Mastering Topic Partition Management in Apache Kafka
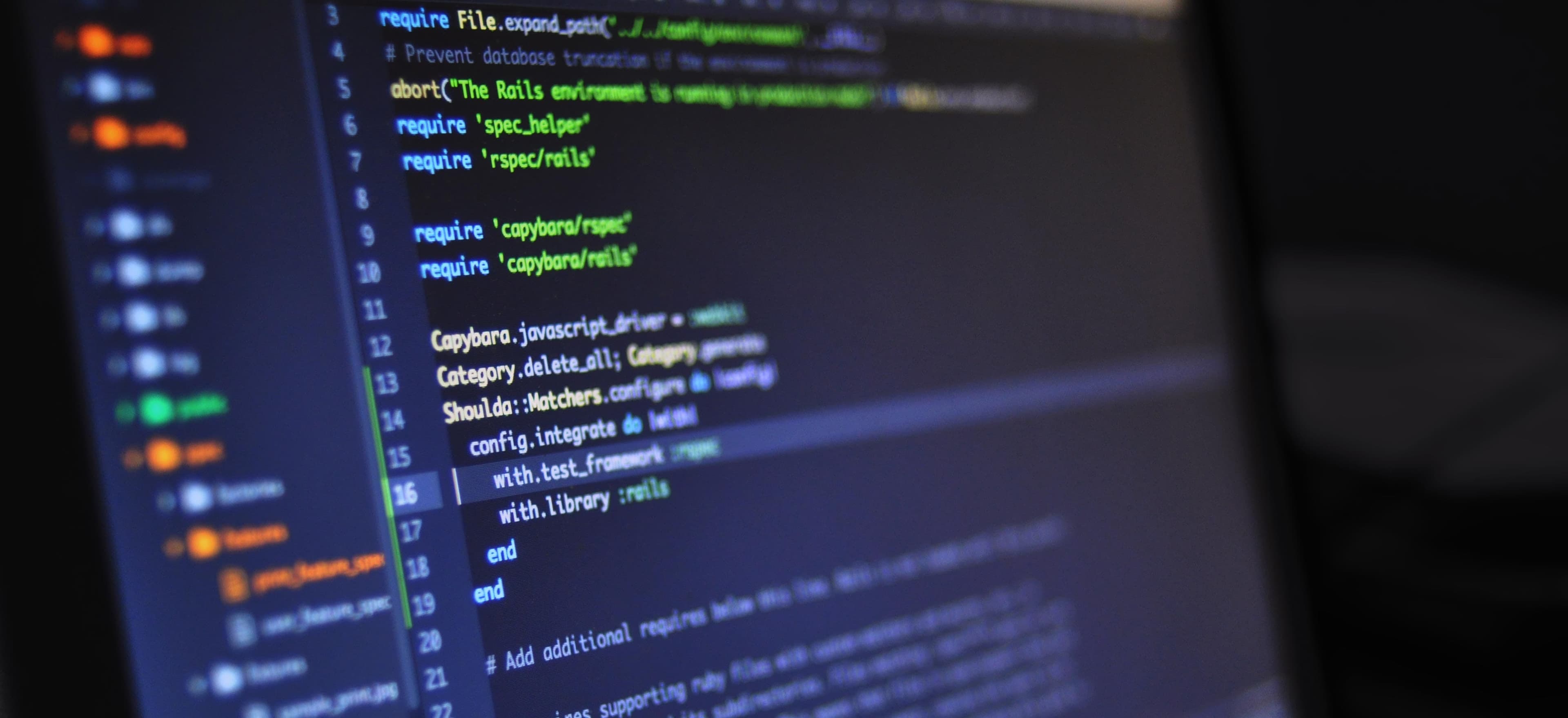
- Published on
Mastering Topic Partition Management in Apache Kafka
Apache Kafka has become the backbone of many modern data architectures due to its robust messaging system that supports high-throughput, fault-tolerant communication among microservices and applications. A key aspect of Kafka’s architecture is its use of topics and partitions. Understanding how to manage these partitions effectively is crucial for optimizing performance and ensuring reliability.
In this post, we’ll dive deep into topic partition management, explaining how partitions work, their significance, and best practices for managing them.
What are Kafka Topics and Partitions?
In Kafka, a topic is a category or feed name to which records are published. When data is produced to a Kafka topic, it is stored in partitions. Each topic can have multiple partitions, which allows Kafka to scale horizontally.
