Understanding the Differences: API Gateway vs. Reverse Proxy vs. Load Balancer
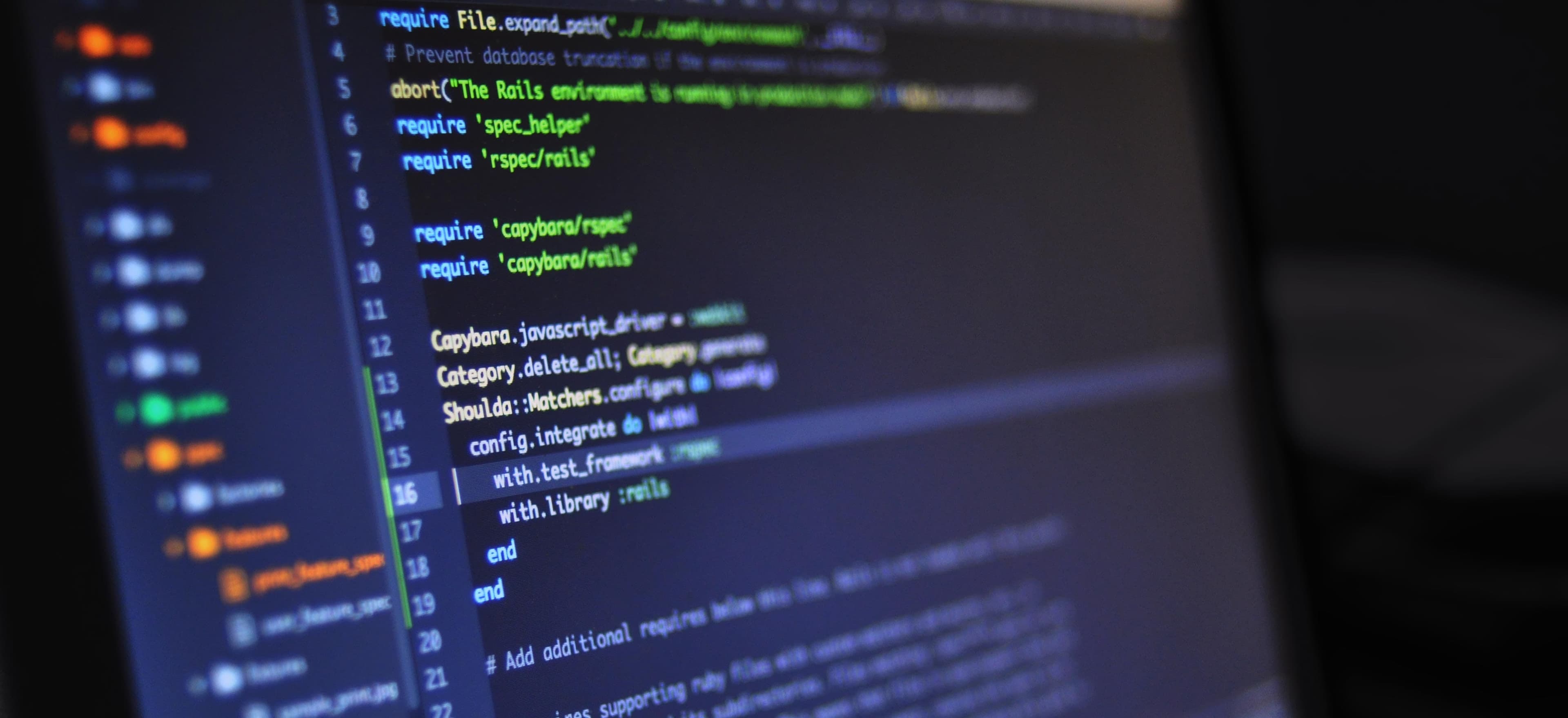
- Published on
Understanding the Differences: API Gateway vs. Reverse Proxy vs. Load Balancer
In the world of microservices, cloud architectures, and distributed systems, understanding the roles and responsibilities of various components is crucial. Among these components, the API Gateway, Reverse Proxy, and Load Balancer play significant roles in application performance and management. In this blog post, we'll explore the functions, use cases, and key differences between these essential architectural elements.
What is an API Gateway?
An API Gateway serves as a single entry point for clients to interact with various backend services. It acts as a mediator between client applications and microservices, providing features like request routing, authentication, and response aggregation.
