Overcoming Common Challenges in Test Infrastructure Setup
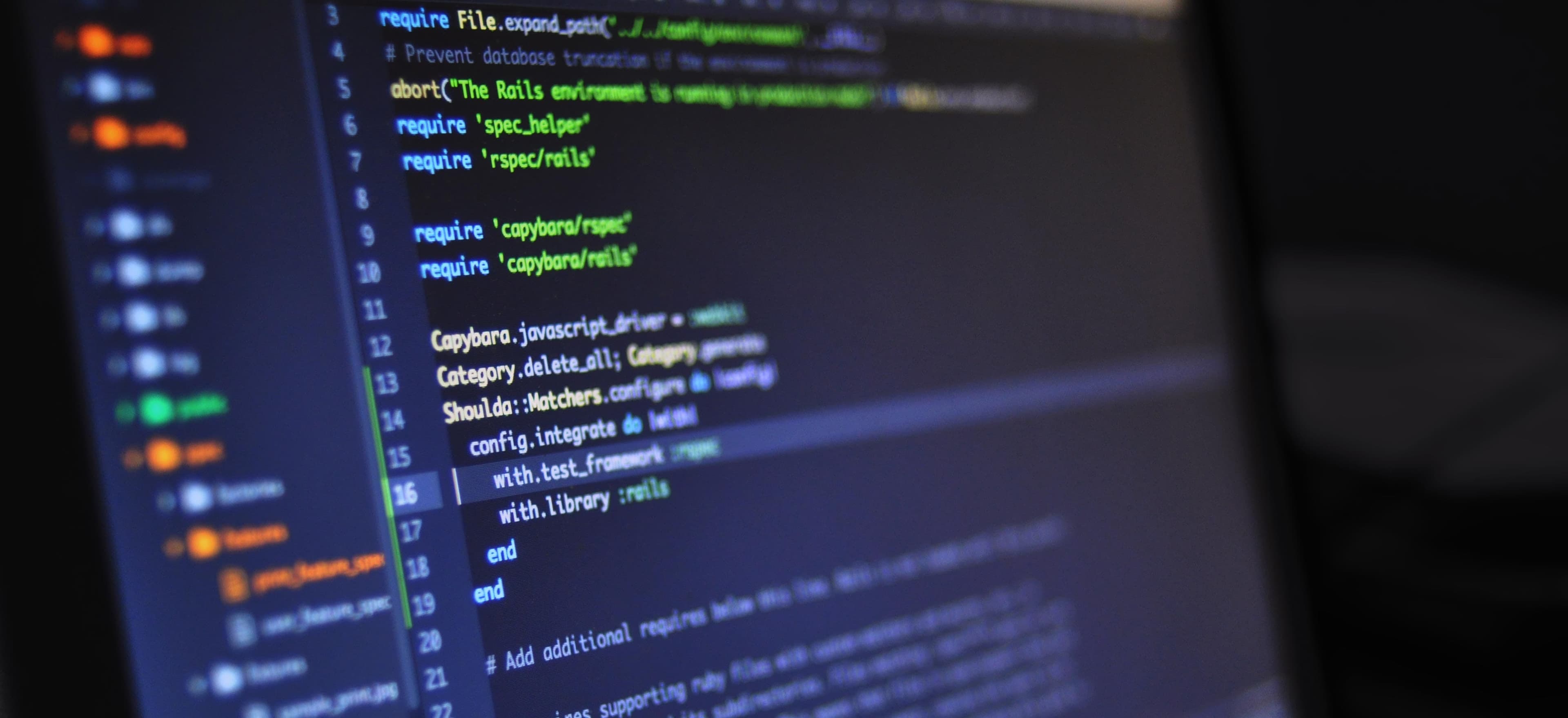
- Published on
Overcoming Common Challenges in Test Infrastructure Setup
Setting up a robust test infrastructure is essential for ensuring the quality of software products. With the exponential growth in software projects, having an efficient and scalable test environment has never been more crucial. However, many developers and teams encounter numerous challenges in establishing this infrastructure. This blog post will explore common hurdles in test infrastructure setup and provide practical solutions, particularly using Java-based tools and frameworks.
