Troubleshooting Java's Project Amber Exposures: A Guide
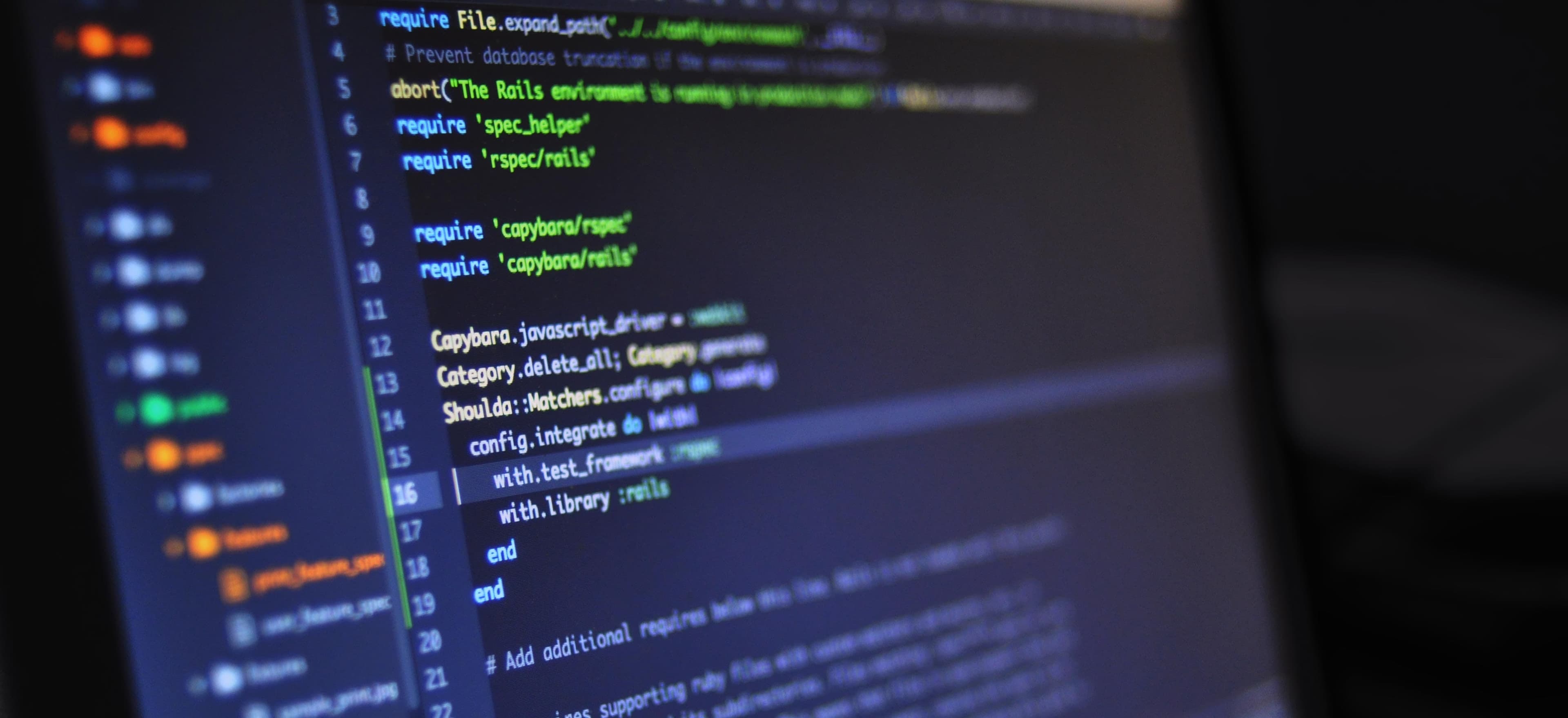
- Published on
Troubleshooting Java's Project Amber Exposures: A Guide
Java has continually made strides toward modernizing its syntax and easing developer workloads. Among various initiatives, Project Amber stands out by introducing new language features aimed at improving developer productivity. However, despite its advancements, issues can arise. This guide will delve into common problems encountered when working with the features introduced by Project Amber, equipping you with troubleshooting strategies and offering best practices.
What is Project Amber?
Project Amber is an exciting ongoing effort in the Java ecosystem that aims to enhance the language's capabilities, offering features such as pattern matching, records, and new syntax enhancements. The core goal is to simplify Java’s syntax, reduce boilerplate code, and provide more powerful abstractions. For an in-depth look at the project's goals, visit the official OpenJDK Project Amber page.
