Troubleshooting URL Activation: Common Argument Issues
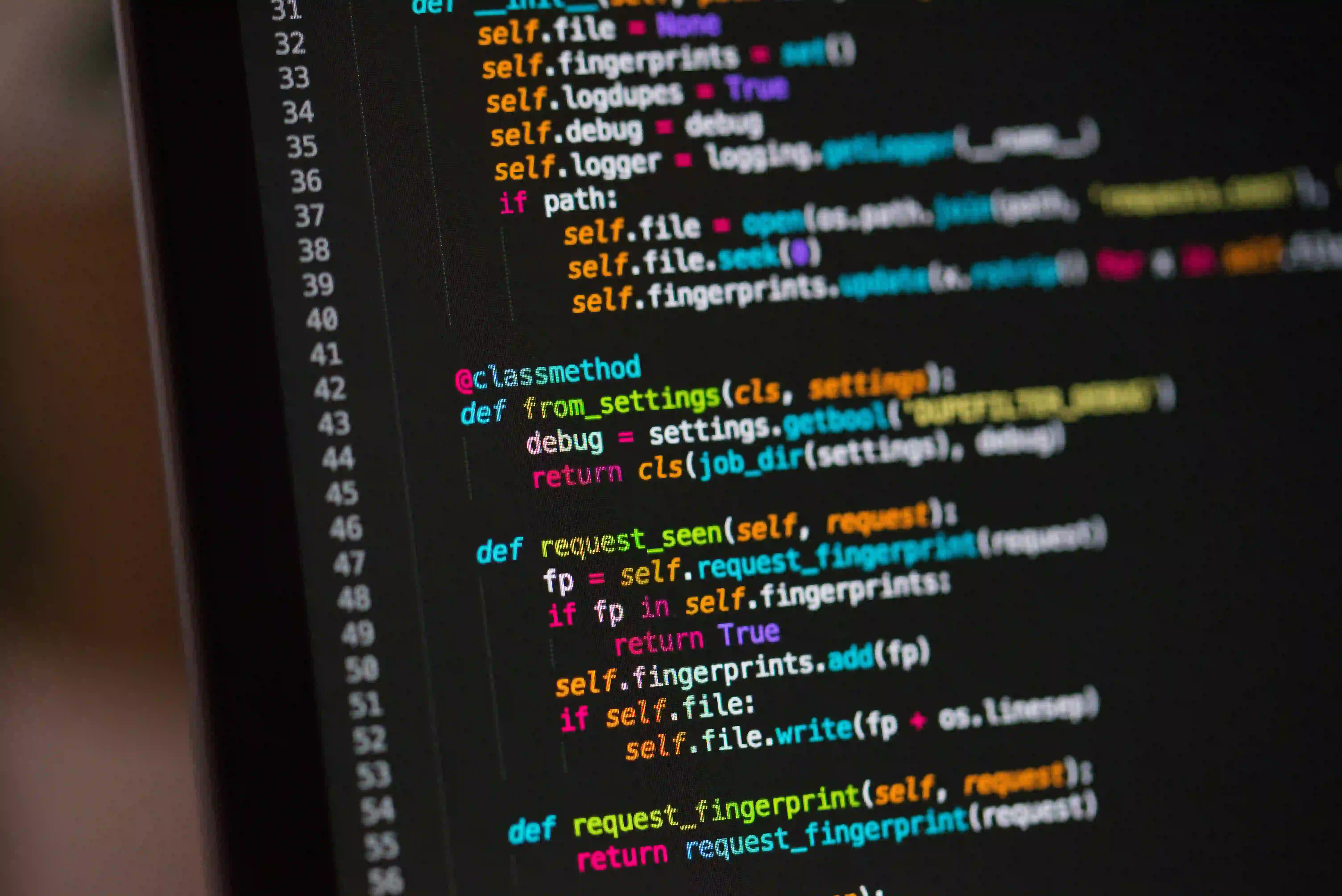
Troubleshooting URL Activation: Common Argument Issues in Java
In the world of web applications, the ability to activate URLs dynamically is a common requirement. Whether it is for an API call, redirecting a user after login, or any other function that involves URLs, understanding how to troubleshoot issues related to URL activation is essential. In this blog post, we will explore common argument issues that can arise when working with URLs in Java, and how to address them effectively.
Understanding URL Activation
Before diving into troubleshooting, let’s clarify what URL activation means in the context of Java. URL activation typically refers to the process of handling, constructing, and invoking specific URLs from your code. This can involve managing query parameters, path variables, or even headers based on what your application requires.
