Overcoming ETag Challenges in Spring REST APIs
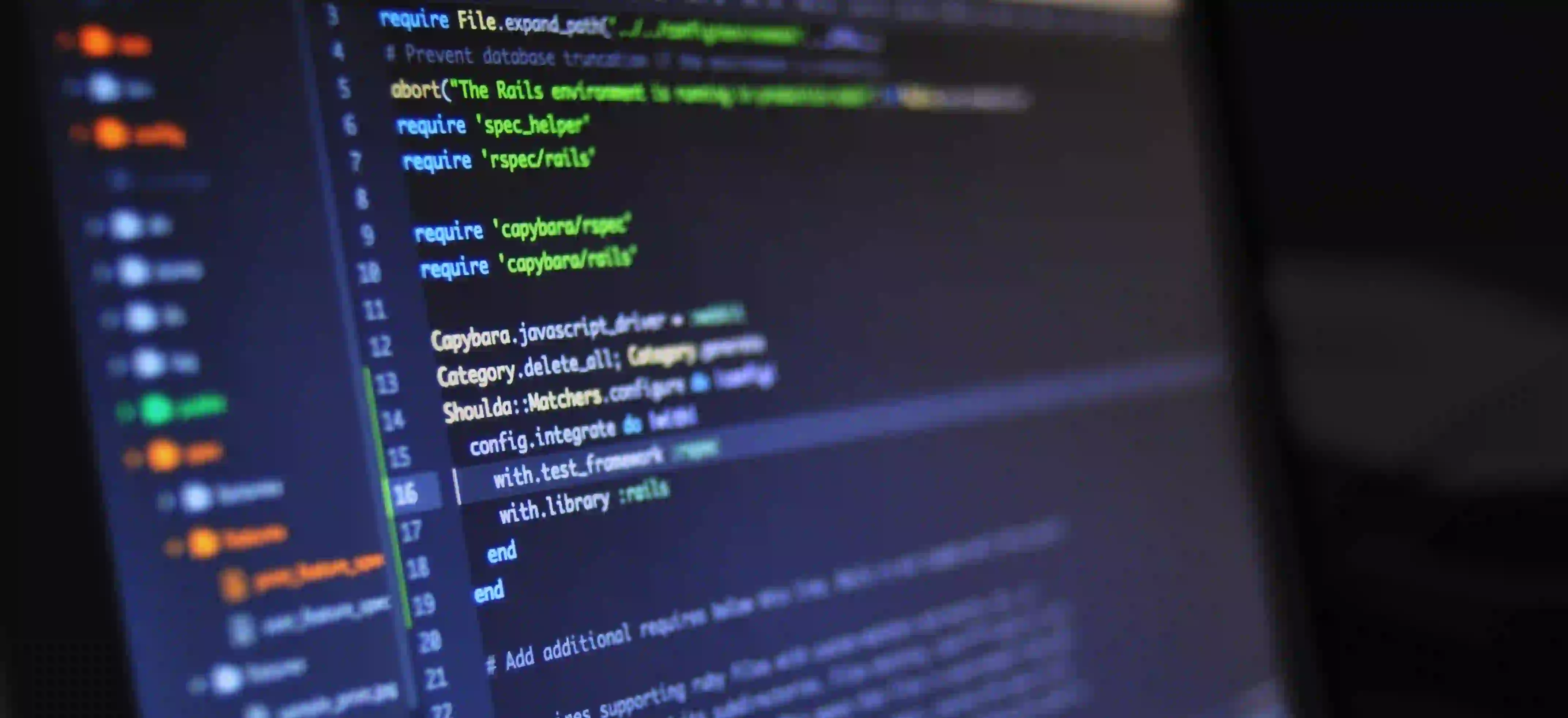
Overcoming ETag Challenges in Spring REST APIs
In today's fast-paced web development environment, efficient data management is key to ensuring a smooth user experience. One of the essential tools in building robust RESTful APIs is ETags (Entity Tags), a mechanism that helps with caching and reducing unnecessary data transfers. However, integrating ETags into a Spring REST API can come with its own set of challenges. In this blog post, we'll explore the common pitfalls associated with ETags and how to overcome them to create a more efficient API.
What are ETags?
