Unlocking ConcurrentLinkedHashMap v1.0.1: Key Issues Solved!
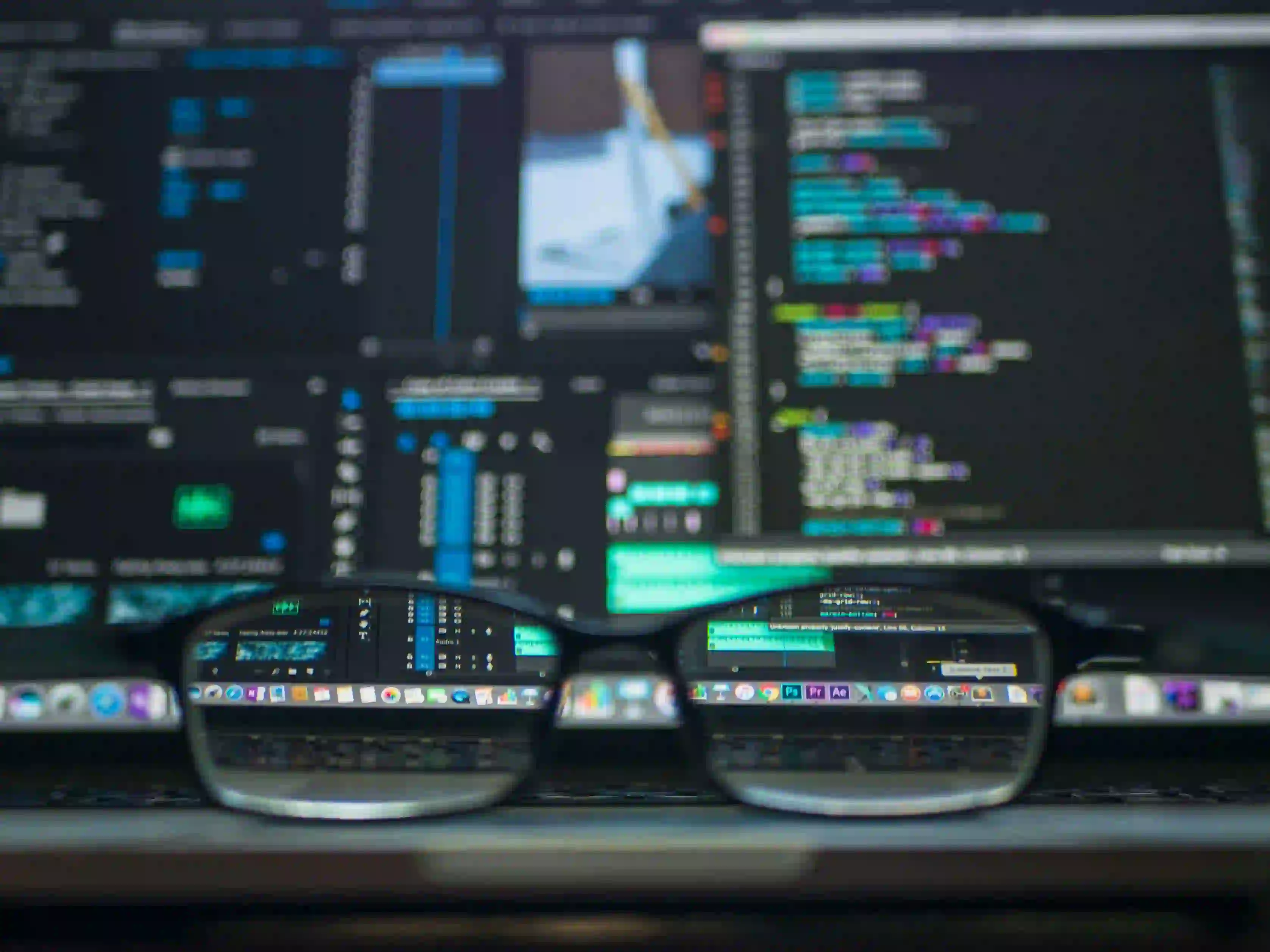
Unlocking ConcurrentLinkedHashMap v1.0.1: Key Issues Solved!
Managing collections in a concurrent environment can be a daunting task for developers. The need for thread-safe collections has led to various solutions, one of which is the ConcurrentLinkedHashMap
. This article dives deep into version 1.0.1, highlighting its key improvements and addressing common issues faced in previous versions.
Understanding ConcurrentLinkedHashMap
Before we delve into the specific improvements of version 1.0.1, it's essential to understand what a ConcurrentLinkedHashMap
is. It is a scalable, high-performance, thread-safe map that allows concurrent access by multiple threads. Unlike traditional HashMap
, which is not synchronized, ConcurrentLinkedHashMap
is built to support high-concurrency environments with efficient performance characteristics.
