Avoid These Common Pitfalls in Password Storage Security
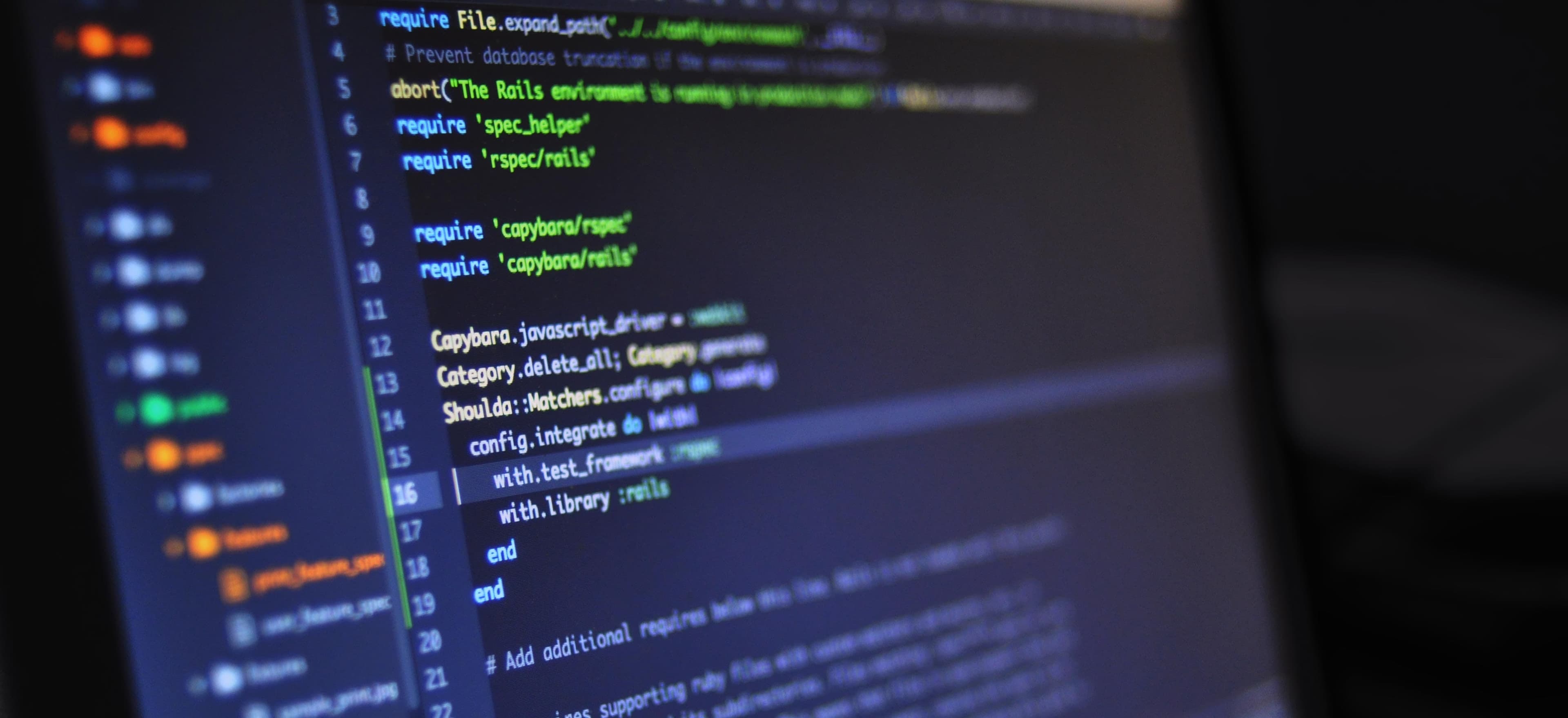
- Published on
Avoid These Common Pitfalls in Password Storage Security
In an era where data breaches are a common occurrence, ensuring the security of user passwords is paramount. As developers, we need to prioritize sound practices in password storage to keep user data safe from malicious actors. In this blog post, we'll examine common pitfalls in password storage security, discuss best practices, and look at examples of how to implement these strategies in Java.
Why Password Storage Matters
Before diving into the common pitfalls, it’s essential to understand why password storage is critical:
- User Trust: Users expect that their data will be stored securely. A data breach can lead to the loss of trust, impacting your application’s reputation.
- Legal Compliance: Many jurisdictions impose regulations on how personal data should be stored. Non-compliance can lead to hefty fines and legal repercussions.
- Avoiding Financial Loss: A security breach can be financially devastating for a company, through both direct losses and damage to brand integrity.
