Understanding the Pitfalls of Miscalculating Allocation Rates
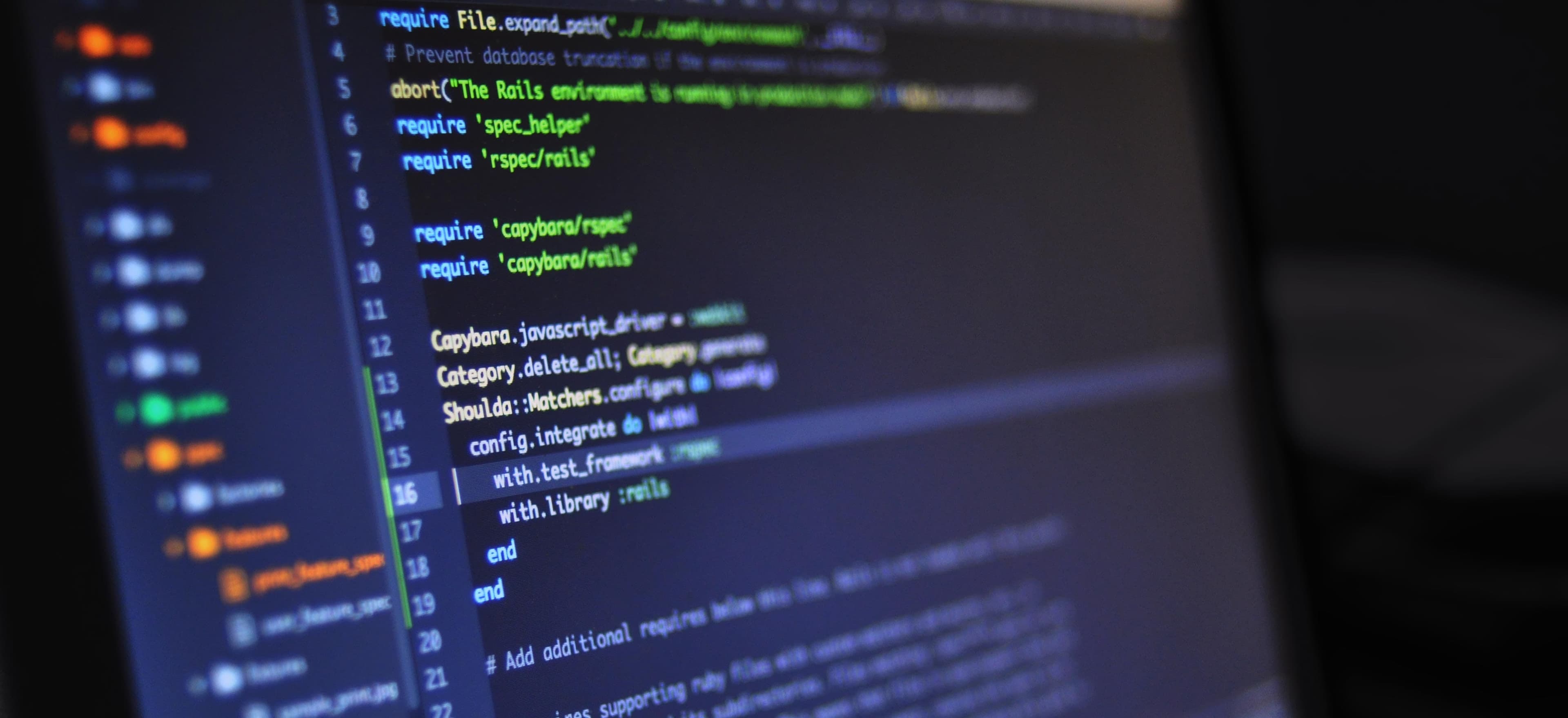
- Published on
Understanding the Pitfalls of Miscalculating Allocation Rates in Java
When developing applications in Java, especially those involving financial calculations or resource allocation, precision is crucial. Miscalculating allocation rates can lead not only to financial discrepancies but also significantly hamper system performance. In this blog post, we will delve into the common pitfalls of miscalculating allocation rates, explore why these mistakes happen, demonstrate the correct approach using Java, and provide best practices to avoid falling into these traps.
What Are Allocation Rates?
Allocation rates refer to the way resources—whether they be memory, CPU usage, or even budget constraints—are distributed among various segments of a system or application.
