Common Pitfalls in Java EE Entity Class Design
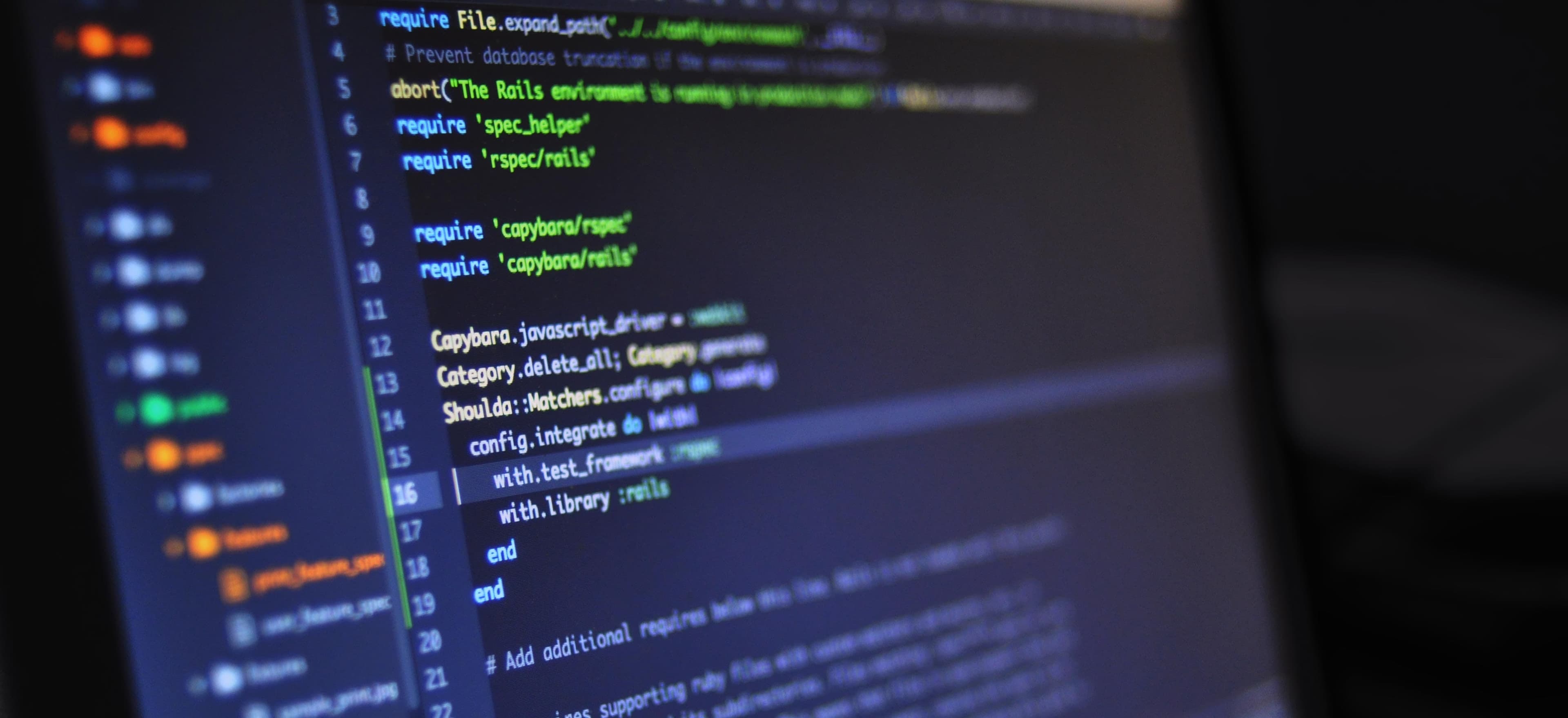
- Published on
Common Pitfalls in Java EE Entity Class Design
Java EE (Enterprise Edition) is a robust platform used for building scalable, portable, and secure enterprise applications. Central to Java EE are entity classes, which represent persistent data and are crucial for any data-driven Java application. However, improper design of these entity classes can lead to various issues, impacting performance and maintainability. In this blog post, we will explore common pitfalls in Java EE entity class design while providing solutions and best practices.
1. Ignoring the Importance of Serializable
Why is Serialization Important?
Java EE typically involves storing and transferring entity objects, especially when dealing with persistent storage or remote messaging. Thus, making entity classes implement the Serializable
interface is essential.
Common Mistake:
Developers often overlook this, which can lead to runtime exceptions or NotSerializableException
when attempting to send objects over a network or to persist them.