Why Troubleshooting Matters
With every new feature comes a learning curve and the potential for confusion. As developers embrace these enhancements, troubleshooting becomes essential to maintain productivity and ensure code quality. Common issues may stem from configuration errors, understanding new language features, or integrating them with existing codebases. This guide will focus on some prevalent pain points and provide insights to help you navigate them effectively.
Common Issues and Solutions
1. Configuring the Java Development Kit (JDK)
Problem:
Developers often face difficulties configuring the correct version of the JDK that supports Project Amber features. Some features are only available in the latest JDK releases.
Solution:
To resolve this, first, ensure you're using JDK 19 or later, as many of Project Amber's features were introduced in JDK 16 and expanded in subsequent versions.
You can check your JDK version via the command line:
java -version
If you're not on the required version, download the latest JDK from the Oracle JDK or Adoptium website.
Key Tip:
Ensure your IDE is set to use the correct JDK version. In IntelliJ IDEA, navigate to File > Project Structure
and confirm the Project SDK is set to the appropriate version.
2. Syntax Errors with Records
Problem:
When using records, a common issue is misunderstanding their immutable nature, leading to unexpected compilation errors.
Explanation:
Records provide a compact syntax for data classes but are immutable by design. Trying to modify the fields directly after instantiation results in compilation errors.
Here’s an example of defining a record:
public record Person(String name, int age) { }
// Initialization
Person person = new Person("Alice", 30);
// Attempting to modify results in an error
// person.age = 31; // This line will cause a compilation error
Solution:
To “modify” a record, you should create a new instance with the desired changes:
Person updatedPerson = new Person(person.name(), 31);
This approach emphasizes the immutability aspect of records, allowing for safer thread management and easier state handling.
3. Pattern Matching Problems
Problem:
Pattern matching for instanceof
can be confusing, particularly for developers accustomed to traditional type-checking mechanisms.
Explanation:
With pattern matching, you can streamline the type-checking process, making the code more readable. Here's a common pitfall:
Object obj = "Java";
if (obj instanceof String) {
String str = (String) obj; // Traditional casting
System.out.println(str.toUpperCase());
}
While this works, it’s cumbersome.
Solution:
Utilize pattern matching to eliminate the need for explicit casting:
if (obj instanceof String str) {
System.out.println(str.toUpperCase()); // Clean and concise
}
This pattern enhances code readability and reduces clutter, aligning with the overarching goal of Project Amber to reduce boilerplate code.
4. Sealed Classes and Interface Usage
Problem:
Developers may struggle with defining and implementing sealed classes, especially when trying to extend them in a non-permitted way.
Explanation:
Sealed classes restrict which classes can extend or implement them, providing more control over the inheritance hierarchy. Here’s how you would define a sealed class:
public sealed class Shape permits Circle, Square { }
public non-sealed class Circle extends Shape { }
Trying to extend Shape
from a class that is not on the permits list will lead to a compilation error.
Solution:
Always ensure that any subclass you create is listed in the permits
clause. This encourages better architecture design and clearer API contracts.
Tips for Effective Troubleshooting:
-
Enable Detailed Logging: Utilize Java’s built-in logging features or libraries like SLF4J to capture detailed logs. These can provide insights into runtime issues that might not appear during compile time.
-
Refer to Documentation: The official Java documentation is a valuable resource for clarifying the nuances of new features.
-
Join Community Forums: Engage with Java communities on platforms like Stack Overflow or Reddit. Often, peers share similar issues that you can learn from.
-
Experiment in Isolation: When encountering a problematic syntax or feature, create a small, isolated project to experiment. This can help identify issues without the complexity of a larger codebase.
-
Utilize IDE Features: Leverage your IDE's error-checking features, such as IntelliSense in IntelliJ or Eclipse, which can provide hints and suggestions based on syntax.
The Bottom Line
Embracing Project Amber's advanced features presents a unique opportunity for developers to enhance their Java applications more efficiently. However, it's essential to navigate these new tools with an informed approach. By understanding common pitfalls and employing the troubleshooting strategies outlined in this guide, you can maximize your Java development experience.
For more information on the features and improvements brought by Project Amber, consider exploring the resources linked throughout this article. Remember, the Java ecosystem is vast, and the community is here to support you!
Happy coding!