Mastering Callable and FutureTask: Common Pitfalls Revealed
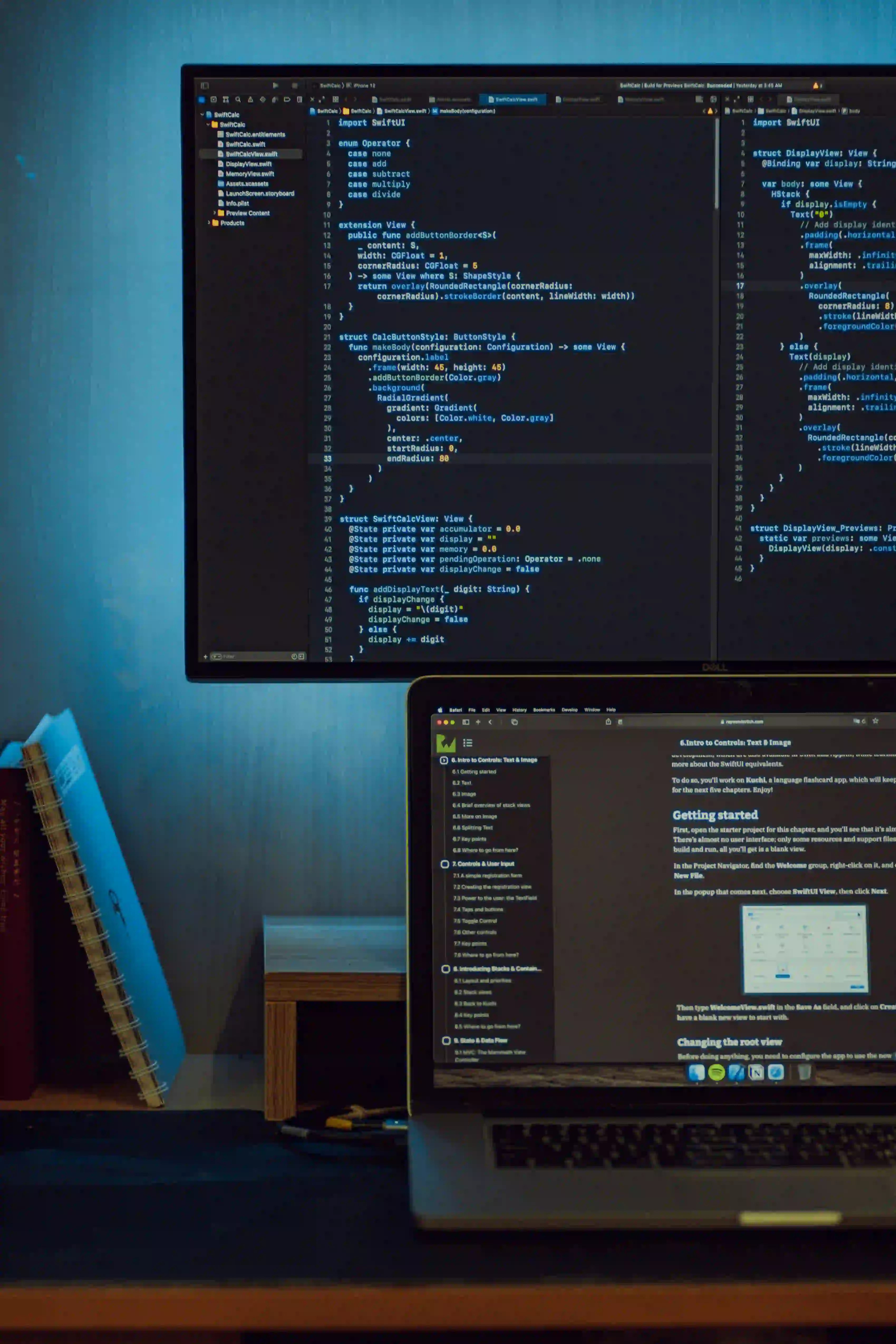
Mastering Callable and FutureTask: Common Pitfalls Revealed
Java’s concurrency framework provides powerful tools, allowing developers to execute tasks asynchronously, manage threads, and improve application performance. Two of the core components of this framework are Callable
and FutureTask
. Understanding them thoroughly not only boosts performance but also helps avoid common pitfalls. In this blog post, we will explore these components, elucidate their differences with practical examples, and expose common traps developers may encounter.
Understanding Callable
A Callable
is a functional interface that supports task execution. Unlike a standard Runnable
, which returns void, a Callable
can return a result and may throw a checked exception.