Common Issues While Activating URLs
When working with URLs, several issues might arise due to incorrect parameters. Here are some of the most common problems along with their solutions:
1. Malformed URLs
One of the most frequent issues developers face is working with malformed URLs. If a URL is not correctly formed, it will lead to exceptions during execution.
Example:
import java.net.URI;
import java.net.URISyntaxException;
public class URLExample {
public static void main(String[] args) {
try {
String url = "http://example.com/somepath"; // Correct URL structure
URI uri = new URI(url);
System.out.println("Valid URI: " + uri.toString());
} catch (URISyntaxException e) {
System.out.println("Malformed URL: " + e.getMessage());
}
}
}
Why this matters: A malformed URL literally cannot be interpreted as a valid resource on the internet. Using URI
helps to catch this error early.
2. Missing or Incorrect Query Parameters
When dynamically constructing URLs, it’s common to append query parameters. Missing, extra, or incorrectly formatted parameters can lead to failure while fetching the resource.
Example:
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
public class URLWithParams {
public static void main(String[] args) {
try {
String baseUrl = "http://example.com/api";
String paramName = "search";
String paramValue = "Java programming";
String encodedParam = URLEncoder.encode(paramValue, "UTF-8");
String fullUrl = baseUrl + "?" + paramName + "=" + encodedParam;
System.out.println("Full URL: " + fullUrl);
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
}
}
Why this matters: Encoding the parameters correctly is crucial for readable URLs. Special characters can break URLs and lead to incorrect requests.
3. Handling Special Characters
Special characters such as spaces, ampersands, or slashes must be encoded when creating URLs. Neglecting this can lead to navigation errors.
Example:
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
public class SpecialCharacters {
public static void main(String[] args) {
try {
String baseUrl = "http://example.com/submit";
String unicodeString = "Hello World! Special Characters: #&=+";
String encodedString = URLEncoder.encode(unicodeString, "UTF-8");
String fullUrl = baseUrl + "?data=" + encodedString;
System.out.println("Encoded URL: " + fullUrl);
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
}
}
Why this matters: The improper handling of special characters can break URL processing; thus, using URLEncoder
ensures that URLs are safe and valid.
4. HTTP and HTTPS Mismatch
Another issue developers encounter is the use of mismatched protocols. If your application sends a request to an HTTPS resource using HTTP, it may get blocked by security protocols.
Example:
import java.net.HttpURLConnection;
import java.net.URL;
public class ProtocolMismatch {
public static void main(String[] args) {
String url = "http://example.com/api"; // Should be HTTPS
try {
HttpURLConnection connection = (HttpURLConnection) new URL(url).openConnection();
System.out.println("Response Code: " + connection.getResponseCode());
} catch (Exception e) {
System.out.println("Exception while connecting: " + e.getMessage());
}
}
}
Why this matters: The HTTP/HTTPS protocol mismatch may block requests, so it’s crucial to ensure that your URL aligns with the server's expected protocols. Always prefer HTTPS for security and assurance of data integrity.
5. Managing Timeouts and Retries
Sometimes, a URL may not be reachable due to a timeout. Implementing retry mechanisms or setting a reasonable timeout can mitigate issues related to transient connectivity.
Example:
import java.net.HttpURLConnection;
import java.net.URL;
public class TimeoutExample {
public static void main(String[] args) {
try {
String url = "https://example.com/api";
HttpURLConnection connection = (HttpURLConnection) new URL(url).openConnection();
connection.setConnectTimeout(5000); // 5 seconds timeout
connection.setReadTimeout(5000); // 5 seconds read timeout
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
System.out.println("Connection successful!");
} else {
System.out.println("Failed to connect: " + responseCode);
}
} catch (Exception e) {
System.out.println("Error during connection: " + e.getMessage());
}
}
}
Why this matters: Setting appropriate timeout values ensures that your application is responsive and user-friendly, even when network conditions are unpredictable.
Wrapping Up
Managing URLs in Java can be quite straightforward, but overlooking minor details can turn into complex problems. By understanding these common argument issues and adopting best practices, you will enhance the robustness of your web applications.
Additional Resources
For further reading, consider checking these links:
- Java Networking Basics
- Understanding URLs and Their Components
By implementing the solutions discussed in this post and continuously learning about URL handling in Java, you will position yourself for success in web development. Remember, the devil is in the details!