ETags are HTTP headers that help identify the resource version. When a client requests a resource, the server responds with the resource and an ETag, representing the current version. Upon subsequent requests, the client can send this ETag in the If-None-Match
header. If the resource hasn't changed, the server can respond with a 304 Not Modified
status instead of sending the entire resource again, saving bandwidth and improving performance.
Basic Example
Here's a simple example of how a Spring REST controller can manage ETag headers:
@RestController
@RequestMapping("/api")
public class MyResourceController {
@GetMapping("/resource/{id}")
public ResponseEntity<MyResource> getResource(@PathVariable String id) {
MyResource resource = resourceService.findById(id);
String eTag = generateETag(resource);
return ResponseEntity.ok()
.eTag(eTag)
.body(resource);
}
private String generateETag(MyResource resource) {
return String.valueOf(resource.getLastModified().getTime());
}
}
Commentary
This code snippet demonstrates how to set an ETag header for a resource. Using the last modified timestamp as the ETag value is a common practice, as it directly links the ETag's validity to the resource state.
Challenges with ETags
While ETags offer several advantages, developers often face challenges when implementing them. Here are a few common hurdles:
1. ETag Generation
The process of generating ETags can vary based on the resource's state. Developers must decide how to create a unique and consistent identifier that accurately reflects changes.
Solution: Use attributes that reliably indicate resource modifications. For instance:
- Hash of the content: You can hash the resource's content to generate a unique identifier.
- Database versioning: If your resource comes from a database, consider using a version number.
Example:
private String generateETag(MyResource resource) {
return Integer.toString(resource.getVersion());
}
2. Stale Content
If a resource is not correctly updated, clients may receive stale data. This issue arises because ETags rely on the resource's state at the time of the request.
Solution: Always update the ETag when modifying or deleting resources. Ensure that your database operations maintain the integrity of the versioning system.
3. Conflict Resolution
When multiple clients modify the same resource simultaneously, this can create a conflict. Clients may not be aware of other changes and may end up overwriting important updates.
Solution: Implement an optimistic locking strategy. Compare the ETag value before an update. If the ETag does not match, return a 409 Conflict
response.
Example:
@PutMapping("/resource/{id}")
public ResponseEntity<MyResource> updateResource(
@PathVariable String id,
@RequestHeader("If-Match") String eTag,
@RequestBody MyResource updatedResource) {
MyResource existingResource = resourceService.findById(id);
if (!generateETag(existingResource).equals(eTag)) {
return ResponseEntity.status(HttpStatus.CONFLICT).build();
}
updatedResource.setId(id);
resourceService.update(updatedResource);
return ResponseEntity.ok(updatedResource);
}
Commentary
In the code above, notice how we're checking the incoming ETag against the current ETag for the resource. If they do not match, we return a 409 Conflict
status, informing the client of the issue.
Implementing ETag in Spring Boot
To fully leverage ETags in a Spring Boot application, it's essential to adopt good practices. Below is an example of how you can consistently integrate ETags across your entire application.
Configuration
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Bean
public MyResourceInterceptor myResourceInterceptor() {
return new MyResourceInterceptor();
}
}
Interceptor for ETags
import org.springframework.stereotype.Component;
import org.springframework.web.servlet.HandlerInterceptor;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@Component
public class MyResourceInterceptor implements HandlerInterceptor {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) {
String eTag = request.getHeader("If-None-Match");
// Logic to verify the ETag goes here
return true;
}
}
Commentary
In this configuration, we create a handler interceptor that can process incoming requests and check their ETags. This way, we have a centralized mechanism for managing ETags, which can help in keeping the logic cleaner and more maintainable.
Testing ETag Functionality
Testing is crucial in ensuring that your implementation works as expected. Use tools like Postman or JUnit for this purpose. Here’s a simple test using JUnit and MockMvc:
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.*;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*;
@Test
public void testETag() throws Exception {
String eTag = "12345";
mockMvc.perform(get("/api/resource/1")
.header("If-None-Match", eTag))
.andExpect(status().isNotModified());
}
Bringing It All Together
ETags are a powerful tool for optimizing API interactions, but they come with their own set of challenges. By understanding how to generate ETags, handle conflicts, and implement proper caching strategies, you can efficiently integrate ETags into your Spring REST applications.
By following these best practices and solutions outlined in this post, you can enhance the user experience of your application significantly. To learn more about handling RESTful APIs in Spring, check out the official Spring Documentation.
Remember, the key to a successful implementation lies in rigorous testing and constant iterations. Happy coding!