Java Line Separators: Avoiding OS Dependency Issues
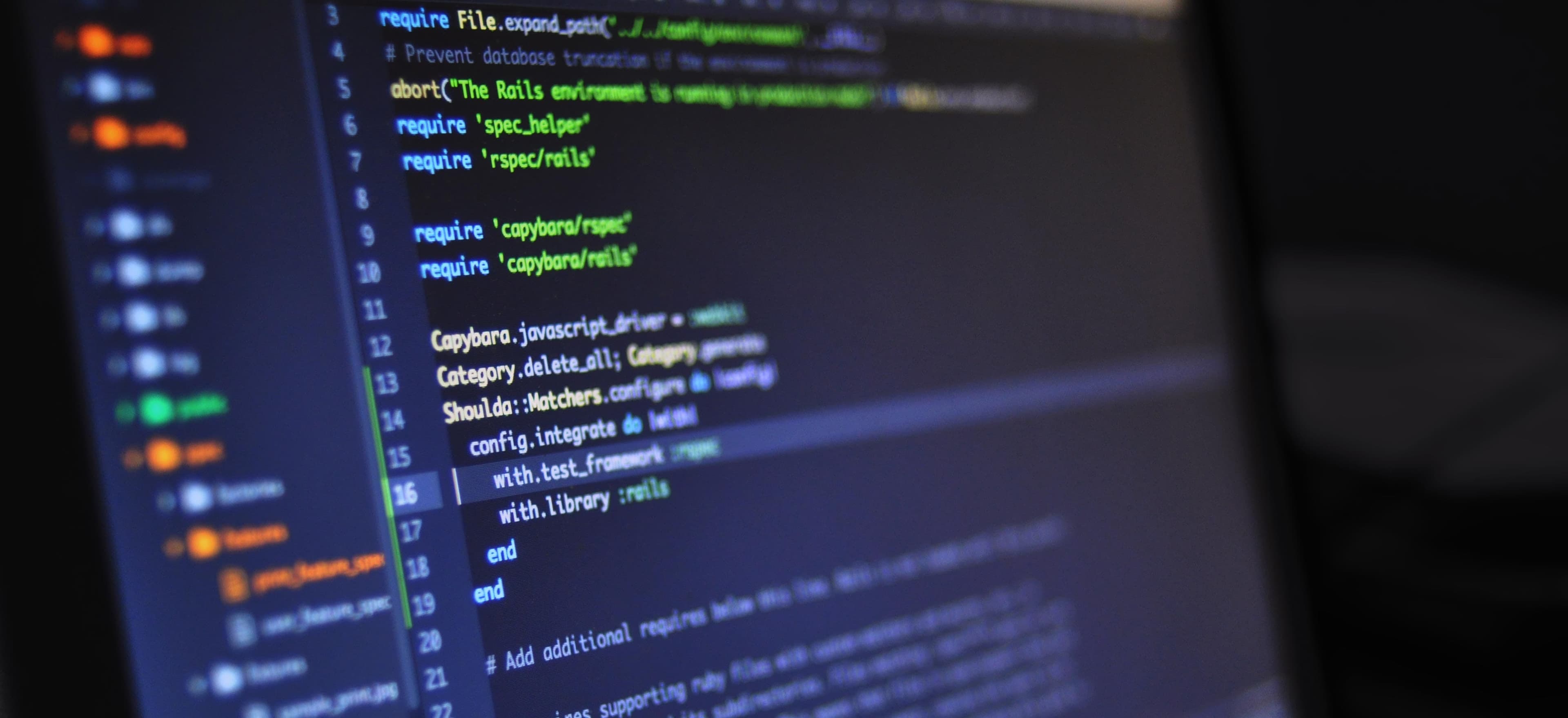
- Published on
Java Line Separators: Avoiding OS Dependency Issues
When developing applications in Java, an essential task is ensuring that your code runs smoothly across different operating systems. One common pitfall is dealing with line separators. Different platforms use different characters for line breaks. Windows uses carriage return + line feed (CRLF, or '\r\n'), while Unix/Linux and macOS use just line feed (LF, or '\n'). In this blog post, we will explore how to effectively handle line separators in Java to avoid common pitfalls and OS dependency issues.
