Common Cross-Browser Testing Pitfalls to Avoid
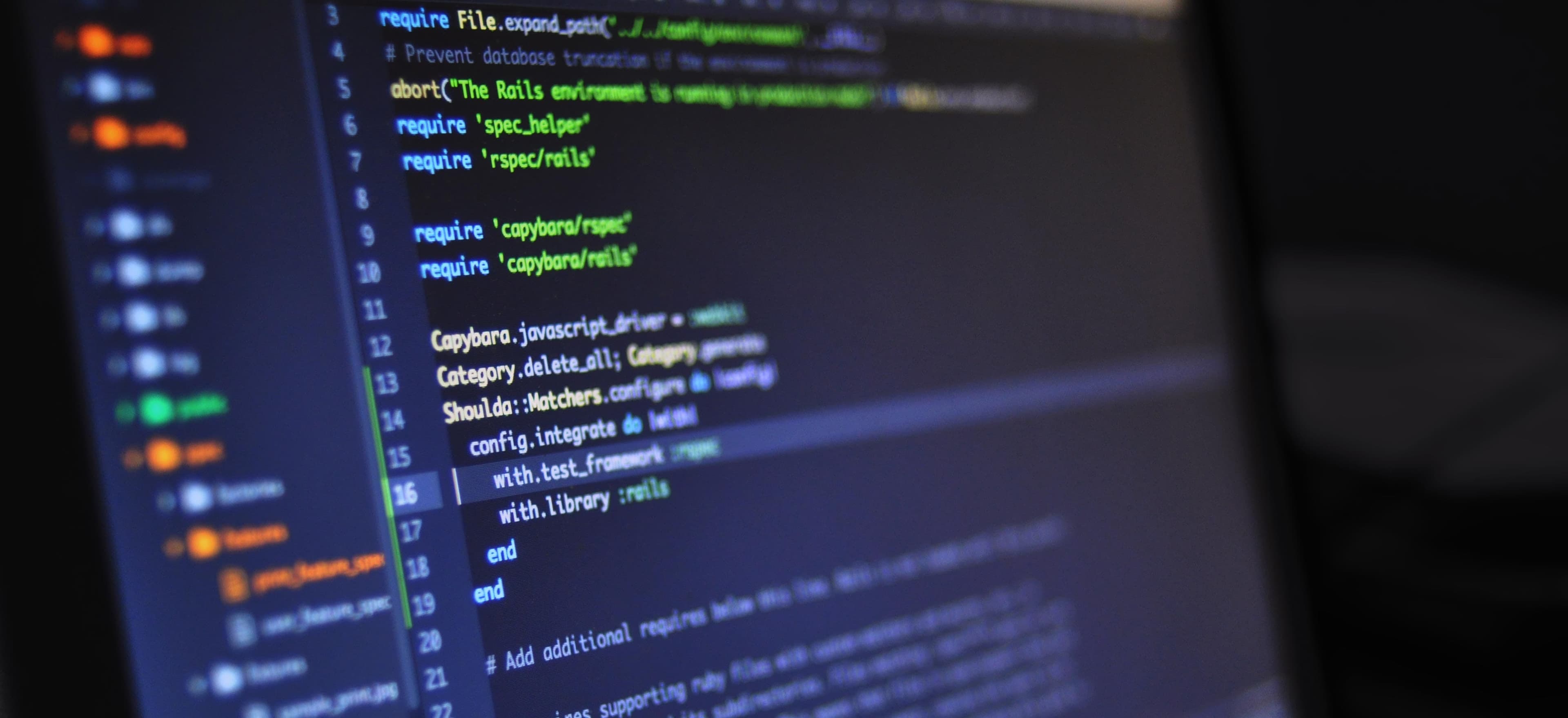
- Published on
Common Cross-Browser Testing Pitfalls to Avoid
Cross-browser testing has become an essential part of web development. As a developer, ensuring your website or web application performs consistently and correctly across various browsers is crucial for providing a seamless user experience. However, many developers encounter common pitfalls in cross-browser testing that can hinder their effectiveness.
In this post, we'll explore key pitfalls to avoid during cross-browser testing. We'll also provide actionable tips and relevant code snippets to help you improve your testing strategy.
1. Ignoring Browser Compatibility
Why It Matters
One of the most significant mistakes developers make is relying too heavily on modern browsers like Chrome or Safari. This oversight can alienate users on other platforms, like Firefox, Internet Explorer, or Edge.
Solution
Always validate your designs against the most commonly used browsers. Use tools such as BrowserStack or Sauce Labs to test across a range of browsers and devices.
