Troubleshooting Common Xtext DSL Development Issues
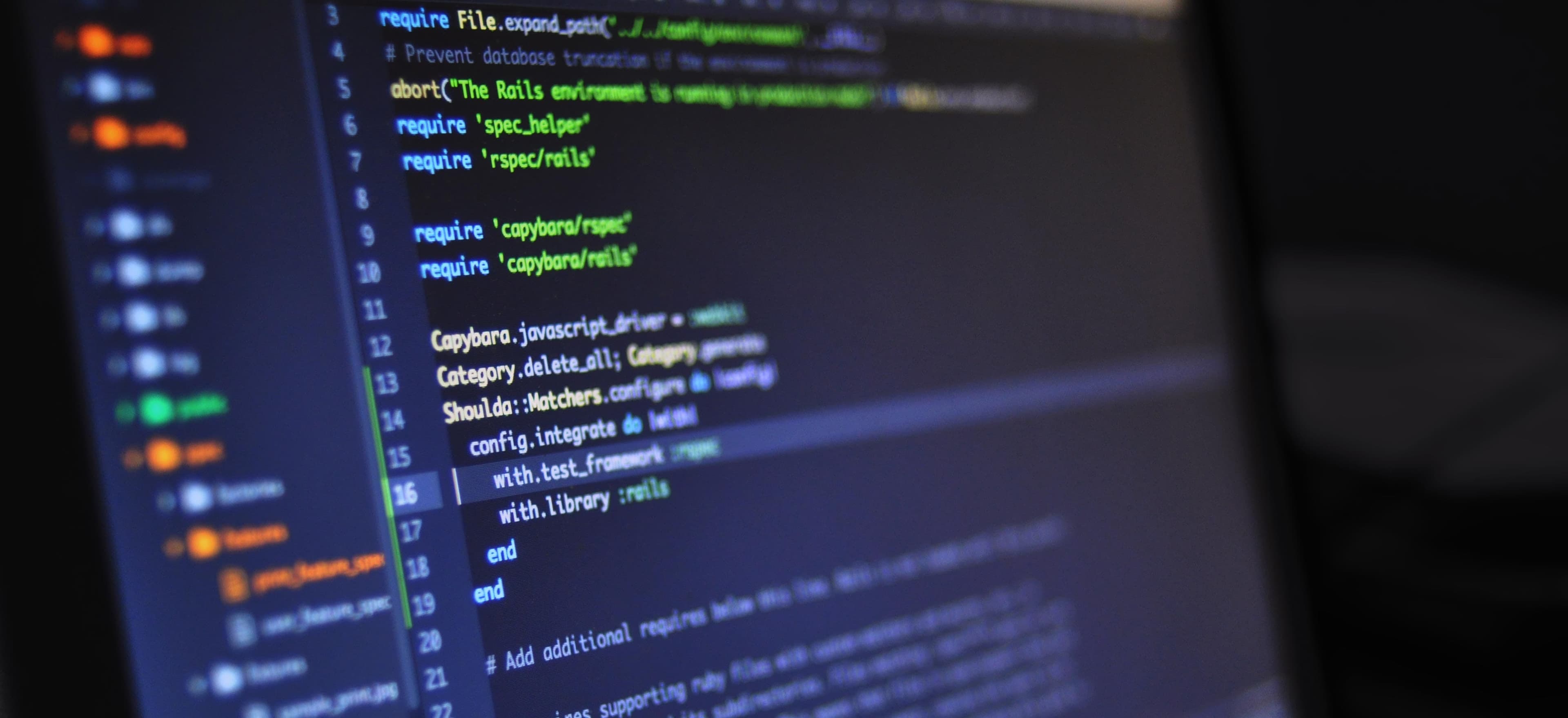
- Published on
Troubleshooting Common Xtext DSL Development Issues
Domain-Specific Languages (DSLs) offer tremendous flexibility and focus for specific tasks in software development, allowing developers to create languages tailored to their project needs. Xtext, a framework for developing DSLs, provides tools and capabilities for efficient language development. However, like any powerful tool, it comes with its challenges. In this blog post, we will explore common issues encountered during Xtext DSL development and suggest solutions to help you overcome them.
