Overcoming Cold Start Issues in AWS Serverless Spring Boot
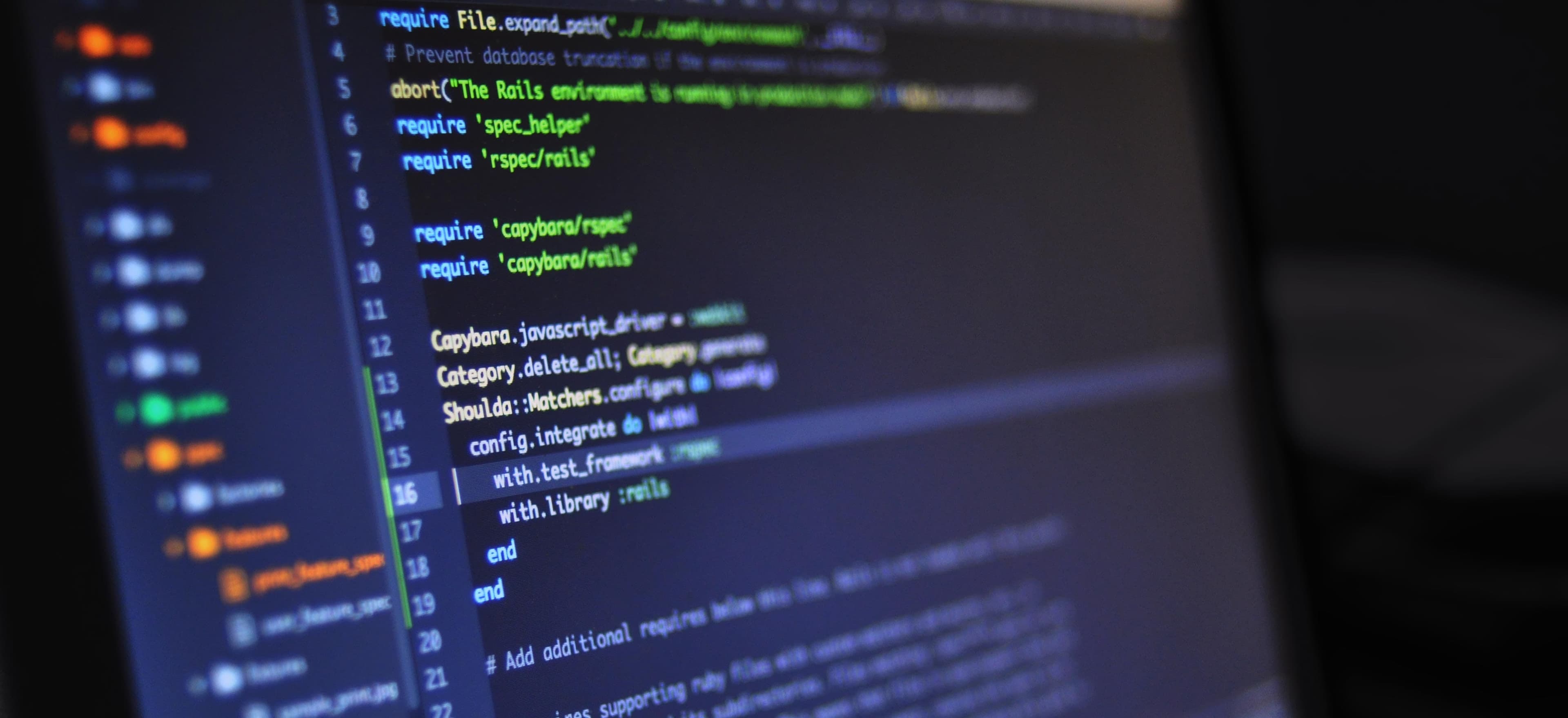
- Published on
Overcoming Cold Start Issues in AWS Serverless Spring Boot
In the world of cloud computing, serverless architecture has emerged as a game-changer, offering scalable solutions while minimizing operational overhead. Among the leading serverless platforms is Amazon Web Services (AWS), which provides various tools and services to facilitate serverless application development. However, one of the critical challenges in serverless architectures, particularly in AWS Lambda with Spring Boot applications, is the phenomenon known as "cold starts."
In this blog post, we will delve deeper into cold start issues in AWS serverless Spring Boot applications. We will explore what cold starts are, why they occur, and how to effectively mitigate their impact. By the end of this post, you’ll have a solid understanding of the techniques you can employ to optimize your serverless Spring Boot applications.
What Are Cold Starts?
In simple terms, a cold start occurs when an AWS Lambda function is invoked for the first time or after it has been inactive for a certain period. During this time, AWS needs to provision the necessary compute resources before your code can run, leading to increased latency. This delay can be particularly detrimental for web applications that expect quick responses, such as those built with Spring Boot.
Here's a brief summary of what happens during a cold start:
- Provisioning: AWS provisions the execution environment for the function.
