Common Pitfalls in Spring Dependency Injection
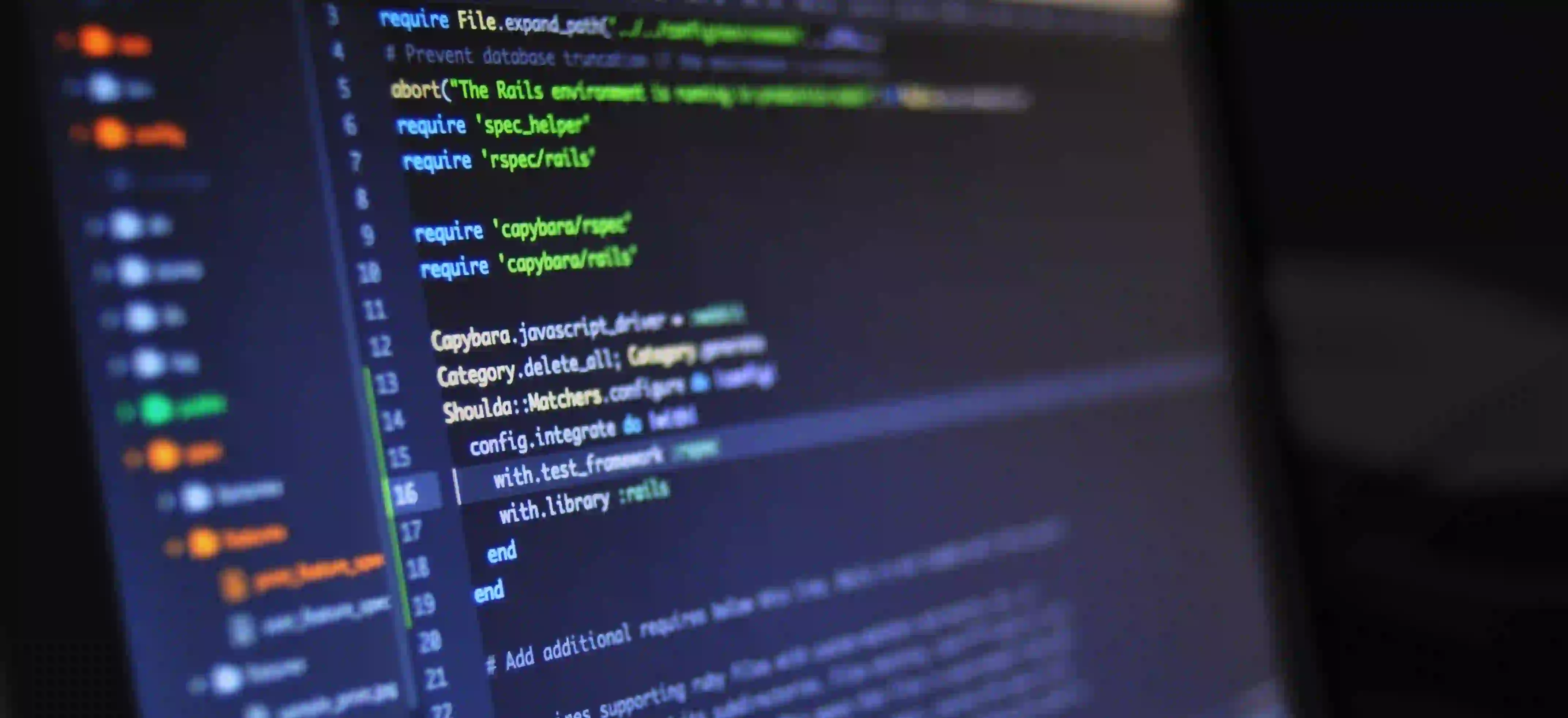
Common Pitfalls in Spring Dependency Injection
Spring Framework is a powerful tool for Java developers, with its dependency injection (DI) feature being one of its core advantages. Dependency Injection promotes loose coupling and enhances testability in your code. However, there are common pitfalls developers encounter when using Spring's DI. In this blog post, we'll explore these pitfalls, explain why they are problematic, and provide solutions with code examples.
What is Dependency Injection?
Before diving into the specifics, let’s clarify what dependency injection is. At its core, dependency injection is a design pattern that allows a class to receive its dependencies from an external source rather than creating them internally.
This pattern offers several benefits:
- Decoupling: Classes are not tightly bound to their dependencies.
- Easier Testing: Mocking dependencies becomes straightforward in unit tests.
- Configuration Flexibility: Dependencies can be modified without changing the class itself.
For more about Spring DI, refer to the official Spring documentation.
Common Pitfalls of Spring Dependency Injection
Now let’s delve into some of the common pitfalls in Spring Dependency Injection.
