Maximizing Efficiency: Common Data Management Pitfalls
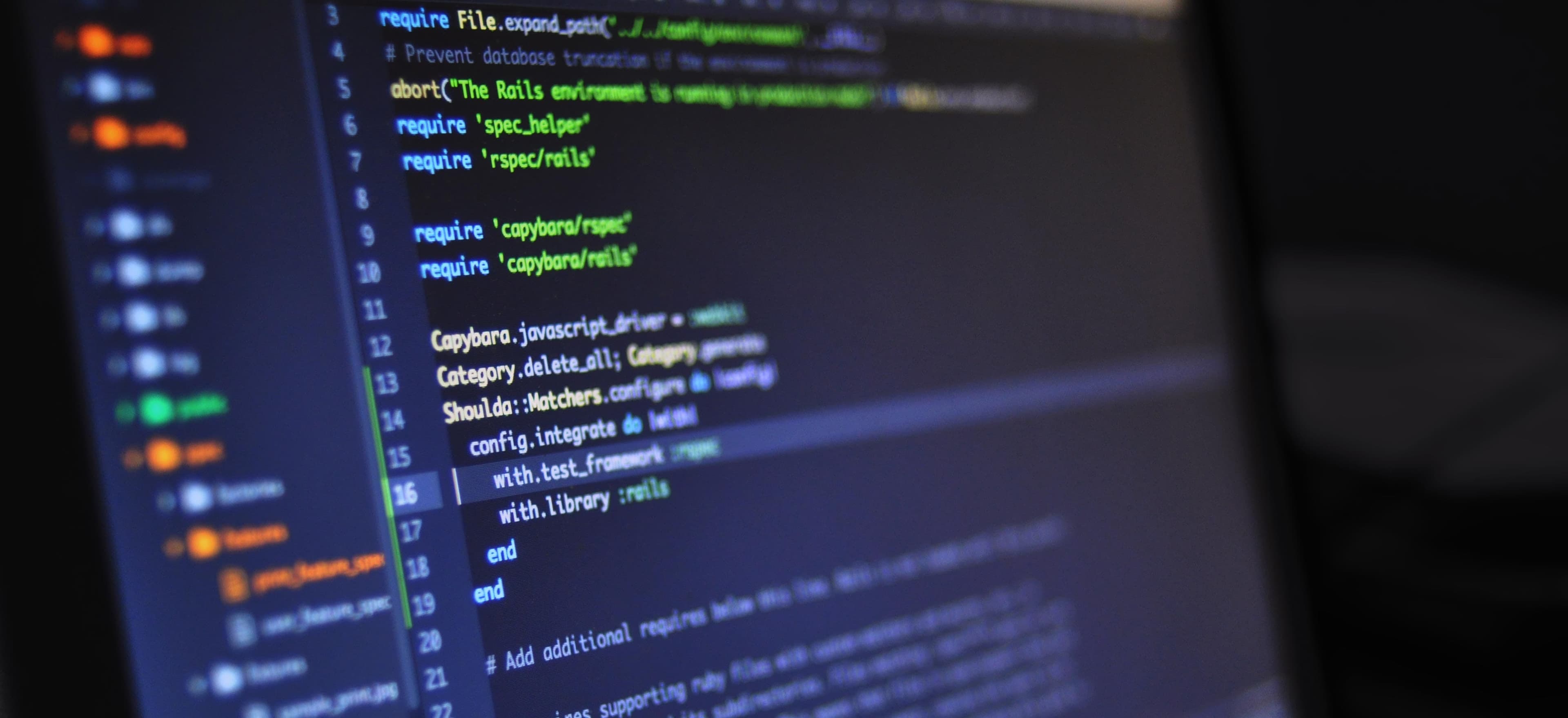
- Published on
Maximizing Efficiency: Common Data Management Pitfalls
In today's data-driven world, effective data management is more crucial than ever. Companies rely on data for decision-making and strategic planning, which means that mishandling data can lead to substantial losses. In this blog post, we'll discuss common data management pitfalls, how to identify them, and practical solutions to avoid the pitfalls that can hinder your data efficiency.
Understanding Data Management
Before diving into the pitfalls, it's essential to grasp what data management encompasses. Data management involves acquiring, storing, organizing, and maintaining data in an efficient manner. Proper data management relies on a combination of technology, best practices, and effective governance. This includes establishing strong policies, leveraging appropriate tools, and nurturing a data-driven culture.
