Common Mistakes in REST Parameter Handling
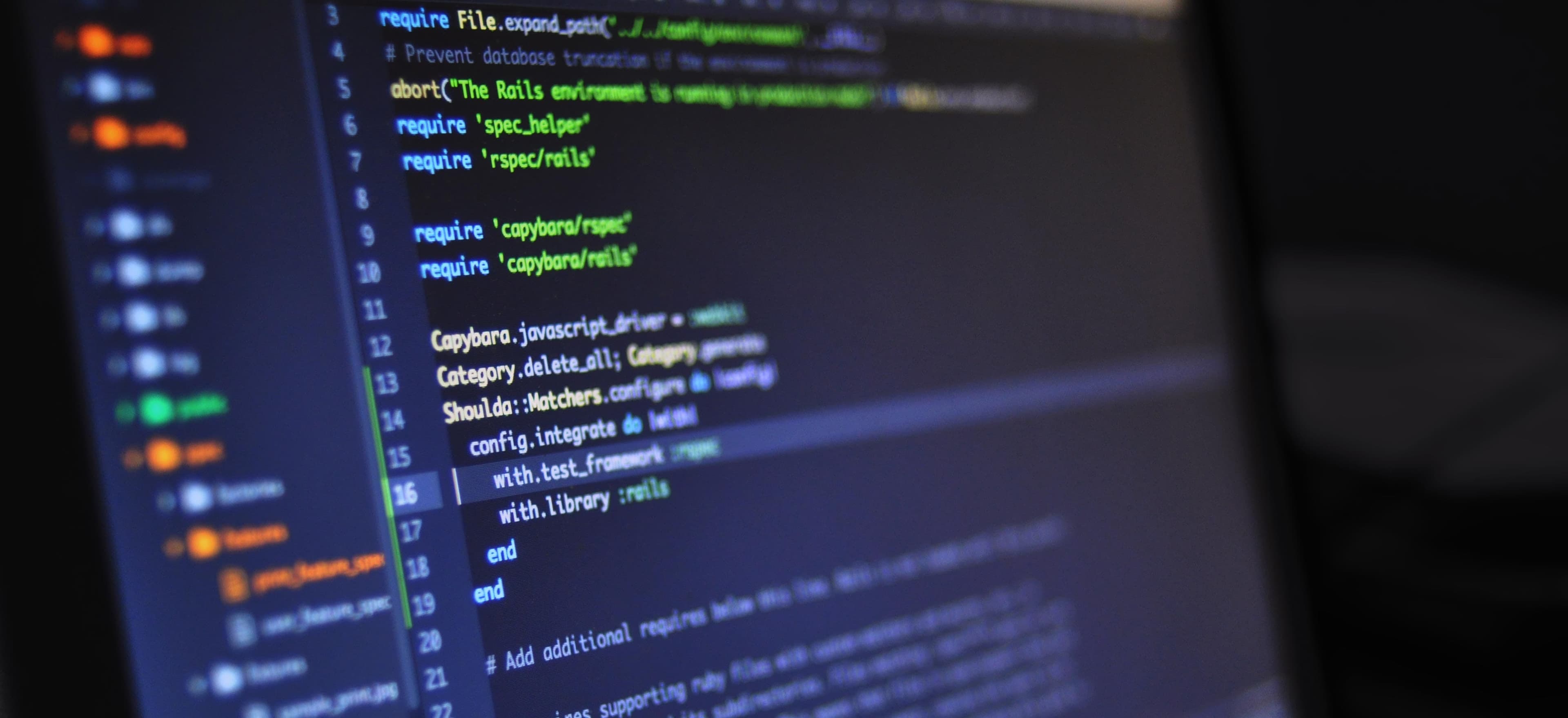
- Published on
Common Mistakes in REST Parameter Handling
The Starting Line
RESTful web services have become a de facto standard for building scalable and efficient applications. Parameters play a pivotal role in RESTful API design — they define how clients interact with your services. However, improper handling of these parameters can lead to a multitude of issues that can plague both functionality and maintainability. This blog post will explore common mistakes in REST parameter handling, offering practical guidance and code examples to help you avoid these pitfalls.
Understanding Parameters in REST
In REST APIs, parameters are key-value pairs that provide inputs to operations. They can generally be classified into three categories:
-
Query Parameters: These are typically found in the URL and modify the function of an endpoint. For example, in the request
/posts?author=john&tag=java
,author
andtag
are query parameters. -
Path Parameters: These are part of the endpoint's URL and are usually used to identify specific resources. For instance, in
/users/123
,123
is a path parameter representing the user ID. -
Body Parameters: These are included in the body of the request. They are often used in POST and PUT requests where you need to send complex data objects.
