Streamlining DTO Creation in Spring Boot with MapStruct
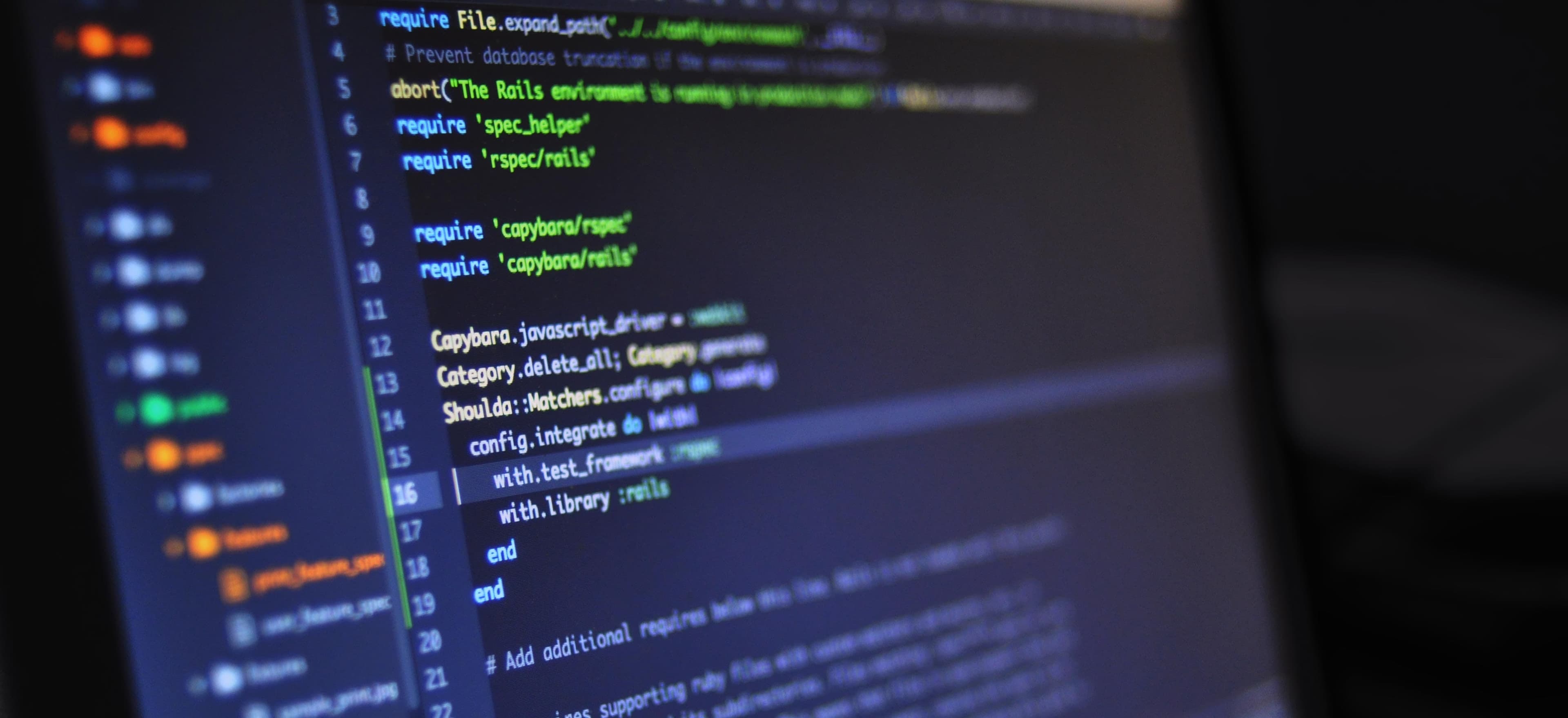
- Published on
Streamlining DTO Creation in Spring Boot with MapStruct
In the world of Java development, particularly with Spring Boot, the need for data transfer objects (DTOs) has become increasingly apparent. DTOs serve as a crucial means of transferring data between different layers of an application. However, building and maintaining these DTOs can become tedious and error-prone. This is where MapStruct shines, providing a code generator for smooth mapping between Java bean types. In this blog post, we will explore how to leverage MapStruct to streamline DTO creation in Spring Boot applications.
Understanding DTOs and Their Importance
Before we dive into the technical aspects, let’s clarify what DTOs are and why they are essential. A DTO is an object that carries data between processes, often in the context of client-server communication. Here are several reasons why DTOs are crucial in applications:
- Separation of Concerns: DTOs separate data representation from business logic, allowing for cleaner APIs and reduced coupling.
- Performance Optimization: By transferring only the necessary data, DTOs can minimize the data payloads sent over the network.
- Validation and Transformation: DTOs can contain validation and transformation logic to ensure that the data being transferred meets the required formats without affecting domain models.
With this foundational understanding, let's turn our attention to how MapStruct can simplify the process of DTO creation.