Understanding Test Infrastructure
Before we dive into the challenges, let's clarify what we mean by test infrastructure. It encompasses the hardware, software, and processes needed to conduct testing effectively. This includes everything from servers and databases to CI/CD pipelines and frameworks like JUnit or TestNG.
The Importance of Test Infrastructure
By having a solid test infrastructure:
- Quality Assurance: Ensures defects are identified early in the development cycle.
- Faster Feedback: Allows for rapid iteration and quicker responses to issues.
- Enhanced Collaboration: Facilitates teamwork across development and QA teams.
Common Challenges in Test Infrastructure Setup
-
Tool Selection
Choosing the right tools can be daunting. The Java ecosystem boasts various testing frameworks like JUnit, TestNG, and others. Each framework has its strengths and weaknesses.
Solution: Assess your project's specific needs. For example, if you're developing a simple application, JUnit might be sufficient. For more complex scenarios requiring parallel test execution, consider TestNG.
// Example of a simple JUnit test import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.assertEquals; public class SimpleMathTest { @Test void testAddition() { assertEquals(2, 1 + 1, "1 + 1 should equal 2"); } }
This test uses JUnit's
@Test
annotation to define a test method that checks the addition operation. Easy to read and maintain. -
Environment Configuration
Configuring the test environment correctly is often challenging. You might have to replicate the production environment, leading to possible discrepancies.
Solution: Use containerization tools like Docker. They allow you to create isolated environments easily.
FROM openjdk:11 COPY ./myapp /usr/src/myapp WORKDIR /usr/src/myapp RUN javac Main.java CMD ["java", "Main"]
This
Dockerfile
sets up a Java environment with OpenJDK 11, compiles a Java file namedMain.java
, and runs it. Containerization helps ensure consistency across environments. -
Data Management
Managing test data can be laborious. You need data that is representative of real scenarios without exposing sensitive information.
Solution: Integrate tools like DbUnit or create utility scripts to load and reset your database state before every test run.
// Example of using DbUnit to load data DatabaseOperation.CLEAN_INSERT.execute(databaseTester.getConnection(), dataSet);
This line utilizes DbUnit to perform a clean insert operation, ensuring that only the specified test data exists before tests run.
-
Test Automation Initiatives
Automation is key in modern testing, but integrating automated tests can be complex.
Solution: Start with your unit tests. They are typically easier to automate and offer critical feedback on your code. Use frameworks like JUnit and CI/CD tools like Jenkins or GitHub Actions to automate the testing process.
# Example GitHub Actions workflow for Java Testing name: Java CI on: [push] jobs: build: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - name: Set up JDK 11 uses: actions/setup-java@v1 with: java-version: '11' - name: Build with Maven run: mvn install
This YAML configuration file sets up a Java Continuous Integration workflow using GitHub Actions. It checks out the code, configures the JDK, and runs the Maven build.
-
Collaboration and Communication
Often, teams working on different parts of a project use different frameworks or testing methodologies, leading to inconsistencies.
Solution: Establish testing guidelines and regular meetings to ensure everyone is on the same page. Encourage the team to share their experiences and solutions.
Best Practices for Test Infrastructure Setup
- Centralize Testing Tools: Use a common repository for all testing tools and frameworks.
- Create Documentation: Document processes, tools, and configurations. It aids onboarding new team members.
- Monitor and Measure: Regularly track test results and the performance of your test infrastructure to identify bottlenecks.
- Continuous Learning: Technology evolves; make sure your team is keeping up with the latest trends and tools in testing.
Closing Remarks
Setting up test infrastructure in Java is filled with challenges. However, with the right approach—tool selection, environment configuration, effective data management, automation, and collaboration—these challenges can be effectively mitigated.
Investing in a robust test infrastructure leads to improved product quality, faster release cycles, and ultimately higher customer satisfaction. As technology continues to evolve, so too should our strategies to adapt to these changes and overcome future hurdles.
Additional Resources
By understanding these common challenges and applying the solutions provided, you can begin to build a test infrastructure that not only meets your current needs but is also scalable for future growth. Happy testing!
Checkout our other articles