Top 5 Tips to Reduce Java Memory Usage in Your Programs
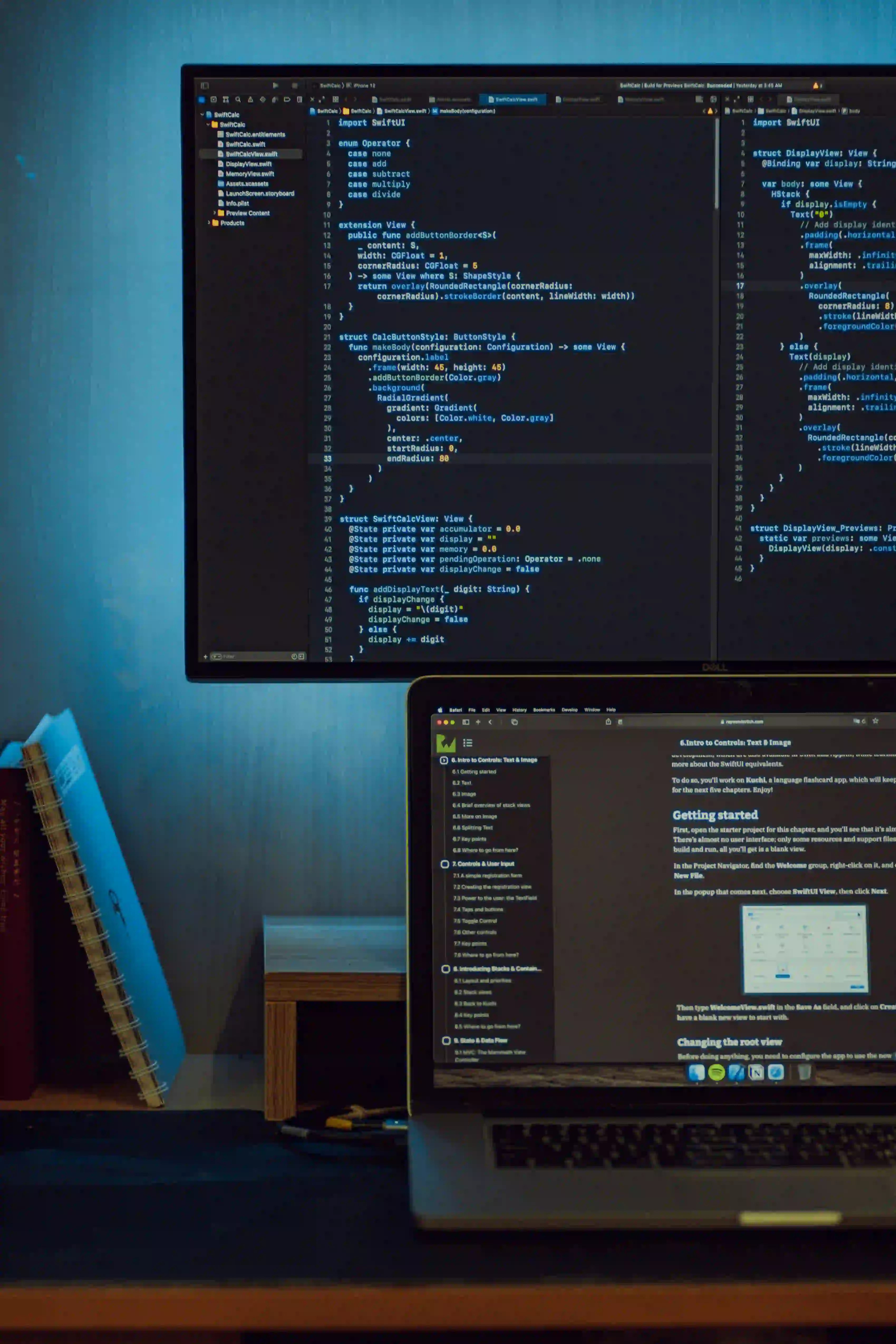
Top 5 Tips to Reduce Java Memory Usage in Your Programs
In the world of software development, memory management is a critical concern, particularly when working with languages like Java. Efficient memory usage not only optimizes performance but also enhances the user experience. Too much memory consumption can lead to slow applications or even crashes, particularly when handling extensive datasets. Here, we'll explore five essential tips to reduce Java memory usage in your programs—each with practical code examples and detailed explanations.
1. Choose the Right Data Structures
Choosing the appropriate data structures can significantly impact memory consumption. Java provides several data structures, each with different memory footprints. For example, ArrayList
consumes more memory than an Array
.