Understanding Line Separators in Java
Java provides a constant for representing the system's line separator, which is accessible through the System
class. This constant is System.lineSeparator()
. By using this, you can write code that is agnostic to the underlying OS.
Example of Using System.lineSeparator()
Here is a simple example to demonstrate how the line separator can be used in Java.
public class LineSeparatorExample {
public static void main(String[] args) {
String line1 = "Hello, World!";
String line2 = "Welcome to Java programming.";
String message = line1 + System.lineSeparator() + line2;
System.out.println(message);
}
}
Why Use System.lineSeparator()
?
- Portability: The code can run on any operating system without modification.
- Clarity: Using this constant makes your code more readable, indicating that it is handling line breaks.
Writing Files with Proper Line Separators
When writing to files, ensuring the correct line breaks are used is crucial for portability. The following code snippet demonstrates how to write text to a file while using System.lineSeparator()
:
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
public class FileWriteExample {
public static void main(String[] args) {
String filePath = "output.txt";
try (BufferedWriter writer = new BufferedWriter(new FileWriter(filePath))) {
writer.write("This is the first line." + System.lineSeparator());
writer.write("This is the second line." + System.lineSeparator());
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why Is This Important?
- Avoids Misinterpretation: Files created on one OS can behave unpredictably when opened on another OS if the line breaks are not handled correctly.
- Better Functionality: Applications that parse these files will behave as expected across different systems.
Reading Files and Handling Line Separators
When it comes to reading files, be mindful of line separators, especially if files originate from different OS environments. You can read files line by line, accommodating any line ending. Utilizing Java's BufferedReader
can simplify this, for example:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReadExample {
public static void main(String[] args) {
String filePath = "output.txt";
try (BufferedReader reader = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why Read Line By Line?
- Efficiency: Helps prevent memory issues when dealing with large files.
- Compatibility:
readLine()
automatically handles line separators, making it easier to work with consistently.
Handling Input from Different Sources
When your application encounters input from various sources (like external APIs or user-generated content), the line separators may differ. It's prudent to normalize inputs.
Example of Normalizing Line Separators
Here is how you can normalize line breaks to the platform’s default using Java:
public class NormalizeLineSeparators {
public static void main(String[] args) {
String input = "First line.\r\nSecond line.\nThird line.";
// Normalize line breaks
String normalized = input.replaceAll("\r\n|\n|\r", System.lineSeparator());
System.out.println(normalized);
}
}
Why Normalize?
- Consistency: Ensures that your application handles line breaks uniformly.
- Data Integrity: Prevents issues when processing or storing user input.
Testing Line Separator Handling
Lastly, always test your application on various operating systems to ensure that your handling of line separators is robust. Consider using tools like JUnit for unit testing line separation functionality.
Example Test Case
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class LineSeparatorTest {
@Test
public void testLineSeparator() {
String platformSeparator = System.lineSeparator();
String testString = "Line 1" + platformSeparator + "Line 2";
assertEquals("Line 1" + platformSeparator + "Line 2", testString);
}
}
Why Write Tests?
- Quality Assurance: Validates your code under different scenarios.
- Documentation: Offers a way to demonstrate expected behavior through examples.
A Final Look
Handling line separators in Java may seem trivial, but it is an important aspect of creating cross-platform applications. By utilizing System.lineSeparator()
, normalizing inputs, and implementing efficient reading and writing strategies, you can avoid common pitfalls associated with OS dependency issues. Make sure to test your applications on multiple platforms to identify and fix any potential line separator issues.
For further reading on Java best practices, you might want to check Oracle's official Java documentation and understand more about file handling in Java.
Once you embrace these techniques, you will be well on your way to enhancing the professionalism and usability of your Java applications. Happy coding!
Checkout our other articles