Understanding Xtext Basics
Before delving into troubleshooting, let's recap the fundamentals of Xtext. Xtext is built on top of the Eclipse IDE and provides an easy way to define grammar and structure for your DSL. You begin by writing an EBNF grammar that defines your language syntax, which Xtext processes to create syntax highlighting, code completion, and other features.
Example Grammar
Here is an example of a simple Xtext grammar for a fictional language:
grammar org.example.SimpleDSL with org.eclipse.xtext.common.Terminals
generate simpleDsl "http://www.example.org/SimpleDSL"
Model:
greetings+=Greeting*;
Greeting:
'Hello' name=ID '!';
In this example, the grammar defines a DSL that allows writing simple greeting statements.
Common Issues and Solutions
1. Parser Errors
Issue
One of the first issues you might encounter is parser errors that arise when your input does not conform to the defined grammar.
Solution
To troubleshoot parser issues, verify the following:
- Your grammar is defined correctly without errors.
- The structure of your input code matches the defined grammar.
For instance, if the input is:
Hello John!
Ensure that it adheres strictly to the grammar rules. You can use Xtext's built-in validation to catch syntax issues. Here is how you can do basic validation:
@Check
public void checkGreeting(Model model) {
for (Greeting greeting : model.getGreetings()) {
if (greeting.getName() == null) {
error("Name must not be null", YourDslPackage.Literals.GREETING__NAME);
}
}
}
2. Code Completion Not Working
Issue
Another common issue is that code completion does not trigger as expected. This can greatly hamper productivity.
Solution
Ensure that the necessary completions are defined within your AbstractMyDSLProposalProvider
. Use the following snippet to add code completions for names:
public class MyDSLProposalProvider extends AbstractMyDSLProposalProvider {
@Override
public void completeGreeting_Name(EObject model, Assignment assignment, ContentAssistContext context) {
if (assignment.getFeature() == YourDslPackage.Literals.GREETING__NAME) {
// Provide proposals
proposals.add(createCompletionProposal("John"));
proposals.add(createCompletionProposal("Doe"));
}
}
}
3. Invalid Model Representation
Issue
Sometimes, your DSL might accept a valid input but provide an incorrect model representation when saved or processed.
Solution
Check your Serializer
and Parser
implementations. Make sure they handle inputs and transform model elements properly. For example, when serializing, ensure that the model's components maintain their integrity by mapping each DSL element back to its corresponding model representation.
@Override
public void doSerialize(Model model, StringBuilder stringBuilder) {
for (Greeting greeting : model.getGreetings()) {
stringBuilder.append("Hello ").append(greeting.getName()).append("!").append(System.lineSeparator());
}
}
4. Runtime Exceptions
Issue
Runtime exceptions can occur if the runtime context of your language is not appropriately configured.
Solution
Use debugging techniques to trace runtime exceptions. Utilize try-catch blocks where you anticipate exceptions may occur. For example:
try {
// code that may throw an exception
} catch (NullPointerException e) {
System.err.println("Encountered a null pointer: " + e.getMessage());
}
You can also use logging libraries such as SLF4J or Log4j to capture logs that can help you understand the state of your application when an issue arises.
5. Testing the DSL
Issue
Testing your DSL can sometimes lead to a disconnect between expected behavior and actual behavior.
Solution
Ensure that unit tests are written for your grammars and semantic checks. JUnit in combination with Xtext testing facilities allows you to run your DSL in a controlled environment. Below is a simple test snippet:
@RunWith(XtextRunner.class)
public class MyDslTest {
@Test
public void testGreetingParsing() {
String input = "Hello John!";
Model model = parse(input);
assertEquals("John", model.getGreetings().get(0).getName());
}
}
This test case ensures that the parser correctly interprets the input and reflects the expected output.
To Wrap Things Up
Creating a DSL using Xtext can provide immense benefits by streamlining and focusing the development process. However, developers may run into a variety of challenges during this process. By understanding common issues—ranging from parsing errors to runtime exceptions—you can effectively troubleshoot and ensure a smoother development journey.
For further reading on best practices in DSL design and implementation, check out Xtext Language Development and Xtext's GitHub Repository. Embrace these resources, and watch your DSL development process flourish!
In conclusion, the key to successful DSL development lies in understanding the Xtext framework's mechanics and addressing issues as they arise. With patience and practice, you will master the art of creating powerful, efficient, and meaningful DSLs. Happy coding!
Checkout our other articles