Overcoming Common Mistakes in Java's Template Method Pattern
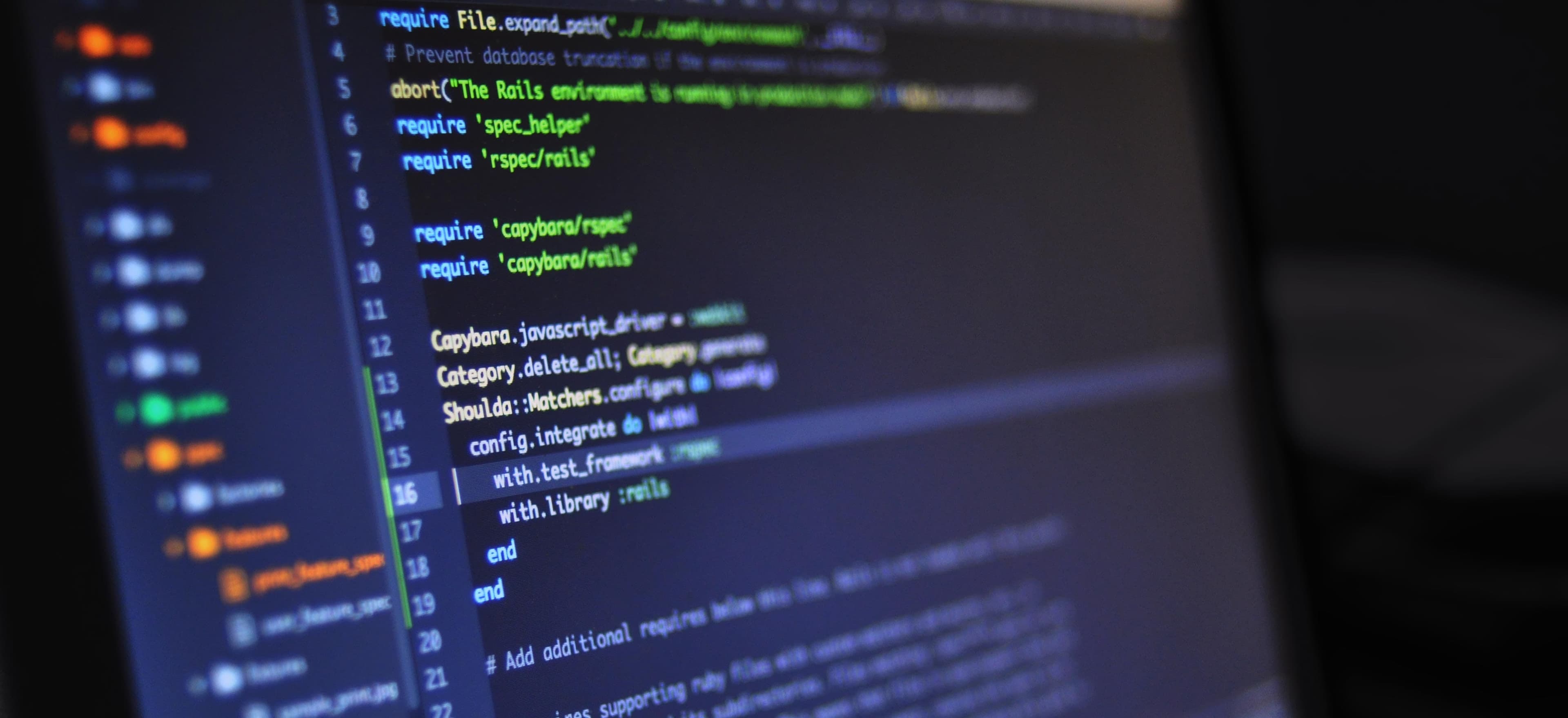
- Published on
Overcoming Common Mistakes in Java's Template Method Pattern
The Template Method Pattern is a fundamental design pattern in software engineering, particularly embraced in object-oriented programming. It is a behavioral pattern that defines the skeleton of an algorithm in a method, deferring some steps to subclasses. This allows subclasses to redefine certain steps of an algorithm without changing the algorithm's structure.
In this blog post, we'll explore some common mistakes developers often make when using the Template Method Pattern in Java, discuss how to avoid them, and share best practices for implementing this design pattern effectively.
Understanding the Template Method Pattern
Before diving into the mistakes, let’s ensure we grasp the core concept of the Template Method Pattern. The pattern involves creating a base class that defines a sequence of steps in an algorithm (the template method), while allowing subclasses to modify specific parts of the sequence.
