Navigating Common Pitfalls in Factory Design Examples
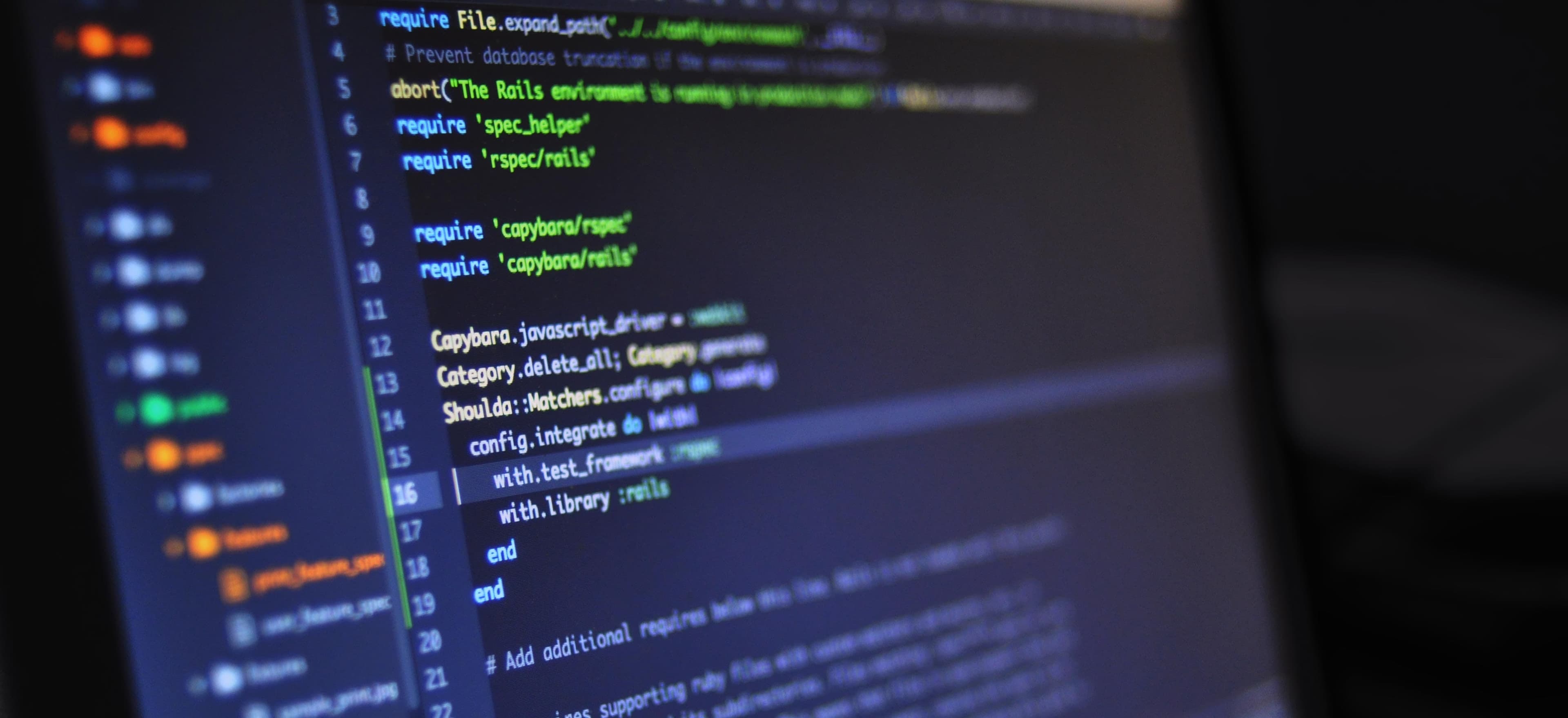
- Published on
Navigating Common Pitfalls in Factory Design Examples
When it comes to modern software development, design patterns play an essential role in creating efficient and maintainable code. Among these patterns, the Factory Design Pattern stands out due to its versatility and ability to create objects without specifying the exact class of object that will be created. However, despite its benefits, implementing the Factory Design Pattern can lead to common pitfalls if not approached carefully. In this blog post, we will explore these pitfalls, backed with Java code snippets, and ways to avoid them.
Understanding the Factory Design Pattern
The Factory Design Pattern falls under the category of creational patterns, which deal with object creation mechanisms. It simplifies the process of object creation by allowing a class to delegate the instantiation of objects to factory classes.
Advantages of Using Factory Pattern
- Loose Coupling: By relying on interfaces or abstract classes, the Factory Pattern decouples client code from specific implementations.
