Mastering Java 8 Lambdas with the Decorator Pattern
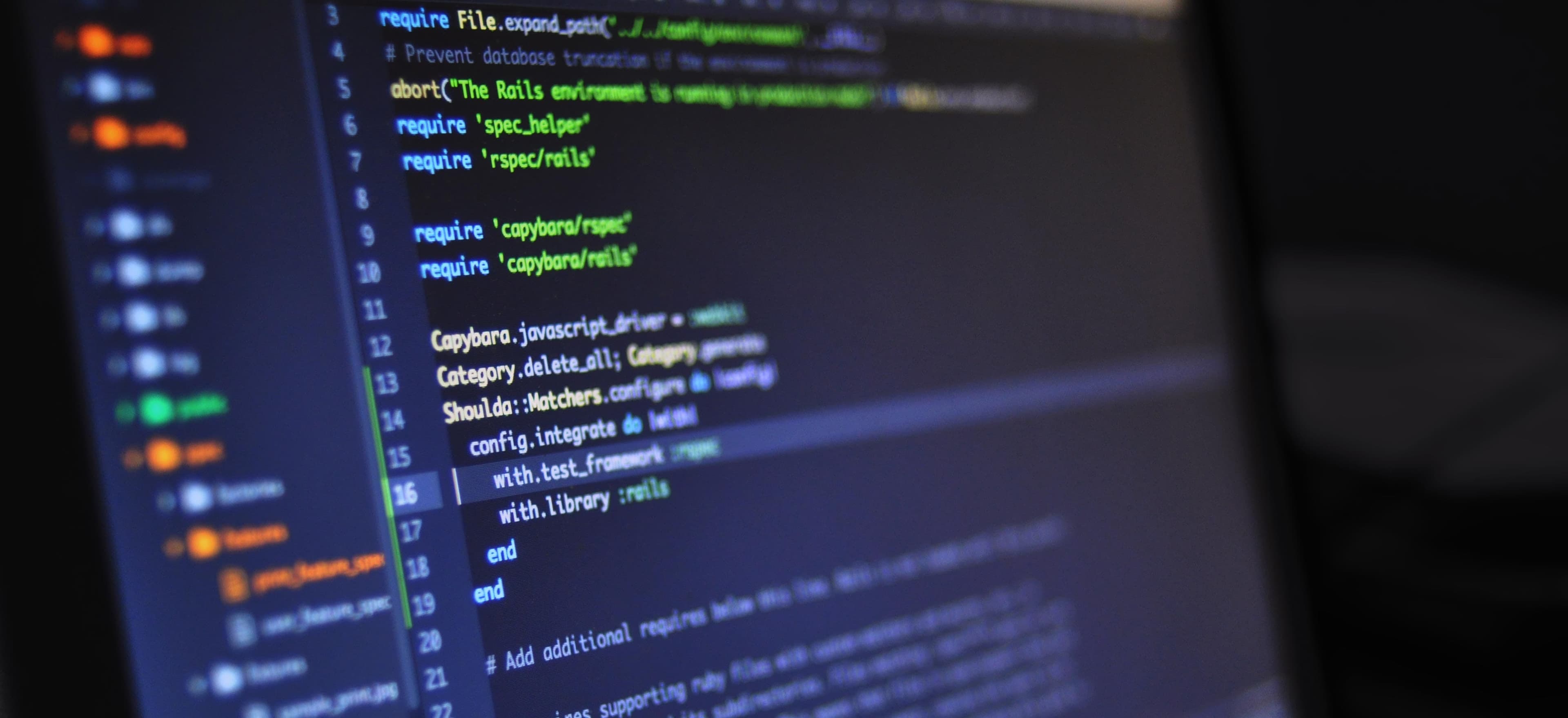
- Published on
Mastering Java 8 Lambdas with the Decorator Pattern
Java 8 introduced significant enhancements to the language, including lambda expressions that simplify code, especially when combined with functional programming paradigms. One intriguing application of lambdas is in design patterns — notably, the Decorator Pattern. This post will guide you through the intricacies of Java 8 lambdas and their synergy with the Decorator Pattern, creating more powerful, maintainable, and dynamic code.
Table of Contents
- Understanding the Decorator Pattern
- Lambda Expressions: A Quick Overview
- Implementing the Decorator Pattern Using Lambdas
- Benefits of Combining Lambdas with the Decorator Pattern
- Real-World Applications and Examples
- Conclusion
Understanding the Decorator Pattern
Definition
The Decorator Pattern is a structural design pattern that allows you to add responsibilities to individual objects dynamically and provides a flexible alternative to subclassing for extending functionality. It involves a set of decorator classes that are used to wrap concrete components.
Example
Imagine you have a simple Coffee
interface:
public interface Coffee {
String getDescription();
double cost();
}
You might have a basic implementation like this:
public class SimpleCoffee implements Coffee {
@Override
public String getDescription() {
return "Simple coffee";
}
@Override
public double cost() {
return 2.00;
}
}
Now, if you want to add functionality, such as milk or sugar, you would create decorators for each that implement the Coffee
interface.
