How to Avoid Data Loss with Spring Integration Splitters
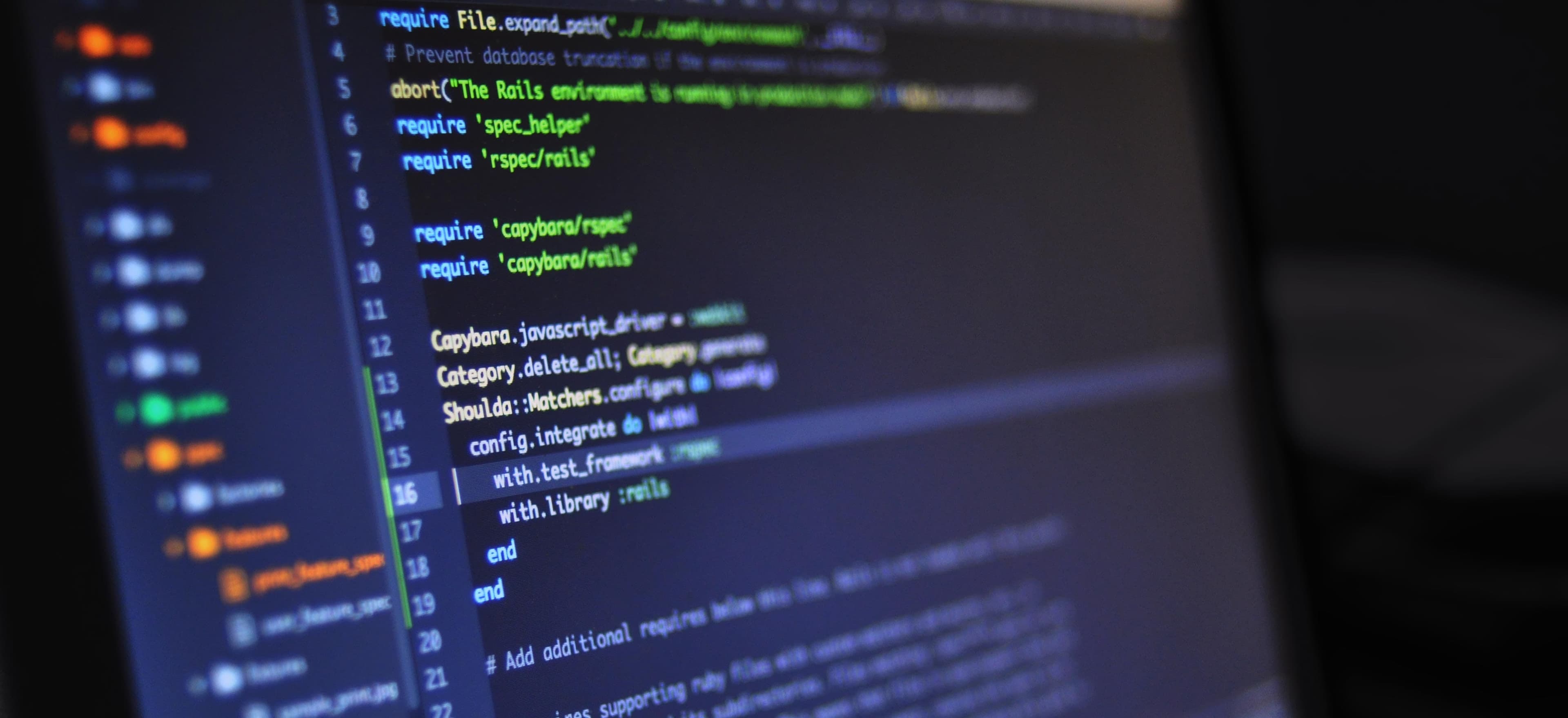
- Published on
How to Avoid Data Loss with Spring Integration Splitters
In the world of application development, data loss is one of the critical issues that developers strive to avoid. When you are building complex integration workflows, using Spring Integration Splitters can help you manage message handling effectively, but they can also introduce risks if not used correctly. In this article, we'll explore how to leverage Spring Integration Splitters while ensuring data integrity and avoiding loss.
Table of Contents
- What is Spring Integration?
- Understanding Splitters
- Challenges with Splitters
- Best Practices to Avoid Data Loss with Splitters
- Example: Implementing Spring Integration Splitters Safely
- Conclusion
What is Spring Integration?
Spring Integration is an extension of the Spring Framework that supports building messaging-centric applications. It provides a wide variety of components that simplify the implementation of messaging patterns in your application. These components include channels, endpoints, transformers, and more.
For a deeper understanding of Spring Integration, check the official documentation.