The Common Pitfalls
1. Lack of Data Governance
Issue: One common pitfall is the absence of data governance. Without established guidelines and policies for data management, organizations may suffer from inconsistent data practices.
Why It Matters: Good governance ensures that data is categorized, secured, and accessible. Without this, organizations face redundancy, inefficiency, and vulnerabilities to data breaches.
Solution: Implement a data governance framework. This involves defining roles and responsibilities, creating data quality standards, and ensuring compliance with regulations. For practical examples, refer to resources like DAMA which offers guidelines for data management frameworks.
2. Poor Data Quality
Issue: Data may frequently be inaccurate, incomplete, or outdated. Poor data quality can arise from various sources, including human error or integration issues across systems.
Why It Matters: Inaccurate data leads to faulty analytics and ultimately misguided business decisions.
Solution: Regularly implement data cleansing processes. For example, utilizing tools like Apache NiFi can automate data quality checks. Below is a simple Java-based code snippet that demonstrates basic data validation:
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class DataValidator {
public static boolean isValidEmail(String email) {
String emailRegex = "^[A-Za-z0-9+_.-]+@(.+)$";
Pattern pattern = Pattern.compile(emailRegex);
Matcher matcher = pattern.matcher(email);
return matcher.matches();
}
public static void main(String[] args) {
String testEmail = "test@example.com";
if (isValidEmail(testEmail)) {
System.out.println("Valid email address");
} else {
System.out.println("Invalid email address");
}
}
}
Explanation: This code validates whether the given string follows the email format. Validating data before processing can significantly reduce the risk of integrating incorrect inputs.
3. Siloed Data
Issue: Data silos occur when data is isolated in individual departments or systems without interconnectivity.
Why It Matters: When departments hoard data, they limit collaboration and create inconsistency across the organization.
Solution: Foster a culture of data sharing. Using centralized data repositories or implementing a typical data lake architecture can help achieve this. A data lake allows organizations to store structured and unstructured data in one location, making it accessible and useful for various purposes.
4. Overlooking Data Security
Issue: Many organizations underestimate the importance of securing their data, leading to potential breaches.
Why It Matters: Data breaches can damage reputations and lead to legal consequences and financial loss.
Solution: Implement robust security measures including:
- Role-based access control
- Data encryption
- Regular security audits
Using Java, you can implement a secure data storage method with basic encryption like so:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class EncryptionUtil {
public static String encrypt(String data, SecretKey secretKey) throws Exception {
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encryptedData = cipher.doFinal(data.getBytes());
return Base64.getEncoder().encodeToString(encryptedData);
}
public static void main(String[] args) throws Exception {
String originalData = "Sensitive Data";
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(128); // You can choose key size
SecretKey secretKey = keyGen.generateKey();
String encryptedData = encrypt(originalData, secretKey);
System.out.println("Encrypted Data: " + encryptedData);
}
}
Explanation: This Java code encrypts sensitive data using AES encryption. It’s a fundamental step in data security—ensuring that unauthorized access is prevented.
5. Ignoring Analytics and Visualization
Issue: Some organizations treat data as a purely operational necessity rather than as a strategic asset.
Why It Matters: Ignoring analytics limits organizations' capabilities to derive insights and value from their data.
Solution: Invest in proper analytics tools and visualization software such as Tableau or Power BI. They empower organizations to make data-driven decisions. Moreover, utilizing libraries like Apache Spark with Java can improve data processing speeds, allowing deeper insights.
6. Not Training Employees
Issue: Employees may not have the necessary skills to leverage data effectively.
Why It Matters: A well-trained team is pivotal to maximizing the potential of data assets.
Solution: Provide ongoing training on data management tools and practices. Consider investing in workshops, seminars, or online courses to strengthen your team's data competencies.
Bringing It All Together
Maximizing efficiency in data management requires vigilance against common pitfalls. By establishing data governance, improving data quality, fostering data sharing, ensuring data security, utilizing analytics, and investing in employee training, organizations can create a more effective data management strategy.
Staying informed about industry-best practices and continually refining your processes leads to better decision-making and ultimately, better business outcomes.
For further reading on effective data management practices, you might find The Data Warehouse Institute (TDWI) useful.
By recognizing these pitfalls and acting on them, organizations can not only avoid inefficiencies but also gain invaluable insights from their data, paving the way for successful outcomes.
Happy coding, and may your data always be clean and secure!
Checkout our other articles