Fixing Auto-Increment Issues in Spring Test Methods
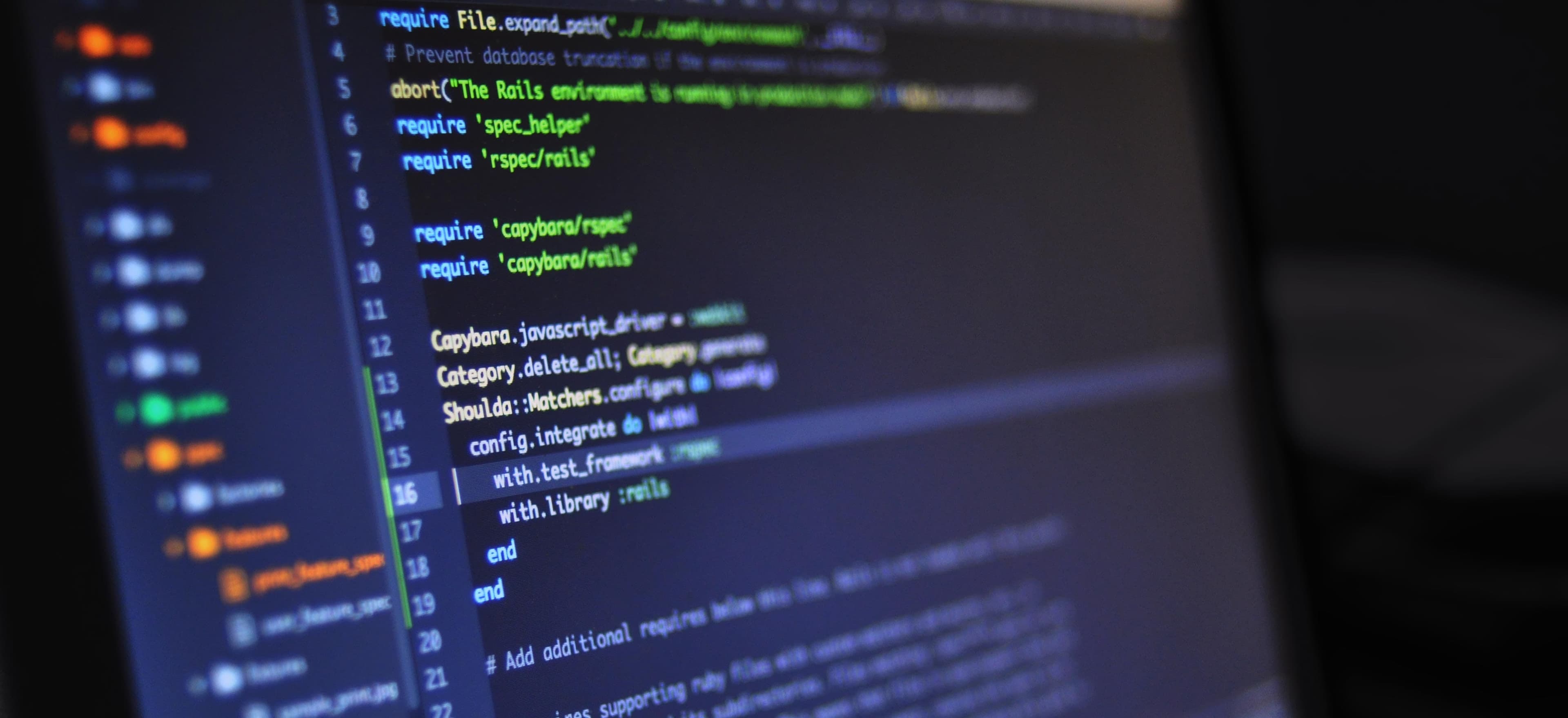
- Published on
Fixing Auto-Increment Issues in Spring Test Methods
When it comes to testing in Spring, especially when dealing with databases, developers can run into a variety of issues. One common challenge is ensuring that auto-incremented fields behave as expected in test cases. This blog post aims to delve into possible solutions for fixing auto-increment issues in Spring test methods.
