Understanding Spring Boot's Common Configuration Pitfalls
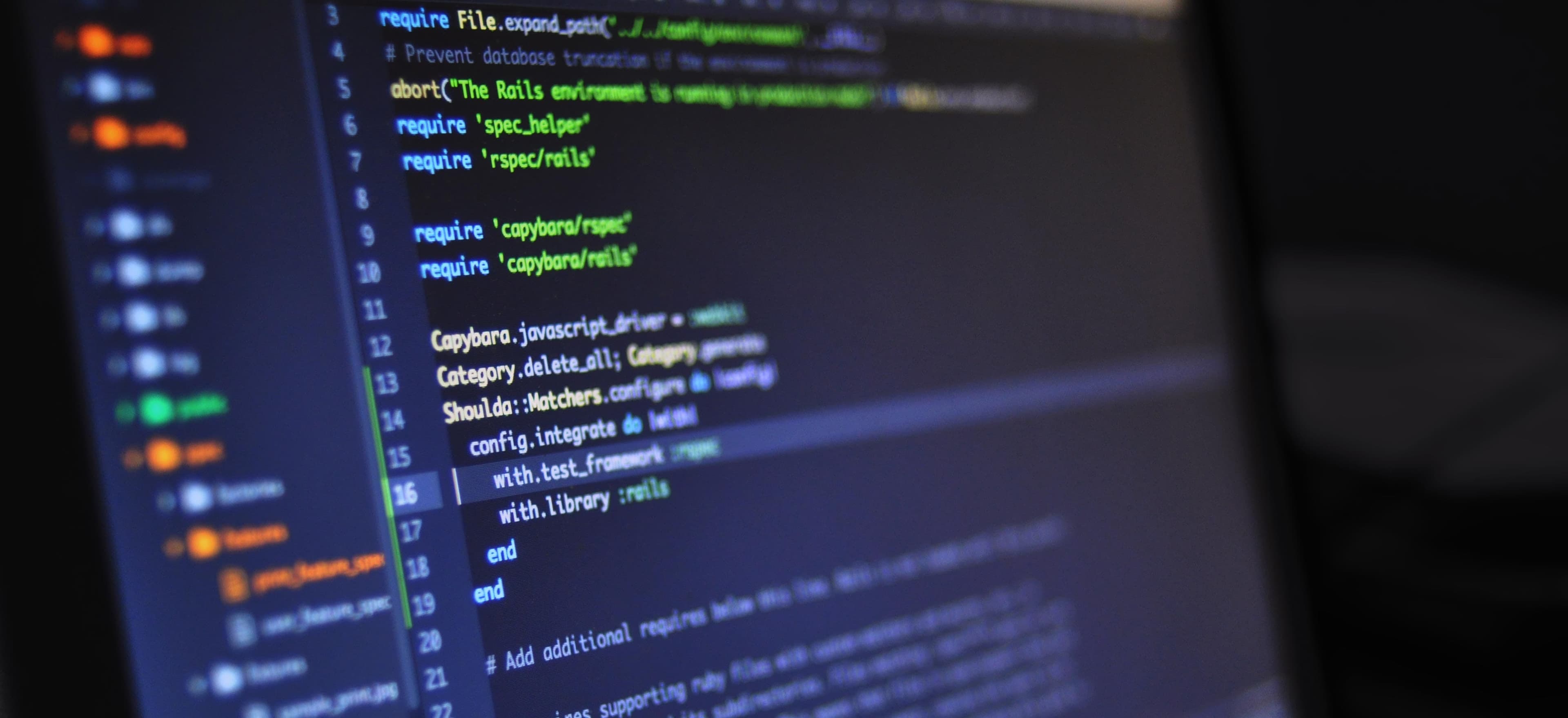
- Published on
Understanding Spring Boot's Common Configuration Pitfalls
Spring Boot has revolutionized the way Java developers build applications by offering a plethora of features that facilitate rapid application development. However, with such a vast array of options comes the risk of misconfiguration. This blog post aims to dissect some of the common configuration pitfalls developers encounter when working with Spring Boot, providing practical solutions and best practices.
