Debugging Dilemmas: Navigating Tool Conflicts in Code Testing
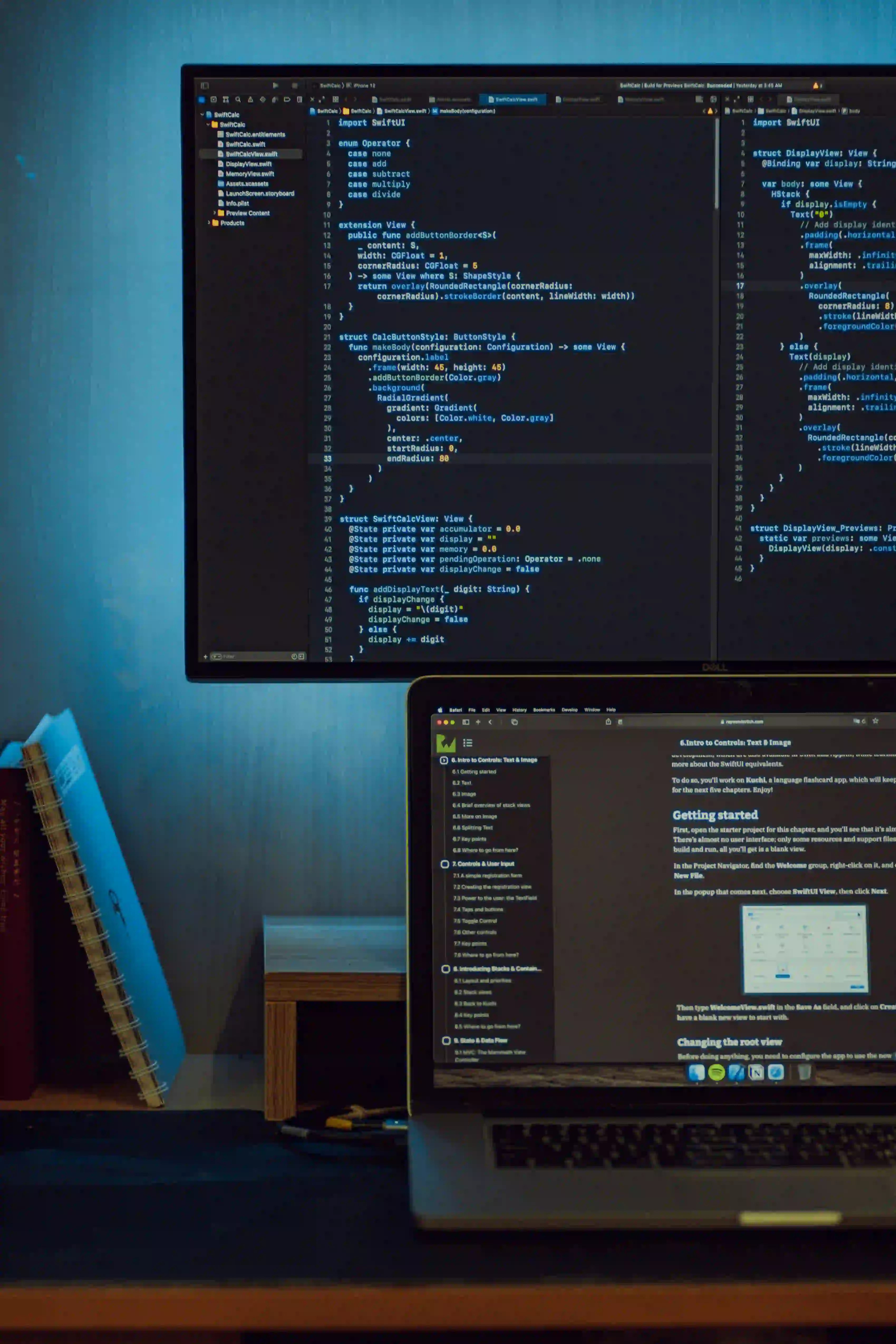
Debugging Dilemmas: Navigating Tool Conflicts in Code Testing
Debugging is an inherent aspect of software development. Mastering it requires not only skill but also a solid understanding of various tools and frameworks. In this blog post, we will explore common tool conflicts during code testing, how to identify them, and effective strategies to resolve them. Whether you're a seasoned developer or a newcomer, these insights will enhance your debugging prowess.