Understanding Auto-Increment Behavior
Auto-increment is a feature in SQL that automatically generates a unique number whenever a new record is inserted into a table. While this simplifies record management, it can cause complications during testing. When executing tests, especially with in-memory databases, developers may find records not being assigned unique identifiers correctly.
Imagine you have an entity User
defined in your Java project:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// Getters and setters
}
The Challenge
In a testing scenario, if multiple instances of User
are created and saved, they may share IDs or give you unexpected values due to the state of the auto-increment sequence not being reset.
Strategies for Fixing Auto-Increment Issues
1. Use @Transactional
Annotation
By annotating your test methods with @Transactional
, you can ensure that all the database operations performed within that method are wrapped in a transaction. Once the method completes, the transaction rolls back, leaving the database in its original state. This is particularly useful for ensuring that the auto-increment counter resets after every test, which helps avoid ID collisions.
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.annotation.Rollback;
import org.springframework.transaction.annotation.Transactional;
@Transactional
public class UserRepositoryTest {
@Autowired
private UserRepository userRepository;
@Test
@Rollback
public void testCreateUser() {
User user = new User();
user.setName("John Doe");
userRepository.save(user);
// Validate that the user was saved correctly
assertNotNull(user.getId());
}
}
2. Clean the Database Before Each Test
Another effective strategy involves manually cleaning the database before each test. Spring provides the @BeforeEach
method where you can execute cleanup logic. Here’s how to implement it:
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
// Import necessary annotations
public class UserRepositoryTest {
@Autowired
private UserRepository userRepository;
@BeforeEach
public void cleanup() {
userRepository.deleteAll();
}
@Test
public void testSaveMultipleUsers() {
User user1 = new User();
user1.setName("Jane Doe");
userRepository.save(user1);
User user2 = new User();
user2.setName("Joe Bloggs");
userRepository.save(user2);
// Verify that each user has been assigned a unique ID
assertNotEquals(user1.getId(), user2.getId());
}
}
3. Using H2 in Memory Database
When developing and testing with Spring, you might choose H2 as an in-memory database. H2 has some limitations with sequences compared to other databases like MySQL or PostgreSQL. However, you can set it up to handle auto-increment fields properly. Here’s an example configuration:
spring:
datasource:
url: jdbc:h2:mem:testdb;DB_CLOSE_DELAY=-1;DB_CLOSE_ON_EXIT=FALSE
driver-class-name: org.h2.Driver
username: sa
password:
h2:
console:
enabled: true
By using H2, you can also configure the auto-increment for testing purposes:
CREATE TABLE user (
id BIGINT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255)
);
4. Resetting Auto-Increment Sequence
For databases that require resetting the auto-increment sequence explicitly, you can do so in your setup.
@BeforeAll
public static void resetAutoIncrement() {
jdbcTemplate.execute("ALTER TABLE user AUTO_INCREMENT = 1");
}
This ensures that every time the tests run, the auto-increment field starts from a known state.
5. Mocking the Repository
In some testing scenarios, you might not even want to rely on the database. Instead, you can mock your repository using Mockito:
import static org.mockito.Mockito.*;
public class UserServiceTest {
private UserService userService;
private UserRepository userRepository;
@BeforeEach
public void setup() {
userRepository = mock(UserRepository.class);
userService = new UserService(userRepository);
}
@Test
public void testSaveUser() {
User user = new User();
user.setName("John Doe");
when(userRepository.save(any())).thenReturn(user);
User savedUser = userService.save(user);
assertNotNull(savedUser);
assertEquals("John Doe", savedUser.getName());
}
}
Mocking allows you to focus solely on your service logic without overwhelming concerns about the database state or constraints.
Wrapping Up
Testing auto-incremented fields can present challenges in a Spring environment. Whether you opt for using the @Transactional
annotation, cleaning the database before each test, setting up an in-memory database, resetting sequences, or mocking components, each method comes with its own advantages.
By applying these strategies, you can ensure a smoother development process and avoid potential pitfalls associated with auto-increment issues in test methods. Testing should be about verification and assurance, not dealing with unforeseen database conflicts!
Further Reading
- Spring Documentation: Testing Database
- Hibernate Documentation: Entity and Table Management
If you have other strategies that worked for you or personal experiences with Spring testing, feel free to share them in the comments! Happy coding!
Checkout our other articles