Understanding RESTful API Errors: Common Pitfalls to Avoid
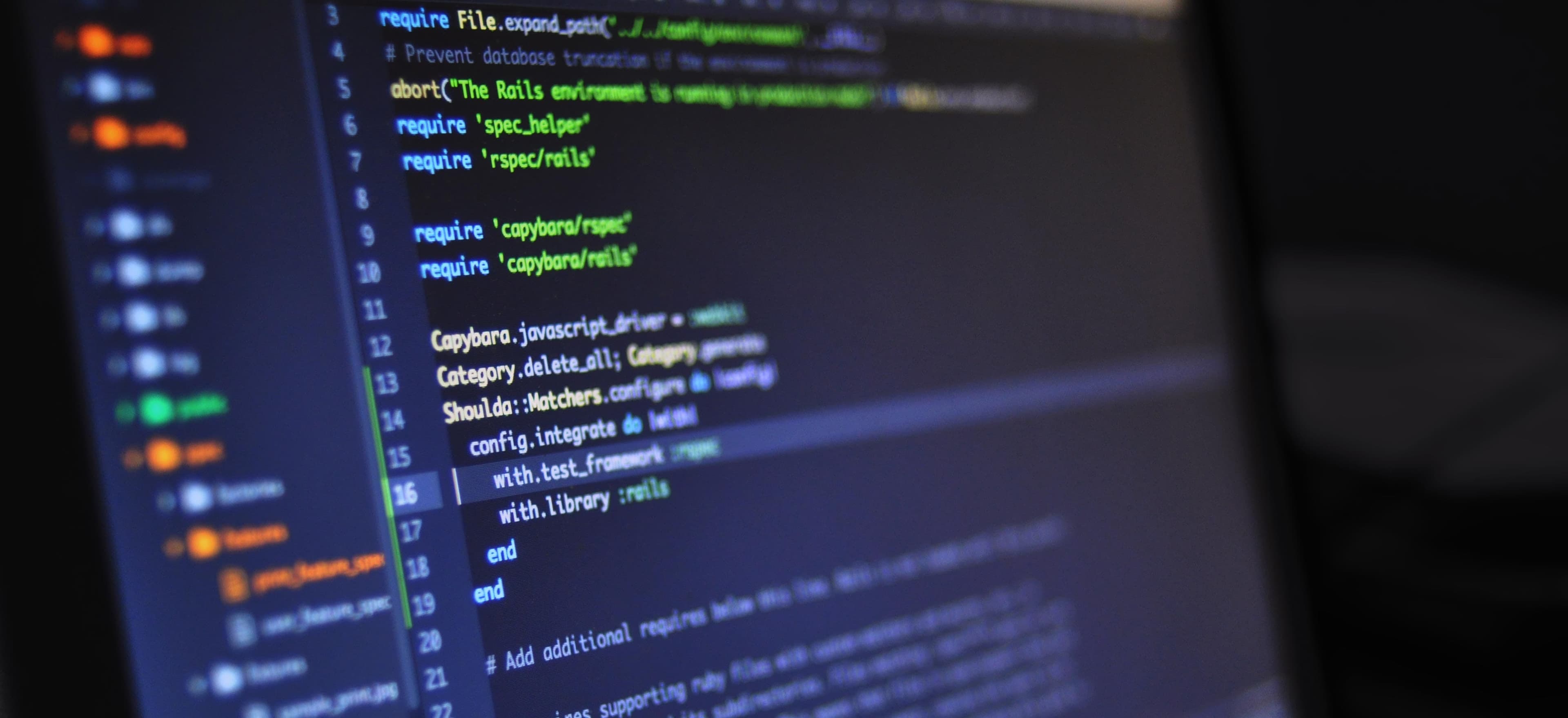
- Published on
Understanding RESTful API Errors: Common Pitfalls to Avoid
In the ever-evolving world of software development, RESTful APIs (Representational State Transfer APIs) have become a cornerstone for building scalable applications. While developing or consuming these APIs, encountering errors is inevitable. This blog post aims to shed light on common pitfalls that developers face when dealing with RESTful API errors. Understanding these can greatly improve the software development lifecycle, making it smoother and more efficient.
What is a RESTful API?
A RESTful API allows different systems to communicate over the internet via HTTP methods like GET, POST, PUT, and DELETE. REST stands for Representational State Transfer, which emphasizes stateless communication between the client and server. It utilizes standard HTTP response codes to indicate the outcome of requests.
Importance of Error Handling
Robust error handling is crucial in ensuring a seamless user experience and keeping the API service running smoothly. APIs are often the backbone of applications, and any hiccup in communication can lead to a frustrating experience for users. This makes it essential to not only identify but also handle errors effectively.