Example Scenario
Let's say you designed a page using CSS Grid, which has excellent support in modern browsers but less so in older ones.
.container {
display: grid;
grid-template-columns: repeat(3, 1fr);
}
Why: User agents like IE11 may not support CSS Grid. In this case, consider using a fallback layout or CSS Flexbox to ensure all users can view your site properly.
2. Not Considering Different Screen Resolutions
Why It Matters
Users browse the web on various devices, from mobile phones to large desktop screens. Failing to account for this diversity can lead to unexpected layout issues.
Solution
Utilize CSS media queries and flexible layouts to ensure your application responds elegantly to different screen sizes.
Code Snippet
@media (max-width: 768px) {
.container {
display: block;
}
}
Why: This code snippet will change the layout from a grid to a single column whenever the screen width is less than 768 pixels. This approach allows you to provide an optimal viewing experience for mobile users.
3. Overlooking Browser-Specific Bugs
Why It Matters
Different browsers may interpret HTML and CSS differently. Not acknowledging this can lead to inconsistent behavior and layout issues.
Solution
Test edge cases specific to each browser. Use feature detection libraries like Modernizr to gracefully handle these discrepancies.
Example Scenario
Consider the use of Web Fonts. Some browsers might struggle with rendering them correctly.
@font-face {
font-family: 'OpenSans';
src: url('OpenSans-Regular.woff2') format('woff2');
}
Why: Browsers like Safari can have issues with specific font formats. It is essential to provide fallbacks or to conditionally load different font formats based on browser support.
4. Misunderstanding JavaScript Compatibility
Why It Matters
JavaScript features can differ significantly across browsers. Developers may rely on the latest ECMAScript (ES6+) features without realizing that not all users have up-to-date browsers.
Solution
Use tools like Babel to transpile your JS code to ensure compatibility across older browsers.
Code Snippet
const greetUser = (name = "Guest") => {
console.log(`Hello, ${name}!`);
};
greetUser("Alice");
Why: The arrow function and default parameter syntax used here may not work in all browsers. Transpiling your code allows you to use modern syntax while maintaining support for older browsers.
5. Neglecting Testing Interactivity
Why It Matters
Interactive elements such as buttons and forms may behave differently across browsers, leading to user frustration if these issues go unnoticed.
Solution
Thoroughly test all interactive components. Use the W3C Markup Validation Service to check for any HTML or JavaScript errors that could affect functionality.
Example Scenario
Consider a simple button click event:
<button id="submitBtn">Submit</button>
<script>
document.getElementById('submitBtn').addEventListener('click', () => {
alert('Button Clicked');
});
</script>
Why: Some older versions of Safari might not recognize the addEventListener method. Always make sure to check compatibility against their Browser Compatibility Table
6. Crawling Through Console Errors
Why It Matters
Ignoring console errors can lead to undiagnosed issues across browsers. Many browsers have their own error handling and logging systems that might display broken functionality.
Solution
Regularly examine the console during testing and be proactive about addressing any issues. Tools like Sentry can help you track down these console errors in production.
Example Scenario
Let's say you are using a third-party library:
<script src="https://cdn.example.com/library.js"></script>
Why: If the CDN is down or serving an outdated version of the library, errors will likely show in the console across browsers. Always have fallback logic or local versions available to mitigate this risk.
7. Insufficient Automation Testing
Why It Matters
Manual testing can be time-consuming and might not cover all scenarios and browsers. Relying solely on manual tests can result in missed bugs across different platforms.
Solution
Incorporate automated testing tools such as Selenium or Cypress in your development workflow.
Code Snippet
Here's a basic Selenium test in Java:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class CrossBrowserTest {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.get("http://yourwebsite.com");
String pageTitle = driver.getTitle();
System.out.println("Page title is: " + pageTitle);
driver.quit();
}
}
Why: The above code initializes a ChromeDriver instance to test page title rendering. By scaling this approach, you can automate tests across multiple browsers and screen resolutions.
The Bottom Line
Avoiding these common cross-browser testing pitfalls can dramatically improve your web application's reliability and user experience. By considering browser compatibility, different screen resolutions, browser-specific bugs, and automating tests, you can save yourself a lot of headaches down the line.
No testing process is perfect, but being aware of these pitfalls will prepare you to tackle them head-on. For more insights, you can explore articles on Effective Cross-Browser Testing.
By integrating the best practices mentioned in this post, you can ensure that your web applications look and function beautifully across all platforms. Happy testing!
Checkout our other articles