Mastering Enum to String: Avoid Common Pitfalls
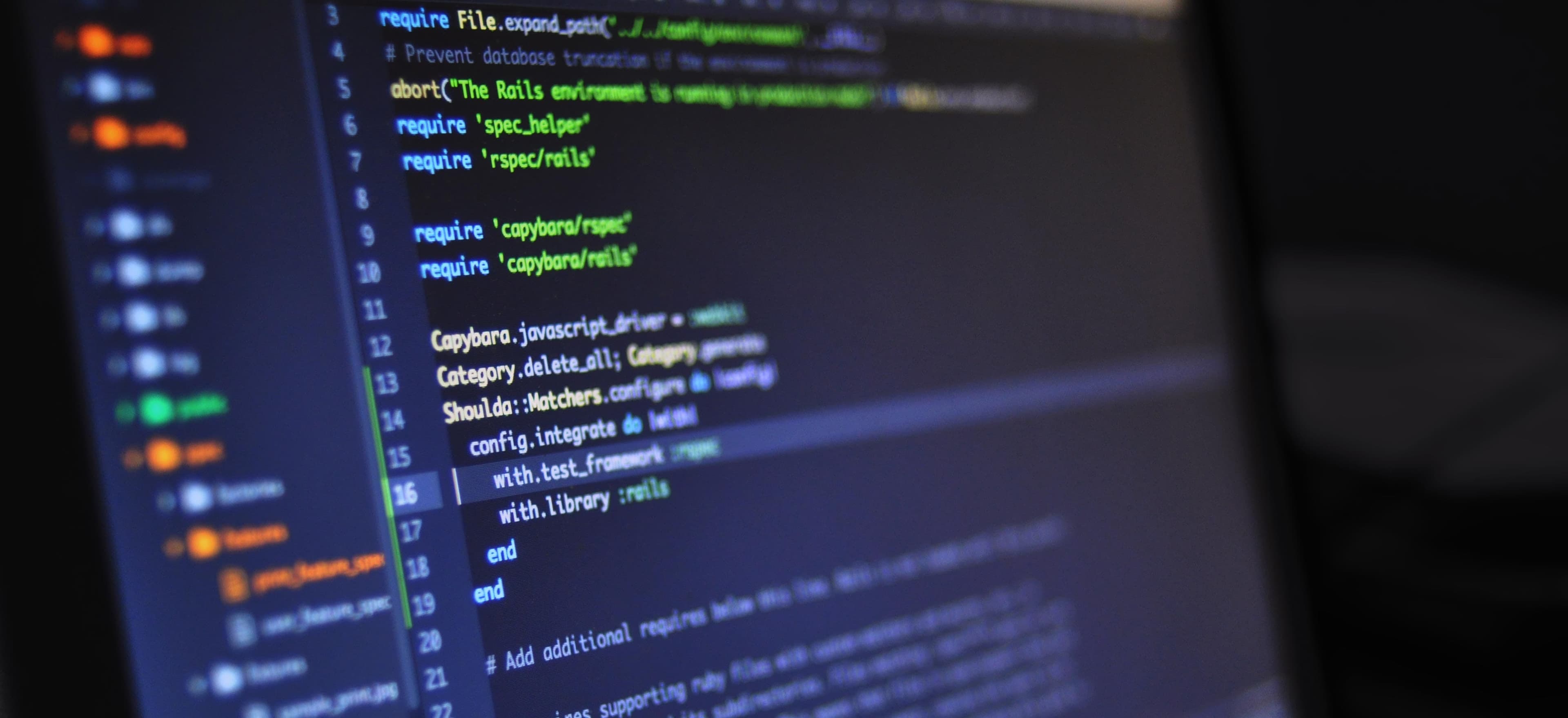
- Published on
Mastering Enum to String: Avoid Common Pitfalls in Java
Enums in Java are special classes that represent a group of constants. They are powerful and versatile. However, converting enum values to strings can sometimes lead to confusion and errors. In this post, we'll explore the intricacies of converting enums to strings, identify common pitfalls, and provide best practices to avoid them.
What is an Enum?
In Java, an enum (short for enumeration) is a type that defines a collection of constants. Enums provide a way to define a fixed set of possible values in a type-safe manner.
Example of Enum Definition
Here is a simple example of defining an enum:
public enum Color {
RED, GREEN, BLUE;
}
Enums can have fields, methods, and constructors, making them quite powerful.
