Avoiding Common Pitfalls of Random Number Generators
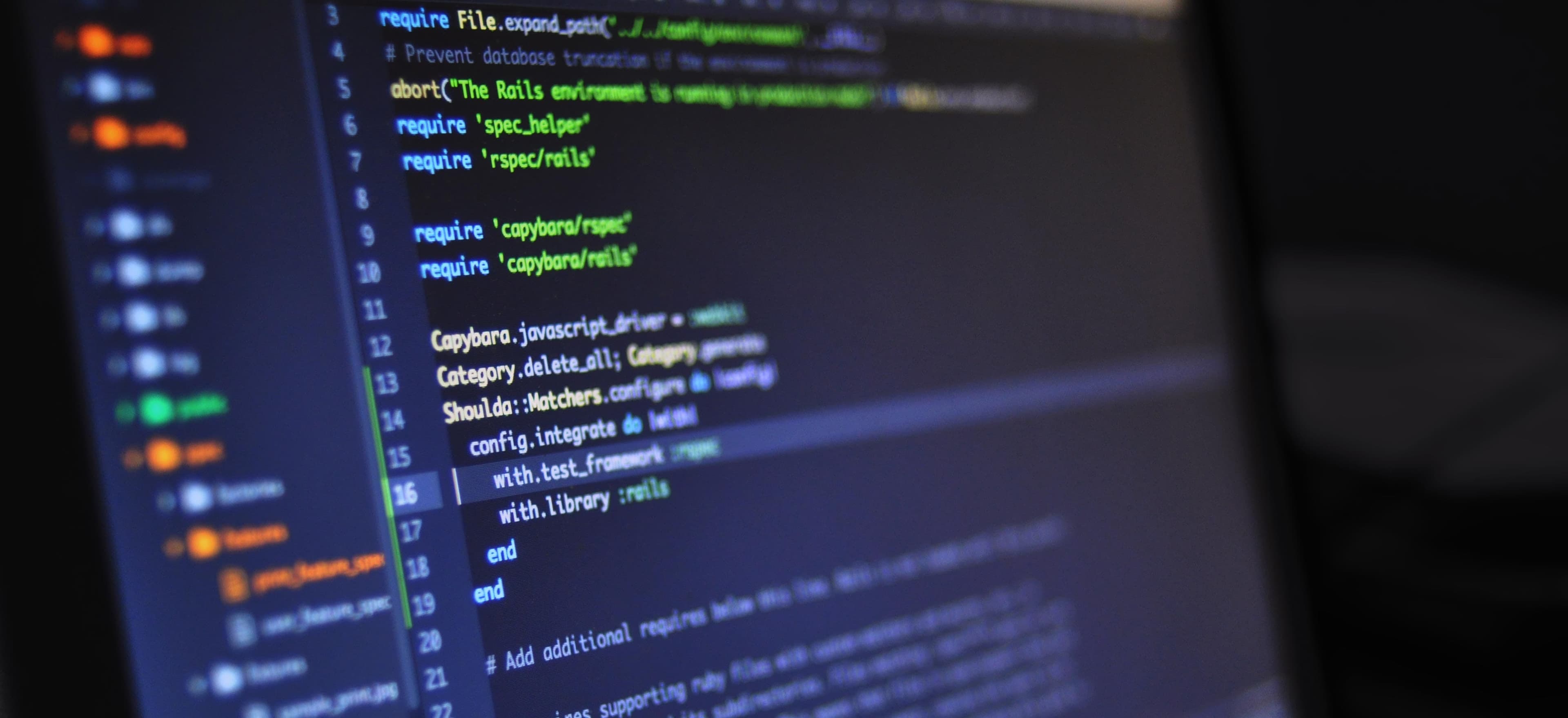
- Published on
Avoiding Common Pitfalls of Random Number Generators in Java
Random number generation is an essential component in many areas of software development, from games to cryptographic applications. However, when misused, it can lead to predictable outcomes and potential vulnerabilities. This blog post will provide insights into the common pitfalls of random number generators (RNGs) in Java and how to avoid them.
Understanding Random Number Generation
Before diving into the pitfalls, it's important to understand what random number generation is and why it's crucial. Random numbers are used in various fields, including gaming, simulations, statistical sampling, and cryptography.
In Java, the principal class for generating random numbers is java.util.Random
. However, Java 8 introduced another option called java.security.SecureRandom
, which offers a higher degree of randomness and security.