Here's a simple illustration:
abstract class DataProcessor {
// Template Method
public void process() {
readData();
processData();
saveData();
}
protected abstract void readData();
protected abstract void processData();
protected abstract void saveData();
}
In this example, DataProcessor
has a defined method process()
that dictates the order of operations while delegating the actual implementation to subclasses. This separation of concerns is key to effective code organization and reuse.
Common Mistakes and Solutions
1. Overcomplicating the Base Class
One frequent mistake is overcomplicating the base class by including too much logic. The base class should only focus on the broader flow of the algorithm.
Solution: Keep the base class simple. Use the abstract methods to delegate specific behaviors to subclasses.
Example:
class CsvDataProcessor extends DataProcessor {
@Override
protected void readData() {
// Logic to read CSV data
System.out.println("Reading data from CSV file...");
}
@Override
protected void processData() {
// Logic to process the CSV data
System.out.println("Processing CSV data...");
}
@Override
protected void saveData() {
// Logic to save processed data
System.out.println("Saving processed data...");
}
}
In this example, CsvDataProcessor
implements all required methods while keeping its logic focused and contained.
2. Not Utilizing Hooks
The Template Method Pattern allows for the use of "hooks"—optional methods that can be defined in the abstract class. Failing to use hooks can lead to code duplication across subclasses.
Solution: Implement hook methods when necessary, allowing subclasses to extend functionality without changing the template method.
Example:
abstract class DataProcessorWithHooks {
public void process() {
if (isDataRequired()) {
readData();
}
processData();
saveData();
}
protected boolean isDataRequired() {
return true; // Default implementation
}
protected abstract void readData();
protected abstract void processData();
protected abstract void saveData();
}
class ConditionalDataProcessor extends DataProcessorWithHooks {
@Override
protected boolean isDataRequired() {
return false; // Skips reading data
}
@Override
protected void readData() {
// This method may never be called
}
// Other implementations remain the same
}
In this case, ConditionalDataProcessor
can change the behavior of process()
by overriding isDataRequired()
.
3. Ignoring Single Responsibility Principle
It's tempting to cram multiple unrelated functionalities into a single template method. This violates the Single Responsibility Principle (SRP) leading to harder-to-maintain code.
Solution: Keep your template methods focused on a single task or responsibility.
Example:
abstract class ReportGenerator {
public void generateReport() {
gatherData();
formatReport();
printReport();
}
protected abstract void gatherData();
protected void formatReport() {
System.out.println("Formatting report...");
}
protected abstract void printReport();
}
By separating concerns, it becomes easier to maintain and extend functionality. For instance, by simply overriding formatReport()
in a subclass, you can add customization without modifying the existing methods.
4. Poor Naming Conventions
Using vague names or abbreviations for classes and methods can lead to confusion. Clear, descriptive naming enhances readability and maintainability.
Solution: Adopt consistent and meaningful naming conventions.
Example:
class SalesReportGenerator extends ReportGenerator {
@Override
protected void gatherData() {
System.out.println("Gathering sales data...");
}
@Override
protected void printReport() {
System.out.println("Printing sales report...");
}
}
A class name like SalesReportGenerator
immediately conveys its purpose. Well-named methods and classes make it intuitive for other developers to understand the code.
Best Practices
-
Prefer Composition Over Inheritance: While inheritance is central in the Template Method Pattern, consider flavored strategies using composition when applicable. This often results in more flexible designs.
-
Use Abstract Classes for Template Methods: Abstract classes serve as a better foundation for your base class compared to interfaces in the context of template methods, owing to their ability to define behavior and state.
-
Testing Template Methods: Ensure you adequately test your Template Methods, especially for the various subclasses. Each subclass should be treated as a unit of functionality.
-
Avoid Statefulness: Template Method Pattern should ideally be designed to be stateless to avoid side-effects. Manage state through subclass instances to avoid unexpected behavior.
-
Document Your Template: Provide adequate comments within the base class to explain steps in the algorithm. This becomes especially important in larger applications.
My Closing Thoughts on the Matter
The Template Method Pattern offers a structured way to design algorithms in Java. However, it is essential to avoid common pitfalls that can lead to convoluted designs and poor maintainability. By focusing on clear and concise implementations, utilizing hooks where necessary, and adhering to principles like SRP, you can leverage this powerful design pattern more effectively.
For more insights on design patterns in Java, you can visit Refactoring Guru or explore the Java Design Patterns reference for a broader understanding.
Happy coding!
Checkout our other articles