Converting Enums to Strings
When you need to display an enum value or store it as a string in a database, you will often need to convert it to a string. The simplest way to convert an enum to a string is to use the name()
method. This will return the exact name of the enum constant:
Color color = Color.RED;
String colorString = color.name(); // "RED"
However, there are common pitfalls associated with this straightforward conversion.
Pitfall #1: Using name()
Inconsistently
The name()
method returns the original enum name as defined. This can be misleading if you expect a more user-friendly representation.
Consider the following enum:
public enum PaymentStatus {
PENDING_PAYMENT,
PAID,
PAYMENT_FAILED;
}
If you convert the PENDING_PAYMENT
enum to a string using name()
:
PaymentStatus status = PaymentStatus.PENDING_PAYMENT;
String statusString = status.name(); // "PENDING_PAYMENT"
This string may not be user-friendly. Ideally, you might want to display "Pending Payment" instead.
Solution: Custom toString() Method
To tackle this, override the toString()
method to provide a more descriptive output:
public enum PaymentStatus {
PENDING_PAYMENT("Pending Payment"),
PAID("Paid"),
PAYMENT_FAILED("Payment Failed");
private final String displayName;
PaymentStatus(String displayName) {
this.displayName = displayName;
}
@Override
public String toString() {
return this.displayName;
}
}
Now, calling toString()
will give you a user-friendly string:
String statusString = status.toString(); // "Pending Payment"
This approach is clean, readable, and self-documenting.
Pitfall #2: Ignoring Localization
If your application needs to support multiple languages, hard-coding strings into your enum toString()
method can be problematic. Instead, consider using a resource bundle for localization.
Here's how you can modify our previous example:
import java.util.Locale;
import java.util.ResourceBundle;
public enum PaymentStatus {
PENDING_PAYMENT,
PAID,
PAYMENT_FAILED;
public String getLocalizedString(Locale locale) {
ResourceBundle bundle = ResourceBundle.getBundle("messages", locale);
return bundle.getString(this.name());
}
}
In this case, the messages.properties
file could contain:
PENDING_PAYMENT=Pending Payment
PAID=Paid
PAYMENT_FAILED=Payment Failed
Pitfall #3: Inconsistent Enum Values
Mixing different naming conventions can cause issues when converting enums to strings. For instance, using underscores in some enums but camel case in others can lead to inconsistencies.
Best Practice: Follow a Consistent Naming Convention
Decide on a naming convention for your enums. For instance, it is common to use UPPER_CASE
for enum constants. If you need different formats, you can always provide a method to convert them:
public enum UserRole {
ADMIN("Administrator"),
MODERATOR("Moderator"),
USER("Regular User");
private final String displayName;
UserRole(String displayName) {
this.displayName = displayName;
}
public String toSnakeCase() {
return this.name().toLowerCase().replace('_', ' ');
}
}
By maintaining a uniform style across your application, your codebase becomes more maintainable.
Common Enum Conversion Patterns
Using EnumMap for Enhanced Lookup
If you need to associate enums with more complex data (like colors or other metadata), consider using an EnumMap
.
import java.util.EnumMap;
public enum Fruit {
APPLE, BANANA, ORANGE;
}
EnumMap<Fruit, String> fruitColors = new EnumMap<>(Fruit.class);
fruitColors.put(Fruit.APPLE, "Red");
fruitColors.put(Fruit.BANANA, "Yellow");
fruitColors.put(Fruit.ORANGE, "Orange");
String appleColor = fruitColors.get(Fruit.APPLE); // "Red"
This structure allows efficient lookups and a clearer association between your enum constants and their respective properties.
Safety with Switch Expression
When switching on enums, Java 14 and later provide a more concise way to handle switches. This reduces errors and keeps your conversion logic clean:
public String describeFruit(Fruit fruit) {
return switch (fruit) {
case APPLE -> "A round fruit that is typically red or green.";
case BANANA -> "A long yellow fruit.";
case ORANGE -> "A citrus fruit.";
};
}
Key Takeaways
Converting enums to strings can be straightforward, but it involves careful design considerations to avoid common pitfalls. By implementing user-friendly strings, considering localization, maintaining consistency, leveraging EnumMap
, and utilizing modern features like the switch expression, you can avoid many common mistakes.
For further reading on best practices with enums, you may visit Oracle's official Java documentation.
By mastering these concepts, you will make your Java applications more robust, user-friendly, and maintainable. Happy coding!