Understanding Splitters
Splitters are crucial in a Spring Integration context. As the name suggests, they break a single message into multiple messages. This allows for parallel processing, improving performance and throughput.
Here's a basic example:
@Bean
public IntegrationFlow splitFlow() {
return IntegrationFlows.from("inputChannel")
.split()
.handle("processor", "process")
.get();
}
Why Use a Splitter?
Splitters are used to divide a message payload into manageable portions for further processing. This granularity enables better resource utilization, parallelism, and can help in managing workloads effectively.
Challenges with Splitters
While Splitters offer substantial flexibility, they can introduce challenges, especially regarding message handling. The fundamental risks include:
- Message loss: If a message fails during processing and isn't properly handled, it can get lost.
- Concurrency issues: Multiple instances of a message may result in race conditions if not managed correctly.
- Data integrity: Splitting messages without proper context can lead to partial updates or corrupt data states.
Best Practices to Avoid Data Loss with Splitters
To mitigate risks, here are some best practices:
1. Use Error Handling Mechanisms
An essential safeguard in any messaging system is robust error handling. Spring Integration allows you to specify error channels for handling errors gracefully.
Here is how you can configure error handling:
@Bean
public IntegrationFlow splitFlowWithErrorHandling() {
return IntegrationFlows.from("inputChannel")
.split()
.handle("processor", "process")
.get();
}
@Bean
public IntegrationFlow errorFlow() {
return IntegrationFlows.from("errorChannel")
.handle("errorHandler", "handleError")
.get();
}
2. Ensure Proper Message Routing
After splitting messages, ensure that they are routed correctly to their respective handlers. A lost message can often result from improper routing.
@Bean
public IntegrationFlow routingFlow() {
return IntegrationFlows.from("inputChannel")
.split()
.route(Message.class, this::determineRoute)
.handle("processor", "process")
.get();
}
private String determineRoute(Message<?> message) {
// Define logic to determine route based on message content
}
3. Consider Transaction Management
Utilizing transactions can help in maintaining consistency. If one part of a transaction fails, the entire process can roll back, preventing data loss.
Example of using transactions in Spring Integration:
@Bean
public IntegrationFlow transactionalFlow() {
return IntegrationFlows.from("inputChannel")
.split()
.handle("processor", "process")
.get();
}
@Transactional
public void process(Message<?> message) {
// Processing logic
// In case of exception, the transaction will rollback
}
4. Monitor Performance and Scaling
Keeping an eye on your processes allows for timely interventions before data loss occurs. Spring Integration supports various mechanisms, such as application metrics and logging.
Make use of Spring Boot Actuator to monitor your application effectively. You can enable various endpoints for health and metrics reporting:
management:
endpoints:
web:
exposure:
include: health, metrics
Example: Implementing Spring Integration Splitters Safely
Let's create a simple Spring Integration application that uses splitters while following best practices to avoid data loss.
Step 1: Set Up Spring Boot Application
Ensure you have the necessary dependencies in your pom.xml
or build.gradle
.
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
Step 2: Configure Splitter Flow with Error Handling
@Configuration
@EnableIntegration
public class IntegrationConfig {
@Bean
public MessageChannel inputChannel() {
return new DirectChannel();
}
@Bean
public MessageChannel errorChannel() {
return new DirectChannel();
}
@Bean
public IntegrationFlow splitFlow() {
return IntegrationFlows.from(inputChannel())
.split()
.handle("processor", "processMessage")
.get();
}
@Bean
public IntegrationFlow errorFlow() {
return IntegrationFlows.from(errorChannel())
.handle("errorHandler", "handleError")
.get();
}
}
Step 3: Create a Processor Service
Implement a processor that handles each split message:
@Service
public class MessageProcessor {
public void processMessage(String message) {
// Simulate message processing
if (message.contains("error")) {
throw new RuntimeException("Processing error!");
}
System.out.println("Processing message: " + message);
}
}
Step 4: Configure an Error Handler
Design an error handler that captures failed messages:
@Service
public class ErrorHandler {
public void handleError(Message<?> message) {
// Handle the error, possibly logging it or sending it to another service
System.err.println("Error processing message: " + message.getPayload());
}
}
Lessons Learned
Navigating the intricacies of data integration with Spring Integration Splitters involves a keen understanding of best practices to avoid data loss. By implementing robust error handling, ensuring proper message routing, utilizing transactions, and actively monitoring performance, you can build resilient integration flows that stand the test of time.
By following the strategies and example provided in this post, you will be better equipped to prevent data loss in your Spring Integration applications. For more information, feel free to check out Spring Integration's official documentation.
Embracing these practices not only secures the data but also enhances the overall efficiency of your application. Happy coding!