Lambda Expressions: A Quick Overview
What Are Lambdas?
Introduced in Java 8, lambda expressions are a clear and concise way to represent a method interface using an expression. They are particularly useful when you need to pass behavior as a parameter (function as a first-class citizen).
Example of a Lambda Expression:
// Traditional way
Runnable runnable = new Runnable() {
@Override
public void run() {
System.out.println("Running the task");
}
};
// With Lambda Expression
Runnable lambdaRunnable = () -> System.out.println("Running the task");
Implementing the Decorator Pattern Using Lambdas
Now, let's blend lambda expressions with the Decorator Pattern. Instead of creating multiple concrete decorator classes, we can use lambdas to create decorators on-the-fly, making our design more dynamic.
The Coffee Example with Lambdas
We can define our decorators using the Function
interface to represent the wrapping:
import java.util.function.Function;
public class CoffeeShop {
public static void main(String[] args) {
Coffee simpleCoffee = new SimpleCoffee();
// Decorating with milk
Coffee milkCoffee = decorateWithMilk(simpleCoffee);
System.out.println(milkCoffee.getDescription() + " $" + milkCoffee.cost());
// Decorating with sugar
Coffee sugarMilkCoffee = decorateWithSugar(milkCoffee);
System.out.println(sugarMilkCoffee.getDescription() + " $" + sugarMilkCoffee.cost());
}
public static Coffee decorateWithMilk(Coffee coffee) {
return new Coffee() {
@Override
public String getDescription() {
return coffee.getDescription() + ", milk";
}
@Override
public double cost() {
return coffee.cost() + 0.50;
}
};
}
public static Coffee decorateWithSugar(Coffee coffee) {
return new Coffee() {
@Override
public String getDescription() {
return coffee.getDescription() + ", sugar";
}
@Override
public double cost() {
return coffee.cost() + 0.25;
}
};
}
}
Lambda Implementation
Now let's refactor using lambdas:
import java.util.function.Function;
public class CoffeeShop {
public static void main(String[] args) {
Coffee simpleCoffee = new SimpleCoffee();
// Decorating with milk using lambdas
Function<Coffee, Coffee> milkDecorator = coffee ->
new Coffee() {
@Override
public String getDescription() {
return coffee.getDescription() + ", milk";
}
@Override
public double cost() {
return coffee.cost() + 0.50;
}
};
// Applying the decorator
Coffee milkCoffee = milkDecorator.apply(simpleCoffee);
System.out.println(milkCoffee.getDescription() + " $" + milkCoffee.cost());
// Decorate with sugar
Function<Coffee, Coffee> sugarDecorator = coffee ->
new Coffee() {
@Override
public String getDescription() {
return coffee.getDescription() + ", sugar";
}
@Override
public double cost() {
return coffee.cost() + 0.25;
}
};
// Applying both decorators
Coffee sugarMilkCoffee = sugarDecorator.apply(milkCoffee);
System.out.println(sugarMilkCoffee.getDescription() + " $" + sugarMilkCoffee.cost());
}
}
In this code, Function<Coffee, Coffee>
is a functional interface, representing the transformation from one Coffee
to another, enabling a flexible way to add decorators.
Benefits of Combining Lambdas with the Decorator Pattern
- Reduced Boilerplate: Fewer classes to create leads to reduced code clutter.
- Increased Flexibility: You can create decorators dynamically based on runtime conditions, leading to highly adaptable code.
- Higher Readability: Lambdas can lead to cleaner, easier-to-read implementations that are more intuitive.
- Enhanced Maintainability: Reducing the number of classes also lowers the surface area for bugs and makes your code easier to maintain.
Real-World Applications and Examples
The decorator pattern combined with lambdas can be beneficial in various domains:
- UI Components: Widgets can be dynamically enhanced with functionalities like borders, shadows, or colors without altering their underlying structure.
- Streams API: Four core classes in Java 8's Stream API use the decorator pattern. Stream operations like filter, map, and reduce often add new behavior to data sources in a functional style.
- Logging Frameworks: You can add logging dynamically to methods or classes without hardcoding the logging functionality.
As a practical example, consider how you might use this approach in a complex web application managing user preferences or themes — decorators could easily modify underlying UI elements based on user input.
Additional Resource
You can learn more about the Decorator Pattern in this Refactoring Guru article.
A Final Look
Mastering Java 8 lambdas and the Decorator Pattern can significantly enhance your coding practices. With powerful and flexible design, you can create maintenance-friendly applications while keeping your codebase clean. Lambdas streamline the implementation of the Decorator Pattern, allowing you to focus on the behavior of your objects rather than the structure. By blending these modern programming techniques, you can build robust applications that are easy to modify and scale.
Remember, the key is to focus on code clarity while striving for functionality. Happy coding!
Checkout our other articles