Optimizing Nutch Performance with Cassandra for Web Crawling
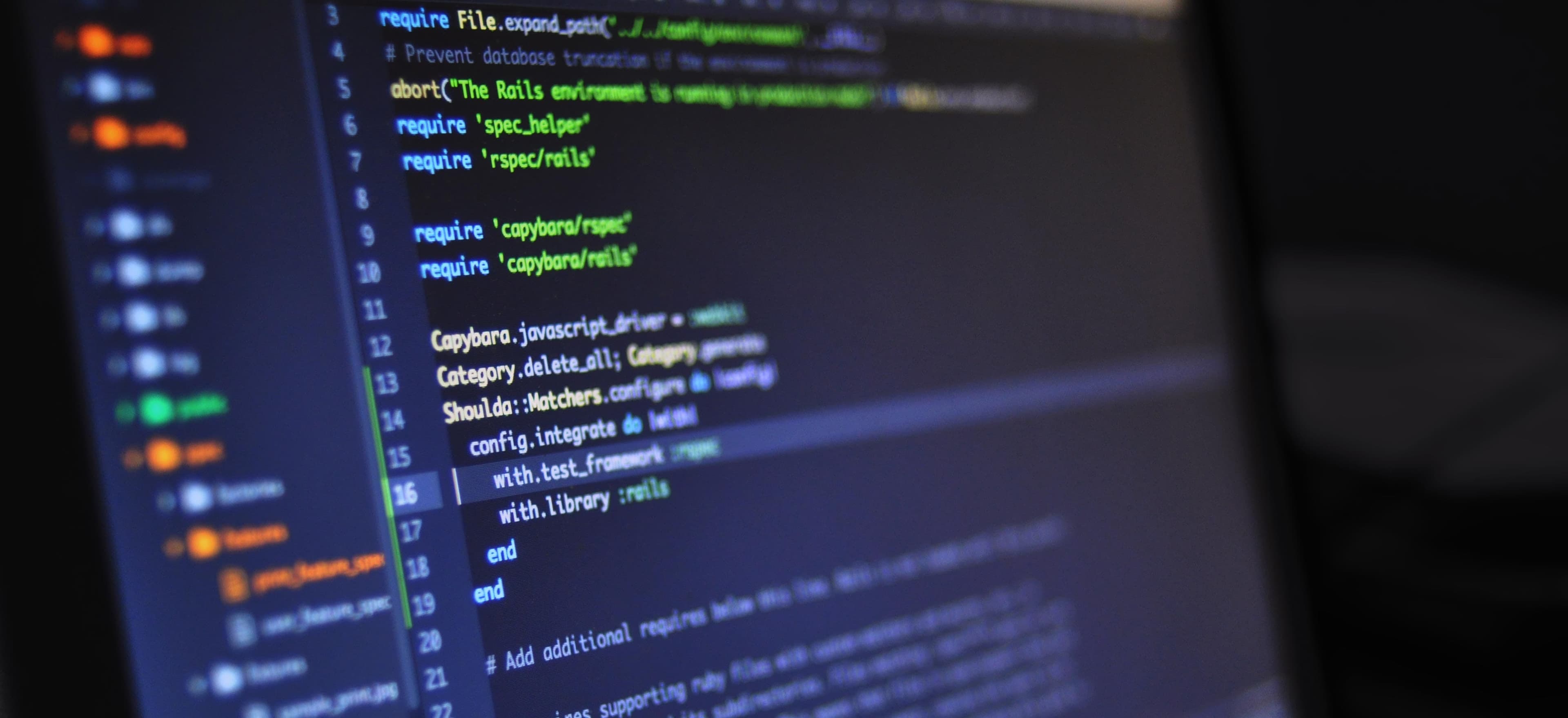
- Published on
Optimizing Nutch Performance with Cassandra for Web Crawling
Web crawling is a fundamental component of search engines, enabling them to index the vast amount of data present on the internet. Apache Nutch is a highly extensible and scalable open-source web crawler based on Java. When combined with a high-performance database like Apache Cassandra, it can efficiently manage and store large volumes of web crawling data. This blog post will delve into optimizing Nutch's performance with Cassandra, specifically focusing on best practices, code snippets, and architectural insights.
Understanding the Nutch and Cassandra Relationship
Apache Nutch is built to handle the crawling, indexing, and serving of web content. In contrast, Apache Cassandra is a distributed NoSQL database designed for handling large amounts of data across many servers with no single point of failure.
The synergy between Nutch and Cassandra lies in:
- Scalability: Cassandra can scale horizontally, which means it can handle increased loads by adding more machines.
- Availability: It offers high availability without downtime, making it ideal for web crawling operations.
- Performance: With its optimized write paths and data replication strategies, Cassandra can handle high-speed data ingestion which is crucial for crawling.