Key Concept: Pseudorandom vs. True Random
One of the fundamental distinctions to make is between pseudorandom and true random number generators:
-
Pseudorandom number generators (PRNGs) like
Random
produce a sequence of numbers that approximate the properties of random numbers. However, given the same seed value, they will produce the same sequence repeatedly. -
True random number generators (TRNGs), on the other hand, rely on unpredictable physical phenomena, making them more suitable for applications requiring high security.
Common Pitfalls of Random Number Generators
1. Using Poor Seed Values
Using a poor or predictable seed is one of the most common mistakes when working with RNGs. If you use a fixed seed, you will generate the same sequence of numbers upon every run.
Example of Poor Seeding
Random random = new Random(12345); // Fixed seed
int randomNumber = random.nextInt(100); // This will always generate the same number
Instead of using a fixed seed, consider utilizing the current time or other unpredictable sources.
Improved Seeding
Random random = new Random(System.currentTimeMillis());
int randomNumber = random.nextInt(100); // Generates different numbers each time
2. Not Understanding the Distribution
Random number generators can produce numbers uniformly or non-uniformly. Misunderstanding the output distribution can lead to biased results, especially in statistical applications.
Example of Uniform Distribution
Random random = new Random();
for (int i = 0; i < 10; i++) {
System.out.println(random.nextInt(10)); // Generates uniformly distributed numbers between 0 and 9
}
Always ensure that the method you are using fits your required distribution. If you need a normal distribution, consider using libraries like Apache Commons Math or similar.
3. Ignoring Thread Safety
When dealing with multithreaded applications, shared instances of Random
can lead to unpredictable results due to race conditions.
Example of Non-thread-safe Usage
class RandomGenerator implements Runnable {
private Random random;
public RandomGenerator(Random random) {
this.random = random;
}
@Override
public void run() {
System.out.println(random.nextInt(100));
}
}
Random sharedRandom = new Random();
for (int i = 0; i < 10; i++) {
new Thread(new RandomGenerator(sharedRandom)).start();
}
Best Practice: Using ThreadLocal
To avoid thread-safety issues, you can use the ThreadLocal
class to ensure each thread gets its own instance of Random
.
class RandomGenerator implements Runnable {
private static ThreadLocal<Random> threadLocalRandom = ThreadLocal.withInitial(Random::new);
@Override
public void run() {
System.out.println(threadLocalRandom.get().nextInt(100));
}
}
for (int i = 0; i < 10; i++) {
new Thread(new RandomGenerator()).start();
}
4. Using Math.random()
While it may seem convenient, Math.random()
is less flexible than java.util.Random
and cannot be seeded. This can produce predictable results when used incorrectly.
Basic Use of Math.random()
double randomValue = Math.random(); // This generates a double between 0.0 and 1.0
For more control, use java.util.Random
or java.security.SecureRandom
.
5. Not Considering Cryptography
For applications involving cryptography, using java.util.Random
is not recommended due to the predictable nature of its algorithm. Instead, you should use java.security.SecureRandom
, which provides a stronger random generation mechanism.
Using SecureRandom
import java.security.SecureRandom;
SecureRandom secureRandom = new SecureRandom();
int secureRandomNumber = secureRandom.nextInt(100); // Cryptographically strong random number
The SecureRandom
class ensures a higher level of randomness, making it essential for sensitive applications like generating keys, tokens, or passwords.
To Wrap Things Up
Properly utilizing random number generators in Java requires understanding common pitfalls and being aware of the appropriate practices to follow. To recap:
- Seed Wisely: Avoid fixed seeds and utilize dynamic sources for unpredictability.
- Understand the Distribution: Ensure the RNG fits the problem's needs, especially regarding output distribution.
- Prioritize Thread Safety: Use
ThreadLocal
to prevent race conditions in multithreaded environments. - Choose the Right Method: Prefer
SecureRandom
overMath.random()
orjava.util.Random
for cryptographic needs.
By paying attention to these aspects, you can enhance the reliability and effectiveness of your random number generation in Java applications. For further reading, explore the official Java documentation on Random and the SecureRandom documentation.
Final Thoughts
With a thorough understanding of RNG and the common mistakes developers make, you can effectively implement randomness in your applications. As always, conduct detailed testing to guarantee the integrity and unpredictability of your generated numbers. Happy coding!
Checkout our other articles