Solving MongoDB's Optimistic Locking Retry Challenges
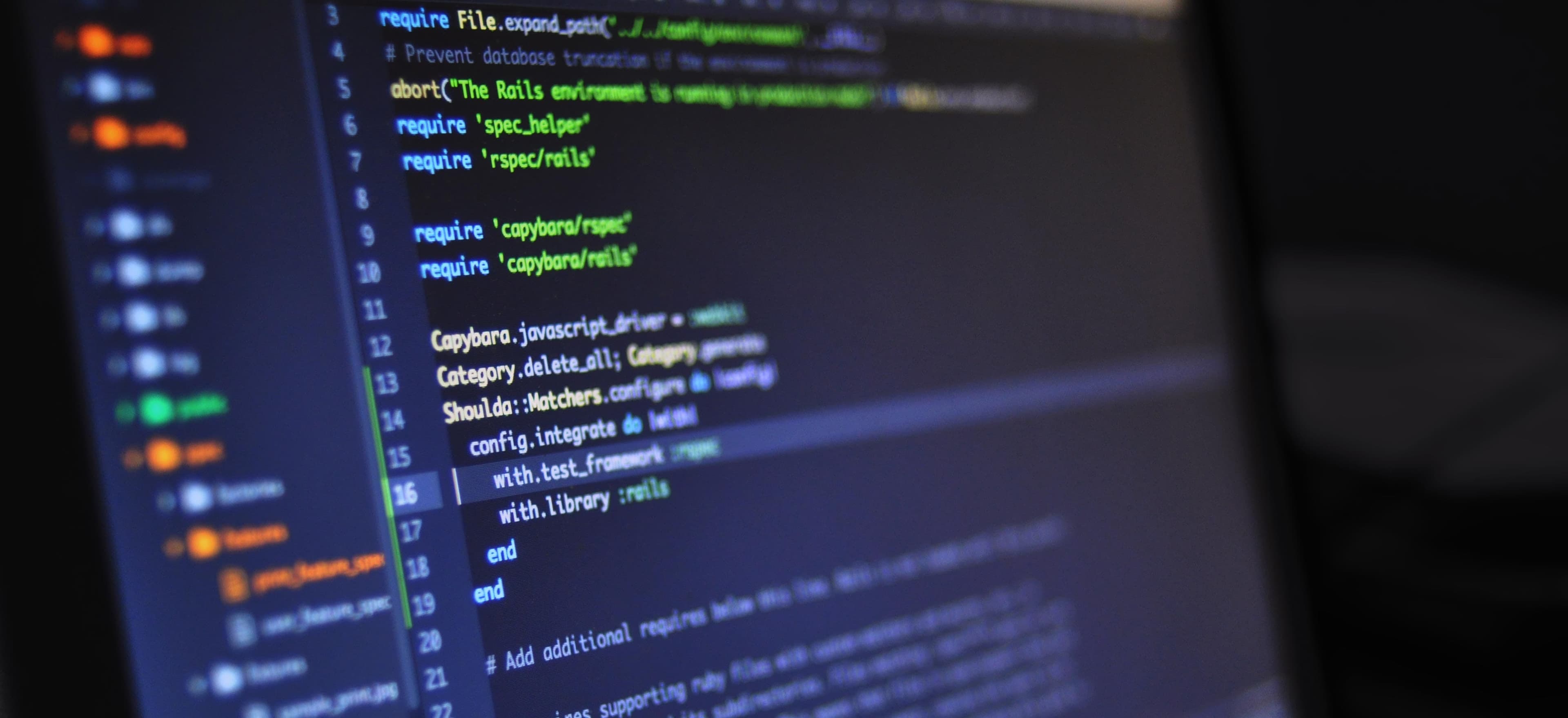
- Published on
Solving MongoDB's Optimistic Locking Retry Challenges
Optimistic locking is a significant pattern in applications that are required to update data concurrently while maintaining data integrity. Although MongoDB supports some functionality for optimistic locking, it can present challenges, especially during retry operations. In this article, we will explore what optimistic locking is, why it presents difficulties, and how to solve MongoDB's optimistic locking retry challenges through effective patterns and code implementations.
What is Optimistic Locking?
Optimistic locking is a concurrency control mechanism that assumes multiple transactions can complete without affecting each other. Unlike pessimistic locking, which locks the data before making changes, optimistic locking lets transactions proceed without restrictions and checks for conflicts before completing an update.
In the case of MongoDB, optimistic locking is often implemented using a document versioning system. Each document maintains a version field that is incremented every time an update occurs. When attempting to perform an update, the application checks if the version number matches the expected value. If it does, the update proceeds, and the version number is incremented. If not, a conflict is detected, and the update fails.
