Fighting Interface Over-Segregation: A Developer's Dilemma
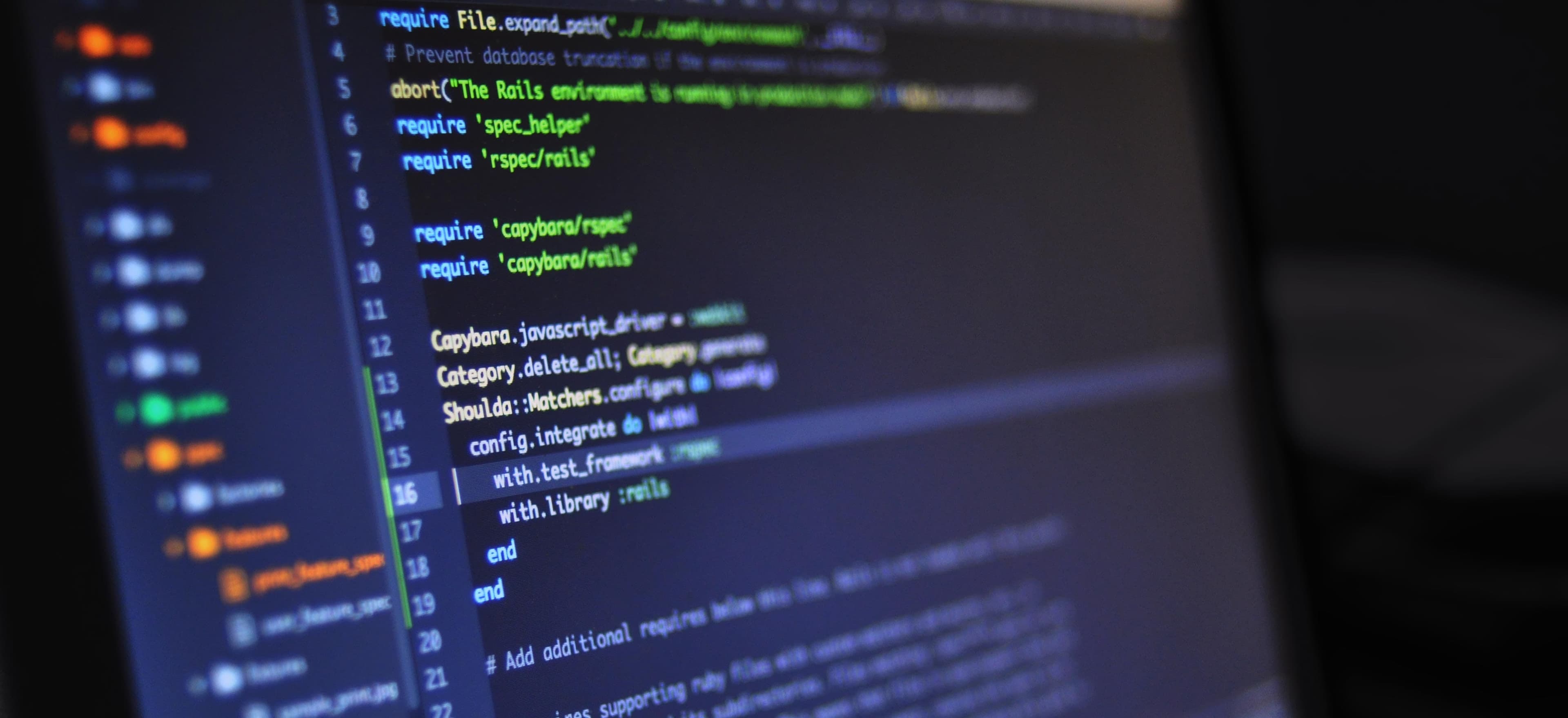
- Published on
Fighting Interface Over-Segregation: A Developer's Dilemma
In software design, interfaces are vital for creating flexible and scalable applications. However, as developers strive for perfection, they can fall into the trap of interface over-segregation—a complex issue that involves too many tightly focused interfaces. This blog post will explore the downsides of interface over-segregation, its implications on development, and how we can strike a balance for a clean and maintainable architecture.