Why Array Over ArrayList?
- An array has a fixed size and allocates memory only for what it needs. In contrast,
ArrayList
maintains overhead for dynamic resizing.
Example: Array versus ArrayList
// Using an Array
int[] numbers = new int[5]; // Allocates memory for 5 integers
// Using an ArrayList
List<Integer> numberList = new ArrayList<>(); // Initial capacity starts at 10 by default
By opting for arrays, you can limit memory wastage. Choose data structures that fit your application context and ensure to minimize overhead.
Additional Resource:
For more information on Java Collections, refer to the official documentation here.
2. Minimize Object Creation
Creating unnecessary objects leads to increased memory usage and frequent garbage collection. Reusing objects can mitigate this problem.
Why Avoid Unnecessary Object Creation?
- Each object in Java consumes memory. If multiple instances of a class aren't necessary, you can significantly reduce memory consumption by reusing them.
Example: Object Reuse
public class User {
private String name;
// Constructor
public User(String name) {
this.name = name;
}
}
// Create a single instance
User user1 = new User("Alice");
// Instead of creating a new User for similar purpose:
User user2 = user1; // Reuse the existing instance
By reusing objects as shown, you can keep the memory footprint low. Consider using design patterns like Singleton for classes that should only have one instance.
Additional Resource:
Learn more about design patterns here.
3. Use Primitive Types Instead of Wrapper Classes
Java provides wrapper classes (e.g., Integer
, Double
, Character
) for primitive types. However, these wrappers consume more memory than their primitive counterparts due to additional functionalities and overhead.
Why Use Primitive Types?
- Primitive data types are more memory efficient. For instance, an
int
consumes 4 bytes, whereas anInteger
object consumes memory for the 4 bytes used by theint
, plus additional overhead for the object itself.
Example: Primitive vs. Wrapper
// Using Primitive Type
int age = 30;
// Using Wrapper Class
Integer ageWrapper = new Integer(30); // More memory usage
Using primitives can drastically reduce memory usage, especially in large arrays or loops. Always prefer primitive types when the added features of the wrapper class are not necessary.
Additional Resource:
For more versatile understanding, check out Primitive Data Types in Java.
4. Optimize String Usage
In Java, String
objects are immutable, meaning that every modification creates a new String
instance. This can be expensive in terms of memory when dealing with large numbers of string manipulations.
Why Optimize Strings?
- String concatenation using the
+
operator can lead to excessive memory usage. Instead, consider usingStringBuilder
orStringBuffer
, which allows mutable string manipulation.
Example: String Concatenation vs. StringBuilder
// Using String Concatenation
String str = "Hello";
str += " World"; // Creates a new String object
// Using StringBuilder
StringBuilder sb = new StringBuilder("Hello");
sb.append(" World"); // Modifies existing object
Using StringBuilder
, you manipulate strings more memory efficiently, especially beneficial in loops or large-scale string operations.
Additional Resource:
For deeper insights on String manipulation, visit this Java String Basics.
5. Enable Garbage Collection Tuning
Java's garbage collector (GC) automatically recycles memory by removing objects that are no longer in use. However, you can influence its behavior through proper tuning.
Why Tune GC?
- An improperly tuned garbage collector may lead to excessive memory usage or frequent pauses in application execution. Different GC algorithms and settings suit different applications.
Example: Setting Up Garbage Collector Options
You can set JVM options to choose a specific garbage collector:
java -XX:+UseG1GC -Xms512m -Xmx1024m -jar myJavaApp.jar
-XX:+UseG1GC
enables the G1 garbage collector.-Xms512m
sets the initial heap size.-Xmx1024m
sets the maximum heap size.
Tuning these parameters according to your application's needs can lead to reduced memory consumption and improved performance.
Additional Resource:
To learn more about garbage collection, visit the Oracle Garbage Collection Tuning Guide.
Key Takeaways
Reducing memory usage in Java programs is essential for optimal performance. By choosing the right data structures, minimizing object creation, using primitive types, optimizing String usage, and tuning garbage collection settings, you can significantly enhance your application's efficiency.
Memory management may seem complex, but with these five strategies, you can take substantial strides toward a more efficient Java program. Implementing these tips will not only improve performance but will also lead to a more responsive and stable application for your users.
For deeper insights into Java performance and memory optimization techniques, continue exploring platforms like Baeldung, and ensure your Java applications are running at peak performance!
Happy coding!