Table of Contents
Diving Into the Subject
Spring Boot is designed to make it easy to set up and run applications. Nevertheless, it is essential to follow best practices and be aware of potential pitfalls when configuring your application. Misconfigurations can lead to performance issues, security vulnerabilities, and maintenance headaches.
Common Configuration Pitfalls
1. Profile Mismanagement
Spring profiles allow you to segregate parts of your application configuration and make it available only in certain environments. However, misconfiguring profiles is a common pitfall.
Example:
You might have a dev
profile and a prod
profile. If you forget to activate the right profile, your application might load development configurations in production, exposing your application to vulnerabilities.
Solution: Use the following command to activate your profile explicitly:
java -jar your-application.jar --spring.profiles.active=prod
Also, ensure that your properties files are named according to the profiles:
application-dev.properties
application-prod.properties
By clearly organizing your configuration files, you eliminate the guesswork and reduce the risk of errors.
2. Improper Bean Scoping
Spring manages the lifecycle of beans, and one of the critical aspects is understanding their scope. Using the wrong scope for a bean can lead to issues, especially in web applications.
Example: Defining a bean as a singleton when it should be request-scoped can result in unexpected behavior, particularly when that bean holds user-specific data.
@Bean
@Scope("request")
public UserService userService() {
return new UserService();
}
Why: By declaring the UserService
bean as request-scoped, we ensure that a new instance is created for each request. This behavior is crucial for applications that maintain user sessions or transactions.
3. Misusing @Value and @ConfigurationProperties
Both @Value
and @ConfigurationProperties
are used to externalize configuration. However, using them interchangeably can lead to complications.
Example of @Value:
@Value("${app.default.username}")
private String defaultUsername;
Example of @ConfigurationProperties:
@ConfigurationProperties(prefix="app")
public class AppProperties {
private String defaultUsername;
// getters and setters
}
Why: @Value
is suitable for individual properties, while @ConfigurationProperties
is better for grouping related properties. The latter promotes a cleaner code structure and type-safe configuration.
Solution:
Choose wisely based on your needs. If you're managing related properties, prefer @ConfigurationProperties
.
4. Ignoring Logging Configuration
A common oversight in application configuration is underestimating the importance of logging. Without adequate logging, diagnosing issues becomes unnecessarily complicated.
Example:
By default, Spring Boot sets the logging level to INFO
. In production, you might want to adjust this setting.
To modify your logging configuration, include the following in your application.properties
:
logging.level.root=WARN
logging.level.com.yourpackage=DEBUG
Why: Tailoring logging levels helps in filtering out unnecessary log statements, making it easier to spot issues without sifting through mountains of logs.
For a more controlled logging setup, consider using Logback or Log4j.
5. Failing to Optimize Data Sources
Configuring database connections improperly can lead to performance bottlenecks. A common mistake is hard-coding database properties or failing to utilize connection pools efficiently.
Example:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=password
Solution: Use HikariCP for connection pooling, allowing for performance gains through reusing connections.
spring.datasource.hikari.maximum-pool-size=20
spring.datasource.hikari.minimum-idle=5
spring.datasource.hikari.idle-timeout=30000
Why: Connection pooling reuses existing connections-avoiding the overhead of establishing new ones and significantly improving performance under load.
Best Practices
By understanding these pitfalls, you can adopt the following best practices:
- Centralize Configuration: Maintain all common configurations in a centralized properties file for consistency.
- Documentation: Always document your configuration choices. Code comments can clarify why a particular configuration was chosen.
- Use Profiles Wisely: Ensure that your properties files and active profiles match your deployment stages (development, testing, production).
- Test Your Configuration: Regularly validate your application’s behavior against various environments to identify misconfigurations early.
Closing Remarks
Spring Boot offers much flexibility, but with that flexibility comes the complexity of configuration management. By being aware of common pitfalls and implementing best practices, you can mitigate risks, enhance performance, and ensure a smoother development experience.
For a deeper dive into Spring Boot configuration, consider exploring the official Spring Boot documentation or joining relevant development communities. Avoiding these pitfalls will not only save you time but also improve the maintainability of your applications in the long run.
By taking a proactive approach to Spring Boot configurations, you can enjoy the powerful features it offers with reduced risk and increased efficiency. Happy coding!