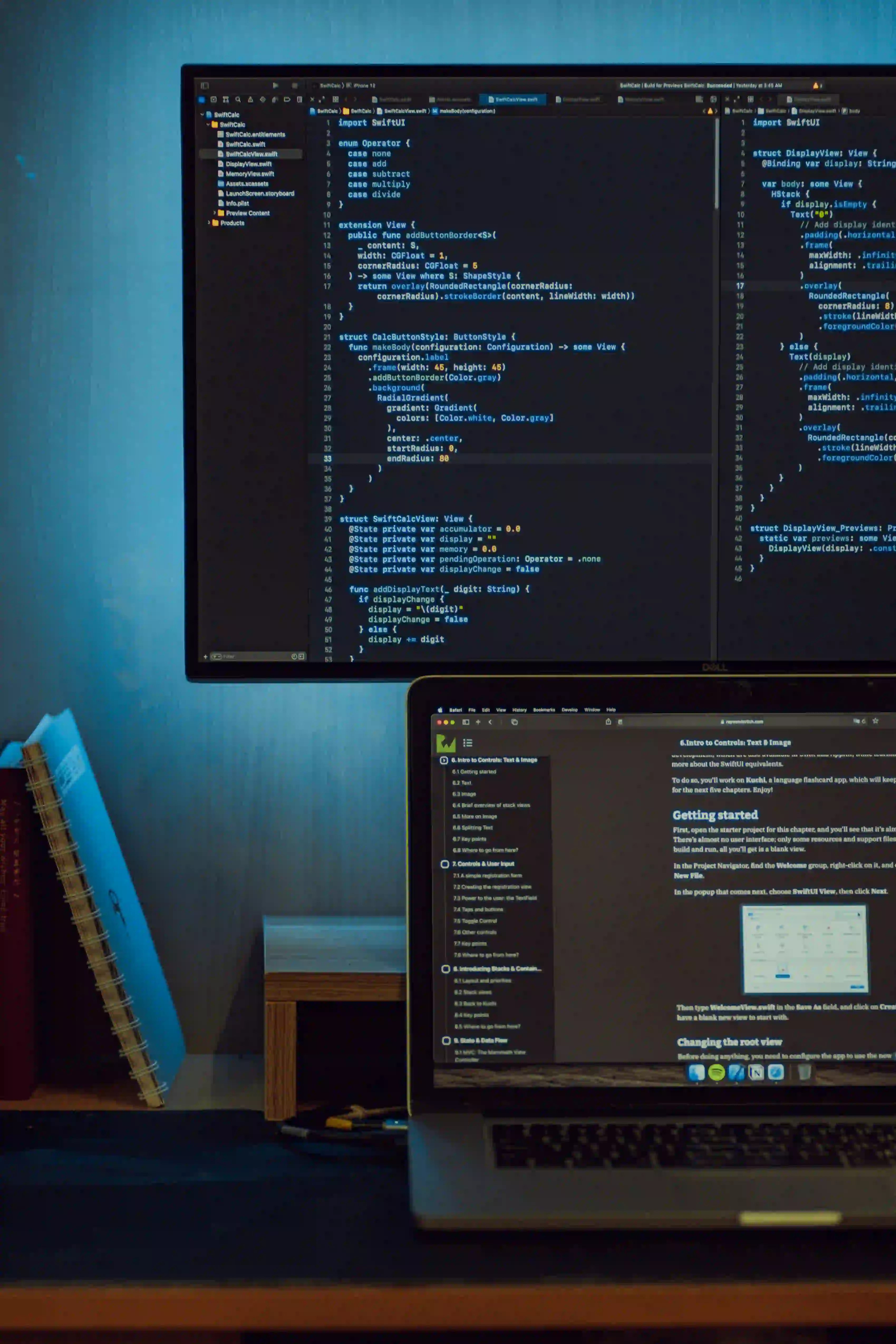
Troubleshooting JWT Decoding Failures: Common Issues Explained
JSON Web Tokens (JWT) are a popular method for securely transmitting information between parties. They enable stateless authentication and are favored in modern web applications. However, decoding JWTs can sometimes result in failures that lead to frustrating debugging sessions. In this blog post, we'll explore common issues related to JWT decoding failures, their causes, and solutions to help you troubleshoot these problems effectively.
Understanding JWT Structure
Before diving into troubleshooting, let’s briefly revisit the structure of a JWT. A JWT is divided into three parts:
- Header: Contains metadata about the token, such as the signing algorithm.