Why Partitions Matter
-
Concurrency: Partitions allow Kafka to handle multiple producers and consumers. Each partition is an ordered, immutable sequence of records that are continually appended. By spreading data across partitions, Kafka can read and write data in parallel, dramatically increasing throughput.
-
Scalability: The ability to add more partitions means you can scale your Kafka deployment. More partitions allow for more brokers and more consumers, which translates to handling more loads.
-
Fault Tolerance: Kafka replicates partitions across brokers for fault tolerance. If one broker fails, others can take over, ensuring that data remains accessible.
Understanding Partitions: The Structure
Each partition in Kafka is identified by a number (partition 0, partition 1, etc.) and is associated with a single topic. The data in a partition is stored in the form of records, which consist of a key, value, and timestamp. Below is how you might visualize the structure of a topic with partitions.
Topic: orders
Partition 0: [Record 1] -> [Record 2] -> [Record 3]
Partition 1: [Record A] -> [Record B]
Partition 2: [Record X] -> [Record Y] -> [Record Z]
Code Snippet: Creating Partitions for a Topic
In this Java example using the Kafka Admin Client, we’ll demonstrate how to create a topic with multiple partitions.
import org.apache.kafka.clients.admin.AdminClient;
import org.apache.kafka.clients.admin.NewTopic;
import java.util.Collections;
import java.util.Properties;
public class KafkaAdmin {
public static void main(String[] args) {
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
AdminClient adminClient = AdminClient.create(props);
// Define a new topic 'orders' with 3 partitions and replication factor of 1
NewTopic newTopic = new NewTopic("orders", 3, (short) 1);
// Create the topic
adminClient.createTopics(Collections.singleton(newTopic));
// Close the admin client
adminClient.close();
System.out.println("Topic created successfully!");
}
}
Why Use Partitions?
Using partitions allows you to balance load across your brokers. In the code above, we're creating a topic named orders
with three partitions. This design choice will help in distributing load effectively when messages are produced and consumed.
Partition Keying and Order Guarantees
When producing messages to a topic, you can specify a key. Kafka will route records with the same key to the same partition, ensuring that they are processed in order.
Code Snippet: Producing Messages with Keys
Here’s an example to show how to produce messages with a key that ensures ordering.
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerRecord;
import java.util.Properties;
public class KafkaProducerExample {
public static void main(String[] args) {
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
KafkaProducer<String, String> producer = new KafkaProducer<>(props);
// Produce messages with a specified key
for (int i = 0; i < 10; i++) {
String key = (i % 2 == 0) ? "even" : "odd"; // Keys are 'even' or 'odd'
producer.send(new ProducerRecord<>("orders", key, "Order #" + i));
}
// Close producer
producer.close();
System.out.println("Messages produced successfully!");
}
}
Why Specify a Key?
By specifying a key, you ensure that all records with the same key go to the same partition. This guarantees order processing for those records. For instance, if your application requires that Order #0
is processed before Order #2
, you can route them together.
Best Practices for Partition Management
1. Determine the Right Number of Partitions
Choosing the number of partitions depends on your application. More partitions can lead to better performance, but more partitions mean higher overhead. Analyzing your throughput and lag is essential.
- Throughput: The total amount of data being processed.
- Lag: The difference between the latest produced message and the latest consumed message.
2. Monitor and Adjust
Use tools like Kafka Manager or Confluent Control Center to monitor partition metrics. Pay close attention to:
- Consumer lag
- Partition distribution across brokers
- Under-replicated partitions
If you notice one partition is getting more traffic than others, consider adding more partitions to balance the load.
3. Rebalance Consumers
With multiple consumers, ensure they are balanced across partitions. Kafka supports automatic rebalancing when a consumer joins or leaves a group. However, occasionally, manual intervention is required based on application behavior.
4. Configure Replication Factor Appropriately
Replication is crucial for fault tolerance. A common practice is to set the Replication Factor to at least 3 for production environments.
Final Thoughts
Mastering partition management in Apache Kafka can significantly enhance your data pipeline's performance and reliability. By leveraging Kafka's fundamental partitioning feature, you can achieve a scalable and resilient architecture.
You can find further details on Partitioning in Kafka and Kafka’s Manager Tools for additional insights and advanced configurations.
Investing your time in understanding and managing partitions will pay dividends in the responsiveness and efficiency of your applications. Tune those configurations, monitor your setup, and embrace the power of Kafka!
Checkout our other articles