Solution:
Always implement the Serializable
interface in your entity classes. Here’s an example:
import java.io.Serializable;
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class User implements Serializable {
@Id
private Long id;
private String username;
// Constructors, getters, setters
}
In this manner, your User
class can be serialized without issues, ensuring data integrity during transport.
2. Using Non-Serializable Fields
Why Avoid Non-Serializable Fields?
If your entity includes non-serializable fields, it may lead to unexpected failures.
Common Mistake:
Including fields such as Connection
, Statement
, or any third-party library objects that are not serializable.
Solution:
@Entity
public class Order implements Serializable {
@Id
private Long orderId;
private String orderDescription;
// Avoid any non-serializable field
// private Connection connection; // BAD PRACTICE
public Long getOrderId() {
return orderId;
}
public void setOrderId(Long orderId) {
this.orderId = orderId;
}
}
Make sure to use only serializable fields and reserve complex objects exclusively for business logic, separate from your entity classes.
3. Not Utilizing Identifier Generation Strategies
Why Identifier Generation Matters
Using a proper identifier generation strategy can prevent key conflicts and ensure uniqueness across entities.
Common Mistake:
Hardcoding identifiers or manually managing them can lead to duplicate keys and integrity issues.
Solution:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY) // Automatically generates unique IDs
private Long productId;
private String productName;
// Getters and setters
}
Implementing the @GeneratedValue
annotation allows the database to handle the uniqueness of IDs, significantly reducing the risk of conflicts while ensuring seamless integration with various database types.
For further reading on identifier generation in JPA, check this JPA Generation Types documentation.
4. Over-Reliance on Lazy Loading
What is Lazy Loading?
Lazy loading is a design pattern that delays the loading of an entity’s data until it is needed. It can optimize performance, but it can also lead to issues.
Common Mistake:
Setting too many fields to lazy fetch can result in LazyInitializationException
when access is attempted outside of a transaction.
Solution:
@Entity
public class Order {
@Id
private Long orderId;
@OneToMany(fetch = FetchType.LAZY) // Use cautiously
private List<OrderItem> items;
// Getters and setters
}
Consider using FetchType.EAGER
when necessary to ensure vital relationships are loaded when you retrieve the parent entity, thereby preventing lazy loading pitfalls.
5. Lack of Entity Validation
Why Validate Entities?
Not validating data at the entity level can result in corrupted or unwanted data getting persisted.
Common Mistake:
Skipping validation logic leads to poor data integrity and can create unexpected errors at the application level.
Solution:
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
@Entity
public class Customer {
@Id
private Long customerId;
@NotNull
@Size(min = 1, max = 100) // Ensures customerName is not empty and below 100 characters
private String customerName;
// Getters and setters
}
Integrating Bean Validation API with annotations like @NotNull
and @Size
can help maintain high data integrity within your entity classes and provide immediate feedback to developers.
6. Forgetting About Encapsulation and Access Modifiers
Why is Encapsulation Important?
Encapsulation is a core principle of Object-Oriented Programming. It promotes better structure, readability, and maintainability.
Common Mistake:
Exposing all fields directly via public access can lead to unintended modifications.
Solution:
@Entity
public class Employee {
@Id
private Long employeeId;
private String name;
// Private setter for encapsulation
private void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
Controlling access can help preserve invariants, ensuring fields are only modified in allowed ways, thus maintaining the integrity of the entity.
7. Inefficient Use of Relationships
Why Relationships Matter
Properly defined relationships can ensure data consistency and better performance. However, poor design can lead to complex queries and performance hits.
Common Mistake:
Overusing bidirectional relationships without necessity can complicate data retrieval and storage processes.
Solution:
@Entity
public class Category {
@Id
private Long categoryId;
@OneToMany(mappedBy = "category", cascade = CascadeType.ALL)
private List<Product> products;
// Getters and setters
}
@Entity
public class Product {
@Id
private Long productId;
@ManyToOne
private Category category;
// Getters and setters
}
Bidirectional relationships should only be used when absolutely necessary. Assess the need for traversing the relationship in both directions to keep things simple and maintainable.
The Bottom Line
Designing entity classes in Java EE involves several key considerations to avoid common pitfalls. From ensuring proper serialization and identifier management to implementing encapsulation and validation, each practice plays a crucial role in the overall health of your application.
Following these guidelines will not only improve your current development practices but will also have a lasting impact on maintainability and scalability in your Java EE projects. For more nuanced discussions on JPA and related best practices, consider exploring Java EE Best Practices.
By avoiding these pitfalls, you will build more reliable, efficient, and maintainable applications that can grow alongside the demands of your business. Happy coding!
Checkout our other articles