- Payload: Contains the claims (the information you want to transmit).
- Signature: Ensures that the sender of the JWT is who it claims to be and that the message wasn’t changed.
The general format looks like this:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
Each part is Base64Url encoded, and they are separated by dots.
Common Issues and Their Solutions
1. Invalid Token Format
Problem: The token you’re trying to decode may not be in the correct format.
Cause: JWTs must have exactly three parts. If the input string does not contain two periods, it’s likely not a valid JWT.
Solution: Check the format before decoding. Use regular expressions to validate the structure. Here’s a simple Java example:
public boolean isValidJWT(String token) {
String jwtPattern = "^[A-Za-z0-9-_=]+\\.[A-Za-z0-9-_=]+\\.[A-Za-z0-9-_.+/=]*$";
return token.matches(jwtPattern);
}
Why: This regex ensures that your token adheres to the base structure of a JWT, preventing decoding of malformed tokens.
2. Expired Token
Problem: The token might have expired, and your system is rejecting it during the decoding phase.
Cause: JWTs have an expiration time (exp) claim that specifies when they are no longer valid.
Solution: Always check the exp claim against the current time before trusting a decoded token. Here’s how you can do it:
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
...
String secretKey = "your-256-bit-secret";
Claims claims = Jwts.parser()
.setSigningKey(secretKey)
.parseClaimsJws(token)
.getBody();
if (claims.getExpiration().before(new Date())) {
throw new RuntimeException("Token has expired.");
}
Why: Checking the expiration ensures that your application only accepts valid tokens within their valid timeframe, enhancing security.
3. Incorrect Signature
Problem: The signature verification process fails.
Cause: This can occur if the token was modified or if it was signed with a different secret key or algorithm than expected.
Solution: Ensure your application uses the correct secret or public key as well as validating the algorithm. Here’s a Java snippet:
Claims claims = Jwts.parser()
.setSigningKey(secretKey)
.parseClaimsJws(token)
.getBody();
Why: This code confirms that the token is valid and untampered with by verifying the signature against the known secret key.
4. Unsupported Signing Algorithm
Problem: The JWT is signed with an algorithm your library does not support.
Cause: Libraries have specific sets of supported algorithms, and using an unsupported algorithm can lead to decoding issues.
Solution: Verify that the algorithm specified in the header is supported by your decoding library. You can restrict allowed algorithms in the parsing configurations:
JwtParser parser = Jwts.parserBuilder()
.setSigningKey(secretKey)
.requireAudience("your-audience")
.build();
Claims claims = parser.parseClaimsJws(token).getBody();
Why: This usage ensures that the token is only decoded using the correct configuration, adding another layer of security.
5. Altered Payload
Problem: The payload portion of your JWT has been altered or tampered with after signing.
Cause: If the token was intercepted and modified, the signature verification will fail during decoding.
Solution: Always verify the signature as mentioned earlier. Never rely on payload data without first confirming its integrity. Directly use a library’s built-in functions which will often include payload validation automatically.
6. UTF-8 Encoding Issues
Problem: Issues can arise from improperly encoded characters in the JWT string.
Cause: Tokens may be encoded in different character sets, leading to decoding issues in some implementations.
Solution: Ensure your tokens are correctly encoded in UTF-8. Before decoding, confirm the encoding using the following:
String token = new String(yourByteArray, StandardCharsets.UTF_8);
Why: By enforcing UTF-8 encoding, you can avoid potential mismatches during decoding that could lead to data corruption or invalid tokens.
7. Invalid JSON in Payload
Problem: The payload might not be a valid JSON object.
Cause: Any issue in the structure of the JSON payload can result in decoding failures.
Solution: Always ensure that the payload can be parsed into a valid JSON. A simple way to test this in Java is:
try {
JSONObject json = new JSONObject(payload);
} catch (JSONException e) {
throw new RuntimeException("Invalid JSON in the payload.", e);
}
Why: Validating the payload as a JSON object ensures that downstream processes can interpret it without issues.
In Conclusion, Here is What Matters
Decoding JWTs can be straightforward, but failures often arise due to common pitfalls. By understanding the structure and potential issues of JWTs, developers can quickly troubleshoot these problems. Whether it's validating format, expiry, signature, or even the JSON structure, being proactive about these checks can save time and enhance the security of your application.
For further reading on JWT and security practices, check out resources like JWT.io or the official documentation of libraries like Java JWT.
Keep coding securely, and always validate your JWTs!