Struggling with Selenium's Link Text? Here's the Fix!
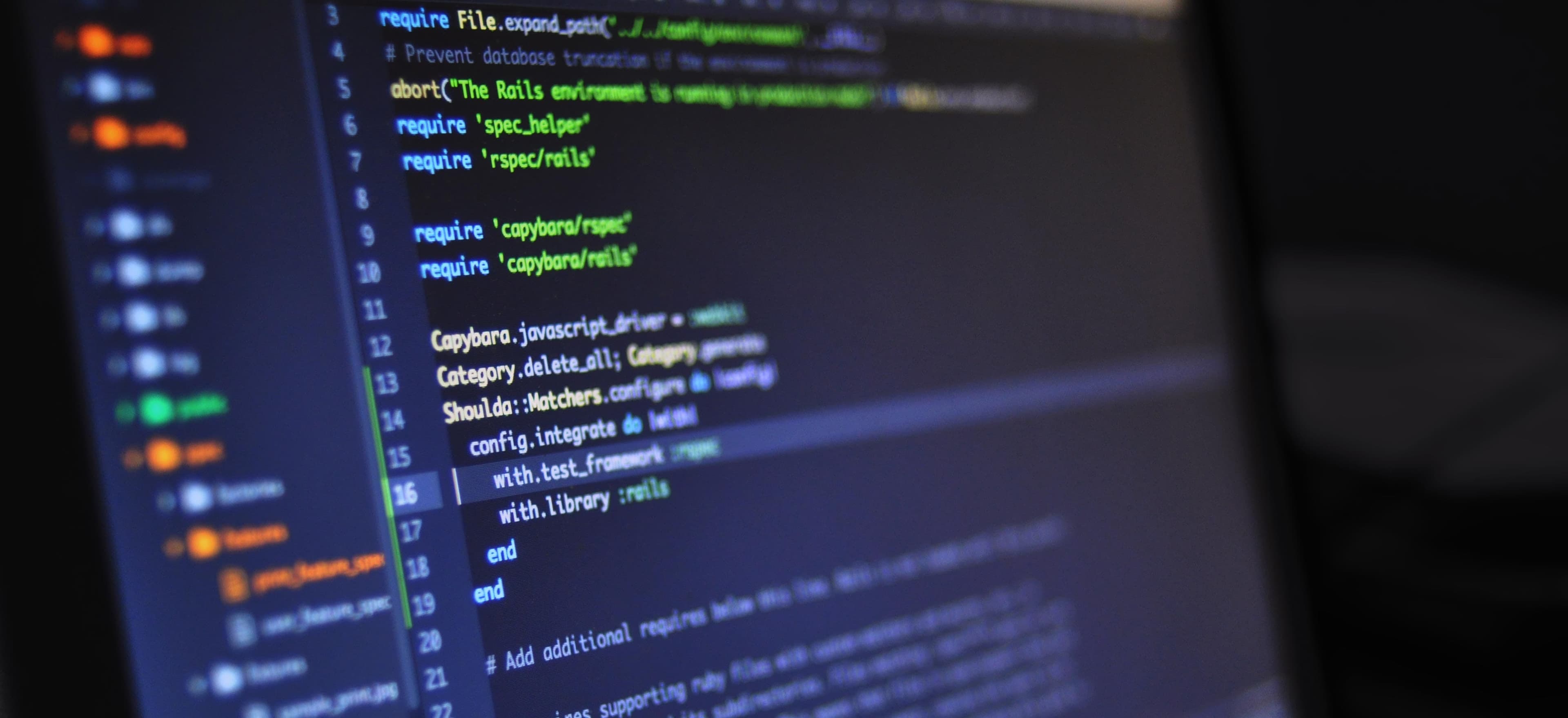
- Published on
Struggling with Selenium's Link Text? Here's the Fix!
Selenium is a powerful tool for automated testing of web applications. While it streamlines our testing process, it can sometimes come with its own set of challenges. A frequent pain point developers encounter revolves around selecting elements using link text. If you've ever experienced issues with locating elements via link text, don’t worry—you’re not alone. In this blog post, we'll explore why the linkText
locator sometimes fails and provide effective workarounds to ensure your tests run smoothly.
Understanding Link Text in Selenium
Before we dive into solutions, let’s clarify what link text is in the context of Selenium. The linkText
locator strategy is used to find anchor tags (<a>
) on a web page by their visible text. The basic method looks like this:
WebElement link = driver.findElement(By.linkText("Click Here"));
link.click();
Using link text is straightforward; however, it may lead to issues if the text is dynamic or if there are multiple links with the same text.
Common Issues with Link Text
-
Dynamic Text: If the link's text changes based on user interaction or data fetched from a server, your link text might not always match what you’re searching for.
-
Duplicate Text: If there are multiple links with the same text,
findElement(By.linkText("text"))
will return the first one it finds, which could lead to erroneous behavior in your tests. -
Hidden Links: Sometimes links may be hidden through CSS, or they may not be present in the DOM when the test runs, resulting in a
NoSuchElementException
. -
Whitespace Sensitivity: Link text is sensitive to whitespace. Extra spaces or different casing can interfere with the correct identification of the link.
Solutions to Link Text Issues
1. Use Partial Link Text
If the full link text is dynamic or duplicated, consider using partialLinkText
. This allows you to locate links that only partially match the text.
WebElement link = driver.findElement(By.partialLinkText("Click"));
link.click();
With partialLinkText
, you only need a substring of the text, making your test more flexible and less fragile.
2. Utilizing XPath
When faced with ambiguities or dynamic text, XPath is a robust alternative. It offers a versatile means to navigate XML documents and can cater to complex conditions.
Here’s a basic example:
WebElement link = driver.findElement(By.xpath("//a[contains(text(), 'Click Here')]"));
link.click();
This XPath will find any anchor tag containing "Click Here" in its text and click it. More complex expressions can further refine your search.
Why Use XPath?
XPath is particularly useful when:
- Your application has dynamic content.
- You want to target elements based on additional attributes (e.g., classes, IDs).
- Elements are generated via JavaScript after the page load.
3. CSS Selectors
Another potential remedy is utilizing CSS selectors, which can help identify links based on attributes and hierarchical relations.
WebElement link = driver.findElement(By.cssSelector("a.my-class"));
link.click();
CSS selectors can be faster than XPath, particularly in modern web browsers. They offer a clean way to find elements using class, ID, or even combinations of attributes.
4. Wait Mechanisms
Timings issues can also play a role in the failure of locating elements. Implement smart waits to ensure that the elements are located correctly.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement link = wait.until(ExpectedConditions.elementToBeClickable(By.linkText("Click Here")));
link.click();
Here, we ensure that the specified link is clickable before attempting to interact with it. This helps in reducing issues caused by timing.
Best Practices
-
Avoid Hard-Coding Text: Hard-coded link texts can make your tests brittle. Instead, consider storing them in a configuration file or constants.
-
Keep Text Consistent: If you have control over the application code, make sure the link text remains consistent.
-
Add Assertions: When testing, always ensure assertions accompany your interactions to validate expected outcomes.
A Final Look
Navigating Selenium's linkText
locator can be tricky, but with these strategies, you can mitigate common issues effectively. Leverage partialLinkText
, XPath, CSS selectors, and clear timing practices to improve the reliability of your tests.
For more information on using Selenium WebDriver efficiently, take a look at Selenium Official Documentation and explore more advanced techniques and features.
By integrating these practices into your testing suite, you can elevate your automated testing experience and maintain robust test coverage. The challenges of link text selection will become merely a footnote in your Selenium journey!