Common RESTful API Errors and Their Causes
1. HTTP Status Code Errors
HTTP status codes are the first indicators of an error. Here are some common status codes you may encounter:
- 400 Bad Request: The server could not understand the request due to invalid syntax.
- 401 Unauthorized: Authentication is required and has failed or has not been provided.
- 403 Forbidden: The server understands the request but refuses to authorize it.
- 404 Not Found: The server can't find the requested resource.
- 500 Internal Server Error: The server encountered an unexpected condition.
Why it Matters: Using the correct HTTP status codes not only helps in effective debugging but also provides a meaningful response to the client, making it easier to correct errors.
Example: Handling a 404 Error in Java
@RequestMapping(value = "/api/resource/{id}", method = RequestMethod.GET)
public ResponseEntity<Resource> getResource(@PathVariable("id") Long id) {
Resource resource = resourceService.findById(id);
if (resource == null) {
return new ResponseEntity<>(HttpStatus.NOT_FOUND);
}
return new ResponseEntity<>(resource, HttpStatus.OK);
}
In the example above, the REST API checks if a resource exists. If it does not, a 404 status code is returned with the appropriate message.
2. Incorrect API Endpoint
Accidentally invoking the wrong endpoint can lead to unnecessary errors. This often happens due to typos or outdated documentation.
Why it Matters: A well-documented API should contain clear details about available endpoints, so developers avoid errors by knowing what to expect.
Example: API Endpoint Documentation
Consider creating an API documentation as part of your project. You can use tools like Swagger or Postman to provide interactive documentation for your API consumers. This will serve to minimize confusion about which endpoints to use.
3. Missing or Incorrect Parameters
Omitting required query parameters or sending wrong types can lead to various errors. Most APIs require certain parameters to be present in the request body or URL.
Example: Validation in Java
@PostMapping("/api/resource")
public ResponseEntity<String> createResource(@RequestBody Resource resource) {
if (resource.getName() == null || resource.getName().isEmpty()) {
return new ResponseEntity<>("Name is required", HttpStatus.BAD_REQUEST);
}
resourceService.save(resource);
return new ResponseEntity<>("Resource created successfully", HttpStatus.CREATED);
}
This code checks if the required field 'Name' is present before proceeding with resource creation. If it's missing, a 400 error is returned to the client.
4. Authentication and Authorization Issues
Authentication failures can cause significant issues, especially when consuming third-party APIs. It's essential to define a clear strategy to manage tokens and user credentials.
Why it Matters: Understanding authentication mechanisms (like OAuth, JWT) is crucial to ensure that your API is secure while remaining accessible.
Example: Adding Bearer Token Authentication
@RestController
@RequestMapping("/api")
public class MyController {
@GetMapping("/secure-data")
public ResponseEntity<String> getSecureData(@RequestHeader("Authorization") String authHeader) {
if (!isValidToken(authHeader)) {
return new ResponseEntity<>("Unauthorized", HttpStatus.UNAUTHORIZED);
}
return new ResponseEntity<>("Secure Data", HttpStatus.OK);
}
private boolean isValidToken(String token) {
// Logic to validate the token
return true; // Simplified for example
}
}
In this snippet, validation checks are performed against the provided Authorization token before accessing secure data.
Best Practices for Avoiding RESTful API Errors
1. Implement Comprehensive Logging
Logging helps you identify exactly where an error originated. Ensure you log both the request and response, along with the relevant status codes. Utilize logging frameworks like SLF4J or Log4j in Java applications.
2. Use Proper Status Codes
Always return the correct HTTP status codes in your responses. This not only aids in debugging but also improves the user experience when consuming your API.
3. Validate Inputs
Never trust any input from clients. Validate all parameters and request bodies to protect your system from invalid data and potential attacks.
4. Document Your API
Keep your API well documented. Use tools to auto-generate documentation from your codebase to maintain accuracy. This transparency will help in diagnosing issues faster.
5. Regular Updates and Maintenance
Make sure to update both your API and its documentation regularly. Keep an eye on deprecating functionalities and communicate these changes clearly to your users.
The Bottom Line
Handling RESTful API errors effectively can make a significant difference in the quality and reliability of your application. By understanding common pitfalls, proper error handling strategies, and adopting best practices, developers can ensure a smoother integration experience.
Whether you are a seasoned developer or just starting in API development, keeping these points in mind will undoubtedly help you create more robust APIs.
Further Reading
- Learning REST APIs: A Beginner’s Guide
- Best Practices for API Documentation
Being aware of common errors, their causes, and best practices for error management will not only enhance your skills but also lead to delivering superior products. Get out there and start coding responsibly!