By understanding the different types of parameters, we can better manage their usage in a RESTful application.
Common Mistakes in Parameter Handling
1. Ignoring Validation for Query Parameters
One of the most common oversights when dealing with query parameters is neglecting to validate their values. Failing to validate these parameters can lead to unexpected behavior or even security vulnerabilities.
Why Validation is Important
Validation ensures that the values received are of the expected format and fall within the acceptable range. This prevents malicious users from sending invalid data which could compromise your application.
Example Code Snippet
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api/posts")
public class PostController {
@GetMapping
public List<Post> getPosts(@RequestParam(required = false) String author,
@RequestParam(required = false, defaultValue = "1") int page) {
if (page < 1) {
throw new IllegalArgumentException("Page number must be greater than zero");
}
// Proceed to fetch posts based on author and page
return postService.fetchPosts(author, page);
}
}
In this example, we validate the page
parameter to ensure it is a positive integer. This reduces the risk of returning invalid data and enhances overall efficiency.
2. Not Utilizing HTTP Methods Correctly
Another frequent mistake is misusing HTTP methods for RESTful invocations. Each method (GET, POST, PUT, DELETE) has a specific semantic meaning and abusing these can lead to confused APIs.
Why Proper Use is Critical
Using HTTP methods incorrectly can lead to significant limitations in your API's usability and leads to confusion among users about how to properly invoke API functions.
Example Code Snippet
@RestController
@RequestMapping("/api/users")
public class UserController {
@PostMapping
public User createUser(@RequestBody User user) {
// Create user logic
}
@GetMapping("/{id}")
public User getUser(@PathVariable int id) {
// Retrieve user logic
}
@PutMapping("/{id}")
public User updateUser(@PathVariable int id, @RequestBody User user) {
// Update user logic
}
@DeleteMapping("/{id}")
public void deleteUser(@PathVariable int id) {
// Delete user logic
}
}
In this example, all CRUD operations are clearly defined with appropriate HTTP methods. This makes the API predictable and user-friendly.
3. Inconsistent Naming Conventions
Inconsistently naming API parameters can confuse developers. A poorly designed API might have different formats for similar concepts, making it harder to use.
Why Consistency Matters
Uniformity in naming conventions ensures that clients can easily understand and predict API behavior. This minimizes learning curves and accelerates integration.
Example Code Snippet
@GetMapping("/products")
public List<Product> getProducts(@RequestParam(name = "category") String category,
@RequestParam(name = "sortBy", defaultValue = "name") String sortBy) {
// Fetch products based on category and sort
}
Here, we use consistent naming for query parameters. Clients can readily understand category
and sortBy
are used to filter products.
4. Exposing Implementation Details
When designing APIs, exposing too much implementation detail through URLs or parameters can lead to tight coupling.
Why Encapsulation is Essential
Encapsulating implementation details allows you to change underlying systems without breaking your API. Keep your public interfaces clean and user-friendly.
Example Code Snippet
@GetMapping("/search")
public List<Product> searchProducts(@RequestParam String query) {
// Business logic for searching products
}
Instead of exposing unique identifiers or internal structures, we use a simple query
parameter to search products. This adds a layer of abstraction.
5. Overloading GET Requests with Side Effects
When using GET requests, some developers mistakenly incorporate side effects, such as creating or updating resources. This violates REST principles.
Why REST Should Be Pure
GET requests should be idempotent, meaning they should not modify resources. This makes your API predictable and cacheable.
6. Neglecting Documentation
Failing to document parameters can be detrimental. Without clear documentation, users struggle to understand the API.
Why Documentation is Key
Comprehensive documentation enables better client experience and ease of integration with your API.
Example Documentation Structure
### API Documentation
#### GET /api/posts
**Query Parameters:**
- `author` (optional): The author of the posts.
- `page` (optional): The page number for pagination. Defaults to 1.
**Response:**
Returns a list of posts.
The Bottom Line
Handling REST parameters may seem straightforward, but it is a nuanced process that can bring about challenges. By being aware of common pitfalls — such as neglecting validation, misusing HTTP methods, inconsistent naming conventions, exposing implementation details, overloading GET requests, and failing to document properly — you can significantly improve the quality of your RESTful APIs.
For a deeper dive into RESTful API principles, you might find these resources helpful:
- RESTful Web Services Tutorial
- Understanding REST
By avoiding these common mistakes, you create robust, maintainable, and user-friendly APIs that can scale effectively. Happy coding!
Checkout our other articles