Why Use Optimistic Locking?
Opting for optimistic locking offers several benefits:
- Increased Concurrency: Since pessimistic locking blocks other operations until a transaction is complete, optimistic locking allows more transactions to proceed concurrently.
- Reduced Locking Overhead: Eliminates the need for locking mechanisms that can lead to deadlock situations and performance bottlenecks.
However, optimistic locking isn't without its challenges, most notably during retry operations.
The Challenges of Optimistic Locking in MongoDB
-
Retry Logic Complexity: When a version conflict occurs, implementing a retry mechanism can become complicated. The application must decide whether to retry the operation, retrieve the latest state, or inform the user about the conflict.
-
Data Staleness: If you simply retry the operation without refreshing the data, you risk working with stale data, leading to potential loss of information or unintended consequences.
-
Versioning Management: Properly managing version numbers across concurrent operations can be cumbersome, especially as scalability increases.
Implementing Optimistic Locking in MongoDB
Let's delve into how to effectively implement optimistic locking in MongoDB, keeping in mind the retry challenges highlighted.
Step 1: Document Structure
First, you'll want to add a version field to your document schema. Here's an example using Mongoose, a popular ODM (Object Document Mapping) for MongoDB:
const mongoose = require('mongoose');
const userSchema = new mongoose.Schema({
name: { type: String, required: true },
age: { type: Number, required: true },
version: { type: Number, default: 0 } // Added version field for optimistic locking
});
const User = mongoose.model('User', userSchema);
Step 2: Performing Updates with Version Check
When updating a document, you can use the following code. This ensures that the version field has not changed since the document was retrieved.
async function updateUser(userId, updatedData) {
const session = await mongoose.startSession();
session.startTransaction();
try {
// Step 1: Retrieve the user
const user = await User.findById(userId).session(session);
if (!user) {
throw new Error('User not found');
}
// Step 2: Check the version
const version = user.version;
const versionedUpdate = await User.findOneAndUpdate(
{ _id: userId, version: version },
{ ...updatedData, version: version + 1 },
{ new: true, session }
);
// Step 3: If the update was successful, commit the transaction
if (!versionedUpdate) {
throw new Error('Version mismatch detected. Update failed.');
}
await session.commitTransaction();
return versionedUpdate;
} catch (error) {
await session.abortTransaction();
throw error;
} finally {
session.endSession();
}
}
Explanation of the Code:
-
Starting a Session: This begins a new session for the transaction, ensuring that all operations are automatically rollback-able in the event of an error.
-
Retrieving the User: Before making updates, we must first retrieve the user. This allows us to capture the current version.
-
Version Check and Update: The
findOneAndUpdate
method checks both the user's ID and the current version before applying the update. If a version mismatch occurs, the operation will not succeed. -
Transaction Handling: If the update is successful, the transaction is committed. Any errors cause the transaction to abort, keeping the database state consistent.
Step 3: Implementing Retry Logic
When a version conflict is detected, you may want to implement a retry strategy. Here’s an example using a simple back-off mechanism:
async function updateUserWithRetry(userId, updatedData, maxRetries = 5) {
for (let attempt = 0; attempt < maxRetries; attempt++) {
try {
return await updateUser(userId, updatedData);
} catch (error) {
if (error.message.includes('Version mismatch detected')) {
// Optional: Delay before retrying
console.log(`Attempt ${attempt + 1} failed. Retrying...`);
await new Promise(res => setTimeout(res, 100 * Math.pow(2, attempt))); // Exponential backoff
} else {
throw error; // Pass non-retriable error up the stack
}
}
}
throw new Error('Max retries exceeded.');
}
Explanation of the Retry Logic:
-
Looping Attempts: The function attempts to update the user document a defined number of times.
-
Error Handling: If a version mismatch error occurs, we wait before retrying (using exponential back-off) to allow for potential data convergence among concurrent operations.
-
Final Error Throwing: If all attempts fail, an error is thrown to inform the caller.
Key Takeaways
Optimistic locking can significantly enhance the performance and scalability of applications using MongoDB. However, it can introduce challenges, particularly surrounding retry logic and data integrity.
By structuring your documents wisely, implementing robust update methods with version checks, and carefully managing retries, you can mitigate these challenges effectively. Always be aware of the complexity brought on by concurrent data access, and ensure your application gracefully handles these scenarios.
For further reading on MongoDB and optimistic locking, consider checking out the official MongoDB documentation and Mongoose documentation.
With a thoughtful approach to optimistic locking, your applications can strike a balance between data integrity and performance, providing a better user experience.