Example of Callable
Here’s a simple example of a Callable
implementation:
import java.util.concurrent.Callable;
public class MyCallable implements Callable<Integer> {
@Override
public Integer call() throws Exception {
return 123; // Returning an integer result
}
}
In this code, the call
method returns an integer. In this context, implementing a Callable
allows us to specify a task and expect a result after its execution.
FutureTask Explained
A FutureTask
is one of the notable classes that can wrap a Callable
. It provides a method to check if the task is completed, retrieve the result, and also cancels the task if necessary.
Example of FutureTask
Here’s how you might use a FutureTask
:
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.FutureTask;
public class FutureTaskExample {
public static void main(String[] args) {
Callable<Integer> callableTask = new MyCallable();
FutureTask<Integer> futureTask = new FutureTask<>(callableTask);
Thread thread = new Thread(futureTask);
thread.start();
try {
Integer result = futureTask.get(); // Blocks until the result is available
System.out.println("Result from Callable: " + result);
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
}
}
In this example, we created a new thread to run the FutureTask
. The get()
method retrieves the result or throws an exception if the task failed.
Differences Between Callable and Runnable
- Return Type: A
Callable
returns a value, but aRunnable
does not. - Exception Handling: A
Callable
can throw checked exceptions, which makes it versatile compared toRunnable
.
For more information on concurrency in Java, consider checking out the Java Concurrency Tutorials.
Common Pitfalls When Using Callable and FutureTask
Understanding Callable
and FutureTask
is one thing, but properly leveraging them in concurrent applications is where developers can stumble. Let’s explore several common pitfalls and how to avoid them.
Pitfall 1: Not Handling InterruptedException
Developers may fail to address the InterruptedException
when calling get()
on the FutureTask
. Interrupted exceptions can arise when other threads interrupt the task execution.
How to Handle
Always listen for interruptions and handle them gracefully:
try {
Integer result = futureTask.get();
} catch (InterruptedException e) {
// Restore interrupted status
Thread.currentThread().interrupt();
System.out.println("Task was interrupted!");
} catch (ExecutionException e) {
// Handle exceptions thrown in the callable task
System.out.println("Task failed with exception: " + e.getCause());
}
Pitfall 2: Forgetting to Start the FutureTask
It’s possible to create a FutureTask
but forget to start it in a new thread. The task will, therefore, not execute, leading to an indefinite wait when calling get()
.
How to Avoid This
Always ensure the FutureTask
is wrapped in a thread and started:
Thread thread = new Thread(futureTask);
thread.start(); // Remember to start the thread!
Pitfall 3: Dangling FutureTask Reference
If you’re not careful about holding references to your FutureTask
, you can inadvertently create memory leaks.
How to Mitigate
Once the result has been retrieved, allow the FutureTask
reference to go out of scope, or explicitly set it to null:
Integer result = futureTask.get();
futureTask = null; // Help garbage collection
Pitfall 4: Calling get() Multiple Times
Calling get()
on a FutureTask
multiple times can also lead to unexpected behavior. Once the task is completed, subsequent calls to get()
return the same result.
How to Prevent
Use a local variable to store the result instead of allowing multiple accesses:
Integer result;
try {
result = futureTask.get();
} catch (Exception ex) {
// Handle exception
}
// Use 'result' without calling 'futureTask.get()' again.
Pitfall 5: Failing to Cancel a Task
If you wish to cancel an ongoing task, simply asking for the result doesn't stop it. You must call cancel()
.
Cancelling a FutureTask
boolean canceled = futureTask.cancel(true); // true interrupts if running
if (canceled) {
System.out.println("The task was canceled.");
}
Best Practices
- Always Check for Interruptions: Your tasks should regularly check for interruptions.
- Exception Handling: Always handle checked exceptions when using
Callable
. - Thread Management: Properly manage threads, ensuring they are started and terminated correctly.
- Use ExecutorService: For better task management, consider using
ExecutorService
instead of manually handling threads.
Bringing It All Together
Mastering Callable
and FutureTask
is crucial for crafting efficient, responsive Java applications. By avoiding the pitfalls discussed, you can harness the full power of Java's concurrency features.
If you're looking to further explore Java concurrency, refer to Java Concurrency in Practice by Brian Goetz. This book provides comprehensive insights and illustrates best practices in developing concurrent applications.
Getting Started
Begin implementing your own Callable
and FutureTask
, and check for these common mistakes during development. Embrace the challenge of concurrent programming, and you'll see your Java applications thrive with new levels of performance!