1. Not Understanding Bean Lifecycle
In Spring, every object managed by the Spring container is a Spring bean. These beans have lifecycle methods that are important for their management.
The Pitfall
Developers can forget to configure or understand the lifecycle methods, leading to unexpected behavior. For instance, using the @PostConstruct
annotation improperly can lead to issues if the method relies on other beans that are not yet initialized.
The Solution
Always be aware of the lifecycle stages: creation, initialization, and destruction. Utilize @PostConstruct
and @PreDestroy
for lifecycle callbacks.
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
public class MyBean {
public MyBean() {
System.out.println("Constructor called");
}
@PostConstruct
public void init() {
System.out.println("Bean is going through init.");
}
@PreDestroy
public void destroy() {
System.out.println("Bean will destroy now.");
}
}
Why This Matters: Understanding when these annotations are called helps avoid logic errors tied to bean initialization.
2. Misuse of Singleton Scope
Spring beans default to singleton scope, meaning only one instance of the bean is created per Spring container.
The Pitfall
Mistakenly relying on singletons when a prototype scope is needed can lead to stale state issues. If one client modifies the bean state, all other clients accessing that singleton will see the changed state.
The Solution
Carefully assess whether a bean should be singleton or prototype scoped.
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
@Component
@Scope("prototype") // Use prototype when each request needs a new instance
public class PrototypeBean {
private String name;
public PrototypeBean(String name) {
this.name = name; // Each instance can have different state.
}
}
Why This Matters: Using prototype scope ensures that each bean has its own unique state, crucial for multi-threaded environments.
3. Autowiring Confusion
One aspect of Spring DI is the use of the @Autowired
annotation, which allows Spring to resolve and inject collaborating beans.
The Pitfall
In certain cases, autowiring can lead to ambiguous wiring errors. For instance, if there are multiple beans of the same type, Spring will throw an exception.
The Solution
To resolve ambiguity, use @Qualifier
to specify which bean should be injected.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.stereotype.Component;
@Component
public class ServiceConsumer {
private final MyService service;
@Autowired
public ServiceConsumer(@Qualifier("mySpecialService") MyService service) {
this.service = service;
}
}
Why This Matters: @Qualifier
allows precise control over dependency injection, reducing runtime errors caused by ambiguity.
4. Circular Dependencies
A circular dependency occurs when two or more beans refer to each other, leading to a situation where neither can be initialized.
The Pitfall
Circular dependencies can cause errors during startup and make the codebase complex and hard to maintain.
The Solution
Refactor dependencies to eliminate circular references. One approach is through setter injection instead of constructor injection.
@Component
public class A {
private B b;
@Autowired
public void setB(B b) {
this.b = b; // Setter injection helps avoid circular dependency
}
}
@Component
public class B {
private A a;
@Autowired
public void setA(A a) {
this.a = a;
}
}
Why This Matters: Opting for setter injection allows Spring to create a proxy, thereby managing the circular reference correctly.
5. Heavy Use of @ComponentScan
@ComponentScan
is a powerful Spring feature that automatically detects and registers beans. However, using it indiscriminately can create clutter.
The Pitfall
Overusing @ComponentScan
can lead to unnecessary beans being loaded, increasing memory usage and possibly leading to conflicts.
The Solution
Use @ComponentScan
with a specific base package to limit the scope of scanning.
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
@Configuration
@ComponentScan(basePackages = "com.myapp.services") // Specify only necessary packages
public class AppConfig {
}
Why This Matters: Targeted component scanning helps control application complexity and resource utilization.
6. Ignoring Exception Handling
Spring's dependency injection may throw exceptions during runtime, especially related to bean creation.
The Pitfall
Ignoring exception handling can lead to uncaught exceptions crashing the application, making debugging difficult.
The Solution
Add appropriate exception handling within configuration classes and during bean initialization.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class ConfigurableBean {
private final Dependency dependency;
@Autowired
public ConfigurableBean(Dependency dependency) {
this.dependency = dependency;
if (this.dependency == null) {
throw new IllegalArgumentException("Dependency cannot be null");
}
}
}
Why This Matters: Proper exception handling improves stability and predictability, making it easier to manage failures.
The Closing Argument
Spring Dependency Injection simplifies dependency management, but it is not without its challenges. Understanding the common pitfalls, such as bean lifecycle intricacies, mismanaged scopes, confusion with autowiring, circular dependencies, excessive component scanning, and exception handling is crucial for smooth development.
Whether you are new to Spring or a seasoned developer, keeping these pitfalls in mind can lead to cleaner, more maintainable code. For a more in-depth look, consider browsing the Spring Framework documentation.
Remember, a well-understood dependency injection framework can greatly enhance your application's structure and functionality. Happy coding!