Why Use ConcurrentLinkedHashMap?
- Thread Safety: It provides a safe way for multiple threads to read from and write to the map concurrently.
- Performance: It offers better performance than synchronized collections like
Hashtable
andCollections.synchronizedMap()
. - Memory Efficiency: The design allows for significant memory savings when managing large datasets.
Key Improvements in v1.0.1
The release of ConcurrentLinkedHashMap v1.0.1 addresses several significant issues that previous versions faced:
- Reduced Memory Footprint: The new implementation optimizes memory usage, which is crucial for applications managing large volumes of data.
- Enhanced Concurrency Support: Improvements in concurrency control mechanisms reduce the chances of contention during updates.
- Bug Fixes: Several bugs that led to unexpected behavior in earlier versions have been rectified.
These improvements result in a more robust, performant, and reliable collection for developers aiming to manage data in multi-threaded environments effectively.
Installation
To get started with ConcurrentLinkedHashMap, you can include it in your Maven project as follows:
<dependency>
<groupId>com.googlecode.concurrentlinkedhashmap</groupId>
<artifactId>concurrentlinkedhashmap</artifactId>
<version>1.0.1</version>
</dependency>
If you're using Gradle, add the following line to your dependencies:
implementation 'com.googlecode.concurrentlinkedhashmap:concurrentlinkedhashmap:1.0.1'
Basic Operations
To get a better understanding of ConcurrentLinkedHashMap
, let’s look at some basic operations, including insertion, retrieval, and removal.
Code Snippet: Basic Usage
import com.google.common.collect.ConcurrentLinkedHashMap;
import java.util.concurrent.ExecutionException;
public class LinkedHashMapExample {
public static void main(String[] args) {
// Creating a ConcurrentLinkedHashMap
ConcurrentLinkedHashMap<String, String> map = new ConcurrentLinkedHashMap.Builder<String, String>()
.maximumWeightedCap(100)
.build();
// Inserting key-value pairs
map.put("Key1", "Value1");
map.put("Key2", "Value2");
// Retrieving a value
String value = map.get("Key1");
System.out.println("Retrieved Value: " + value);
// Removing a key-value pair
map.remove("Key2");
System.out.println("Key2 removed!");
}
}
Explanation of the Code
Be sure to take special note of the following:
- Builder Pattern: The
ConcurrentLinkedHashMap.Builder
allows you to create a map with configurations on the maximum capacity. - Map Operations: Basic operations like
put
,get
, andremove
are performed similarly to a regularMap
interface. However, these operations are thread-safe.
Common Issues Addressed in v1.0.1
1. Memory Usage
Earlier versions often faced complaints regarding memory usage, especially in high-concurrency scenarios. The v1.0.1 implementation optimizes how mappings are stored, significantly reducing the memory footprint.
2. Concurrency Problems
In multi-threaded environments, contention can significantly degrade performance. Users previously complained about slowdowns during updates and retrievals. With version 1.0.1, these performance problems have been contained, allowing for smoother concurrent access.
3. Unexpected Behavior Fixes
Bugs are a natural part of software development. Version 1.0.1 successfully resolves several issues like NullPointerExceptions and concurrent modifications that could lead to inconsistent states.
Advanced Features
The ConcurrentLinkedHashMap v1.0.1 offers several advanced features that can further enhance your application:
Cache Eviction
Using the maximumWeightedCap
method allows you to manage memory by automatically evicting entries based on their weightings. This is particularly useful in cache implementations where older or less frequently accessed entries are removed to make room for new data.
Bulk Operations
For extensive data processing, bulk operations can be a more efficient approach. There are ways to insert or remove multiple entries at once, minimizing the overhead involved in multiple calls.
Best Practices
To make the most out of ConcurrentLinkedHashMap, consider these best practices:
- Fine-Tune the Maximum Capacity: Adjust the
maximumWeightedCap
according to your application's requirements. - Use Concurrent Data Structures: When working with collections, favor concurrent data structures to avoid threading issues.
- Always Test for Concurrency: Ensure thorough testing in multi-threaded environments to catch bugs early.
Wrapping Up
The release of ConcurrentLinkedHashMap v1.0.1 marks a significant milestone in offering developers an efficient, reliable, and thread-safe map implementation. With enhancements around memory management, concurrency handling, and bug fixes, this version stands as a go-to collection for modern Java applications.
For further details on the ConcurrentLinkedHashMap, consider checking out The Javaâ„¢ Tutorials and the Java Concurrency in Practice book, both of which provide excellent insights into working with collections and concurrent programming in Java.
Unlock the full potential of your applications with ConcurrentLinkedHashMap v1.0.1 today!