Key Functions of an API Gateway:
- Request Routing: Directs client requests to the appropriate service.
- Authentication and Authorization: Validates user credentials and permissions.
- Rate Limiting: Controls the number of requests per user.
- Response Aggregation: Combines results from multiple services into a single response.
- Monitoring and Analytics: Tracks usage metrics and logs for analysis.
Example Code: Setting Up an API Gateway with Spring Cloud Gateway
import org.springframework.cloud.gateway.route.RouteLocator;
import org.springframework.cloud.gateway.route.builder.RouteLocatorBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class GatewayConfig {
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("path_route", r -> r.path("/api/v1/**")
.uri("http://backend-service:8080"))
.build();
}
}
Why Use This Code?: This Spring Cloud Gateway configuration sets up a route that directs all traffic from "/api/v1/**" to a backend service running on port 8080. This simplifies the architecture by centralizing routing logic.
What is a Reverse Proxy?
A Reverse Proxy is a server that sits between clients and one or multiple backend servers. It forwards client requests to the appropriate server based on various algorithms or configurations. A reverse proxy can cache content, provide SSL termination, and enhance security by hiding backend servers.
Key Functions of a Reverse Proxy:
- Traffic Distribution: Balances incoming requests among multiple servers.
- Caching: Stores copies of frequently requested resources to improve load times.
- SSL Termination: Handles encrypted traffic, reducing the workload on backend servers.
- Anonymity: Obscures the identity of backend servers.
Example Code: Setting Up a Reverse Proxy with NGINX
server {
listen 80;
location / {
proxy_pass http://backend-service:8080;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}
}
Why Use This Code?: This NGINX configuration forwards all incoming requests to a backend service. The included headers help preserve information about the original client, which can be useful for logging and analytics.
What is a Load Balancer?
A Load Balancer distributes network or application traffic across multiple servers. Its primary goal is to optimize resource use, maximize throughput, reduce response time, and prevent overload on any single server.
Key Functions of a Load Balancer:
- Traffic Distribution: Balances requests across multiple servers.
- Health Checking: Monitors backend servers for availability and performance.
- Session Persistence: Maintains session state with the same server.
- Application-Level Routing: Directs requests based on application logic.
Example Code: Setting Up a Load Balancer with AWS ELB
To create a Load Balancer on AWS, you would typically use their Management Console or AWS CLI. Below is an example of AWS CLI command to create a simple Application Load Balancer:
aws elbv2 create-load-balancer \
--name my-load-balancer \
--subnets subnet-12345abcde subnet-67890efg12 \
--security-groups sg-12345abcde
Why Use This Code?: This command sets up an Application Load Balancer on AWS, specifying the subnets and security groups to use. The load balancer can distribute incoming application traffic in a smart manner.
API Gateway vs. Reverse Proxy vs. Load Balancer: Key Differences
| Feature | API Gateway | Reverse Proxy | Load Balancer | |------------------------|-------------------------------------|-----------------------------------|-------------------------------------| | Purpose | Handle API requests & responses | Forward requests to backend services | Distribute traffic across servers | | Authentication | Yes | No | No | | Caching | Yes (optional) | Yes | No | | Monitoring & Analytics | Yes | Limited | Basic | | Request Routing | Yes | Limited | Yes | | SSL Termination | Yes | Yes | Yes |
Use Cases
- API Gateway: Ideal for microservices architecture where you need a centralized point for request management and security.
- Reverse Proxy: Useful for caching and SSL termination or when obfuscating backend services is necessary.
- Load Balancer: Essential for ensuring high availability and distributing loads across multiple servers.
Closing the Chapter
Understanding the key differences and functions of API Gateways, Reverse Proxies, and Load Balancers is crucial for architects and developers building contemporary applications. Each component plays a distinct role in improving performance, enhancing security, or managing application traffic.
Choosing the right solution will depend on your specific use case, existing infrastructure, and organizational needs. Leveraging combinations of these components can lead to more robust, scalable, and efficient architectures.
For further reading, you may want to check out API Gateway vs. Reverse Proxy vs. Load Balancer on AWS for deeper insights into how AWS approaches these architectures.
By understanding these elements, you empower your applications to handle more traffic and facilitate smoother interactions between clients and backend services. Happy coding!
Checkout our other articles