Efficiently Concatenating Strings in Java: A Quick Guide
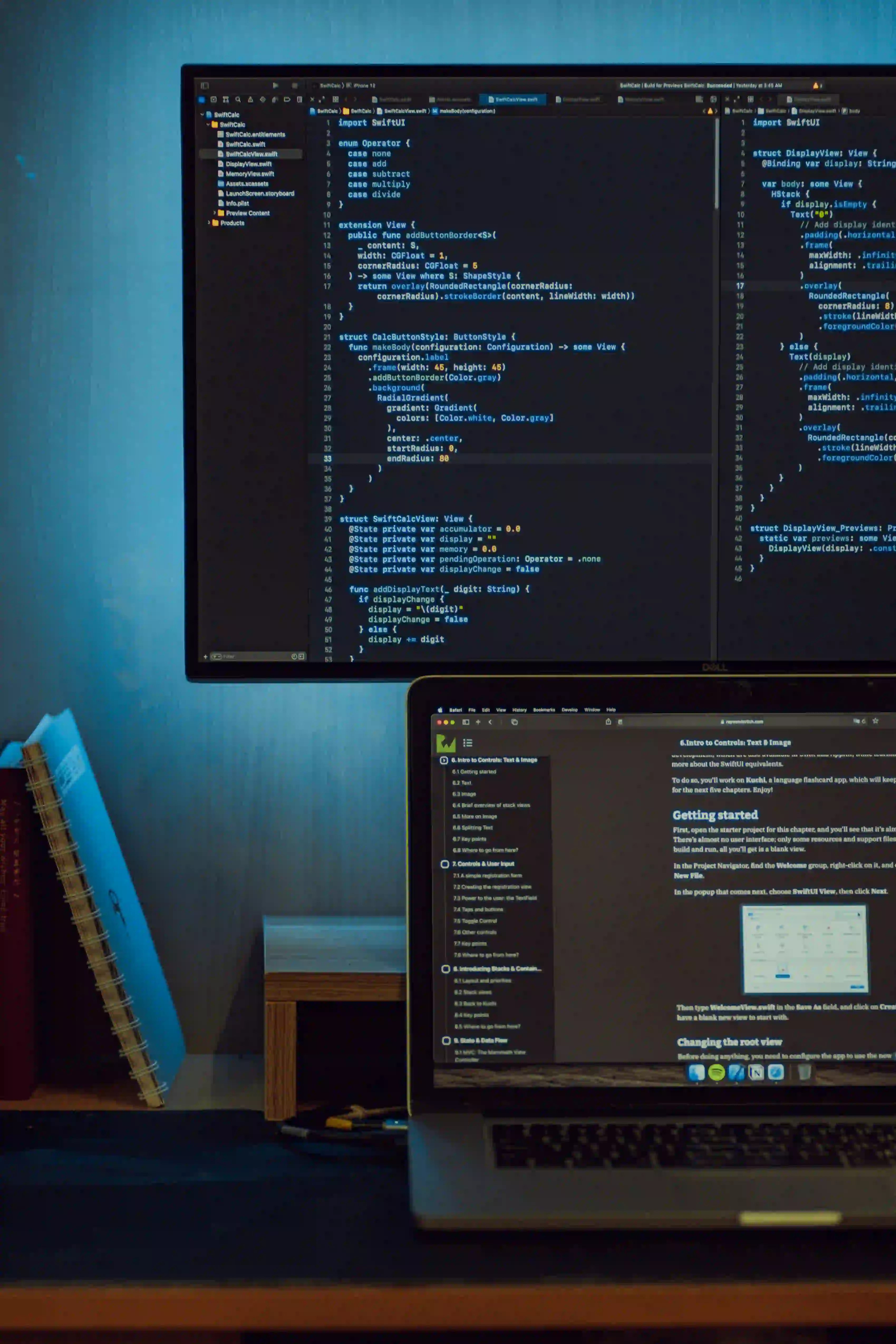
Efficiently Concatenating Strings in Java: A Quick Guide
In Java, string manipulation is an essential skill for any developer. One common operation developers perform is concatenating strings. As simple as it sounds, there are various methods to concatenate strings, each with its implications for performance and memory usage. This post will explore several ways to concatenate strings in Java, evaluating their efficiency and use cases.
Understanding Strings in Java
Before delving into concatenation techniques, let's clarify how Java handles strings. Strings in Java are immutable, meaning they cannot be changed once created. This immutability is a fundamental design choice that enhances security and performance but also presents a challenge when concatenating.