Common Pitfalls in Password Storage
1. Storing Plain-text Passwords
The Pitfall: Some developers might think that simply storing passwords without any encryption or hashing is acceptable. This is akin to leaving the front door to your home wide open.
The Fix: Always hash and salt passwords before storage. Hashing converts the password into a fixed-size string of characters, which makes it difficult to reverse-engineer back to the original password. Salting adds an extra layer of security by appending a unique random string to each password before hashing.
Example Code Snippet
Here’s how to securely hash a password using Java’s built-in libraries.
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.util.Base64;
public class PasswordUtil {
public static String hashPassword(String password) {
String salt = generateSalt();
return salt + ":" + hashWithSalt(password, salt);
}
private static String hashWithSalt(String password, String salt) {
try {
MessageDigest md = MessageDigest.getInstance("SHA-256");
md.update((salt + password).getBytes());
byte[] byteData = md.digest();
return Base64.getEncoder().encodeToString(byteData);
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException(e);
}
}
private static String generateSalt() {
SecureRandom sr = new SecureRandom();
byte[] salt = new byte[16];
sr.nextBytes(salt);
return Base64.getEncoder().encodeToString(salt);
}
}
In this example:
- generateSalt(): Generates a new random salt for the password.
- hashPassword(String password): Concatenates the salt with the password and returns the hash stored as "salt:hash".
2. Using Weak Hashing Algorithms
The Pitfall: Some developers may opt for outdated or weak hashing algorithms like MD5 or SHA-1. These algorithms are vulnerable to various attacks, including collision attacks.
The Fix: Use stronger algorithms designed specifically for password hashing, such as bcrypt, Argon2, or PBKDF2. These algorithms are resistant to brute-force attacks because they are deliberately slow.
Example Code Snippet
Below is an example of using the BCrypt hashing function in Java with the JBCrypt library.
import org.mindrot.jbcrypt.BCrypt;
public class BCryptUtil {
public static String hashPassword(String password) {
return BCrypt.hashpw(password, BCrypt.gensalt());
}
public static boolean checkPassword(String password, String hashed) {
return BCrypt.checkpw(password, hashed);
}
}
In this snippet:
- hashPassword(String password): Generates a BCrypt hash of the provided password.
- checkPassword(String password, String hashed): Validates the provided password against the stored hash.
For further reading on strong hashing algorithms referred above, you can visit this resource.
3. Reusing Salts
The Pitfall: Developers may mistakenly think reusing the same salt across all users adds convenience and is sufficient for security efforts. This undermines the unique hashing mechanism and makes passwords more vulnerable.
The Fix: Generate a unique salt for each password. This ensures that even if two users have the same password, their hashes will still differ, effectively mitigating the risks of rainbow table attacks.
4. Failing to Update Passwords Regularly
The Pitfall: Believe it or not, some applications allow password lifetimes to exceed reasonable amounts. This can lead to scenarios where compromised passwords remain valid indefinitely.
The Fix: Establish a password expiration policy. Encourage users to change their passwords regularly, especially if you suspect they might have been compromised.
5. Neglecting Security Questions
The Pitfall: Utilizing easily guessable security questions can be more harmful than helpful. For example, using questions like "What is your mother's maiden name?" and allowing multiple users to answer can lead to vulnerabilities.
The Fix: Use robust and personalized security questions or consider alternative verification methods such as two-factor authentication (2FA). 2FA requires users to provide two distinct forms of identification before accessing their account, significantly increasing security.
6. Not Using HTTPS
The Pitfall: Sending passwords over HTTP can expose them to sniffing attacks. If attackers can view the raw HTTP traffic, they can retrieve user credentials easily.
The Fix: Always ensure that your application uses HTTPS to encrypt data in transit. This means that even if an attacker intercepts the traffic, they are unable to read the contents.
You may also want to read about the importance of HTTPS in this insightful article.
7. Insufficient Password Length
The Pitfall: Setting a minimum character length for passwords that is too low (like 6 characters) makes it easier for attackers using brute force techniques to crack passwords.
The Fix: Require a minimum of at least 12 to 14 characters and encourage users to combine upper-case letters, lower-case letters, numbers, and special characters for stronger passwords.
My Closing Thoughts on the Matter
Password storage security is not something to be taken lightly. Avoiding these common pitfalls ensures that user data remains secure and that your software retains its credibility in today’s digital landscape.
The examples provided illustrate effective ways to hash and manage passwords securely in Java. By implementing strong hashing algorithms, using unique salts, and maintaining secure connection channels, you can vastly improve the security of your application.
Remember, security is a continuous effort. Regularly review and update your practices to stay ahead of potential threats.
By prioritizing these practices, not only will you safeguard your application, but you’ll also foster trust and safety for your users.
Checkout our other articles