Understanding Tool Conflicts
A tool conflict arises when two or more development tools interfere with each other, leading to unexpected behavior in the code. Such conflicts can stem from various reasons, including:
- Version Mismatches: Different components of a development environment might rely on divergent versions of dependencies or frameworks.
- Configuration Overlaps: Multiple tools may try to configure the same environment settings, leading to clashes.
- Library Dependencies: Conflicts can occur when libraries have incompatible updates or dependencies.
When dealing with these conflicts, the primary goal is to ensure a smooth testing and debugging environment.
Common Debugging Tools and Their Conflicts
1. Integrated Development Environments (IDEs)
IDEs, such as IntelliJ IDEA and Eclipse, are great for writing and debugging Java code. However, they may conflict with specific plugins or libraries.
Example: When using Gradle with IntelliJ IDEA, you might face issues if the Gradle version specified in build.gradle
is incompatible with the IntelliJ version.
Check for compatibility in the Gradle Documentation.
// build.gradle
plugins {
id 'java'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework:spring-core:5.3.10' // Ensure this version is compatible with your IDE
}
2. Build Tools
Gradle and Maven are popular build tools in Java projects. Conflicts can arise when both tools are configured in the same project, leading to inconsistent builds.
If you attempt to use both, ensure that the dependencies in each build file do not overlap unnecessarily. This can lead to version clashes that complicate debugging.
To prevent these issues, select one build tool and stick with it throughout the development cycle.
3. Testing Frameworks
JUnit and TestNG are widely used for unit testing in Java. Depending on the project's setup, using both testing frameworks simultaneously can lead to confusion and redundancy.
Consider a setup using JUnit:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class SimpleTest {
@Test
void addition() {
assertEquals(2, 1 + 1); // Testing basic addition
}
}
Integrating TestNG in a mixed project can create conflicts in annotations and test execution order. Therefore, explicitly decide on utilizing either JUnit or TestNG for clarity.
Diagnosing Tool Conflicts
Identifying tool conflicts involves systematic analysis. Follow these steps:
- Check Versions: Use command-line tools like
gradle -v
ormvn -v
to check for version discrepancies. - Review Logs: Look at error logs. They often indicate which tools are conflicting.
- Isolate Tools: Temporarily disable certain plugins or tools and check if the issues persist.
- Simplify Builds: Reduce your build configuration to its essentials. This will help you pinpoint where conflicts arise.
Strategies for Resolving Conflicts
1. Create a Clear and Consistent Environment
Utilize Docker or Vagrant to maintain consistent development environments across all team members. This minimizes the chances of conflicts arising from local setups. For example, a simple Dockerfile for a Java application might look like this:
# Dockerfile
FROM openjdk:11-jre-slim
VOLUME /tmp
COPY target/myapp.jar app.jar
ENTRYPOINT ["java", "-jar", "/app.jar"]
Using Docker ensures each team member works in the same environment, eliminating local setup discrepancies.
2. Use Dependency Management Tools
Both Maven and Gradle provide powerful mechanisms for managing dependencies. Using a dependency management tool allows you to specify exact versions and avoid potential conflicts.
In Gradle, you can enforce a specific library version across all modules with the following snippet:
configurations.all {
resolutionStrategy {
force 'org.springframework:spring-core:5.3.10' // Force specific version across all dependencies
}
}
3. Run Tests Regularly
Incorporate continuous integration (CI) tools like Jenkins, Travis CI, or GitHub Actions to run tests on every commit. CI can quickly surface conflicts between tools or dependencies that may not appear during local development.
Here's an example GitHub Actions configuration for a Java project:
name: Java CI
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK 11
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Build with Gradle
run: ./gradlew build
Regular testing catches conflicts earlier, streamlining the debugging process.
4. Engage in Community Support
When you encounter conflicts that you cannot resolve independently, engage with developer communities. Forums like Stack Overflow and the Java subreddit are invaluable resources where you can ask for help and share your experiences.
Final Thoughts
Navigating tool conflicts during code testing can be a daunting task. However, with the right strategies and an understanding of the underlying issues, you can mitigate the challenges. Focus on creating a consistent environment, employing effective dependency management, and making use of community resources. These practices will lead to a more productive debugging experience.
By following the guidelines presented in this blog, you’ll be well equipped to tackle any tool conflicts that arise in your Java projects. Remember, effective debugging is not just about fixing errors; it's about preventing them from occurring in the first place. Happy coding!