What is Interface Segregation?
The Interface Segregation Principle (ISP) is one of the five SOLID principles of object-oriented programming. It states that a client should not be forced to implement interfaces it does not use. In simpler terms, it encourages the creation of smaller, more specific interfaces rather than large, all-encompassing ones.
Example of Interface Segregation
Consider a scenario where we have an interface Animal
:
public interface Animal {
void eat();
void fly();
void swim();
}
In this case, some animals can eat but don’t fly or swim, like dogs. This forces implementations to provide unnecessary methods, violating ISP. A better design would be:
public interface Eater {
void eat();
}
public interface Swimmer {
void swim();
}
public interface Flyer {
void fly();
}
By segregating the interfaces, Dog
only needs to implement Eater
, while Bird
can implement both Flyer
and Eater
. This approach enhances code clarity and adheres to the ISP.
The Costs of Over-Segregation
While adhering to ISP is essential, over-segregation can lead to its own set of problems. Below are some common pitfalls developers encounter when interfaces are excessively segregated.
1. Increased Complexity
When an application has too many tiny interfaces, it can lead to increased complexity. Developers may find it challenging to keep track of all the interfaces and their relationships. This complexity may complicate implementations, causing confusion rather than clarity.
2. Fragile Systems
Systems with many interfaces can become fragile. If one interface changes, it might ripple across multiple implementations, leading to a cascade of required changes. This fragility can slow down development and increase the likelihood of introducing bugs.
3. Inconsistent Implementations
Too many specific interfaces can lead to inconsistent implementations across the application. Different developers may interpret the design principles differently, resulting in varied approaches that do not align with your organizational code standards.
How to Strike the Right Balance
Balancing the benefits of segmentation with the drawbacks of over-segregation requires thoughtful consideration. Here are some strategies developers can use to avoid this dilemma.
1. Keep Interfaces Focused but Flexible
Instead of creating excessively specific interfaces, structure your interfaces to handle multiple concerns where it makes sense. This means ensuring that an interface provides enough functionality without becoming unwieldy.
Example:
Consider an interface for a payment method. A tightly focused interface could be unnecessary when a single PaymentProcessor
interface can handle various payment types, like credit cards and PayPal.
public interface PaymentProcessor {
void processPayment(double amount);
void refundPayment(double amount);
}
2. Use Default Methods
With Java 8 and later, developers can utilize default methods in interfaces that allow them to evolve without creating new interfaces. This can help alleviate over-segregation while still maintaining necessary functionalities.
public interface PaymentProcessor {
void processPayment(double amount);
default void refundPayment(double amount) {
// Default refund logic, can be overridden.
}
}
3. Review Interface Usage
Regularly revisit your interfaces and their implementations to ensure they still meet your development needs. Ask whether each interface serves a distinct purpose and benefits the project. If it does not, consider refactoring.
4. Favor Composition Over Inheritance
When possible, favor composition over inheritance. Instead of creating a complex hierarchy of interfaces, build simpler interfaces and allow objects to compose behaviors on the fly.
public class PaymentService {
private final PaymentProcessor paymentProcessor;
public PaymentService(PaymentProcessor paymentProcessor) {
this.paymentProcessor = paymentProcessor;
}
public void handlePayment(double amount) {
paymentProcessor.processPayment(amount);
}
}
A Final Look
Interface segregation is a crucial principle for clean architecture, but overdoing it can lead to unintended consequences. A well-balanced approach enhances code usability without introducing unnecessary complexity. Remember to keep interfaces focused but not overly specific, utilize default methods where appropriate, conduct regular code reviews, and prefer composition to minimize dependencies within your code.
In the world of software design, every tool has its place. A nuanced understanding of the balance between interface segregation and over-segregation is essential for developers aspiring to write maintainable, scalable code.
For additional resources on interface design principles, check out Martin Fowler's articles or read more about SOLID principles which provide a strong foundation for good design.
As you continue to refine your skills in Java and software architecture, remember to keep this balance in the forefront of your design considerations. Happy coding!