- Enhanced Code Maintainability: Changes in the creation process require less alteration in the client code.
- Increased Flexibility: The pattern allows changes in object creation without changing client logic.
Common Pitfalls in the Factory Design Pattern
1. Overusing the Factory Pattern
While the Factory Design Pattern is powerful, overusing it can add unnecessary complexity to simple applications. For small projects, creating a factory for each object type may lead to bloated code and reduce readability.
Example of Overuse:
// Simple Product interface
interface Product {
void use();
}
// Concrete Product
class ConcreteProductA implements Product {
public void use() {
System.out.println("Using Product A");
}
}
// Factory class
class ProductFactory {
public Product createProduct(String type) {
if (type.equals("A")) {
return new ConcreteProductA();
}
// More product creation logic
return null;
}
}
// Client Code
public class Client {
public static void main(String[] args) {
ProductFactory factory = new ProductFactory();
Product product = factory.createProduct("A");
product.use();
}
}
In this case, if your project doesn’t have many product types, a factory may not be necessary. Evaluate your project complexity before introducing this pattern.
2. Not Using Interfaces or Abstract Classes
Another common pitfall is failing to create appropriate interfaces or abstract classes when designing a factory. This can lead to tightly coupled code that diminishes the benefits of the Factory Pattern.
Improved Design Example:
// Define an abstract Product
interface Product {
void use();
}
// Implementing concrete products
class ConcreteProductA implements Product {
public void use() {
System.out.println("Using Product A");
}
}
class ConcreteProductB implements Product {
public void use() {
System.out.println("Using Product B");
}
}
// Factory with interface usage
class ProductFactory {
public Product createProduct(String type) {
switch (type) {
case "A":
return new ConcreteProductA();
case "B":
return new ConcreteProductB();
default:
throw new IllegalArgumentException("Unknown product type");
}
}
}
Using interfaces allows for better code maintainability and easier testing. Each product class adheres to a common contract, making your system more robust.
3. Ignoring Singleton Scenarios
Sometimes factories may need to be a singleton. Failing to implement a singleton pattern when appropriate can lead to undesired object creation, wasting resources and causing inconsistency in state.
Singleton Factory Example:
class SingletonProductFactory {
private static SingletonProductFactory instance;
private SingletonProductFactory() {}
public static SingletonProductFactory getInstance() {
if (instance == null) {
instance = new SingletonProductFactory();
}
return instance;
}
public Product createProduct(String type) {
if (type.equals("A")) {
return new ConcreteProductA();
}
return null;
}
}
Ensure that whenever you need a single instance of a factory across your application, you employ a Singleton pattern. This ensures consistency and controlled resource allocation.
4. Lack of Clear Object Creation Logic
Transforming complex object creation logic into the Factory can also lead to a confusing design. If your Factory methods become too exhaustive or intricate, they may counteract the benefits of using a factory.
Rather, Implement Simple Factories:
class SimpleProductFactory {
public Product createProduct(String type) {
switch (type) {
case "A":
return new ConcreteProductA();
default:
throw new IllegalArgumentException("Unknown product type");
}
}
}
Keep your factories focused on creating objects. If there's complex logic involved (e.g., parameter validation or context resolution), consider separating that functionality into its own service or utility.
5. Neglecting Dependency Injection
Factory methods can become unwieldy when they need to manage dependencies themselves. Instead of relying on the factory to provide all required dependencies, consider employing dependency injection techniques.
Example:
class ProductService {
private final ProductFactory productFactory;
public ProductService(ProductFactory productFactory) {
this.productFactory = productFactory;
}
public void performAction(String productType) {
Product product = productFactory.createProduct(productType);
product.use();
}
}
By leveraging dependency injection, the object instantiation and dependencies are kept separate, promoting better testability and adherence to design principles like Separation of Concerns.
Wrapping Up
The Factory Design Pattern, like any design pattern, comes with its own set of challenges. By being aware of common pitfalls such as overuse, lack of abstraction, singleton oversight, convoluted creation logic, and neglecting dependency injection, you can navigate through these challenges effectively.
Additional Learning Resources
- Design Patterns: Elements of Reusable Object-Oriented Software - A foundational book on patterns.
- Understanding Java Design Patterns - A deep dive into various design patterns in Java.
By taking the time to evaluate and refine your Factory implementation, you'll enhance your code's readability, maintainability, and overall quality. Always ensure that a design pattern serves your project needs rather than complicating them.