Setting Up Nutch with Cassandra
Before diving into performance optimization, ensure that you have Nutch and Cassandra installed and configured. You can follow the official Nutch installation guide, while for Cassandra, refer to the Cassandra Quick Start Guide.
Nutch Configuration for Cassandra
After installing both applications, modify your Nutch configuration files to integrate with Cassandra. The primary configuration file you will need to edit is nutch-site.xml
. Below is an essential snippet you'll want to add or modify:
<property>
<name>cassandra.hosts</name>
<value>localhost</value>
</property>
<property>
<name>cassandra.keyspace</name>
<value>nutch</value>
</property>
<property>
<name>cassandra.port</name>
<value>9042</value>
</property>
In this code block:
cassandra.hosts
: Specifies the host where Cassandra is running.cassandra.keyspace
: Defines the keyspace in which Nutch will store its crawling data.cassandra.port
: Indicates the port used for communication with the Cassandra server.
Creating Keyspaces and Tables
Before Nutch can store data, you'll need to create a relevant keyspace and tables in Cassandra. Here’s an example of how to create a keyspace and a table suitable for web crawling:
CREATE KEYSPACE nutch WITH replication = { 'class': 'SimpleStrategy', 'replication_factor': 1 };
CREATE TABLE nutch.crawled_links (
url text PRIMARY KEY,
content blob,
fetched_at timestamp
);
Why This Matters
- Keyspace Definition: The
nutch
keyspace enables organization and management of data more effectively. - Table Structure: The
crawled_links
table allows storing each URL's content and the timestamp it was fetched. This is essential for tracking the crawl.
Performance Optimization Techniques
1. Tuning Nutch Crawl Settings
The performance of Nutch can be significantly affected by its crawler settings. Here are some key configurations to consider:
- Max Fetching Threads: Set the maximum number of threads in
nutch-default.xml
:
<property>
<name>http.threads.fetch</name>
<value>10</value>
</property>
This setting will allow more simultaneous fetches, increasing the crawler's speed. However, beware of server overload.
- Politeness Delay: This value determines how often the crawler sends requests to a server. Adjust it based on the rate limiting of the sites you crawl.
<property>
<name>http.robots.agents</name>
<value>my-crawler</value>
</property>
<property>
<name>http.robots.max.crawl.delay</name>
<value>5000</value> <!-- milliseconds -->
</property>
2. Batch Processing for Cassandra Writes
When dealing with large batches of data, writing to Cassandra should be carefully structured. Utilizing batch processing can significantly optimize performance:
PreparedStatement pstmt = session.prepare("INSERT INTO nutch.crawled_links (url, content, fetched_at) VALUES (?, ?, ?)");
BatchStatement batch = new BatchStatement();
for (WebPage page : pages) {
batch.add(pstmt.bind(page.getUrl(), page.getContent(), page.getFetchedAt()));
}
session.execute(batch);
Why Batch Writes?
- Reduced Overhead: Batch writes reduce the number of network round trips required to communicate with the database.
- Improved Throughput: They inherently improve the speed of data insertion, essential for a crawler handling thousands of pages simultaneously.
3. Clustering Cassandra Nodes
As your crawling operations scale, consider clustering multiple Cassandra nodes. This allows you to distribute read and write requests across multiple instances, balancing the workload.
4. Use of Compression
Cassandra supports compression, which can significantly reduce the size of your stored data.
CREATE TABLE nutch.crawled_links (
url text PRIMARY KEY,
content blob,
fetched_at timestamp
) WITH compression = {'sstable_compression': 'LZ4Compressor'};
Benefits of Compression:
- Storage Efficiency: Reduces the amount of storage needed for large datasets.
- Faster I/O Operations: Compressed data can lead to lower I/O operations, thereby enhancing read performance.
Monitoring and Maintenance
Once you've implemented optimizations, monitoring your system becomes vital. Use tools like DataStax Monitoring or Prometheus to keep an eye on Nutch and Cassandra operations.
Regularly monitor:
- Latency: Measure how fast pages are fetched and stored.
- Error Rates: Analyze any errors occurring due to network timeouts or Cassandra write issues.
- Resource Utilization: Observe CPU, memory, and disk I/O usage to identify bottlenecks.
Bringing It All Together
Integrating Apache Nutch with Apache Cassandra offers a robust solution for optimizing web crawling operations. With proper configurations, careful architectural decisions, and performance optimizations, your crawling infrastructure can efficiently handle the complexities of web data management.
By following the outlined methods, you can ensure a smoother crawling process, enhanced data handling capability, and ultimately a more effective search engine indexing system.
For further insights into configuring Nutch with larger environments, you can refer to the Nutch and Cassandra documentation.
Happy crawling!