Finding the Middle Element of a Linked List in One Pass
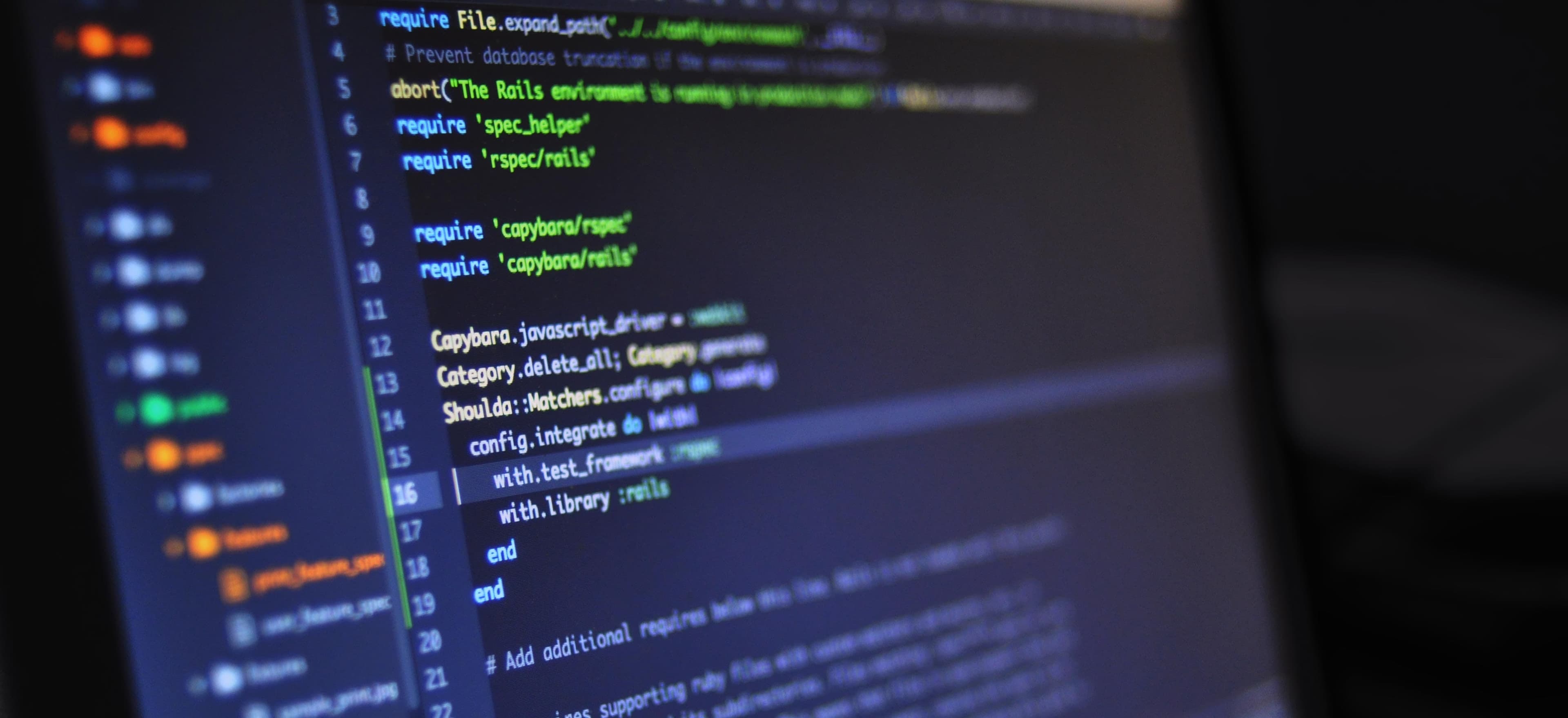
- Published on
Finding the Middle Element of a Linked List in One Pass
In data structures and algorithms, a linked list is a fundamental data structure that consists of nodes. Each node contains data and a reference (link) to the next node in the sequence. One common operation we perform on linked lists is finding the middle element. In this blog post, we will delve into how to efficiently find the middle element of a linked list in one pass, using the two-pointer technique.
Understanding the Problem
Before we dive into the solution, let’s define what we mean by the "middle element" of a linked list. For a linked list with an odd number of elements, the middle is straightforward—it's the actual center element. However, for an even number of elements, there are two central nodes, and we typically choose the second one as the "middle."
For instance, given a linked list like:
1 -> 2 -> 3 -> 4 -> 5
The middle element is 3
.
For an even-length list like:
1 -> 2 -> 3 -> 4
The middle will be 3
as well.
The Two-Pointer Technique
To find the middle of the linked list in one pass, we will use a two-pointer technique. The idea is to use two pointers: one (slow
) will move one step at a time, while the other (fast
) will move two steps at a time. When the fast
pointer reaches the end of the list, the slow
pointer will be at the middle.
Why Use Two Pointers?
- Efficiency: This approach only requires O(n) time complexity and O(1) space complexity, as we don’t need any extra data structures.
- Simplicity: The implementation is straightforward, making our code easy to read and maintain.
Implementation
Below is a sample implementation of the two-pointer technique in Java.
class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
class LinkedList {
Node head;
public void add(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
return;
}
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
public int findMiddle() {
Node slow = head;
Node fast = head;
// Traverse the list
while (fast != null && fast.next != null) {
slow = slow.next; // Move slow by one
fast = fast.next.next; // Move fast by two
}
// When fast reaches the end, slow is at the middle
return slow.data;
}
}
Code Explanation
- Node Class: Represents each element of the linked list. It holds data and a reference to the next node.
- add Method: Adds a new node to the end of the linked list.
- findMiddle Method:
- Initially, both
slow
andfast
pointers point to the head of the linked list. - The
while
loop checks thatfast
andfast.next
aren't null to ensure we do not encounter a NullPointerException. - In each iteration,
slow
moves one step, whilefast
moves two steps. - When the
fast
pointer reaches the end,slow
will be pointing at the middle node.
- Initially, both
How to Use the Code
To use this LinkedList
class, simply create an instance, add elements, and call the findMiddle
method:
public class Main {
public static void main(String[] args) {
LinkedList list = new LinkedList();
list.add(1);
list.add(2);
list.add(3);
list.add(4);
list.add(5);
System.out.println("Middle Element: " + list.findMiddle());
// For an even-length linked list
LinkedList evenList = new LinkedList();
evenList.add(1);
evenList.add(2);
evenList.add(3);
evenList.add(4);
System.out.println("Middle Element of Even List: " + evenList.findMiddle());
}
}
Execution Result
When you run the above code, you will get the output:
Middle Element: 3
Middle Element of Even List: 3
Final Considerations
Finding the middle element of a linked list is a common problem in programming interviews and competitive programming. Using the two-pointer approach not only enhances efficiency but also simplifies the task. By understanding how the slow and fast pointers work together, you can tackle variations of this problem in various contexts.
For further exploration on linked lists and related problems, consider reading about:
- Reversing a Linked List
- Detecting a Cycle in a Linked List
Happy coding! If you have any questions or want to share your experiences with linked lists, feel free to leave a comment below!
Checkout our other articles