WebSockets vs SSE: Choosing the Right Tech for Real-Time Apps
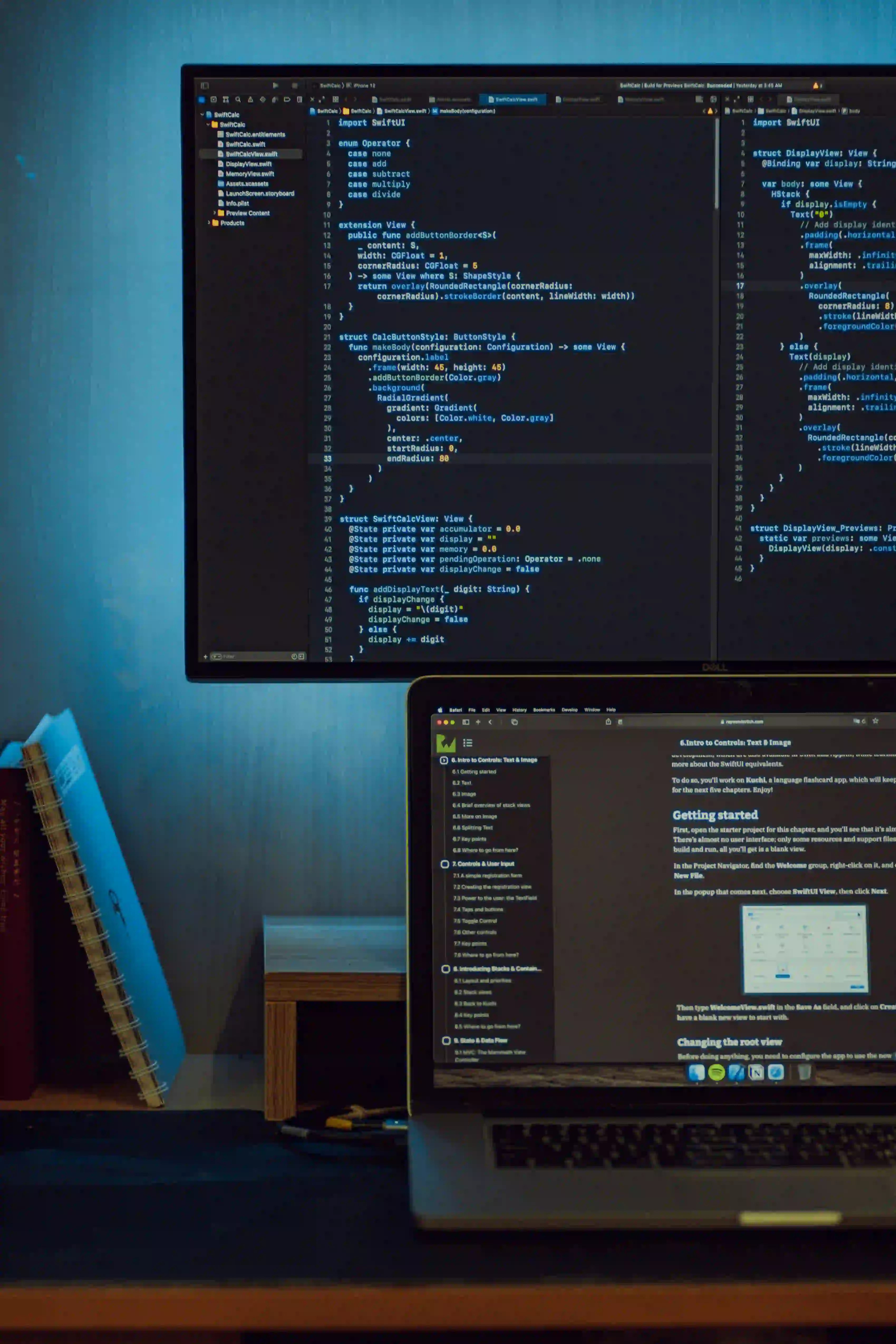
WebSockets vs SSE: Choosing the Right Tech for Real-Time Apps
In the world of web development, real-time communication is a hallmark of modern applications. With the increasing demand for interactive user experiences, developers must choose the right technology to deliver real-time updates efficiently. Two primary contenders in this arena are WebSockets and Server-Sent Events (SSE). But which one is best for your use case? This comprehensive comparison guides you through diverse aspects to help you make an informed decision.