What is MapStruct?
MapStruct is an annotation processor that generates type-safe and efficient mapping code. It uses simple Java annotations to describe the mapping of properties between Java objects and, in doing so, eliminates a lot of boilerplate code.
Key Features of MapStruct
- Compile-time Type Checking: MapStruct checks for type safety at compile time, minimizing runtime errors.
- Performance: It generates code that is as efficient as writing manual mapping logic.
- Simplicity: The configuration is straightforward and intuitive, requiring minimal setup.
Setting Up MapStruct in a Spring Boot Project
Before we create DTOs and mappers, let's set up MapStruct in a Spring Boot project.
Step 1: Add Dependencies
To include MapStruct, you'll need to add the following dependencies to your pom.xml
if you're using Maven:
<dependency>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct</artifactId>
<version>1.5.4.Final</version>
</dependency>
<dependency>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct-processor</artifactId>
<version>1.5.4.Final</version>
<scope>provided</scope>
</dependency>
For Gradle users, you can add the following lines in your build.gradle
:
implementation 'org.mapstruct:mapstruct:1.5.4.Final'
annotationProcessor 'org.mapstruct:mapstruct-processor:1.5.4.Final'
After adding these dependencies, refresh your project to ensure that MapStruct is integrated correctly.
Step 2: Create a Sample Entity and DTO
Let's create a simple domain model and its corresponding DTO.
Domain Entity
package com.example.demo.entity;
public class User {
private Long id;
private String username;
private String email;
// Getters and Setters
}
DTO
package com.example.demo.dto;
public class UserDTO {
private Long id;
private String username;
// Getters and Setters
}
Step 3: Create the Mapper Interface
We need to create an interface for mapping between User
and UserDTO
. This is where the magic of MapStruct comes into play.
package com.example.demo.mapper;
import com.example.demo.dto.UserDTO;
import com.example.demo.entity.User;
import org.mapstruct.Mapper;
import org.mapstruct.Mapping;
@Mapper(componentModel = "spring")
public interface UserMapper {
@Mapping(source = "email", target = "email") // Not needed in DTO
UserDTO toDTO(User user);
User toEntity(UserDTO userDTO);
}
Explanation of the Mapper Code
- Mapper Annotation: The
@Mapper
annotation indicates that this interface is a MapStruct mapper that should be registered as a Spring bean due to thecomponentModel = "spring"
configuration. - Mapping Methods: We defined two methods –
toDTO
for mapping aUser
entity to aUserDTO
andtoEntity
for the reverse operation. - Field Mapping: In the example, we explicitly configured the mapping of the
email
field (note that it is not included inUserDTO
), highlighting how the mapping can be customized as per requirements.
Step 4: Using the Mapper in a Spring Boot Service
Now that we have our mapper set up, we can utilize it inside a Spring Boot service.
package com.example.demo.service;
import com.example.demo.dto.UserDTO;
import com.example.demo.entity.User;
import com.example.demo.mapper.UserMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.stream.Collectors;
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public UserDTO convertToDTO(User user) {
return userMapper.toDTO(user);
}
public List<UserDTO> convertToDTOs(List<User> users) {
return users.stream()
.map(userMapper::toDTO)
.collect(Collectors.toList());
}
}
Why Use the Mapper in a Service Layer?
Using mappers in the service layer ensures:
- Isolation: The conversion logic is encapsulated in the mapper and is not scattered across the service methods.
- Maintainability: If your mappings change, you only need to edit the mapper, making it easier to maintain.
- Reusability: The mapper can be reused across different services, avoiding code duplication.
Integrating with Spring Data JPA
If you're using Spring Data JPA for data access, you might often find yourself needing to convert between entity and DTO. Here’s how you can integrate this setup more closely with repository operations.
Step 1: Create a User Repository
package com.example.demo.repository;
import com.example.demo.entity.User;
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> { }
Step 2: Fetching and Mapping the Users
Let's enhance our UserService
to fetch users from the repository and convert them to DTOs.
public List<UserDTO> getAllUsers() {
List<User> users = userRepository.findAll();
return convertToDTOs(users);
}
Example of a Complete User Flow
Using MapStruct along with Spring Data JPA, you can create a clean flow for user management. Retrieve data from the database, map entities to DTOs, and send DTOs to the presentation layer, all with minimal boilerplate code.
@RestController
@RequestMapping("/api/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping
public List<UserDTO> getAllUsers() {
return userService.getAllUsers();
}
}
Wrapping Up
Using MapStruct significantly reduces the amount of boilerplate code related to DTO conversion in Spring Boot applications. By leveraging type-safe mappings generated at compile-time, developers can achieve cleaner, more maintainable codebases.
MapStruct plays a pivotal role in streamlining DTO creation, ensuring that your applications not only perform optimally but also maintain the clarity and organization of data transfer.
For more information on MapStruct, check out the official documentation.
Happy coding!
Checkout our other articles