For example, in a financial context, allocation rates can determine how much budget is set aside for various departments within a company, based on predicted performance metrics or historical data.
Why It Matters
Miscalculating allocation rates can lead to:
- Resource Waste: Over-allocating resources leads to inefficiencies.
- Underfunding: Critical areas might not receive the necessary resources, which could jeopardize projects.
- Performance Issues: Incorrect allocation may slow down processes, resulting in poor user experiences.
Common Pitfalls to Avoid
1. Ignoring Data Quality
It's essential to ensure that the data used for calculations is accurate and up-to-date. Often, developers might overlook the quality of input data, leading to erroneous allocation rates.
Example in Java:
Imagine you have a method that calculates an allocation rate based on past sales data. If that data is outdated or incorrect, the outcome will be flawed.
public double calculateAllocationRate(double totalBudget, double expense) {
if (totalBudget <= 0) {
throw new IllegalArgumentException("Total budget must be greater than zero.");
}
return expense / totalBudget;
}
// Usage
double totalBudget = 10000;
double expense = 2500;
double allocationRate = calculateAllocationRate(totalBudget, expense);
System.out.println("Allocation Rate: " + allocationRate);
In this example, if totalBudget
was inaccurate, the calculated allocationRate
would misrepresent the true financial health and resource allocation efficiency.
2. Simplistic Calculations
Sometimes, developers use simplistically designed formulas which do not take into account the complexities involved in resource allocation.
Example of a Complex Calculation
Let's say you need to allocate a marketing budget based not only on past expenses but also forecasted revenue.
public double complexAllocationRate(double totalBudget, double pastExpenses, double forecastRevenue) {
if (totalBudget <= 0) {
throw new IllegalArgumentException("Total budget must be greater than zero.");
}
return (pastExpenses + 0.1 * forecastRevenue) / totalBudget; // factoring in future forecasts
}
// Usage
double totalBudget = 15000;
double pastExpenses = 3000;
double forecastedRevenue = 60000;
double complexAllocationRate = complexAllocationRate(totalBudget, pastExpenses, forecastedRevenue);
System.out.println("Complex Allocation Rate: " + complexAllocationRate);
In this snippet, we adjust the allocation based on forecasted data, providing a more balanced approach to resource distribution.
3. Lack of Granularity
Another common downfall is using overly broad allocation categories. For instance, merging multiple departments under a single budget can obscure the necessity for departmental needs.
Demonstration
public class Department {
String name;
double budgetAllocation;
public Department(String name, double budgetAllocation) {
this.name = name;
this.budgetAllocation = budgetAllocation;
}
}
public double getTotalAllocation(Department[] departments) {
double total = 0;
for (Department dept : departments) {
total += dept.budgetAllocation;
}
return total;
}
// Usage
Department[] departments = {
new Department("HR", 5000),
new Department("Marketing", 7000),
new Department("IT", 3000)
};
double totalDepartmentAllocation = getTotalAllocation(departments);
System.out.println("Total Allocation across departments: " + totalDepartmentAllocation);
By segmenting allocations by department, you can gain better insight into each department's needs and efficacy.
Best Practices for Accurate Allocation Calculations
1. Utilize Data Validation Techniques
Always validate incoming data. Implement checks to ensure data integrity before performing calculations.
2. Incorporate Historical Trends
Use historical data to benchmark allocation rates. By analyzing past trends, you can anticipate resource needs more accurately.
3. Implement Logging and Monitoring
When running calculations, always log results. If something appears off, monitoring systems can alert you to discrepancies promptly.
public void logAllocationRate(double allocationRate) {
System.out.println("Logging Allocation Rate: " + allocationRate + " at " + new java.util.Date());
}
Logging provides an essential feedback loop; it allows you to trace back any miscalculations.
4. Conduct Regular Reviews
Mechanisms should be in place for regular audits of allocation rates and mechanisms to reassess when necessary.
A Final Look
Miscalculating allocation rates can lead to dire consequences, from inefficient resource management to financial misalignments. By being vigilant and employing best practices, developers can mitigate risks. Understanding and utilizing Java's powerful functionalities allows for clearer and more accurate resource distribution.
To further explore allocation strategies and advanced financial calculations, you may find these resources helpful:
- Java Performance Tuning
- Effective Java
Let this guide serve as a reminder of the significance of accurate allocation rate calculations and the complexities involved in achieving them. Happy coding!
Checkout our other articles