- Deployment: The function code is loaded into memory.
- Initialization: Any initialization code is executed, including loading frameworks and any required dependencies.
- Execution: Finally, the function processes the event.
Cold starts can lead to latency spikes that frustrate users, so mitigating these effects is crucial for a smooth user experience.
Factors Influencing Cold Starts
Several factors can contribute to the cold start problem:
- Lambda Runtime: Different runtimes have varying initialization times. Java, and by extension Spring Boot, tends to have longer cold start times than languages such as Python or Node.js due to their larger memory footprint and slow startup time.
- Function Size: The size of the application and its dependencies can significantly impact the cold start time.
- Memory Configuration: AWS allows you to adjust the memory allocation of Lambda functions. Higher memory configurations can lead to faster cold starts but will incur higher costs.
- Deployment Package: The way you package your application can also affect startup times. Utilizing tools like AWS SAM or Serverless Framework can streamline packaging and deployment.
Strategies to Mitigate Cold Starts
Let’s explore several effective strategies to overcome cold start issues in AWS Serverless Spring Boot applications.
1. Optimize Your Lambda Memory Configuration
One of the simplest ways to speed up cold starts is by allocating more memory to your Lambda functions. By default, Lambda functions start with 128 MB of memory, but increasing this value can lead to significant improvements in performance.
For example, if you allocate 512 MB or 1024 MB, you might notice that the function starts faster. This is because more memory enables AWS to allocate more CPU power during initialization.
@Autowired
private someService;
void handler(Request request, Context context) {
// Use the service
}
By increasing memory size, you can usually reduce your cold start time, especially for Spring Boot applications that have multiple class loading overhead.
2. Reduce Your Deployment Package Size
A larger deployment package means longer cold start times. It is essential to minimize your package size by excluding unnecessary files and dependencies.
First, analyze your pom.xml
or build.gradle
file to ensure you’re only including essential libraries. Also, consider using a tool like ProGuard or the Maven Shade Plugin to create an Uber jar or a fat JAR that contains only the needed parts.
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-shade-plugin</artifactId>
<version>3.2.4</version>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>shade</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
This Maven configuration minimizes the size of your Spring Boot application, ensuring that load and execution times are significantly reduced.
3. Warm-Up Strategies
Warm-up strategies involve sending dummy requests to your Lambda function periodically, ensuring that it remains "warm." This is not a foolproof solution, but it can minimize cold starts for frequently used functions.
You can set up a scheduled CloudWatch event that triggers your Lambda function at regular intervals. This ensures that the function stays warm and ready to respond quickly.
{
"ScheduleExpression": "rate(5 minutes)",
"Target": {
"Arn": "arn:aws:lambda:us-east-1:123456789012:function:mySpringBootFunction",
"Input": "{}"
}
}
4. Use AWS Lambda Provisioned Concurrency
Provisioned Concurrency is a feature that ensures a set number of Lambda instances are always kept warm and ready to handle requests. With this solution, you can specify a fixed number of instances that are always pre-initialized and available for immediate invocation, significantly reducing response time.
Here's a simple way to enable Provisioned Concurrency:
- In the AWS Console, navigate to your Lambda function.
- Go to the "Configuration" tab.
- Under "Concurrency," enable provisioned concurrency and specify the desired number of instances.
Using Provisioned Concurrency comes at an additional cost, but it can be worth it for high-traffic applications that require low latency.
5. Adopt a Lightweight Framework
While Spring Boot is an excellent framework for building Java applications, it may not be the most lightweight choice for serverless functions. If your application doesn't leverage many of Spring's features, consider employing a lighter framework or a pure Java approach.
6. Use Amazon API Gateway Caching
If you're using AWS API Gateway in front of your Lambda function, enabling caching can also help reduce the perceived latency for users accessing your application. Cached responses are faster than invoking the Lambda function, meaning even if a cold start occurs, users might not notice a delay.
To enable caching:
- Go to your API Gateway settings.
- Under "Resources," select the resource or method you want to cache.
- Enable "Enable Caching."
The Bottom Line
Cold starts can be a significant hinderance in applications built using AWS Lambda and Spring Boot. However, by employing strategies such as optimizing memory configuration, reducing deployment package size, using warm-up strategies, enabling AWS Provisioned Concurrency, adopting lighter frameworks, and using API Gateway caching, you can effectively reduce cold start latency.
Understanding the underlying dynamics of cold starts and implementing these optimized strategies will not only enhance performance but also positively impact user experience.
For further reading on serverless architectures and AWS Lambda best practices, feel free to explore the AWS Lambda Developer Guide or check out Spring Boot in the Serverless World. Cloud technologies are constantly evolving, so keep exploring and learning!
By proactively addressing cold start issues, you can deliver a more seamless and efficient experience for your users. Happy coding!
Checkout our other articles