When you concatenate strings using the +
operator, Java doesn't modify the original strings. Instead, it creates a new string object. This can lead to performance issues, especially within loops where numerous concatenations are required.
Basic String Concatenation with the +
Operator
The simplest way to concatenate strings is by using the +
operator. Here's an example:
String firstName = "John";
String lastName = "Doe";
String fullName = firstName + " " + lastName;
System.out.println(fullName); // Output: John Doe
Why use the +
operator?
- Simplicity: It is easy to read and understand.
- Conciseness: Great for concatenating a small number of strings.
However, for large-scale operations, this approach can lead to excessive memory usage and slower performance.
Using the StringBuilder
Class
For scenarios involving multiple concatenation operations, consider using StringBuilder
. This class provides an efficient way to manipulate strings as it operates on a mutable sequence of characters.
Here's how to use it:
StringBuilder sb = new StringBuilder();
sb.append("John");
sb.append(" ");
sb.append("Doe");
String fullName = sb.toString();
System.out.println(fullName); // Output: John Doe
Why use StringBuilder?
- Performance: Modifying the contents of a
StringBuilder
does not create new string objects, which saves on memory and improves speed. - Flexibility: You can append, insert, and delete without worrying about string immutability.
For extensive string manipulation or when you expect to concatenate a significant number of strings, StringBuilder
is often the best choice.
Using the StringBuffer
Class
Similar to StringBuilder
, StringBuffer
is another class for concatenating strings. The key difference? StringBuffer
is synchronized, making it thread-safe.
Here’s an example:
StringBuffer sb = new StringBuffer();
sb.append("John");
sb.append(" ");
sb.append("Doe");
String fullName = sb.toString();
System.out.println(fullName); // Output: John Doe
Why use StringBuffer?
- Thread Safety: If multiple threads are accessing and modifying the string,
StringBuffer
provides a safer way to perform concatenation.
When to use which?
- Use
StringBuilder
for single-threaded applications. - Use
StringBuffer
when multiple threads need to access the same string concurrently.
Performance Test: Comparing Concatenation Techniques
To truly appreciate the differences between these methods, let’s conduct a performance benchmark.
public class StringConcatenationPerformance {
public static void main(String[] args) {
long startTime;
long endTime;
// Using + operator
startTime = System.currentTimeMillis();
String result1 = "";
for (int i = 0; i < 10000; i++) {
result1 += "Hello ";
}
endTime = System.currentTimeMillis();
System.out.println("Time taken using + : " + (endTime - startTime) + "ms");
// Using StringBuilder
startTime = System.currentTimeMillis();
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 10000; i++) {
sb.append("Hello ");
}
String result2 = sb.toString();
endTime = System.currentTimeMillis();
System.out.println("Time taken using StringBuilder : " + (endTime - startTime) + "ms");
// Using StringBuffer
startTime = System.currentTimeMillis();
StringBuffer sbf = new StringBuffer();
for (int i = 0; i < 10000; i++) {
sbf.append("Hello ");
}
String result3 = sbf.toString();
endTime = System.currentTimeMillis();
System.out.println("Time taken using StringBuffer : " + (endTime - startTime) + "ms");
}
}
Observations
When you run the program above, you will typically find that the +
operator performs significantly slower than StringBuilder
and StringBuffer
, especially in large loops. The difference magnifies with increasing string operations, illustrating the importance of choosing the right method for string concatenation.
Alternative Method: Using Streams (Java 8 and Above)
An elegant and modern way to concatenate strings is using the Collectors.joining()
method introduced in Java 8. This method is particularly useful when you have a collection of strings.
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamConcatenationExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Doe");
String result = names.stream()
.collect(Collectors.joining(", "));
System.out.println(result); // Output: John, Jane, Doe
}
}
Why use Streams?
- Readability: It provides a clear and concise way to combine strings, especially when working with collections.
- Functional Style: Aligns with Java’s move towards functional programming constructs.
The Last Word
String concatenation in Java may appear straightforward, but it’s crucial to understand the underlying mechanics to make the best choice for your specific use case. While the +
operator is convenient for short concatenations, StringBuilder
and StringBuffer
are superior for the performance of larger strings. Moreover, Java Streams provide a modern, elegant approach for handling collections of strings.
For additional reading about Java strings, you can refer to the Java documentation.
Remember, the choice of concatenation method can significantly impact the performance of your Java applications. Always profile your code to make informed decisions.
By employing the right methods for string concatenation, you can ensure efficient and clean code in your Java applications. Happy coding!