Understanding WebSockets
WebSockets offer a full-duplex communication channel over a single TCP connection. This means that once the connection is established, both the client and server can send and receive messages simultaneously.
Key Features of WebSockets:
- Bi-directional Communication: Both client and server can send messages independently, which is ideal for use cases like chat applications or live notifications.
- Low Latency: WebSockets maintain a persistent connection, resulting in lower latency compared to traditional polling.
- Browser Support: Modern browsers offer robust support for WebSockets, making it a widely-adopted solution.
Example of a WebSocket Connection
const socket = new WebSocket('ws://yourserver.com/path');
socket.onopen = () => {
console.log('WebSocket connection established');
socket.send('Hello Server!');
};
socket.onmessage = (message) => {
console.log(`Received: ${message.data}`);
};
socket.onclose = () => {
console.log('WebSocket connection closed');
};
In this example, we are establishing a WebSocket connection. The onopen
event handler indicates that the connection is ready, the onmessage
handler processes incoming messages, and the onclose
event triggers when the connection is terminated.
Understanding Server-Sent Events (SSE)
On the other hand, Server-Sent Events (SSE) allow servers to send updates to the clients automatically over HTTP. With SSE, the server can send data to the client whenever new information is available.
Key Features of SSE:
- Uni-directional Communication: SSE is designed for one-way data streaming from server to client. This makes it suitable for applications like news feeds, stock price updates, or real-time notifications.
- Automatic Reconnection: If the connection drops, the browser automatically attempts to reconnect to the server.
- Built-in Support for Text-based Data: SSE operates on the text/event-stream MIME type, making it straightforward for real-time text updates.
Example of SSE Connection
const eventSource = new EventSource('/events');
eventSource.onmessage = (event) => {
console.log(`New message: ${event.data}`);
};
eventSource.onerror = (error) => {
console.error('Error occurred:', error);
};
In this snippet, we’re establishing an SSE connection. The onmessage
event handler captures messages from the server, while the onerror
handler manages potential errors.
Comparison of WebSockets and SSE
To determine which technology best fits your needs, consider the following key differences:
1. Communication Direction
- WebSockets: Bi-directional communication allows for complex interactions.
- SSE: Only supports server-to-client streaming.
2. Use Cases
- WebSockets: Ideal for applications requiring real-time data exchange, such as multiplayer games, online collaboration tools, and chat applications.
- SSE: Best suited for server notification, dashboards, and live updates where client interaction isn’t required.
3. Browser Support
Both technologies enjoy strong browser support, but consider checking Can I Use for specific scenarios relevant to your user base.
4. Complexity and Overhead
SSEs are simpler to implement due to their built-in features (like automatic reconnections) and work well with existing HTTP infrastructure. Conversely, WebSocket requires handling both the protocol and server resources, which may increase complexity.
5. Performance
WebSockets usually offer better performance in terms of latency and data transfer. In contrast, the continuous connection nature of WebSockets may consume more server resources.
6. Security
Both WebSockets and SSE can run over HTTPS for added security. However, WebSockets require additional measures like token-based authentication as both transport and data format are distinct from HTTP.
Choosing the Right Technology
When deciding between WebSockets and SSE, you should ask yourself the following questions:
-
What type of communication do I need?
- If you require two-way communication, opt for WebSockets.
- If one-way communication suffices, SSE is more straightforward.
-
What is the expected user experience?
- For interactive applications needing real-time feedback, WebSockets might be preferable.
- For simple notifications and feed updates, SSE is intuitive and effective.
-
What are the resource considerations?
- Consider your server capacity and the complexity you’re prepared to manage when choosing.
-
What is my target audience?
- If you have a diverse user base, ensure to check compatibility with older browsers or devices, which might favor SSE.
In Conclusion, Here is What Matters
Both WebSockets and Server-Sent Events have their distinct strengths. By understanding the requirements of your project and considering factors such as communication direction, use cases, complexity, and performance, you can choose the right technology for your real-time applications effectively.
For further reading on the technical nitty-gritty, you can check out the official documentation for WebSockets and SSE. Choosing the right technology could mean a world of difference in creating delightful user experiences, so consider your options carefully.
Happy coding!